Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / FacetDescriptionElement.cs / 1305376 / FacetDescriptionElement.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Data.Metadata.Edm; using System.Xml; using System.Diagnostics; namespace System.Data.EntityModel.SchemaObjectModel { internal abstract class FacetDescriptionElement : SchemaElement { int? _minValue; int? _maxValue; object _defaultValue; bool _isConstant; // won't be populated till you call CreateAndValidate FacetDescription _facetDescription; public FacetDescriptionElement(TypeElement type, string name) : base(type, name) { } protected override bool ProhibitAttribute(string namespaceUri, string localName) { if (base.ProhibitAttribute(namespaceUri, localName)) { return true; } if (namespaceUri == null && localName == XmlConstants.Name) { return false; } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.MinimumAttribute)) { HandleMinimumAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.MaximumAttribute)) { HandleMaximumAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.DefaultValueAttribute)) { HandleDefaultAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.ConstantAttribute)) { HandleConstantAttribute(reader); return true; } return false; } ///////////////////////////////////////////////////////////////////// // Attribute Handlers ////// Handler for the Minimum attribute /// /// xml reader currently positioned at Minimum attribute protected void HandleMinimumAttribute(XmlReader reader) { int value = -1; if (HandleIntAttribute(reader, ref value)) { _minValue = value; } } ////// Handler for the Maximum attribute /// /// xml reader currently positioned at Maximum attribute protected void HandleMaximumAttribute(XmlReader reader) { int value = -1; if (HandleIntAttribute(reader, ref value)) { _maxValue = value; } } ////// Handler for the Default attribute /// /// xml reader currently positioned at Default attribute protected abstract void HandleDefaultAttribute(XmlReader reader); ////// Handler for the Constant attribute /// /// xml reader currently positioned at Constant attribute protected void HandleConstantAttribute(XmlReader reader) { bool value = false; if (HandleBoolAttribute(reader, ref value)) { _isConstant = value; } } public abstract EdmType FacetType{ get; } public int? MinValue { get { return _minValue; } } public int? MaxValue { get { return _maxValue; } } public object DefaultValue { get { return _defaultValue; } set { _defaultValue = value; } } public FacetDescription FacetDescription { get { Debug.Assert(_facetDescription != null, "Did you forget to call CreateAndValidate first?"); return _facetDescription; } } internal void CreateAndValidateFacetDescription(string declaringTypeName) { _facetDescription = new FacetDescription(Name, FacetType, MinValue, MaxValue, DefaultValue, _isConstant, declaringTypeName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Data.Metadata.Edm; using System.Xml; using System.Diagnostics; namespace System.Data.EntityModel.SchemaObjectModel { internal abstract class FacetDescriptionElement : SchemaElement { int? _minValue; int? _maxValue; object _defaultValue; bool _isConstant; // won't be populated till you call CreateAndValidate FacetDescription _facetDescription; public FacetDescriptionElement(TypeElement type, string name) : base(type, name) { } protected override bool ProhibitAttribute(string namespaceUri, string localName) { if (base.ProhibitAttribute(namespaceUri, localName)) { return true; } if (namespaceUri == null && localName == XmlConstants.Name) { return false; } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.MinimumAttribute)) { HandleMinimumAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.MaximumAttribute)) { HandleMaximumAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.DefaultValueAttribute)) { HandleDefaultAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.ConstantAttribute)) { HandleConstantAttribute(reader); return true; } return false; } ///////////////////////////////////////////////////////////////////// // Attribute Handlers ////// Handler for the Minimum attribute /// /// xml reader currently positioned at Minimum attribute protected void HandleMinimumAttribute(XmlReader reader) { int value = -1; if (HandleIntAttribute(reader, ref value)) { _minValue = value; } } ////// Handler for the Maximum attribute /// /// xml reader currently positioned at Maximum attribute protected void HandleMaximumAttribute(XmlReader reader) { int value = -1; if (HandleIntAttribute(reader, ref value)) { _maxValue = value; } } ////// Handler for the Default attribute /// /// xml reader currently positioned at Default attribute protected abstract void HandleDefaultAttribute(XmlReader reader); ////// Handler for the Constant attribute /// /// xml reader currently positioned at Constant attribute protected void HandleConstantAttribute(XmlReader reader) { bool value = false; if (HandleBoolAttribute(reader, ref value)) { _isConstant = value; } } public abstract EdmType FacetType{ get; } public int? MinValue { get { return _minValue; } } public int? MaxValue { get { return _maxValue; } } public object DefaultValue { get { return _defaultValue; } set { _defaultValue = value; } } public FacetDescription FacetDescription { get { Debug.Assert(_facetDescription != null, "Did you forget to call CreateAndValidate first?"); return _facetDescription; } } internal void CreateAndValidateFacetDescription(string declaringTypeName) { _facetDescription = new FacetDescription(Name, FacetType, MinValue, MaxValue, DefaultValue, _isConstant, declaringTypeName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
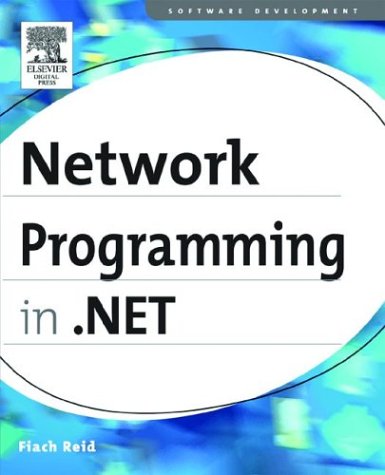
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberNameValidator.cs
- ThreadExceptionEvent.cs
- UnaryNode.cs
- EventMappingSettingsCollection.cs
- TabletDevice.cs
- GeneralTransform3D.cs
- MeasurementDCInfo.cs
- SqlProcedureAttribute.cs
- AdCreatedEventArgs.cs
- HasRunnableWorkflowEvent.cs
- ExpressionReplacer.cs
- TableLayoutColumnStyleCollection.cs
- OleDbError.cs
- SqlDataSourceView.cs
- GenericTextProperties.cs
- DummyDataSource.cs
- XPathAncestorIterator.cs
- OutputCacheSection.cs
- XmlObjectSerializerContext.cs
- CollectionConverter.cs
- GenericAuthenticationEventArgs.cs
- RealizationDrawingContextWalker.cs
- PropertyGrid.cs
- PngBitmapDecoder.cs
- EncodingInfo.cs
- WindowExtensionMethods.cs
- BrowserCapabilitiesCodeGenerator.cs
- SemanticResolver.cs
- DataServiceRequest.cs
- COM2ComponentEditor.cs
- MouseButton.cs
- ComboBox.cs
- Rule.cs
- AnnotationDocumentPaginator.cs
- LOSFormatter.cs
- LambdaCompiler.Generated.cs
- PriorityQueue.cs
- DeclarativeExpressionConditionDeclaration.cs
- FormViewCommandEventArgs.cs
- DataGridViewToolTip.cs
- DocumentViewerAutomationPeer.cs
- JsonEncodingStreamWrapper.cs
- XmlCharCheckingWriter.cs
- GuidConverter.cs
- DirectoryNotFoundException.cs
- BufferedStream.cs
- SerializationInfo.cs
- TargetControlTypeCache.cs
- HashAlgorithm.cs
- MappedMetaModel.cs
- RemoveStoryboard.cs
- CounterCreationData.cs
- MultiView.cs
- SafeNativeMethodsMilCoreApi.cs
- TypeLoadException.cs
- StrongName.cs
- AccessKeyManager.cs
- WmlFormAdapter.cs
- StrongNameHelpers.cs
- XsdDuration.cs
- SynchronizationContext.cs
- SynchronizationScope.cs
- TextSelection.cs
- PageDeviceFont.cs
- TemplateLookupAction.cs
- IntegerValidatorAttribute.cs
- ReachDocumentPageSerializer.cs
- ItemList.cs
- StorageEntityTypeMapping.cs
- LinearGradientBrush.cs
- invalidudtexception.cs
- TdsParserStateObject.cs
- smtppermission.cs
- DesignRelationCollection.cs
- RuntimeArgument.cs
- TraceSwitch.cs
- ConfigurationStrings.cs
- LookupNode.cs
- SaveFileDialogDesigner.cs
- WindowInteropHelper.cs
- DataGridLength.cs
- CompilationRelaxations.cs
- OneOfConst.cs
- SendMailErrorEventArgs.cs
- SpeechUI.cs
- AdornerLayer.cs
- PackagePartCollection.cs
- SerializationObjectManager.cs
- PerformanceCounterCategory.cs
- SemanticKeyElement.cs
- Camera.cs
- HebrewNumber.cs
- DesignerForm.cs
- HebrewNumber.cs
- MimeMapping.cs
- XPathPatternBuilder.cs
- LineBreakRecord.cs
- RelatedImageListAttribute.cs
- ResolveCompletedEventArgs.cs
- ManipulationPivot.cs