Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / MetadataPropertyCollection.cs / 1305376 / MetadataPropertyCollection.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; using System.Reflection; using System.Data.Common.Utils; namespace System.Data.Metadata.Edm { ////// Metadata collection class supporting delay-loading of system item attributes and /// extended attributes. /// internal sealed class MetadataPropertyCollection : MetadataCollection{ /// /// Constructor taking item. /// /// Item with which the collection is associated. internal MetadataPropertyCollection(MetadataItem item) : base(GetSystemMetadataProperties(item)) { } private readonly static Memoizers_itemTypeMemoizer = new Memoizer (clrType => new ItemTypeInformation(clrType), null); // Given an item, returns all system type attributes for the item. private static IEnumerable GetSystemMetadataProperties(MetadataItem item) { EntityUtil.CheckArgumentNull(item, "item"); Type type = item.GetType(); ItemTypeInformation itemTypeInformation = GetItemTypeInformation(type); return itemTypeInformation.GetItemAttributes(item); } // Retrieves metadata for type. private static ItemTypeInformation GetItemTypeInformation(Type clrType) { return s_itemTypeMemoizer.Evaluate(clrType); } /// /// Encapsulates information about system item attributes for a particular item type. /// private class ItemTypeInformation { ////// Retrieves system attribute information for the given type. /// Requires: type must derive from MetadataItem /// /// Type internal ItemTypeInformation(Type clrType) { Debug.Assert(null != clrType); _itemProperties = GetItemProperties(clrType); } private readonly List_itemProperties; // Returns system item attributes for the given item. internal IEnumerable GetItemAttributes(MetadataItem item) { foreach (ItemPropertyInfo propertyInfo in _itemProperties) { yield return propertyInfo.GetMetadataProperty(item); } } // Gets type information for item with the given type. Uses cached information where // available. private static List GetItemProperties(Type clrType) { List result = new List (); foreach (PropertyInfo propertyInfo in clrType.GetProperties(BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic)) { foreach (MetadataPropertyAttribute attribute in propertyInfo.GetCustomAttributes( typeof(MetadataPropertyAttribute), false)) { result.Add(new ItemPropertyInfo(propertyInfo, attribute)); } } return result; } } /// /// Encapsulates information about a CLR property of an item class. /// private class ItemPropertyInfo { ////// Initialize information. /// Requires: attribute must belong to the given property. /// /// Property referenced. /// Attribute for the property. internal ItemPropertyInfo(PropertyInfo propertyInfo, MetadataPropertyAttribute attribute) { Debug.Assert(null != propertyInfo); Debug.Assert(null != attribute); _propertyInfo = propertyInfo; _attribute = attribute; } private readonly MetadataPropertyAttribute _attribute; private readonly PropertyInfo _propertyInfo; ////// Given an item, returns an instance of the item attribute described by this class. /// /// Item from which to retrieve attribute. ///Item attribute. internal MetadataProperty GetMetadataProperty(MetadataItem item) { return new MetadataProperty(_propertyInfo.Name, _attribute.Type, _attribute.IsCollectionType, new MetadataPropertyValue(_propertyInfo, item)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; using System.Reflection; using System.Data.Common.Utils; namespace System.Data.Metadata.Edm { ////// Metadata collection class supporting delay-loading of system item attributes and /// extended attributes. /// internal sealed class MetadataPropertyCollection : MetadataCollection{ /// /// Constructor taking item. /// /// Item with which the collection is associated. internal MetadataPropertyCollection(MetadataItem item) : base(GetSystemMetadataProperties(item)) { } private readonly static Memoizers_itemTypeMemoizer = new Memoizer (clrType => new ItemTypeInformation(clrType), null); // Given an item, returns all system type attributes for the item. private static IEnumerable GetSystemMetadataProperties(MetadataItem item) { EntityUtil.CheckArgumentNull(item, "item"); Type type = item.GetType(); ItemTypeInformation itemTypeInformation = GetItemTypeInformation(type); return itemTypeInformation.GetItemAttributes(item); } // Retrieves metadata for type. private static ItemTypeInformation GetItemTypeInformation(Type clrType) { return s_itemTypeMemoizer.Evaluate(clrType); } /// /// Encapsulates information about system item attributes for a particular item type. /// private class ItemTypeInformation { ////// Retrieves system attribute information for the given type. /// Requires: type must derive from MetadataItem /// /// Type internal ItemTypeInformation(Type clrType) { Debug.Assert(null != clrType); _itemProperties = GetItemProperties(clrType); } private readonly List_itemProperties; // Returns system item attributes for the given item. internal IEnumerable GetItemAttributes(MetadataItem item) { foreach (ItemPropertyInfo propertyInfo in _itemProperties) { yield return propertyInfo.GetMetadataProperty(item); } } // Gets type information for item with the given type. Uses cached information where // available. private static List GetItemProperties(Type clrType) { List result = new List (); foreach (PropertyInfo propertyInfo in clrType.GetProperties(BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic)) { foreach (MetadataPropertyAttribute attribute in propertyInfo.GetCustomAttributes( typeof(MetadataPropertyAttribute), false)) { result.Add(new ItemPropertyInfo(propertyInfo, attribute)); } } return result; } } /// /// Encapsulates information about a CLR property of an item class. /// private class ItemPropertyInfo { ////// Initialize information. /// Requires: attribute must belong to the given property. /// /// Property referenced. /// Attribute for the property. internal ItemPropertyInfo(PropertyInfo propertyInfo, MetadataPropertyAttribute attribute) { Debug.Assert(null != propertyInfo); Debug.Assert(null != attribute); _propertyInfo = propertyInfo; _attribute = attribute; } private readonly MetadataPropertyAttribute _attribute; private readonly PropertyInfo _propertyInfo; ////// Given an item, returns an instance of the item attribute described by this class. /// /// Item from which to retrieve attribute. ///Item attribute. internal MetadataProperty GetMetadataProperty(MetadataItem item) { return new MetadataProperty(_propertyInfo.Name, _attribute.Type, _attribute.IsCollectionType, new MetadataPropertyValue(_propertyInfo, item)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
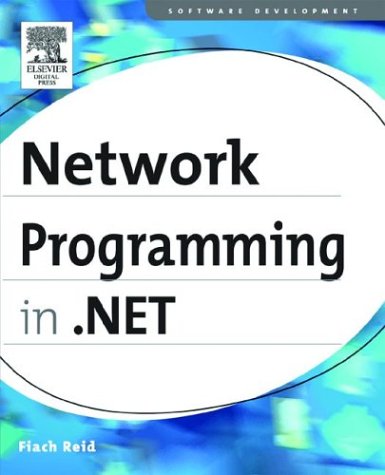
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PreviewKeyDownEventArgs.cs
- Msmq.cs
- EntityStoreSchemaFilterEntry.cs
- ChannelBinding.cs
- XmlValidatingReaderImpl.cs
- FamilyTypeface.cs
- PathFigureCollection.cs
- ActionFrame.cs
- HostedTransportConfigurationBase.cs
- ProxyWebPart.cs
- SqlConnectionHelper.cs
- XPathSingletonIterator.cs
- WindowsSlider.cs
- ThicknessAnimationBase.cs
- InternalCache.cs
- SqlColumnizer.cs
- LinkClickEvent.cs
- ListViewSelectEventArgs.cs
- XmlAttributeOverrides.cs
- exports.cs
- CodeBlockBuilder.cs
- SystemIPInterfaceProperties.cs
- EntityParameter.cs
- Int32Rect.cs
- PagePropertiesChangingEventArgs.cs
- DeviceSpecificChoiceCollection.cs
- LinqDataSourceInsertEventArgs.cs
- InArgumentConverter.cs
- Number.cs
- ExceptionTranslationTable.cs
- XmlElement.cs
- CollectionBase.cs
- ConfigurationStrings.cs
- CellTreeNode.cs
- DocumentScope.cs
- HierarchicalDataBoundControl.cs
- MsmqIntegrationBinding.cs
- MarkupCompilePass2.cs
- SeekStoryboard.cs
- HandlerBase.cs
- LicFileLicenseProvider.cs
- ErrorTableItemStyle.cs
- ToolStripSystemRenderer.cs
- TextProperties.cs
- WindowsTreeView.cs
- XmlReflectionImporter.cs
- CodeTypeDeclarationCollection.cs
- DBPropSet.cs
- odbcmetadatacolumnnames.cs
- HandleCollector.cs
- EllipticalNodeOperations.cs
- LoginUtil.cs
- ContentPresenter.cs
- Timer.cs
- XmlDocumentType.cs
- ProfilePropertySettings.cs
- HttpCacheVary.cs
- PerformanceCounterPermission.cs
- HtmlWindowCollection.cs
- CheckBoxList.cs
- PeerServiceMessageContracts.cs
- MULTI_QI.cs
- CodeTypeParameter.cs
- DataSource.cs
- ValidationPropertyAttribute.cs
- OdbcUtils.cs
- ToolStripMenuItem.cs
- UriParserTemplates.cs
- AmbientLight.cs
- SignatureDescription.cs
- ContextBase.cs
- LinqDataSourceSelectEventArgs.cs
- FunctionCommandText.cs
- AutoScrollExpandMessageFilter.cs
- BinaryWriter.cs
- NamedPermissionSet.cs
- XmlIlGenerator.cs
- TextSchema.cs
- PropertyDescriptor.cs
- Separator.cs
- GeometryDrawing.cs
- DescendantQuery.cs
- ExecutionContext.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- DrawingContext.cs
- ArrayExtension.cs
- WindowsProgressbar.cs
- DataServiceEntityAttribute.cs
- SocketElement.cs
- SubclassTypeValidatorAttribute.cs
- ComponentChangingEvent.cs
- LineGeometry.cs
- PageContent.cs
- BitConverter.cs
- PrintEvent.cs
- ListBoxChrome.cs
- Point3DAnimationUsingKeyFrames.cs
- DataGridViewImageColumn.cs
- XmlNodeList.cs
- MSAANativeProvider.cs