Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / ExclusiveNamedPipeTransportManager.cs / 1 / ExclusiveNamedPipeTransportManager.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel; using System.IO; using System.Text; using System.Threading; using System.ServiceModel.Diagnostics; using System.Diagnostics; using System.Security.Principal; sealed class ExclusiveNamedPipeTransportManager : NamedPipeTransportManager { ConnectionDemuxer connectionDemuxer; IConnectionListener connectionListener; public ExclusiveNamedPipeTransportManager(Uri listenUri, NamedPipeChannelListener channelListener) : base(listenUri) { ApplyListenerSettings(channelListener); SetHostNameComparisonMode(channelListener.HostNameComparisonMode); SetAllowedUsers(channelListener.AllowedUsers); } internal override void OnOpen() { connectionListener = new BufferedConnectionListener( new PipeConnectionListener(ListenUri, HostNameComparisonMode, ConnectionBufferSize, AllowedUsers, true, int.MaxValue), MaxOutputDelay, ConnectionBufferSize); if (DiagnosticUtility.ShouldUseActivity) { connectionListener = new TracingConnectionListener(connectionListener, this.ListenUri.ToString(), false); } connectionDemuxer = new ConnectionDemuxer(connectionListener, MaxPendingAccepts, MaxPendingConnections, ChannelInitializationTimeout, IdleTimeout, MaxPooledConnections, OnGetTransportFactorySettings, OnGetSingletonMessageHandler, OnHandleServerSessionPreamble, OnDemuxerError); bool startedDemuxing = false; try { connectionDemuxer.StartDemuxing(); startedDemuxing = true; } finally { if (!startedDemuxing) { connectionDemuxer.Dispose(); } } } internal override void OnClose() { connectionDemuxer.Dispose(); connectionListener.Dispose(); base.OnClose(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
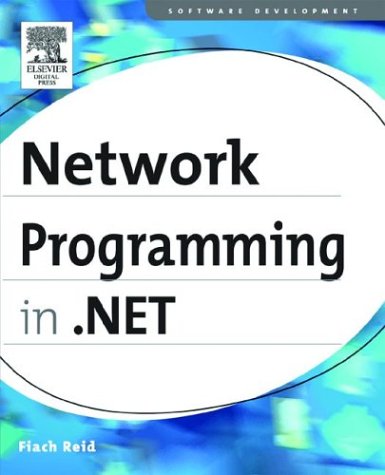
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebResponse.cs
- Operand.cs
- PreviewControlDesigner.cs
- DataGridViewCellCollection.cs
- ExtensionWindow.cs
- NotificationContext.cs
- InvalidOperationException.cs
- ScriptRef.cs
- TextTreeInsertUndoUnit.cs
- ValuePatternIdentifiers.cs
- ToolStripSplitButton.cs
- SettingsContext.cs
- OdbcConnectionFactory.cs
- MultiAsyncResult.cs
- BitVector32.cs
- MeasurementDCInfo.cs
- RenderingBiasValidation.cs
- HttpResponse.cs
- XPathAncestorQuery.cs
- ComponentResourceManager.cs
- PeerDefaultCustomResolverClient.cs
- CodeAttributeDeclaration.cs
- ServiceChannelManager.cs
- FontClient.cs
- Parallel.cs
- XpsFilter.cs
- XPathParser.cs
- Privilege.cs
- TreePrinter.cs
- ProcessInfo.cs
- InlineCollection.cs
- SafeProcessHandle.cs
- Command.cs
- SqlTypeConverter.cs
- KeyFrames.cs
- DataGridAddNewRow.cs
- SchemaTypeEmitter.cs
- Exceptions.cs
- IdnMapping.cs
- AspCompat.cs
- TypeDescriptionProviderAttribute.cs
- BlurBitmapEffect.cs
- FixUp.cs
- SchemaImporterExtension.cs
- BamlStream.cs
- SweepDirectionValidation.cs
- FaultReason.cs
- XPathDocumentIterator.cs
- RoleManagerModule.cs
- ObjectListTitleAttribute.cs
- NameValueFileSectionHandler.cs
- KerberosSecurityTokenAuthenticator.cs
- DocumentPaginator.cs
- GACMembershipCondition.cs
- TextViewBase.cs
- AddingNewEventArgs.cs
- LicenseException.cs
- EncoderExceptionFallback.cs
- HwndHost.cs
- HostExecutionContextManager.cs
- EditorPartCollection.cs
- FlowDocumentPageViewerAutomationPeer.cs
- WorkflowWebService.cs
- ZipFileInfo.cs
- relpropertyhelper.cs
- SecurityHelper.cs
- WebPageTraceListener.cs
- ZipIOLocalFileHeader.cs
- RemotingConfiguration.cs
- ItemCheckEvent.cs
- ColorConvertedBitmapExtension.cs
- ModelPropertyImpl.cs
- NavigationPropertyEmitter.cs
- UserControlCodeDomTreeGenerator.cs
- BindingsCollection.cs
- TokenBasedSetEnumerator.cs
- ConfigurationFileMap.cs
- BindMarkupExtensionSerializer.cs
- Base64Stream.cs
- Literal.cs
- AppSettings.cs
- StopStoryboard.cs
- XmlQueryContext.cs
- PackageRelationship.cs
- ResourceAttributes.cs
- TreeNodeStyle.cs
- AsymmetricKeyExchangeFormatter.cs
- NonParentingControl.cs
- RangeValidator.cs
- SignerInfo.cs
- ThreadStaticAttribute.cs
- TemplatePropertyEntry.cs
- CompilerTypeWithParams.cs
- OdbcCommandBuilder.cs
- PointCollectionConverter.cs
- FunctionDetailsReader.cs
- PrinterSettings.cs
- DefaultTextStoreTextComposition.cs
- EntityFunctions.cs
- SingleObjectCollection.cs