Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / Update / Internal / Propagator.JoinPropagator.SubstitutingCloneVisitor.cs / 1305376 / Propagator.JoinPropagator.SubstitutingCloneVisitor.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common; namespace System.Data.Mapping.Update.Internal { internal partial class Propagator { private partial class JoinPropagator { ////// Describes the mode of behavior for the private enum PopulateMode { ///. /// /// Produce a null extension record (for outer joins) marked as modified /// NullModified, ////// Produce a null extension record (for outer joins) marked as preserve /// NullPreserve, ////// Produce a placeholder for a record that is known to exist but whose specific /// values are unknown. /// Unknown, } ////// Fills in a placeholder with join key data (also performs a clone so that the /// placeholder can be reused). /// ////// Clones of placeholder nodes are created when either the structure of the node /// needs to change or the record markup for the node needs to change. /// private static class PlaceholderPopulator { #region Methods ////// Construct a new placeholder with the shape of the given placeholder. Key values are /// injected into the resulting place holder and default values are substituted with /// either propagator constants or progagator nulls depending on the mode established /// by the ///flag. /// /// The key is essentially an array of values. The key map indicates that for a particular /// placeholder an expression (keyMap.Keys) corresponds to some ordinal in the key array. /// /// Placeholder to clone /// Key to substitute /// Key elements in the placeholder (ordinally aligned with 'key') /// Mode of operation. /// Translator context. ///Cloned placeholder with key values internal static PropagatorResult Populate(PropagatorResult placeholder, CompositeKey key, CompositeKey placeholderKey, PopulateMode mode, UpdateTranslator translator) { EntityUtil.CheckArgumentNull(placeholder, "placeholder"); EntityUtil.CheckArgumentNull(key, "key"); EntityUtil.CheckArgumentNull(placeholderKey, "placeholderKey"); EntityUtil.CheckArgumentNull(translator, "translator"); // Figure out which flags to apply to generated elements. bool isNull = mode == PopulateMode.NullModified || mode == PopulateMode.NullPreserve; bool preserve = mode == PopulateMode.NullPreserve || mode == PopulateMode.Unknown; PropagatorFlags flags = PropagatorFlags.NoFlags; if (!isNull) { flags |= PropagatorFlags.Unknown; } // only null values are known if (preserve) { flags |= PropagatorFlags.Preserve; } PropagatorResult result = placeholder.Replace(node => { // See if this is a key element int keyIndex = -1; for (int i = 0; i < placeholderKey.KeyComponents.Length; i++) { if (placeholderKey.KeyComponents[i] == node) { keyIndex = i; break; } } if (keyIndex != -1) { // Key value. return key.KeyComponents[keyIndex]; } else { // for simple entries, just return using the markup context for this // populator object value = isNull ? null : node.GetSimpleValue(); return PropagatorResult.CreateSimpleValue(flags, value); } }); return result; } #endregion } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common; namespace System.Data.Mapping.Update.Internal { internal partial class Propagator { private partial class JoinPropagator { ////// Describes the mode of behavior for the private enum PopulateMode { ///. /// /// Produce a null extension record (for outer joins) marked as modified /// NullModified, ////// Produce a null extension record (for outer joins) marked as preserve /// NullPreserve, ////// Produce a placeholder for a record that is known to exist but whose specific /// values are unknown. /// Unknown, } ////// Fills in a placeholder with join key data (also performs a clone so that the /// placeholder can be reused). /// ////// Clones of placeholder nodes are created when either the structure of the node /// needs to change or the record markup for the node needs to change. /// private static class PlaceholderPopulator { #region Methods ////// Construct a new placeholder with the shape of the given placeholder. Key values are /// injected into the resulting place holder and default values are substituted with /// either propagator constants or progagator nulls depending on the mode established /// by the ///flag. /// /// The key is essentially an array of values. The key map indicates that for a particular /// placeholder an expression (keyMap.Keys) corresponds to some ordinal in the key array. /// /// Placeholder to clone /// Key to substitute /// Key elements in the placeholder (ordinally aligned with 'key') /// Mode of operation. /// Translator context. ///Cloned placeholder with key values internal static PropagatorResult Populate(PropagatorResult placeholder, CompositeKey key, CompositeKey placeholderKey, PopulateMode mode, UpdateTranslator translator) { EntityUtil.CheckArgumentNull(placeholder, "placeholder"); EntityUtil.CheckArgumentNull(key, "key"); EntityUtil.CheckArgumentNull(placeholderKey, "placeholderKey"); EntityUtil.CheckArgumentNull(translator, "translator"); // Figure out which flags to apply to generated elements. bool isNull = mode == PopulateMode.NullModified || mode == PopulateMode.NullPreserve; bool preserve = mode == PopulateMode.NullPreserve || mode == PopulateMode.Unknown; PropagatorFlags flags = PropagatorFlags.NoFlags; if (!isNull) { flags |= PropagatorFlags.Unknown; } // only null values are known if (preserve) { flags |= PropagatorFlags.Preserve; } PropagatorResult result = placeholder.Replace(node => { // See if this is a key element int keyIndex = -1; for (int i = 0; i < placeholderKey.KeyComponents.Length; i++) { if (placeholderKey.KeyComponents[i] == node) { keyIndex = i; break; } } if (keyIndex != -1) { // Key value. return key.KeyComponents[keyIndex]; } else { // for simple entries, just return using the markup context for this // populator object value = isNull ? null : node.GetSimpleValue(); return PropagatorResult.CreateSimpleValue(flags, value); } }); return result; } #endregion } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
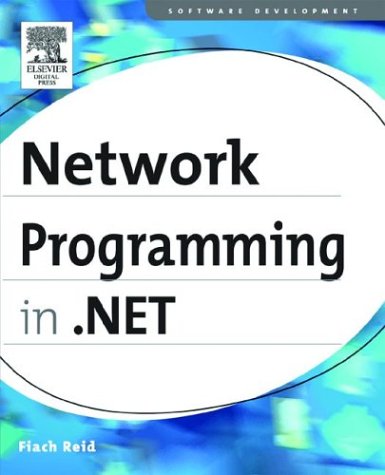
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CombinedGeometry.cs
- StrongTypingException.cs
- RecordConverter.cs
- ScrollPatternIdentifiers.cs
- ProcessStartInfo.cs
- XmlArrayAttribute.cs
- ApplicationProxyInternal.cs
- SqlDelegatedTransaction.cs
- ViewValidator.cs
- MessagePropertyAttribute.cs
- StringAttributeCollection.cs
- SqlRewriteScalarSubqueries.cs
- SystemSounds.cs
- TemplateControlCodeDomTreeGenerator.cs
- ContextInformation.cs
- XmlLoader.cs
- NumberFormatter.cs
- MatrixCamera.cs
- SqlProcedureAttribute.cs
- mongolianshape.cs
- _UncName.cs
- TokenBasedSetEnumerator.cs
- WorkflowMarkupSerializerMapping.cs
- StyleBamlRecordReader.cs
- XmlAttributeAttribute.cs
- GraphicsContainer.cs
- EncoderExceptionFallback.cs
- sqlinternaltransaction.cs
- SHA384.cs
- TextEndOfSegment.cs
- NodeLabelEditEvent.cs
- FrameworkContentElement.cs
- PerformanceCountersElement.cs
- Bidi.cs
- ValidationHelper.cs
- Clause.cs
- RadioButton.cs
- KeyBinding.cs
- SpecularMaterial.cs
- ClrProviderManifest.cs
- TemplatePropertyEntry.cs
- PageFunction.cs
- SafeNativeMethodsOther.cs
- RadioButtonAutomationPeer.cs
- TextDecorations.cs
- WebPartActionVerb.cs
- SspiHelper.cs
- ListItemConverter.cs
- ThreadStaticAttribute.cs
- ValueTypeIndexerReference.cs
- MessageBox.cs
- XmlSchemaAny.cs
- InstanceDataCollection.cs
- XmlResolver.cs
- LOSFormatter.cs
- EdmItemError.cs
- Timeline.cs
- OpenTypeLayoutCache.cs
- BindingCompleteEventArgs.cs
- DeclaredTypeValidatorAttribute.cs
- PointValueSerializer.cs
- SqlSupersetValidator.cs
- LayoutTable.cs
- HttpHandlersSection.cs
- Interop.cs
- WorkflowServiceHost.cs
- MultiTrigger.cs
- ImageButton.cs
- StorageSetMapping.cs
- KeyValueInternalCollection.cs
- BaseTemplateCodeDomTreeGenerator.cs
- FormatterConverter.cs
- Subset.cs
- SrgsElement.cs
- Int32CollectionConverter.cs
- ViewStateException.cs
- WebBrowsableAttribute.cs
- DataGridItemCollection.cs
- ProfileGroupSettings.cs
- XPathItem.cs
- KeySplineConverter.cs
- RequestResizeEvent.cs
- DelegatingStream.cs
- XPathNodeIterator.cs
- InvalidComObjectException.cs
- RuntimeHandles.cs
- RectAnimationClockResource.cs
- DropShadowBitmapEffect.cs
- RegistryHandle.cs
- InvalidDataContractException.cs
- MaskedTextProvider.cs
- EmptyTextWriter.cs
- selecteditemcollection.cs
- IsolatedStorageFileStream.cs
- ProfilePropertySettings.cs
- XmlElementAttribute.cs
- QueryParameter.cs
- _LocalDataStoreMgr.cs
- GridErrorDlg.cs
- EdmError.cs