Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / XPath / Internal / BooleanFunctions.cs / 1 / BooleanFunctions.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; using System.Xml.Xsl; using FT = MS.Internal.Xml.XPath.Function.FunctionType; internal sealed class BooleanFunctions : ValueQuery { Query arg; FT funcType; public BooleanFunctions(FT funcType, Query arg) { this.arg = arg; this.funcType = funcType; } private BooleanFunctions(BooleanFunctions other) : base(other) { this.arg = Clone(other.arg); this.funcType = other.funcType; } public override void SetXsltContext(XsltContext context) { if (arg != null) { arg.SetXsltContext(context); } } public override object Evaluate(XPathNodeIterator nodeIterator) { switch (funcType) { case FT.FuncBoolean : return toBoolean(nodeIterator); case FT.FuncNot : return Not(nodeIterator); case FT.FuncTrue : return true; case FT.FuncFalse : return false; case FT.FuncLang : return Lang(nodeIterator); } return false; } internal static bool toBoolean(double number) { return number != 0 && ! double.IsNaN(number); } internal static bool toBoolean(string str) { return str.Length > 0; } internal bool toBoolean(XPathNodeIterator nodeIterator) { object result = arg.Evaluate(nodeIterator); if (result is XPathNodeIterator) return arg.Advance() != null; if (result is string ) return toBoolean((string)result); if (result is double ) return toBoolean((double)result); if (result is bool ) return (bool)result; Debug.Assert(result is XPathNavigator, "Unknown value type"); return true; } public override XPathResultType StaticType { get { return XPathResultType.Boolean; } } private bool Not(XPathNodeIterator nodeIterator) { return ! (bool) arg.Evaluate(nodeIterator); } private bool Lang(XPathNodeIterator nodeIterator) { string str = arg.Evaluate(nodeIterator).ToString(); string lang = nodeIterator.Current.XmlLang; return ( lang.StartsWith(str, StringComparison.OrdinalIgnoreCase) && (lang.Length == str.Length || lang[str.Length] == '-') ); } public override XPathNodeIterator Clone() { return new BooleanFunctions(this); } public override void PrintQuery(XmlWriter w) { w.WriteStartElement(this.GetType().Name); w.WriteAttributeString("name", funcType.ToString()); if (arg != null) { arg.PrintQuery(w); } w.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
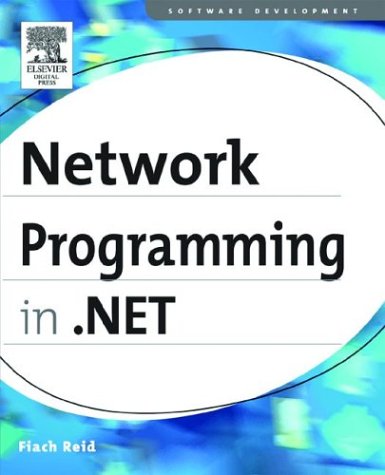
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlFlattener.cs
- UICuesEvent.cs
- TextDecorationUnitValidation.cs
- EmissiveMaterial.cs
- WebPartVerb.cs
- DrawListViewSubItemEventArgs.cs
- XmlSchemaObjectCollection.cs
- FilteredReadOnlyMetadataCollection.cs
- DataGridViewCellParsingEventArgs.cs
- DesignerSerializationVisibilityAttribute.cs
- HtmlImage.cs
- VisualProxy.cs
- ResourcePermissionBase.cs
- MemoryRecordBuffer.cs
- Base64Decoder.cs
- DeviceContext2.cs
- RotateTransform.cs
- InputDevice.cs
- XmlSchemaSequence.cs
- WmfPlaceableFileHeader.cs
- LabelTarget.cs
- Bits.cs
- SkipStoryboardToFill.cs
- PropertyRecord.cs
- StatusBar.cs
- ClientScriptManager.cs
- MinimizableAttributeTypeConverter.cs
- SelectionListComponentEditor.cs
- DataListItemEventArgs.cs
- FontDialog.cs
- ConfigurationSection.cs
- RectAnimationClockResource.cs
- ProgressBar.cs
- ExceptionAggregator.cs
- SystemTcpStatistics.cs
- HttpApplication.cs
- CategoryAttribute.cs
- ProcessModelInfo.cs
- StylusPointPropertyInfo.cs
- PropertyGridView.cs
- AssemblyResourceLoader.cs
- Int32CollectionValueSerializer.cs
- ReachSerializer.cs
- WpfMemberInvoker.cs
- CompensatableSequenceActivity.cs
- ToolStripMenuItem.cs
- XmlCollation.cs
- FileChangesMonitor.cs
- ObjectNotFoundException.cs
- AssociationSetEnd.cs
- HtmlButton.cs
- ToolBarOverflowPanel.cs
- GeneratedView.cs
- ProfessionalColors.cs
- TransactionScope.cs
- SafeTimerHandle.cs
- CqlGenerator.cs
- SelectionProcessor.cs
- InvalidProgramException.cs
- EdmError.cs
- MDIWindowDialog.cs
- HyperLinkField.cs
- StylusLogic.cs
- DirectoryNotFoundException.cs
- ProxyWebPart.cs
- XmlArrayItemAttributes.cs
- ManagementBaseObject.cs
- ObjectStateManager.cs
- TabControlDesigner.cs
- ExtendedPropertyDescriptor.cs
- ChannelPoolSettings.cs
- ConfigurationFileMap.cs
- IRCollection.cs
- GeometryGroup.cs
- IBuiltInEvidence.cs
- VectorKeyFrameCollection.cs
- embossbitmapeffect.cs
- Inline.cs
- PartialTrustVisibleAssemblyCollection.cs
- PaperSource.cs
- SharedStream.cs
- SectionXmlInfo.cs
- DrawingBrush.cs
- tibetanshape.cs
- StorageTypeMapping.cs
- OutArgument.cs
- EventLogPermissionHolder.cs
- DoubleLinkList.cs
- Completion.cs
- DeclaredTypeElement.cs
- DefaultValidator.cs
- InvalidPrinterException.cs
- NegatedConstant.cs
- OutputCacheProfileCollection.cs
- FileChangesMonitor.cs
- ECDsaCng.cs
- ResourceDescriptionAttribute.cs
- EventRouteFactory.cs
- BamlLocalizationDictionary.cs
- Pkcs7Signer.cs