Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / ComponentModel / LicenseException.cs / 1 / LicenseException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Diagnostics; using System.Runtime.Serialization; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1032:ImplementStandardExceptionConstructors")] // must not, a Type is required in all constructors. [Serializable] public class LicenseException : SystemException { private Type type; private object instance; ///Represents the exception thrown when a component cannot be granted a license. ////// public LicenseException(Type type) : this(type, null, SR.GetString(SR.LicExceptionTypeOnly, type.FullName)) { } ///Initializes a new instance of the ///class for the /// specified type. /// public LicenseException(Type type, object instance) : this(type, null, SR.GetString(SR.LicExceptionTypeAndInstance, type.FullName, instance.GetType().FullName)) { } ///Initializes a new instance of the ///class for the /// specified type and instance. /// public LicenseException(Type type, object instance, string message) : base(message) { this.type = type; this.instance = instance; HResult = HResults.License; } ///Initializes a new instance of the ///class for the /// specified type and instance with the specified message. /// public LicenseException(Type type, object instance, string message, Exception innerException) : base(message, innerException) { this.type = type; this.instance = instance; HResult = HResults.License; } ///Initializes a new instance of the ///class for the /// specified innerException, type and instance with the specified message. /// Need this constructor since Exception implements ISerializable. /// protected LicenseException(SerializationInfo info, StreamingContext context) : base (info, context) { type = (Type) info.GetValue("type", typeof(Type)); instance = info.GetValue("instance", typeof(object)); } ////// public Type LicensedType { get { return type; } } ///Gets the type of the component that was not granted a license. ////// Need this since Exception implements ISerializable and we have fields to save out. /// [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) { throw new ArgumentNullException("info"); } info.AddValue("type", type); info.AddValue("instance", instance); base.GetObjectData(info, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Diagnostics; using System.Runtime.Serialization; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1032:ImplementStandardExceptionConstructors")] // must not, a Type is required in all constructors. [Serializable] public class LicenseException : SystemException { private Type type; private object instance; ///Represents the exception thrown when a component cannot be granted a license. ////// public LicenseException(Type type) : this(type, null, SR.GetString(SR.LicExceptionTypeOnly, type.FullName)) { } ///Initializes a new instance of the ///class for the /// specified type. /// public LicenseException(Type type, object instance) : this(type, null, SR.GetString(SR.LicExceptionTypeAndInstance, type.FullName, instance.GetType().FullName)) { } ///Initializes a new instance of the ///class for the /// specified type and instance. /// public LicenseException(Type type, object instance, string message) : base(message) { this.type = type; this.instance = instance; HResult = HResults.License; } ///Initializes a new instance of the ///class for the /// specified type and instance with the specified message. /// public LicenseException(Type type, object instance, string message, Exception innerException) : base(message, innerException) { this.type = type; this.instance = instance; HResult = HResults.License; } ///Initializes a new instance of the ///class for the /// specified innerException, type and instance with the specified message. /// Need this constructor since Exception implements ISerializable. /// protected LicenseException(SerializationInfo info, StreamingContext context) : base (info, context) { type = (Type) info.GetValue("type", typeof(Type)); instance = info.GetValue("instance", typeof(object)); } ////// public Type LicensedType { get { return type; } } ///Gets the type of the component that was not granted a license. ////// Need this since Exception implements ISerializable and we have fields to save out. /// [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) { throw new ArgumentNullException("info"); } info.AddValue("type", type); info.AddValue("instance", instance); base.GetObjectData(info, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
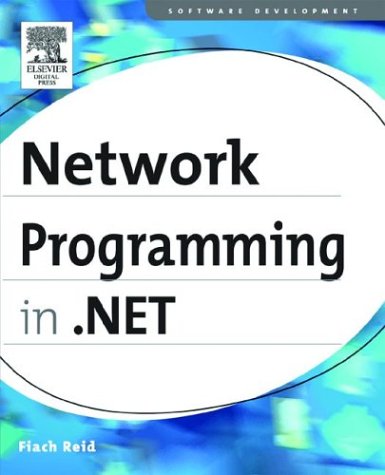
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceRequestException.cs
- PointConverter.cs
- ClientFormsAuthenticationCredentials.cs
- Label.cs
- NativeRightsManagementAPIsStructures.cs
- BlobPersonalizationState.cs
- ParallelTimeline.cs
- GatewayDefinition.cs
- FieldToken.cs
- DashStyles.cs
- ActivityMarkupSerializer.cs
- TextEditorContextMenu.cs
- SqlProfileProvider.cs
- OverflowException.cs
- ListViewItemSelectionChangedEvent.cs
- RegionInfo.cs
- ProtocolsConfigurationEntry.cs
- DoubleCollection.cs
- LinkTarget.cs
- DTCTransactionManager.cs
- IDReferencePropertyAttribute.cs
- NotCondition.cs
- SQLBinary.cs
- PackagePart.cs
- AlternateView.cs
- DashStyle.cs
- Socket.cs
- PartitionedStream.cs
- CollectionViewSource.cs
- GetPageNumberCompletedEventArgs.cs
- URLIdentityPermission.cs
- XmlDataSourceView.cs
- ScrollChrome.cs
- PageCache.cs
- PenContexts.cs
- Helper.cs
- TextTreeUndoUnit.cs
- UnsignedPublishLicense.cs
- UniformGrid.cs
- ScrollEvent.cs
- XamlGridLengthSerializer.cs
- CodeLabeledStatement.cs
- EntityTransaction.cs
- ApplicationInfo.cs
- VisualCollection.cs
- DateTimeFormat.cs
- CompiledXpathExpr.cs
- RealizationDrawingContextWalker.cs
- SafeEventHandle.cs
- ExecutedRoutedEventArgs.cs
- WorkflowInstance.cs
- XAMLParseException.cs
- StoreAnnotationsMap.cs
- ManualResetEvent.cs
- EntityDataSourceReferenceGroup.cs
- StylusPointProperty.cs
- DocumentViewerHelper.cs
- StringSorter.cs
- PrimaryKeyTypeConverter.cs
- AppDomainFactory.cs
- ToolboxComponentsCreatedEventArgs.cs
- Triplet.cs
- RadioButton.cs
- ConsumerConnectionPoint.cs
- CodeParameterDeclarationExpression.cs
- AppSettingsReader.cs
- UriScheme.cs
- SplitContainerDesigner.cs
- SelectedDatesCollection.cs
- DataGridViewCellValueEventArgs.cs
- AssemblyResourceLoader.cs
- RequestCacheValidator.cs
- StrokeNode.cs
- CommandHelpers.cs
- DataBoundLiteralControl.cs
- _NestedMultipleAsyncResult.cs
- SoapRpcMethodAttribute.cs
- AsymmetricAlgorithm.cs
- Processor.cs
- KoreanLunisolarCalendar.cs
- _SslStream.cs
- SqlGatherConsumedAliases.cs
- XsdValidatingReader.cs
- QueryableFilterUserControl.cs
- IDispatchConstantAttribute.cs
- TimeSpanStorage.cs
- ExpressionBinding.cs
- DefaultTextStore.cs
- IntegerValidator.cs
- SecUtil.cs
- Calendar.cs
- clipboard.cs
- CapabilitiesAssignment.cs
- HtmlSelect.cs
- XmlParserContext.cs
- NavigatingCancelEventArgs.cs
- WSSecurityJan2004.cs
- StringKeyFrameCollection.cs
- ViewKeyConstraint.cs
- SqlServer2KCompatibilityCheck.cs