Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Activities / Configuration / WorkflowIdleElement.cs / 1305376 / WorkflowIdleElement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activities.Configuration { using System.ComponentModel; using System.Configuration; using System.Globalization; using System.Runtime; using System.ServiceModel.Activities.Description; using System.ServiceModel.Configuration; public sealed class WorkflowIdleElement : BehaviorExtensionElement { ConfigurationPropertyCollection properties; const string TimeToPersistString = "timeToPersist"; const string TimeToUnloadString = "timeToUnload"; public WorkflowIdleElement() { } [ConfigurationProperty(TimeToPersistString, DefaultValue = WorkflowIdleBehavior.defaultTimeToPersistString)] [TypeConverter(typeof(TimeSpanOrInfiniteConverter))] [ServiceModelTimeSpanValidator(MinValueString = ConfigurationStrings.TimeSpanZero)] public TimeSpan TimeToPersist { get { return (TimeSpan)base[TimeToPersistString]; } set { base[TimeToPersistString] = value; } } [ConfigurationProperty(TimeToUnloadString, DefaultValue = WorkflowIdleBehavior.defaultTimeToUnloadString)] [TypeConverter(typeof(TimeSpanOrInfiniteConverter))] [ServiceModelTimeSpanValidator(MinValueString = ConfigurationStrings.TimeSpanZero)] public TimeSpan TimeToUnload { get { return (TimeSpan)base[TimeToUnloadString]; } set { base[TimeToUnloadString] = value; } } protected internal override object CreateBehavior() { return new WorkflowIdleBehavior() { TimeToPersist = this.TimeToPersist, TimeToUnload = this.TimeToUnload }; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Configuration", "Configuration102:ConfigurationPropertyAttributeRule", MessageId = "System.ServiceModel.Activities.Configuration.WorkflowIdleElement.BehaviorType", Justification = "Not a configurable property; a property that had to be overridden from abstract parent class")] public override Type BehaviorType { get { return typeof(WorkflowIdleBehavior); } } protected override ConfigurationPropertyCollection Properties { get { if (this.properties == null) { ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); properties.Add(new ConfigurationProperty(TimeToPersistString, typeof(TimeSpan), TimeSpan.MaxValue, new TimeSpanOrInfiniteConverter(), new TimeSpanOrInfiniteValidator(TimeSpan.Zero, TimeSpan.MaxValue), ConfigurationPropertyOptions.None)); properties.Add(new ConfigurationProperty(TimeToUnloadString, typeof(TimeSpan), TimeSpan.Parse(WorkflowIdleBehavior.defaultTimeToUnloadString, CultureInfo.InvariantCulture), new TimeSpanOrInfiniteConverter(), new TimeSpanOrInfiniteValidator(TimeSpan.Zero, TimeSpan.MaxValue), ConfigurationPropertyOptions.None)); this.properties = properties; } return this.properties; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
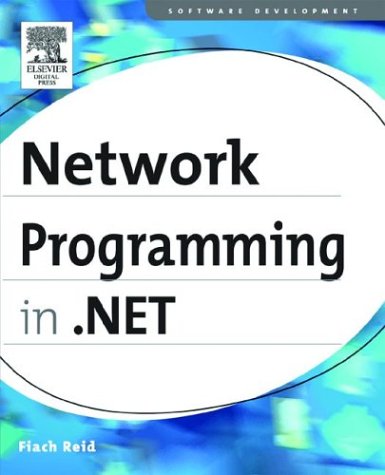
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WSFederationHttpBindingCollectionElement.cs
- WindowsFont.cs
- Imaging.cs
- VScrollBar.cs
- MapPathBasedVirtualPathProvider.cs
- RestHandler.cs
- MenuStrip.cs
- RemotingAttributes.cs
- ListDictionary.cs
- PermissionToken.cs
- ConnectionConsumerAttribute.cs
- ActivityCodeDomReferenceService.cs
- _NetworkingPerfCounters.cs
- TemplateApplicationHelper.cs
- PageCache.cs
- ScriptResourceHandler.cs
- BinaryWriter.cs
- SmtpCommands.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- WaitForChangedResult.cs
- ErrorsHelper.cs
- Subtree.cs
- XmlSchemaComplexType.cs
- CodeDirectiveCollection.cs
- MiniParameterInfo.cs
- ChangePassword.cs
- PrintController.cs
- UserPreferenceChangingEventArgs.cs
- documentation.cs
- DataGridClipboardHelper.cs
- ConfigurationElement.cs
- DataList.cs
- WpfMemberInvoker.cs
- QilBinary.cs
- DataTableMapping.cs
- FlowDocumentPageViewerAutomationPeer.cs
- ThaiBuddhistCalendar.cs
- SeverityFilter.cs
- TextParagraph.cs
- IsolatedStorageException.cs
- Baml2006ReaderFrame.cs
- linebase.cs
- COM2AboutBoxPropertyDescriptor.cs
- ListControlStringCollectionEditor.cs
- PerformanceCounterPermission.cs
- FormParameter.cs
- SizeFConverter.cs
- DrawingGroup.cs
- TypeConverterHelper.cs
- DataExpression.cs
- BufferBuilder.cs
- FileSystemEventArgs.cs
- RegexCode.cs
- GroupStyle.cs
- ObjectTag.cs
- MessageQueueEnumerator.cs
- BaseDataBoundControl.cs
- OrderPreservingSpoolingTask.cs
- ImageSource.cs
- Aes.cs
- EncoderParameter.cs
- QilXmlWriter.cs
- ProtocolsSection.cs
- WasHttpHandlersInstallComponent.cs
- Attributes.cs
- DataGridDesigner.cs
- ViewBox.cs
- SchemaCollectionPreprocessor.cs
- MdiWindowListItemConverter.cs
- CustomErrorsSection.cs
- RuleSettingsCollection.cs
- Stack.cs
- LogicalTreeHelper.cs
- CodePrimitiveExpression.cs
- XNodeSchemaApplier.cs
- LinqDataSourceEditData.cs
- ToolStripSystemRenderer.cs
- OneWayElement.cs
- NamespaceList.cs
- TextDecorationLocationValidation.cs
- ColorContext.cs
- PartManifestEntry.cs
- BitmapCodecInfoInternal.cs
- ServiceReference.cs
- BooleanSwitch.cs
- ObjectQueryExecutionPlan.cs
- SiteIdentityPermission.cs
- ApplicationInfo.cs
- DependencyObject.cs
- TreeNodeCollection.cs
- GeneratedContractType.cs
- TableLayoutSettings.cs
- FormattedTextSymbols.cs
- VirtualizingStackPanel.cs
- TextDecoration.cs
- OracleParameterBinding.cs
- PartialClassGenerationTaskInternal.cs
- CqlLexerHelpers.cs
- Rule.cs
- WindowsRichEditRange.cs