Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Epm / EpmCustomContentDeSerializer.cs / 1305376 / EpmCustomContentDeSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Deserializer for the EPM content on the server // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { #region Namespaces using System.Collections.Generic; using System.Data.Services.Providers; using System.Diagnostics; using System.Linq; using System.ServiceModel.Syndication; using System.Xml; using System.Text; #endregion ///Custom content reader for EPM content internal sealed class EpmCustomContentDeSerializer : EpmContentDeSerializerBase { ///Constructor ///to read content from /// State of the deserializer internal EpmCustomContentDeSerializer(SyndicationItem item, EpmContentDeSerializer.EpmContentDeserializerState state) : base(item, state) { } /// Publicly accessible deserialization entry point /// Type of resource to deserialize /// Token corresponding to object ofinternal void DeSerialize(ResourceType resourceType, object element) { foreach (SyndicationElementExtension extension in this.Item.ElementExtensions) { using (XmlReader extensionReader = extension.GetReader()) { this.DeSerialize(extensionReader, resourceType.EpmTargetTree.NonSyndicationRoot, resourceType, element); } } } /// Called internally to deserialize each /// XmlReader for current extension /// Node in the target path being processed /// ResourceType /// object being deserialized private void DeSerialize(XmlReader reader, EpmTargetPathSegment currentRoot, ResourceType resourceType, object element) { EpmValueBuilder currentValue = new EpmValueBuilder(); do { switch (reader.NodeType) { case XmlNodeType.Element: if (currentRoot.HasContent) { // Throw an exception that we hit mixed-content. //value //throw DataServiceException.CreateBadRequestError(Strings.EpmDeserialize_MixedContent(resourceType.FullName)); } String elementName = reader.LocalName; String namespaceUri = reader.NamespaceURI; EpmTargetPathSegment newRoot = currentRoot.SubSegments .SingleOrDefault(s => s.SegmentNamespaceUri == namespaceUri && s.SegmentName == elementName); if (newRoot == null) { WebUtil.SkipToEnd(reader, elementName, namespaceUri); continue; } currentRoot = newRoot; this.DeserializeAttributes(reader, currentRoot, element, resourceType); if (currentRoot.HasContent) { if (reader.IsEmptyElement) { if (!EpmContentDeSerializerBase.Match(currentRoot, this.PropertiesApplied)) { resourceType.SetEpmValue(currentRoot, element, String.Empty, this); } currentRoot = currentRoot.ParentSegment; } } break; case XmlNodeType.CDATA: case XmlNodeType.Text: case XmlNodeType.SignificantWhitespace: if (!currentRoot.HasContent) { // Throw an exception that we hit mixed-content. // value value //value throw DataServiceException.CreateBadRequestError(Strings.EpmDeserialize_MixedContent(resourceType.FullName)); } currentValue.Append(reader.Value); break; case XmlNodeType.EndElement: if (currentRoot.HasContent) { if (!EpmContentDeSerializerBase.Match(currentRoot, this.PropertiesApplied)) { resourceType.SetEpmValue(currentRoot, element, currentValue.Value, this); } } currentRoot = currentRoot.ParentSegment; currentValue.Reset(); break; case XmlNodeType.Comment: case XmlNodeType.Whitespace: break; case XmlNodeType.None: case XmlNodeType.XmlDeclaration: case XmlNodeType.Attribute: case XmlNodeType.EndEntity: case XmlNodeType.EntityReference: case XmlNodeType.Entity: case XmlNodeType.Document: case XmlNodeType.DocumentType: case XmlNodeType.DocumentFragment: case XmlNodeType.Notation: case XmlNodeType.ProcessingInstruction: throw DataServiceException.CreateBadRequestError(Strings.EpmDeserialize_InvalidXmlEntity); } } while (currentRoot.ParentSegment != null && reader.Read()); } /// value value/// Deserializes the attributes from the /// Current content reader. /// Segment which has child attribute segments. /// Current object. /// Resource type ofand sets values on /// private void DeserializeAttributes(XmlReader reader, EpmTargetPathSegment currentRoot, object element, ResourceType resourceType) { foreach (var attributeSegment in currentRoot.SubSegments.Where(s => s.IsAttribute)) { String attribValue = WebUtil.GetAttributeEx(reader, attributeSegment.SegmentName.Substring(1), attributeSegment.SegmentNamespaceUri); if (attribValue != null) { if (!EpmContentDeSerializerBase.Match(attributeSegment, this.PropertiesApplied)) { resourceType.SetEpmValue(attributeSegment, element, attribValue, this); } } } } /// Collects current XmlReader values into a single string. private class EpmValueBuilder { ///Current value when single text content value is seen. private string elementValue; ///Current value if multiple text content values are seen together. private StringBuilder builder; ///Final value which is concatenation of all text content. internal string Value { get { if (this.builder != null) { return this.builder.ToString(); } return this.elementValue ?? String.Empty; } } ///Appends the current text content value to already held values. /// Current text content value. internal void Append(string value) { if (this.elementValue == null) { this.elementValue = value; } else if (this.builder == null) { string newValue = value; this.builder = new StringBuilder(elementValue.Length + newValue.Length) .Append(elementValue) .Append(newValue); } else { this.builder.Append(value); } } ///Once value is read, resets the current content to null. internal void Reset() { this.elementValue = null; this.builder = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Deserializer for the EPM content on the server // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { #region Namespaces using System.Collections.Generic; using System.Data.Services.Providers; using System.Diagnostics; using System.Linq; using System.ServiceModel.Syndication; using System.Xml; using System.Text; #endregion ///Custom content reader for EPM content internal sealed class EpmCustomContentDeSerializer : EpmContentDeSerializerBase { ///Constructor ///to read content from /// State of the deserializer internal EpmCustomContentDeSerializer(SyndicationItem item, EpmContentDeSerializer.EpmContentDeserializerState state) : base(item, state) { } /// Publicly accessible deserialization entry point /// Type of resource to deserialize /// Token corresponding to object ofinternal void DeSerialize(ResourceType resourceType, object element) { foreach (SyndicationElementExtension extension in this.Item.ElementExtensions) { using (XmlReader extensionReader = extension.GetReader()) { this.DeSerialize(extensionReader, resourceType.EpmTargetTree.NonSyndicationRoot, resourceType, element); } } } /// Called internally to deserialize each /// XmlReader for current extension /// Node in the target path being processed /// ResourceType /// object being deserialized private void DeSerialize(XmlReader reader, EpmTargetPathSegment currentRoot, ResourceType resourceType, object element) { EpmValueBuilder currentValue = new EpmValueBuilder(); do { switch (reader.NodeType) { case XmlNodeType.Element: if (currentRoot.HasContent) { // Throw an exception that we hit mixed-content. //value //throw DataServiceException.CreateBadRequestError(Strings.EpmDeserialize_MixedContent(resourceType.FullName)); } String elementName = reader.LocalName; String namespaceUri = reader.NamespaceURI; EpmTargetPathSegment newRoot = currentRoot.SubSegments .SingleOrDefault(s => s.SegmentNamespaceUri == namespaceUri && s.SegmentName == elementName); if (newRoot == null) { WebUtil.SkipToEnd(reader, elementName, namespaceUri); continue; } currentRoot = newRoot; this.DeserializeAttributes(reader, currentRoot, element, resourceType); if (currentRoot.HasContent) { if (reader.IsEmptyElement) { if (!EpmContentDeSerializerBase.Match(currentRoot, this.PropertiesApplied)) { resourceType.SetEpmValue(currentRoot, element, String.Empty, this); } currentRoot = currentRoot.ParentSegment; } } break; case XmlNodeType.CDATA: case XmlNodeType.Text: case XmlNodeType.SignificantWhitespace: if (!currentRoot.HasContent) { // Throw an exception that we hit mixed-content. // value value //value throw DataServiceException.CreateBadRequestError(Strings.EpmDeserialize_MixedContent(resourceType.FullName)); } currentValue.Append(reader.Value); break; case XmlNodeType.EndElement: if (currentRoot.HasContent) { if (!EpmContentDeSerializerBase.Match(currentRoot, this.PropertiesApplied)) { resourceType.SetEpmValue(currentRoot, element, currentValue.Value, this); } } currentRoot = currentRoot.ParentSegment; currentValue.Reset(); break; case XmlNodeType.Comment: case XmlNodeType.Whitespace: break; case XmlNodeType.None: case XmlNodeType.XmlDeclaration: case XmlNodeType.Attribute: case XmlNodeType.EndEntity: case XmlNodeType.EntityReference: case XmlNodeType.Entity: case XmlNodeType.Document: case XmlNodeType.DocumentType: case XmlNodeType.DocumentFragment: case XmlNodeType.Notation: case XmlNodeType.ProcessingInstruction: throw DataServiceException.CreateBadRequestError(Strings.EpmDeserialize_InvalidXmlEntity); } } while (currentRoot.ParentSegment != null && reader.Read()); } /// value value/// Deserializes the attributes from the /// Current content reader. /// Segment which has child attribute segments. /// Current object. /// Resource type ofand sets values on /// private void DeserializeAttributes(XmlReader reader, EpmTargetPathSegment currentRoot, object element, ResourceType resourceType) { foreach (var attributeSegment in currentRoot.SubSegments.Where(s => s.IsAttribute)) { String attribValue = WebUtil.GetAttributeEx(reader, attributeSegment.SegmentName.Substring(1), attributeSegment.SegmentNamespaceUri); if (attribValue != null) { if (!EpmContentDeSerializerBase.Match(attributeSegment, this.PropertiesApplied)) { resourceType.SetEpmValue(attributeSegment, element, attribValue, this); } } } } /// Collects current XmlReader values into a single string. private class EpmValueBuilder { ///Current value when single text content value is seen. private string elementValue; ///Current value if multiple text content values are seen together. private StringBuilder builder; ///Final value which is concatenation of all text content. internal string Value { get { if (this.builder != null) { return this.builder.ToString(); } return this.elementValue ?? String.Empty; } } ///Appends the current text content value to already held values. /// Current text content value. internal void Append(string value) { if (this.elementValue == null) { this.elementValue = value; } else if (this.builder == null) { string newValue = value; this.builder = new StringBuilder(elementValue.Length + newValue.Length) .Append(elementValue) .Append(newValue); } else { this.builder.Append(value); } } ///Once value is read, resets the current content to null. internal void Reset() { this.elementValue = null; this.builder = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
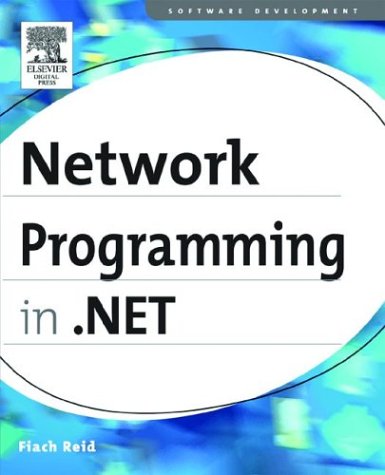
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LineBreak.cs
- HotSpotCollection.cs
- TransactionInformation.cs
- AlignmentXValidation.cs
- FormViewInsertEventArgs.cs
- XPathMessageFilter.cs
- ComponentResourceKey.cs
- LoginView.cs
- XmlFormatExtensionAttribute.cs
- Dictionary.cs
- HTTPNotFoundHandler.cs
- SqlNode.cs
- XmlObjectSerializerReadContext.cs
- ProjectionQueryOptionExpression.cs
- ProxyFragment.cs
- Container.cs
- PartBasedPackageProperties.cs
- TypeResolvingOptionsAttribute.cs
- FileLogRecordHeader.cs
- VirtualPath.cs
- DoubleAnimationUsingPath.cs
- EventOpcode.cs
- RequestContext.cs
- EtwTrackingBehaviorElement.cs
- DrawingContextDrawingContextWalker.cs
- CheckBox.cs
- DataGridViewIntLinkedList.cs
- DocumentSchemaValidator.cs
- CodeTypeMember.cs
- UriExt.cs
- Transaction.cs
- InputBindingCollection.cs
- FlagsAttribute.cs
- AsyncResult.cs
- ObjectSet.cs
- ThreadStartException.cs
- XmlIgnoreAttribute.cs
- DataPagerFieldCollection.cs
- Clipboard.cs
- MemberRelationshipService.cs
- IndentedTextWriter.cs
- XmlWrappingWriter.cs
- XmlQueryOutput.cs
- AssociationEndMember.cs
- DelegateBodyWriter.cs
- HttpServerProtocol.cs
- mansign.cs
- DataError.cs
- Emitter.cs
- PropertyFilterAttribute.cs
- GenericTypeParameterBuilder.cs
- HttpVersion.cs
- ReversePositionQuery.cs
- VolatileResourceManager.cs
- HtmlContainerControl.cs
- WmpBitmapEncoder.cs
- ListBase.cs
- VideoDrawing.cs
- SystemDropShadowChrome.cs
- AspProxy.cs
- ApplicationCommands.cs
- DataGridColumnHeader.cs
- Constraint.cs
- ClientScriptManagerWrapper.cs
- WindowsFormsHelpers.cs
- VersionedStreamOwner.cs
- RawStylusInputCustomDataList.cs
- ImportException.cs
- HwndAppCommandInputProvider.cs
- GACIdentityPermission.cs
- AnnotationComponentChooser.cs
- FocusTracker.cs
- MaskDesignerDialog.cs
- COM2PropertyBuilderUITypeEditor.cs
- FieldToken.cs
- XmlnsDictionary.cs
- ThousandthOfEmRealDoubles.cs
- NodeFunctions.cs
- SoapObjectReader.cs
- JulianCalendar.cs
- BrowserCapabilitiesFactoryBase.cs
- DefaultValueConverter.cs
- HttpResponseHeader.cs
- ToolStripScrollButton.cs
- MarshalByValueComponent.cs
- XsltLoader.cs
- ProcessThreadCollection.cs
- CodeMemberMethod.cs
- CollectionsUtil.cs
- _HelperAsyncResults.cs
- SymbolEqualComparer.cs
- LoginUtil.cs
- Tokenizer.cs
- ValueConversionAttribute.cs
- Vector3DKeyFrameCollection.cs
- AppModelKnownContentFactory.cs
- AttachedPropertyMethodSelector.cs
- SimpleExpression.cs
- BamlBinaryWriter.cs
- AnimationTimeline.cs