Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / IO / Packaging / fixedPageContentExtractor.cs / 1305600 / fixedPageContentExtractor.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Given a DOM node for a fixed page, enumerates its text content. // // History: // 05/11/2004: JohnLarc: Initial implementation //--------------------------------------------------------------------------- using System; using System.Xml; namespace MS.Internal.IO.Packaging { ////// Implements a sequence of (textContent, precedingDelimiter) pairs for /// a fixed page node. /// internal class FixedPageContentExtractor { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Initialize a FixedPageContentExtractor from a DOM node. /// internal FixedPageContentExtractor(XmlNode fixedPage) { _fixedPageInfo = new XmlFixedPageInfo(fixedPage); _nextGlyphRun = 0; } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Return the content of the next glyph run, with a boolean indication /// whether it is separated by a space form the preceding glyph run. /// internal string NextGlyphContent(out bool inline, out uint lcid) { // Right now, we use the simplest possible heuristic for // spacing glyph runs: All pairs of adjacent glyph runs are assumed // to be separated by a word break. inline = false; lcid = 0; // End of page? if (_nextGlyphRun >= _fixedPageInfo.GlyphRunCount) { return null; } // Retrieve inline, lcid and return value from the next glyph run info. GlyphRunInfo glyphRunInfo = _fixedPageInfo.GlyphRunAtPosition(_nextGlyphRun); lcid = glyphRunInfo.LanguageID; // Point to the next glyph run for the next call and return. ++_nextGlyphRun; return glyphRunInfo.UnicodeString; } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Indicates whether no more content can be returned. /// internal bool AtEndOfPage { get { return _nextGlyphRun >= _fixedPageInfo.GlyphRunCount; } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private XmlFixedPageInfo _fixedPageInfo; private int _nextGlyphRun; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Given a DOM node for a fixed page, enumerates its text content. // // History: // 05/11/2004: JohnLarc: Initial implementation //--------------------------------------------------------------------------- using System; using System.Xml; namespace MS.Internal.IO.Packaging { ////// Implements a sequence of (textContent, precedingDelimiter) pairs for /// a fixed page node. /// internal class FixedPageContentExtractor { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Initialize a FixedPageContentExtractor from a DOM node. /// internal FixedPageContentExtractor(XmlNode fixedPage) { _fixedPageInfo = new XmlFixedPageInfo(fixedPage); _nextGlyphRun = 0; } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Return the content of the next glyph run, with a boolean indication /// whether it is separated by a space form the preceding glyph run. /// internal string NextGlyphContent(out bool inline, out uint lcid) { // Right now, we use the simplest possible heuristic for // spacing glyph runs: All pairs of adjacent glyph runs are assumed // to be separated by a word break. inline = false; lcid = 0; // End of page? if (_nextGlyphRun >= _fixedPageInfo.GlyphRunCount) { return null; } // Retrieve inline, lcid and return value from the next glyph run info. GlyphRunInfo glyphRunInfo = _fixedPageInfo.GlyphRunAtPosition(_nextGlyphRun); lcid = glyphRunInfo.LanguageID; // Point to the next glyph run for the next call and return. ++_nextGlyphRun; return glyphRunInfo.UnicodeString; } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Indicates whether no more content can be returned. /// internal bool AtEndOfPage { get { return _nextGlyphRun >= _fixedPageInfo.GlyphRunCount; } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private XmlFixedPageInfo _fixedPageInfo; private int _nextGlyphRun; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
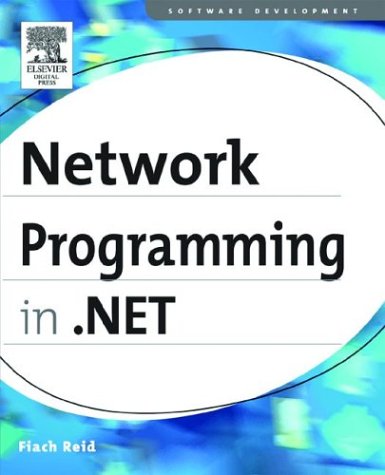
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReachUIElementCollectionSerializer.cs
- WebPartDisplayMode.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- bindurihelper.cs
- VariantWrapper.cs
- XamlBuildTaskServices.cs
- InfoCardClaim.cs
- StyleCollection.cs
- ItemChangedEventArgs.cs
- FormsAuthenticationEventArgs.cs
- CodeStatement.cs
- CodeDomConfigurationHandler.cs
- MasterPageBuildProvider.cs
- Int32CollectionConverter.cs
- StatusBar.cs
- mediaeventargs.cs
- regiisutil.cs
- XmlCompatibilityReader.cs
- Label.cs
- Rect.cs
- RadioButtonFlatAdapter.cs
- DataBinder.cs
- ControlAdapter.cs
- WebPartConnectionsEventArgs.cs
- ElementMarkupObject.cs
- TcpPortSharing.cs
- DragDeltaEventArgs.cs
- KeyGesture.cs
- CustomTypeDescriptor.cs
- PassportAuthenticationEventArgs.cs
- BreakRecordTable.cs
- ThreadNeutralSemaphore.cs
- FixedSOMSemanticBox.cs
- SqlUserDefinedAggregateAttribute.cs
- FormatVersion.cs
- messageonlyhwndwrapper.cs
- Base64Encoding.cs
- ErrorRuntimeConfig.cs
- ControlSerializer.cs
- TCPClient.cs
- DBConnection.cs
- TimeoutException.cs
- ImageDesigner.cs
- MgmtConfigurationRecord.cs
- ResourceExpressionBuilder.cs
- CompositeControl.cs
- AnchoredBlock.cs
- securitycriticaldataformultiplegetandset.cs
- SectionVisual.cs
- XmlEventCache.cs
- BinaryParser.cs
- UsernameTokenFactoryCredential.cs
- SecuritySessionSecurityTokenAuthenticator.cs
- HttpProfileGroupBase.cs
- SequenceRange.cs
- RayHitTestParameters.cs
- SchemaMapping.cs
- HttpHandlersSection.cs
- Camera.cs
- MatrixTransform.cs
- FrameworkObject.cs
- UnsafeNativeMethods.cs
- StandardMenuStripVerb.cs
- XPathScanner.cs
- FileSystemWatcher.cs
- ResourceDisplayNameAttribute.cs
- MsmqIntegrationReceiveParameters.cs
- _ChunkParse.cs
- ExclusiveNamedPipeTransportManager.cs
- ExpressionLink.cs
- MDIWindowDialog.cs
- DesignerSelectionListAdapter.cs
- UnsafeNativeMethods.cs
- ComAdminWrapper.cs
- Message.cs
- FlowLayout.cs
- PageBreakRecord.cs
- DependencyProperty.cs
- GenericWebPart.cs
- MULTI_QI.cs
- ValueTable.cs
- DrawingVisualDrawingContext.cs
- FormViewDesigner.cs
- QueryAccessibilityHelpEvent.cs
- SubMenuStyleCollection.cs
- BitmapMetadataBlob.cs
- WebPartsSection.cs
- DataServiceStreamProviderWrapper.cs
- AttributeProviderAttribute.cs
- Clipboard.cs
- DataGridClipboardCellContent.cs
- PlatformNotSupportedException.cs
- SrgsElementFactoryCompiler.cs
- Literal.cs
- PopupEventArgs.cs
- XmlSchemaChoice.cs
- DomNameTable.cs
- Invariant.cs
- ApplicationHost.cs
- SpecialFolderEnumConverter.cs