Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / DrawingContext.cs / 1 / DrawingContext.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: DrawingContext.cs // // History: // GSchneid: 04/19/2003 // Created it based on the RetainedDrawingContext used in the AvPhat branch. // adsmith: 07/02/2003 // Turned this class into an abstract base class for RetainedDrawingContext // and PaintingDrawingContext. // //----------------------------------------------------------------------------- using System; using System.Security.Permissions; using System.Windows; using System.Windows.Threading; using System.Windows.Media.Animation; using System.Windows.Media; using System.Diagnostics; using MS.Internal; using System.Runtime.InteropServices; using MS.Utility; namespace System.Windows.Media { ////// Drawing context. /// public abstract partial class DrawingContext : DispatcherObject, IDisposable { #region Constructors ////// Default constructor for DrawingContext - this uses the current Dispatcher. /// internal DrawingContext() { // Nothing to do here } #endregion Constructors #region Public Methods ////// Draw Text at the location specified. /// /// The FormattedText to draw. /// The location at which to draw the text. ////// This call is illegal if this object has already been closed or disposed. /// public void DrawText(FormattedText formattedText, Point origin) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.GuidFromId(EventTraceGuidId.GENERICSTRINGGUID), EventType.StartEvent, "DrawingContext.DrawText Start"); } VerifyApiNonstructuralChange(); #if DEBUG MediaTrace.DrawingContextOp.Trace("DrawText(const)"); #endif if (formattedText == null) { return; } formattedText.Draw(this, origin); if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.GuidFromId(EventTraceGuidId.GENERICSTRINGGUID), EventType.EndEvent, "DrawingContext.DrawText End"); } } ////// Closes the DrawingContext and flushes the content. /// Afterwards the DrawingContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// public abstract void Close(); ////// This is the same as the Close call: /// Closes the DrawingContext and flushes the content. /// Afterwards the DrawingContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// void IDisposable.Dispose() { // Call a virtual method for derived Dispose implementations // // Attempting to override a explicit interface member implementation causes // the most-derived implementation to always be called, and the base // implementation becomes uncallable. But FxCop requires the base Dispose // method is always be called. To avoid this situation, we use the *Core // pattern for derived classes, instead of attempting to override // IDisposable.Dispose. VerifyAccess(); DisposeCore(); } #endregion Public Methods #region Protected Methods ////// Dispose functionality implemented by subclasses /// ////// This call is illegal if this object has already been closed or disposed. /// protected abstract void DisposeCore(); ////// This verifies that the API can be called for read only access. /// protected virtual void VerifyApiNonstructuralChange() { this.VerifyAccess(); } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: DrawingContext.cs // // History: // GSchneid: 04/19/2003 // Created it based on the RetainedDrawingContext used in the AvPhat branch. // adsmith: 07/02/2003 // Turned this class into an abstract base class for RetainedDrawingContext // and PaintingDrawingContext. // //----------------------------------------------------------------------------- using System; using System.Security.Permissions; using System.Windows; using System.Windows.Threading; using System.Windows.Media.Animation; using System.Windows.Media; using System.Diagnostics; using MS.Internal; using System.Runtime.InteropServices; using MS.Utility; namespace System.Windows.Media { ////// Drawing context. /// public abstract partial class DrawingContext : DispatcherObject, IDisposable { #region Constructors ////// Default constructor for DrawingContext - this uses the current Dispatcher. /// internal DrawingContext() { // Nothing to do here } #endregion Constructors #region Public Methods ////// Draw Text at the location specified. /// /// The FormattedText to draw. /// The location at which to draw the text. ////// This call is illegal if this object has already been closed or disposed. /// public void DrawText(FormattedText formattedText, Point origin) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.GuidFromId(EventTraceGuidId.GENERICSTRINGGUID), EventType.StartEvent, "DrawingContext.DrawText Start"); } VerifyApiNonstructuralChange(); #if DEBUG MediaTrace.DrawingContextOp.Trace("DrawText(const)"); #endif if (formattedText == null) { return; } formattedText.Draw(this, origin); if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.GuidFromId(EventTraceGuidId.GENERICSTRINGGUID), EventType.EndEvent, "DrawingContext.DrawText End"); } } ////// Closes the DrawingContext and flushes the content. /// Afterwards the DrawingContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// public abstract void Close(); ////// This is the same as the Close call: /// Closes the DrawingContext and flushes the content. /// Afterwards the DrawingContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// void IDisposable.Dispose() { // Call a virtual method for derived Dispose implementations // // Attempting to override a explicit interface member implementation causes // the most-derived implementation to always be called, and the base // implementation becomes uncallable. But FxCop requires the base Dispose // method is always be called. To avoid this situation, we use the *Core // pattern for derived classes, instead of attempting to override // IDisposable.Dispose. VerifyAccess(); DisposeCore(); } #endregion Public Methods #region Protected Methods ////// Dispose functionality implemented by subclasses /// ////// This call is illegal if this object has already been closed or disposed. /// protected abstract void DisposeCore(); ////// This verifies that the API can be called for read only access. /// protected virtual void VerifyApiNonstructuralChange() { this.VerifyAccess(); } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
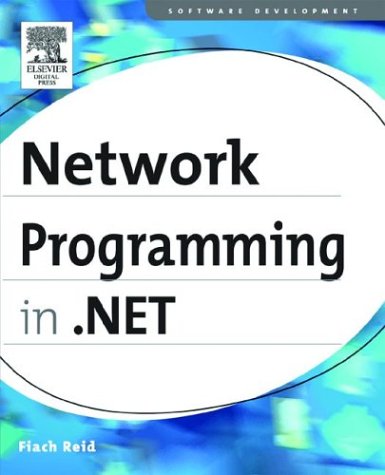
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaAnnotated.cs
- DataStreams.cs
- DetailsViewPageEventArgs.cs
- DSACryptoServiceProvider.cs
- CreateUserErrorEventArgs.cs
- DynamicQueryableWrapper.cs
- coordinator.cs
- Matrix.cs
- CompositeControlDesigner.cs
- DrawTreeNodeEventArgs.cs
- SecurityState.cs
- ContextBase.cs
- Debug.cs
- GetPageCompletedEventArgs.cs
- AttachedPropertyMethodSelector.cs
- WebPartTransformerCollection.cs
- CanonicalFontFamilyReference.cs
- GridViewUpdatedEventArgs.cs
- XmlSchemaExporter.cs
- TdsParserHelperClasses.cs
- IndentTextWriter.cs
- ExtensionQuery.cs
- InvokeMethodActivityDesigner.cs
- ProfileParameter.cs
- ToolStripKeyboardHandlingService.cs
- ExtensibleClassFactory.cs
- SerializableReadOnlyDictionary.cs
- AppearanceEditorPart.cs
- InfiniteIntConverter.cs
- WSSecurityPolicy.cs
- X509PeerCertificateElement.cs
- SchemaImporterExtensionsSection.cs
- XmlHierarchicalDataSourceView.cs
- BrowserCapabilitiesCodeGenerator.cs
- mansign.cs
- ProgressBarAutomationPeer.cs
- CaseDesigner.xaml.cs
- ProcessHostFactoryHelper.cs
- cookie.cs
- Model3D.cs
- PtsHost.cs
- NodeFunctions.cs
- TraceUtility.cs
- TraceHandlerErrorFormatter.cs
- GatewayIPAddressInformationCollection.cs
- DecimalConstantAttribute.cs
- VirtualPathProvider.cs
- Viewport2DVisual3D.cs
- SystemIcmpV4Statistics.cs
- UrlEncodedParameterWriter.cs
- RubberbandSelector.cs
- ClockController.cs
- LinqDataSourceContextEventArgs.cs
- ModelFunctionTypeElement.cs
- EntityCommandCompilationException.cs
- PipelineComponent.cs
- QilXmlReader.cs
- DesignerAutoFormatStyle.cs
- IChannel.cs
- BindingsCollection.cs
- SettingsPropertyCollection.cs
- PictureBoxDesigner.cs
- UrlMappingCollection.cs
- ListViewUpdateEventArgs.cs
- SqlNodeAnnotation.cs
- OleDbCommandBuilder.cs
- SiteMapProvider.cs
- ListViewDataItem.cs
- oledbconnectionstring.cs
- WebPartTransformer.cs
- MILUtilities.cs
- DataControlPagerLinkButton.cs
- FormsIdentity.cs
- SudsWriter.cs
- PropertyGrid.cs
- ScopeCollection.cs
- DSASignatureFormatter.cs
- WinFormsComponentEditor.cs
- ComboBoxRenderer.cs
- XmlSerializableReader.cs
- MenuCommand.cs
- EndPoint.cs
- ZipArchive.cs
- ObjectTag.cs
- DynamicRendererThreadManager.cs
- LocalizableResourceBuilder.cs
- Tablet.cs
- NodeLabelEditEvent.cs
- ActivitiesCollection.cs
- Rethrow.cs
- ComponentCommands.cs
- InvalidateEvent.cs
- CompModSwitches.cs
- SqlAliasesReferenced.cs
- FileNameEditor.cs
- DataGridViewCellStyle.cs
- XmlBufferReader.cs
- DocumentPage.cs
- XmlDataSourceNodeDescriptor.cs
- Literal.cs