Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / Filter / FilterException.cs / 1 / FilterException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization; ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class InvalidExpressionException : DataException { protected InvalidExpressionException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///[To be supplied.] ////// public InvalidExpressionException() : base() {} ///[To be supplied.] ////// public InvalidExpressionException(string s) : base(s) {} public InvalidExpressionException(string message, Exception innerException) : base(message, innerException) {} } ///[To be supplied.] ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class EvaluateException : InvalidExpressionException { protected EvaluateException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///[To be supplied.] ////// public EvaluateException() : base() {} ///[To be supplied.] ////// public EvaluateException(string s) : base(s) {} public EvaluateException(string message, Exception innerException) : base(message, innerException) {} } ///[To be supplied.] ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class SyntaxErrorException : InvalidExpressionException { protected SyntaxErrorException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///[To be supplied.] ////// public SyntaxErrorException() : base() {} ///[To be supplied.] ////// public SyntaxErrorException(string s) : base(s) {} public SyntaxErrorException(string message, Exception innerException) : base(message, innerException) {} } internal sealed class ExprException { private ExprException() { /* prevent utility class from being insantiated*/ } static private OverflowException _Overflow(string error) { OverflowException e = new OverflowException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private InvalidExpressionException _Expr(string error) { InvalidExpressionException e = new InvalidExpressionException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private SyntaxErrorException _Syntax(string error) { SyntaxErrorException e = new SyntaxErrorException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private EvaluateException _Eval(string error) { EvaluateException e = new EvaluateException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private EvaluateException _Eval(string error, Exception innerException) { EvaluateException e = new EvaluateException(error/*, innerException*/); // ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static public Exception InvokeArgument() { return ExceptionBuilder._Argument(Res.GetString(Res.Expr_InvokeArgument)); } static public Exception NYI(string moreinfo) { string err = Res.GetString(Res.Expr_NYI, moreinfo); Debug.Fail(err); return _Expr(err); } static public Exception MissingOperand(OperatorInfo before) { return _Syntax(Res.GetString(Res.Expr_MissingOperand, Operators.ToString(before.op))); } static public Exception MissingOperator(string token) { return _Syntax(Res.GetString(Res.Expr_MissingOperand, token)); } static public Exception TypeMismatch(string expr) { return _Eval(Res.GetString(Res.Expr_TypeMismatch, expr)); } static public Exception FunctionArgumentOutOfRange(string arg, string func) { return ExceptionBuilder._ArgumentOutOfRange(arg, Res.GetString(Res.Expr_ArgumentOutofRange, func)); } static public Exception ExpressionTooComplex() { return _Eval(Res.GetString(Res.Expr_ExpressionTooComplex)); } static public Exception UnboundName(string name) { return _Eval(Res.GetString(Res.Expr_UnboundName, name)); } static public Exception InvalidString(string str) { return _Syntax(Res.GetString(Res.Expr_InvalidString, str)); } static public Exception UndefinedFunction(string name) { return _Eval(Res.GetString(Res.Expr_UndefinedFunction, name)); } static public Exception SyntaxError() { return _Syntax(Res.GetString(Res.Expr_Syntax)); } static public Exception FunctionArgumentCount(string name) { return _Eval(Res.GetString(Res.Expr_FunctionArgumentCount, name)); } static public Exception MissingRightParen() { return _Syntax(Res.GetString(Res.Expr_MissingRightParen)); } static public Exception UnknownToken(string token, int position) { return _Syntax(Res.GetString(Res.Expr_UnknownToken, token, position.ToString(CultureInfo.InvariantCulture))); } static public Exception UnknownToken(Tokens tokExpected, Tokens tokCurr, int position) { return _Syntax(Res.GetString(Res.Expr_UnknownToken1, tokExpected.ToString(), tokCurr.ToString(), position.ToString(CultureInfo.InvariantCulture))); } static public Exception DatatypeConvertion(Type type1, Type type2) { return _Eval(Res.GetString(Res.Expr_DatatypeConvertion, type1.ToString(), type2.ToString())); } static public Exception DatavalueConvertion(object value, Type type, Exception innerException) { return _Eval(Res.GetString(Res.Expr_DatavalueConvertion, value.ToString(), type.ToString()), innerException); } static public Exception InvalidName(string name) { return _Syntax(Res.GetString(Res.Expr_InvalidName, name)); } static public Exception InvalidDate(string date) { return _Syntax(Res.GetString(Res.Expr_InvalidDate, date)); } static public Exception NonConstantArgument() { return _Eval(Res.GetString(Res.Expr_NonConstantArgument)); } static public Exception InvalidPattern(string pat) { return _Eval(Res.GetString(Res.Expr_InvalidPattern, pat)); } static public Exception InWithoutParentheses() { return _Syntax(Res.GetString(Res.Expr_InWithoutParentheses)); } static public Exception InWithoutList() { return _Syntax(Res.GetString(Res.Expr_InWithoutList)); } static public Exception InvalidIsSyntax() { return _Syntax(Res.GetString(Res.Expr_IsSyntax)); } static public Exception Overflow(Type type) { return _Overflow(Res.GetString(Res.Expr_Overflow, type.Name)); } static public Exception ArgumentType(string function, int arg, Type type) { return _Eval(Res.GetString(Res.Expr_ArgumentType, function, arg.ToString(CultureInfo.InvariantCulture), type.ToString())); } static public Exception ArgumentTypeInteger(string function, int arg) { return _Eval(Res.GetString(Res.Expr_ArgumentTypeInteger, function, arg.ToString(CultureInfo.InvariantCulture))); } static public Exception TypeMismatchInBinop(int op, Type type1, Type type2) { return _Eval(Res.GetString(Res.Expr_TypeMismatchInBinop, Operators.ToString(op), type1.ToString(), type2.ToString())); } static public Exception AmbiguousBinop(int op, Type type1, Type type2) { return _Eval(Res.GetString(Res.Expr_AmbiguousBinop, Operators.ToString(op), type1.ToString(), type2.ToString())); } static public Exception UnsupportedOperator(int op) { return _Eval(Res.GetString(Res.Expr_UnsupportedOperator, Operators.ToString(op))); } static public Exception InvalidNameBracketing(string name) { return _Syntax(Res.GetString(Res.Expr_InvalidNameBracketing, name)); } static public Exception MissingOperandBefore(string op) { return _Syntax(Res.GetString(Res.Expr_MissingOperandBefore, op)); } static public Exception TooManyRightParentheses() { return _Syntax(Res.GetString(Res.Expr_TooManyRightParentheses)); } static public Exception UnresolvedRelation(string name, string expr) { return _Eval(Res.GetString(Res.Expr_UnresolvedRelation, name, expr)); } static internal EvaluateException BindFailure(string relationName) { return _Eval(Res.GetString(Res.Expr_BindFailure, relationName)); } static public Exception AggregateArgument() { return _Syntax(Res.GetString(Res.Expr_AggregateArgument)); } static public Exception AggregateUnbound(string expr) { return _Eval(Res.GetString(Res.Expr_AggregateUnbound, expr)); } static public Exception EvalNoContext() { return _Eval(Res.GetString(Res.Expr_EvalNoContext)); } static public Exception ExpressionUnbound(string expr) { return _Eval(Res.GetString(Res.Expr_ExpressionUnbound, expr)); } static public Exception ComputeNotAggregate(string expr) { return _Eval(Res.GetString(Res.Expr_ComputeNotAggregate, expr)); } static public Exception FilterConvertion(string expr) { return _Eval(Res.GetString(Res.Expr_FilterConvertion, expr)); } static public Exception LookupArgument() { return _Syntax(Res.GetString(Res.Expr_LookupArgument)); } static public Exception InvalidType(string typeName) { return _Eval(Res.GetString(Res.Expr_InvalidType, typeName)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
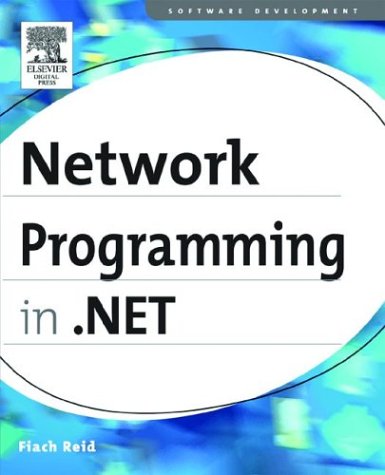
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeParameter.cs
- NavigateEvent.cs
- Int64AnimationBase.cs
- Mappings.cs
- WindowsGraphicsWrapper.cs
- CompositeTypefaceMetrics.cs
- DataGridToolTip.cs
- DataPager.cs
- DetailsViewRow.cs
- Oid.cs
- TextTabProperties.cs
- WindowsListViewSubItem.cs
- EventWaitHandleSecurity.cs
- FolderBrowserDialog.cs
- XmlSignatureManifest.cs
- DataGridRelationshipRow.cs
- ScriptResourceHandler.cs
- CodePageUtils.cs
- DropSource.cs
- ObjectQueryExecutionPlan.cs
- WorkflowInstanceTerminatedRecord.cs
- X509Utils.cs
- CalendarDayButton.cs
- AttachInfo.cs
- Polyline.cs
- sapiproxy.cs
- ReferencedType.cs
- PersonalizationProviderHelper.cs
- ColorTransformHelper.cs
- Faults.cs
- SyndicationLink.cs
- XmlWriterSettings.cs
- SecurityManager.cs
- documentsequencetextcontainer.cs
- PassportAuthentication.cs
- ConsoleKeyInfo.cs
- UInt16Converter.cs
- ParameterElement.cs
- XmlTextReaderImplHelpers.cs
- ZipIOLocalFileHeader.cs
- TitleStyle.cs
- WpfXamlMember.cs
- WebHttpSecurityElement.cs
- BitmapEffectInputConnector.cs
- LocalIdKeyIdentifierClause.cs
- StatusBarPanel.cs
- ZoneButton.cs
- NotifyInputEventArgs.cs
- PointValueSerializer.cs
- TextDecorationCollection.cs
- XPathExpr.cs
- RowsCopiedEventArgs.cs
- fixedPageContentExtractor.cs
- SigningCredentials.cs
- ImageListImage.cs
- TreeViewEvent.cs
- DataGridViewRowHeaderCell.cs
- SetIterators.cs
- CryptoKeySecurity.cs
- DateTimeUtil.cs
- PageCatalogPart.cs
- XamlWrappingReader.cs
- RoleManagerSection.cs
- DataGridViewDesigner.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- GorillaCodec.cs
- Int32.cs
- Vertex.cs
- CopyAction.cs
- GradientStop.cs
- LabelAutomationPeer.cs
- AssemblyHelper.cs
- Point3DAnimation.cs
- ProtectedConfiguration.cs
- StylusDownEventArgs.cs
- DataBindingCollection.cs
- XamlVector3DCollectionSerializer.cs
- RangeValueProviderWrapper.cs
- MimeReturn.cs
- RC2.cs
- AliasExpr.cs
- ApplicationInfo.cs
- DbParameterCollection.cs
- SystemMulticastIPAddressInformation.cs
- DebugControllerThread.cs
- ObjectListDataBindEventArgs.cs
- CodeEventReferenceExpression.cs
- WeakRefEnumerator.cs
- CustomTypeDescriptor.cs
- UpdatePanelControlTrigger.cs
- ReferenceConverter.cs
- BooleanFunctions.cs
- StaticDataManager.cs
- CustomError.cs
- TableCellCollection.cs
- SerializationEventsCache.cs
- RelationshipDetailsRow.cs
- ViewEvent.cs
- Publisher.cs
- FieldNameLookup.cs