Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / AccessibleTech / longhorn / Automation / Win32Providers / MS / Internal / AutomationProxies / ProxyFragment.cs / 1 / ProxyFragment.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Base class for all the Win32 and office Controls. // // The ProxyFragment class is the base class for NODES in the // element tree that are not hwnd based in the case of Win32 controls (e.g. ListViewItem) // // The UIAutomation internal provider does not allow for a node to // iterate through its children. // The ProxyFragment class removes this limitation. // // Class ProxyFragment: // ProxySimple - methods // ElementProviderFromPoint // GetFocus // // // NOTE: ProxyFragment is responsible for hit-testing on its children, since UIAutomation // will not call ElementProviderFromPoint on the Fragment! // // Here are the easy steps for drilling down: // UIAutomation will call ProxyHwnd.ElementProviderFromPoint automatically, // you (implementer) should do a hit test on ProxyHwnd's immediate children only. // If hit-test succeeds and element that you got is ProxyFragment // call ProxyFragment.DrillDownIntoFragment(fragment, x, y) // Each ProxyFragment should implement ElementProviderFromPoint; this method will be used by // DrillDownIntoFragment to drill into the hierarchy of any depth. // Note: You can implement all the drilling down "locally" in your ProxyHwnd.ElementProviderFromPoint, // but it is strongly suggested to do it as described above, so we'll have a generic solution that always works // // // History: // 07/01/2003 : a-jeanp Created // 08/21/2003: alexsn ElementProviderFromPoint corrections // //--------------------------------------------------------------------------- using System; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.ComponentModel; using System.Collections; using System.Runtime.InteropServices; namespace MS.Internal.AutomationProxies { // Base Class for all the Windows Control that supports navigation. // Implements the default behaviors class ProxyFragment : ProxySimple, IRawElementProviderFragmentRoot { // ----------------------------------------------------- // // Constructors // // ----------------------------------------------------- #region Constructors internal ProxyFragment (IntPtr hwnd, ProxyFragment parent, int item) : base (hwnd, parent, item) {} #endregion // ------------------------------------------------------ // // Patterns Implementation // // ----------------------------------------------------- #region ProxyFragment Methods // ------------------------------------------------------ // // Default implementation for ProxyFragment members // // ------------------------------------------------------ // Next Silbing: assumes none, must be overloaded by a subclass if any // The method is called on the parent with a reference to the child. // This makes the implementation a lot more clean than the UIAutomation call internal virtual ProxySimple GetNextSibling (ProxySimple child) { return null; } // Prev Silbing: assumes none, must be overloaded by a subclass if any // The method is called on the parent with a reference to the child. // This makes the implementation a lot more clean than the UIAutomation call internal virtual ProxySimple GetPreviousSibling (ProxySimple child) { return null; } // GetFirstChild: assumes none, must be overloaded by a subclass if any internal virtual ProxySimple GetFirstChild () { return null; } // GetLastChild: assumes none, must be overloaded by a subclass if any internal virtual ProxySimple GetLastChild () { return null; } // Returns a Proxy element corresponding to the specified screen coordinates. // In the derived class implement this method to do fragment specific drill down (if needed) // Fragment should drill down only among its immediate children. internal virtual ProxySimple ElementProviderFromPoint (int x, int y) { return this; } // Returns an item corresponding to the focused element (if there is one), or null otherwise. internal virtual ProxySimple GetFocus () { return this is ProxyHwnd ? this : null; } #endregion #region IRawElementProviderFragmentRoot Interface // NOTE: This method should only be called (by Proxy or/and UIAutomation) on Proxies of type // ProxyHwnd. Anything else indicates a problem IRawElementProviderFragment IRawElementProviderFragmentRoot.ElementProviderFromPoint (double x, double y) { System.Diagnostics.Debug.Assert(this is ProxyHwnd, "Invalid method called ElementProviderFromPoint"); // Do the proper rounding. return ElementProviderFromPoint ((int) (x + 0.5), (int) (y + 0.5)); } IRawElementProviderFragment IRawElementProviderFragmentRoot.GetFocus () { return GetFocus (); } #endregion #region IRawElementProviderFragment Interface // Request to return the element in the specified direction IRawElementProviderFragment IRawElementProviderFragment.Navigate(NavigateDirection direction) { switch (direction) { case NavigateDirection.NextSibling : { // NOTE: Do not use GetParent(), call _parent explicitly return _fSubTree ? _parent.GetNextSibling (this) : null; } case NavigateDirection.PreviousSibling : { // NOTE: Do not use GetParent(), call _parent explicitly return _fSubTree ? _parent.GetPreviousSibling (this) : null; } case NavigateDirection.FirstChild : { return GetFirstChild (); } case NavigateDirection.LastChild : { return GetLastChild (); } case NavigateDirection.Parent : { return GetParent (); } default : { return null; } } } #endregion //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods // Recursively Raise an Event for all the sub elements override internal void RecursiveRaiseEvents (object idProp, AutomationPropertyChangedEventArgs e) { AutomationInteropProvider.RaiseAutomationPropertyChangedEvent (this, e); for (ProxySimple el = GetFirstChild (); el != null; el = this.GetNextSibling (el)) { el.RecursiveRaiseEvents (idProp, e); } } #endregion //----------------------------------------------------- // // Protected Methods // //----------------------------------------------------- #region Protected Methods // This method will return the leaf element that lives in ProxyFragment at Point(x,y) static internal ProxySimple DrillDownFragment(ProxyFragment fragment, int x, int y) { System.Diagnostics.Debug.Assert(fragment != null, "DrillDownFragment: starting point is null"); // drill down ProxySimple fromPoint = fragment.ElementProviderFromPoint(x, y); System.Diagnostics.Debug.Assert(fromPoint != null, @"DrillDownFragment: calling ElementProviderFromPoint on Fragment should not return null"); // Check if we got back a new fragment // do this check before trying to cast to ProxyFragment if (fragment == fromPoint || Misc.Compare(fragment, fromPoint)) { // Point was on the fragment // but not on any element that lives inside of the fragment return fragment; } fragment = fromPoint as ProxyFragment; if (fragment == null) { // we got back a simple element return fromPoint; } // Got a new fragment, continue drilling return DrillDownFragment(fragment, x, y); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
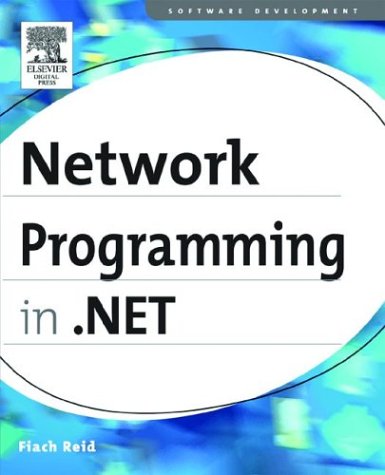
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MSG.cs
- WorkflowRuntimeServiceElementCollection.cs
- MimeParameter.cs
- PersistenceParticipant.cs
- ContentValidator.cs
- XPathItem.cs
- PeerToPeerException.cs
- TextServicesDisplayAttributePropertyRanges.cs
- SQLSingleStorage.cs
- SafeRightsManagementPubHandle.cs
- DataRowExtensions.cs
- SafeMILHandle.cs
- TripleDES.cs
- RemotingConfigParser.cs
- ColorKeyFrameCollection.cs
- XmlSchemaCompilationSettings.cs
- TimeSpanValidator.cs
- SymbolType.cs
- Propagator.ExtentPlaceholderCreator.cs
- BevelBitmapEffect.cs
- MailAddressCollection.cs
- Window.cs
- PasswordPropertyTextAttribute.cs
- DBPropSet.cs
- SerializationHelper.cs
- CuspData.cs
- SharedPersonalizationStateInfo.cs
- ListenerAdapterBase.cs
- IncrementalHitTester.cs
- EntityReference.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- SystemInfo.cs
- XmlSchemaRedefine.cs
- _UriTypeConverter.cs
- XmlSecureResolver.cs
- CallbackValidator.cs
- SizeLimitedCache.cs
- MediaSystem.cs
- TimeStampChecker.cs
- SharedUtils.cs
- RemoteWebConfigurationHostServer.cs
- IPPacketInformation.cs
- EpmCustomContentDeSerializer.cs
- BigInt.cs
- WebPartZoneCollection.cs
- ListViewItem.cs
- BaseTemplateBuildProvider.cs
- UiaCoreApi.cs
- ConfigXmlComment.cs
- MultipartContentParser.cs
- TemplatedMailWebEventProvider.cs
- FixedDSBuilder.cs
- SoapAttributeAttribute.cs
- HtmlInputControl.cs
- WindowProviderWrapper.cs
- JpegBitmapDecoder.cs
- FamilyMapCollection.cs
- UdpSocket.cs
- Window.cs
- ClientConfigurationHost.cs
- EventMappingSettings.cs
- ResXBuildProvider.cs
- PerCallInstanceContextProvider.cs
- AppDomainFactory.cs
- SchemaNamespaceManager.cs
- OrderByQueryOptionExpression.cs
- WebEvents.cs
- MenuItemBinding.cs
- ConfigurationLocation.cs
- PerformanceCounterPermission.cs
- PostBackTrigger.cs
- TableAutomationPeer.cs
- Closure.cs
- WebBrowserNavigatedEventHandler.cs
- TemplateApplicationHelper.cs
- DateTimeUtil.cs
- ManagedCodeMarkers.cs
- PreservationFileWriter.cs
- EventBookmark.cs
- XmlSchemaSimpleTypeRestriction.cs
- TemplateBaseAction.cs
- Command.cs
- XNodeNavigator.cs
- DocumentScope.cs
- Rectangle.cs
- DataContractJsonSerializer.cs
- WindowsTab.cs
- ConfigXmlSignificantWhitespace.cs
- EventLogHandle.cs
- InternalMappingException.cs
- SqlNode.cs
- _NegoState.cs
- XPathNavigator.cs
- Brush.cs
- XmlReaderSettings.cs
- NativeMethods.cs
- HMACSHA512.cs
- TextServicesManager.cs
- SqlDataSourceQueryEditorForm.cs
- XmlSignificantWhitespace.cs