Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / DrawingImage.cs / 1 / DrawingImage.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: DrawingImage class // An ImageSource with a Drawing for content // // // History: // 05/26/2005 : jordanpa - Created it // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; namespace System.Windows.Media { ////// The class definition for DrawingImage /// public sealed partial class DrawingImage : ImageSource { ////// Default DrawingImage ctor /// public DrawingImage() { } ////// DrawingImage ctor that takes a Drawing /// /// The content of the DrawingImage public DrawingImage(Drawing drawing) { Drawing = drawing; } ////// Width of the DrawingImage /// public override double Width { get { ReadPreamble(); return Size.Width; } } ////// Height of the DrawingImage /// public override double Height { get { ReadPreamble(); return Size.Height; } } ////// Get the Metadata of the DrawingImage /// public override ImageMetadata Metadata { get { ReadPreamble(); // DrawingImage does not have any metadata currently defined. return null; } } ////// Size for the DrawingImage /// internal override Size Size { get { Drawing drawing = Drawing; if (drawing != null) { Size size = drawing.GetBounds().Size; if (!size.IsEmpty) { return size; } else { return new Size(); } } else { return new Size(); } } } ////// Precompute is called during the frame preparation phase. Derived classes /// typically check if the brush requires realizations during this phase. /// internal override void Precompute() { Drawing drawing = Drawing; _requiresRealizationUpdates = false; if (drawing != null) { drawing.PrecomputeCore(); _requiresRealizationUpdates = drawing.RequiresRealizationUpdates; } } ////// Checks if realization updates are requried for this resource. /// internal override bool RequiresRealizationUpdates { get { return _requiresRealizationUpdates; } } ////// Derived classes must override this method and update realizations on dependent /// resources if required. /// internal override void UpdateRealizations(RealizationContext ctx) { if (_requiresRealizationUpdates) { Drawing drawing = Drawing; Debug.Assert(drawing != null); // Otherwise _requiresRealizationUpdates would be false. drawing.UpdateRealizations(ctx); } } ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { return true; } private bool _requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: DrawingImage class // An ImageSource with a Drawing for content // // // History: // 05/26/2005 : jordanpa - Created it // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; namespace System.Windows.Media { ////// The class definition for DrawingImage /// public sealed partial class DrawingImage : ImageSource { ////// Default DrawingImage ctor /// public DrawingImage() { } ////// DrawingImage ctor that takes a Drawing /// /// The content of the DrawingImage public DrawingImage(Drawing drawing) { Drawing = drawing; } ////// Width of the DrawingImage /// public override double Width { get { ReadPreamble(); return Size.Width; } } ////// Height of the DrawingImage /// public override double Height { get { ReadPreamble(); return Size.Height; } } ////// Get the Metadata of the DrawingImage /// public override ImageMetadata Metadata { get { ReadPreamble(); // DrawingImage does not have any metadata currently defined. return null; } } ////// Size for the DrawingImage /// internal override Size Size { get { Drawing drawing = Drawing; if (drawing != null) { Size size = drawing.GetBounds().Size; if (!size.IsEmpty) { return size; } else { return new Size(); } } else { return new Size(); } } } ////// Precompute is called during the frame preparation phase. Derived classes /// typically check if the brush requires realizations during this phase. /// internal override void Precompute() { Drawing drawing = Drawing; _requiresRealizationUpdates = false; if (drawing != null) { drawing.PrecomputeCore(); _requiresRealizationUpdates = drawing.RequiresRealizationUpdates; } } ////// Checks if realization updates are requried for this resource. /// internal override bool RequiresRealizationUpdates { get { return _requiresRealizationUpdates; } } ////// Derived classes must override this method and update realizations on dependent /// resources if required. /// internal override void UpdateRealizations(RealizationContext ctx) { if (_requiresRealizationUpdates) { Drawing drawing = Drawing; Debug.Assert(drawing != null); // Otherwise _requiresRealizationUpdates would be false. drawing.UpdateRealizations(ctx); } } ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { return true; } private bool _requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
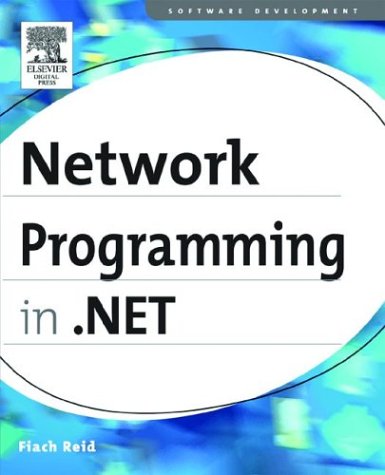
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartVerb.cs
- AssemblyInfo.cs
- EntityParameterCollection.cs
- RotateTransform3D.cs
- ImageMap.cs
- AssemblyBuilderData.cs
- TableChangeProcessor.cs
- SettingsAttributes.cs
- Quaternion.cs
- TextAutomationPeer.cs
- ConnectionPoolManager.cs
- IPipelineRuntime.cs
- FixedTextBuilder.cs
- SqlFacetAttribute.cs
- NavigationProperty.cs
- EntityCollection.cs
- StylusPointProperties.cs
- ThicknessAnimation.cs
- EntitySetBase.cs
- Camera.cs
- DesignerTransaction.cs
- ConnectionsZoneDesigner.cs
- ObjectDataSourceMethodEventArgs.cs
- SmtpReplyReader.cs
- SoapFaultCodes.cs
- PeerDefaultCustomResolverClient.cs
- RectangleGeometry.cs
- XmlNamedNodeMap.cs
- XmlDomTextWriter.cs
- XmlHelper.cs
- ProviderUtil.cs
- AutomationElement.cs
- SmiContext.cs
- ProviderConnectionPoint.cs
- parserscommon.cs
- RoutedEventValueSerializer.cs
- DependencyObjectType.cs
- ParserStreamGeometryContext.cs
- SortedDictionary.cs
- SHA256Cng.cs
- WindowsScrollBarBits.cs
- LinqToSqlWrapper.cs
- AnnotationAuthorChangedEventArgs.cs
- GridViewPageEventArgs.cs
- ObjectTokenCategory.cs
- EnumerableCollectionView.cs
- ImageMetadata.cs
- Bits.cs
- IMembershipProvider.cs
- ServiceManager.cs
- BasicExpandProvider.cs
- JsonMessageEncoderFactory.cs
- HttpCookieCollection.cs
- BinaryNode.cs
- VirtualizingStackPanel.cs
- FixedSOMGroup.cs
- Header.cs
- NullableDoubleMinMaxAggregationOperator.cs
- ProjectionCamera.cs
- CompareInfo.cs
- XmlSchemaObjectTable.cs
- BufferedGenericXmlSecurityToken.cs
- SystemUnicastIPAddressInformation.cs
- HashHelper.cs
- _NetRes.cs
- Atom10FormatterFactory.cs
- GlyphRunDrawing.cs
- ItemAutomationPeer.cs
- DropShadowEffect.cs
- TypeConverterHelper.cs
- SafeCoTaskMem.cs
- SymmetricKey.cs
- ListSourceHelper.cs
- DataGridRowEventArgs.cs
- CodeExporter.cs
- ApplyTemplatesAction.cs
- TabControlCancelEvent.cs
- DataBindingHandlerAttribute.cs
- TextServicesDisplayAttributePropertyRanges.cs
- NotImplementedException.cs
- FlowNode.cs
- CommandPlan.cs
- RoleManagerSection.cs
- CheckBox.cs
- MetafileHeaderWmf.cs
- WebPartZoneCollection.cs
- DataPagerFieldCommandEventArgs.cs
- PersonalizationDictionary.cs
- TypedRowGenerator.cs
- ToolStripPanelRenderEventArgs.cs
- ArithmeticException.cs
- TagMapCollection.cs
- GenericUI.cs
- AutomationEvent.cs
- OleDbFactory.cs
- DoubleKeyFrameCollection.cs
- ColorConverter.cs
- Exception.cs
- ResXBuildProvider.cs
- CapabilitiesAssignment.cs