Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusPointPropertyInfo.cs / 1 / StylusPointPropertyInfo.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Windows.Media; using System.Collections.Generic; using System.Diagnostics; using System.ComponentModel; using MS.Utility; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// StylusPointPropertyInfo /// public class StylusPointPropertyInfo : StylusPointProperty { ////// Instance data /// private int _min; private int _max; private float _resolution; private StylusPointPropertyUnit _unit; ////// For a given StylusPointProperty, instantiates a StylusPointPropertyInfo with default values /// /// public StylusPointPropertyInfo(StylusPointProperty stylusPointProperty) : base (stylusPointProperty) //base checks for null { StylusPointPropertyInfo info = StylusPointPropertyInfoDefaults.GetStylusPointPropertyInfoDefault(stylusPointProperty); _min = info.Minimum; _max = info.Maximum; _resolution = info.Resolution; _unit = info.Unit; } ////// StylusPointProperty /// /// /// minimum /// maximum /// unit /// resolution public StylusPointPropertyInfo(StylusPointProperty stylusPointProperty, int minimum, int maximum, StylusPointPropertyUnit unit, float resolution) : base(stylusPointProperty) //base checks for null { // validate unit if (!StylusPointPropertyUnitHelper.IsDefined(unit)) { throw new InvalidEnumArgumentException("StylusPointPropertyUnit", (int)unit, typeof(StylusPointPropertyUnit)); } // validate min/max if (maximum < minimum) { throw new ArgumentException(SR.Get(SRID.Stylus_InvalidMax), "maximum"); } // validate resolution if (resolution < 0.0f) { throw new ArgumentException(SR.Get(SRID.InvalidStylusPointPropertyInfoResolution), "resolution"); } _min = minimum; _max = maximum; _resolution = resolution; _unit = unit; } ////// Minimum /// public int Minimum { get { return _min; } } ////// Maximum /// public int Maximum { get { return _max; } } ////// Resolution /// public float Resolution { get { return _resolution; } } ////// Unit /// public StylusPointPropertyUnit Unit { get { return _unit; } } ////// Internal helper method for comparing compat for two StylusPointPropertyInfos /// internal static bool AreCompatible(StylusPointPropertyInfo stylusPointPropertyInfo1, StylusPointPropertyInfo stylusPointPropertyInfo2) { if (stylusPointPropertyInfo1 == null || stylusPointPropertyInfo2 == null) { throw new ArgumentNullException("stylusPointPropertyInfo"); } Debug.Assert(( stylusPointPropertyInfo1.Id != StylusPointPropertyIds.X && stylusPointPropertyInfo1.Id != StylusPointPropertyIds.Y && stylusPointPropertyInfo2.Id != StylusPointPropertyIds.X && stylusPointPropertyInfo2.Id != StylusPointPropertyIds.Y), "Why are you checking X, Y for compatibility? They're always compatible"); // // we only take ID and IsButton into account, we don't take metrics into account // return (stylusPointPropertyInfo1.Id == stylusPointPropertyInfo2.Id && stylusPointPropertyInfo1.IsButton == stylusPointPropertyInfo2.IsButton); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Windows.Media; using System.Collections.Generic; using System.Diagnostics; using System.ComponentModel; using MS.Utility; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// StylusPointPropertyInfo /// public class StylusPointPropertyInfo : StylusPointProperty { ////// Instance data /// private int _min; private int _max; private float _resolution; private StylusPointPropertyUnit _unit; ////// For a given StylusPointProperty, instantiates a StylusPointPropertyInfo with default values /// /// public StylusPointPropertyInfo(StylusPointProperty stylusPointProperty) : base (stylusPointProperty) //base checks for null { StylusPointPropertyInfo info = StylusPointPropertyInfoDefaults.GetStylusPointPropertyInfoDefault(stylusPointProperty); _min = info.Minimum; _max = info.Maximum; _resolution = info.Resolution; _unit = info.Unit; } ////// StylusPointProperty /// /// /// minimum /// maximum /// unit /// resolution public StylusPointPropertyInfo(StylusPointProperty stylusPointProperty, int minimum, int maximum, StylusPointPropertyUnit unit, float resolution) : base(stylusPointProperty) //base checks for null { // validate unit if (!StylusPointPropertyUnitHelper.IsDefined(unit)) { throw new InvalidEnumArgumentException("StylusPointPropertyUnit", (int)unit, typeof(StylusPointPropertyUnit)); } // validate min/max if (maximum < minimum) { throw new ArgumentException(SR.Get(SRID.Stylus_InvalidMax), "maximum"); } // validate resolution if (resolution < 0.0f) { throw new ArgumentException(SR.Get(SRID.InvalidStylusPointPropertyInfoResolution), "resolution"); } _min = minimum; _max = maximum; _resolution = resolution; _unit = unit; } ////// Minimum /// public int Minimum { get { return _min; } } ////// Maximum /// public int Maximum { get { return _max; } } ////// Resolution /// public float Resolution { get { return _resolution; } } ////// Unit /// public StylusPointPropertyUnit Unit { get { return _unit; } } ////// Internal helper method for comparing compat for two StylusPointPropertyInfos /// internal static bool AreCompatible(StylusPointPropertyInfo stylusPointPropertyInfo1, StylusPointPropertyInfo stylusPointPropertyInfo2) { if (stylusPointPropertyInfo1 == null || stylusPointPropertyInfo2 == null) { throw new ArgumentNullException("stylusPointPropertyInfo"); } Debug.Assert(( stylusPointPropertyInfo1.Id != StylusPointPropertyIds.X && stylusPointPropertyInfo1.Id != StylusPointPropertyIds.Y && stylusPointPropertyInfo2.Id != StylusPointPropertyIds.X && stylusPointPropertyInfo2.Id != StylusPointPropertyIds.Y), "Why are you checking X, Y for compatibility? They're always compatible"); // // we only take ID and IsButton into account, we don't take metrics into account // return (stylusPointPropertyInfo1.Id == stylusPointPropertyInfo2.Id && stylusPointPropertyInfo1.IsButton == stylusPointPropertyInfo2.IsButton); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
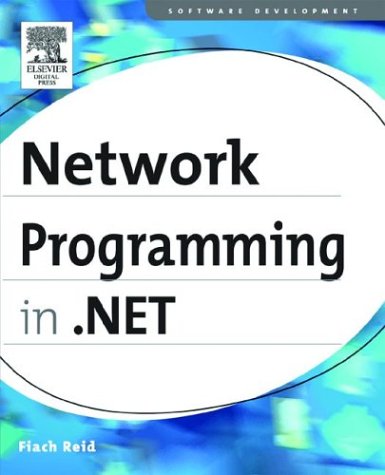
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AuthorizationRule.cs
- CannotUnloadAppDomainException.cs
- ExtractedStateEntry.cs
- Inflater.cs
- HyperlinkAutomationPeer.cs
- EditorPartCollection.cs
- _IPv4Address.cs
- ScopeElement.cs
- ManagementExtension.cs
- SingleAnimationBase.cs
- SelectionEditor.cs
- UserControl.cs
- DocumentViewerConstants.cs
- ConfigurationValues.cs
- ClientEndpointLoader.cs
- ADConnectionHelper.cs
- WorkflowMarkupSerializationException.cs
- HttpCookieCollection.cs
- ImportedNamespaceContextItem.cs
- DynamicPropertyHolder.cs
- Transform3DGroup.cs
- UnsafeNativeMethods.cs
- ScrollItemPatternIdentifiers.cs
- UniqueEventHelper.cs
- LoginName.cs
- Page.cs
- ProjectionCamera.cs
- Timer.cs
- UpdateExpressionVisitor.cs
- FaultDescription.cs
- WindowPattern.cs
- DataGridViewHeaderCell.cs
- WebResourceUtil.cs
- BoundColumn.cs
- ButtonFlatAdapter.cs
- DesignerOptions.cs
- PageEventArgs.cs
- RowUpdatedEventArgs.cs
- CustomAttributeSerializer.cs
- WebBrowser.cs
- TableCellAutomationPeer.cs
- ItemMap.cs
- VScrollBar.cs
- DateTimePickerDesigner.cs
- VersionedStreamOwner.cs
- DispatchOperation.cs
- OracleTransaction.cs
- OLEDB_Util.cs
- QueryStringParameter.cs
- IsolatedStorage.cs
- TaskFileService.cs
- TypeInfo.cs
- NativeMethods.cs
- ConstraintEnumerator.cs
- ChangeTracker.cs
- TypedElement.cs
- WebPartCollection.cs
- ImageAnimator.cs
- HttpPostProtocolImporter.cs
- Margins.cs
- SystemFonts.cs
- AttributeInfo.cs
- DBConnectionString.cs
- SiteMapNode.cs
- HostedElements.cs
- MarginsConverter.cs
- KnownBoxes.cs
- ObjectDataProvider.cs
- Msec.cs
- WaitForChangedResult.cs
- Stream.cs
- QueueProcessor.cs
- ByteStreamMessageEncoderFactory.cs
- TextParagraph.cs
- TransactionValidationBehavior.cs
- COM2Enum.cs
- TrackingParameters.cs
- AttributeEmitter.cs
- BatchParser.cs
- DataGridViewColumnCollection.cs
- InputGestureCollection.cs
- WindowsMenu.cs
- SocketPermission.cs
- ManualResetEvent.cs
- SchemaImporterExtensionsSection.cs
- BindingOperations.cs
- GradientSpreadMethodValidation.cs
- StrongNameKeyPair.cs
- DataSvcMapFile.cs
- UIElementAutomationPeer.cs
- RolePrincipal.cs
- HTMLTextWriter.cs
- BitmapFrame.cs
- ConfigXmlAttribute.cs
- DetailsViewInsertEventArgs.cs
- NavigatorInput.cs
- MimeTypePropertyAttribute.cs
- IPipelineRuntime.cs
- EntityCommand.cs
- CodeIterationStatement.cs