Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / PictureBoxDesigner.cs / 1 / PictureBoxDesigner.cs
[assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.PictureBoxDesigner..ctor()")] namespace System.Windows.Forms.Design { using System.Diagnostics; using System; using System.Design; using System.Windows.Forms; using System.Drawing; using Microsoft.Win32; using System.Drawing.Drawing2D; using System.ComponentModel; using System.Drawing.Design; using System.ComponentModel.Design; using System.Collections; ////// /// This class handles all design time behavior for the group box class. Group /// boxes may contain sub-components and therefore use the frame designer. /// internal class PictureBoxDesigner : ControlDesigner { private DesignerActionListCollection _actionLists; public PictureBoxDesigner() { AutoResizeHandles = true; } ////// /// This draws a nice border around our pictureBox. We need /// this because the pictureBox can have no border and you can't /// tell where it is. /// ///private void DrawBorder(Graphics graphics) { Control ctl = Control; Rectangle rc = ctl.ClientRectangle; Color penColor; // Black or white pen? Depends on the color of the control. // if (ctl.BackColor.GetBrightness() < .5) { penColor = ControlPaint.Light(ctl.BackColor); } else { penColor = ControlPaint.Dark(ctl.BackColor);; } Pen pen = new Pen(penColor); pen.DashStyle = DashStyle.Dash; rc.Width --; rc.Height--; graphics.DrawRectangle(pen, rc); pen.Dispose(); } /// /// /// Overrides our base class. Here we check to see if there /// is no border on the pictureBox. If not, we draw one so that /// the pictureBox shape is visible at design time. /// protected override void OnPaintAdornments(PaintEventArgs pe) { PictureBox pictureBox = (PictureBox)Component; if (pictureBox.BorderStyle == BorderStyle.None) { DrawBorder(pe.Graphics); } base.OnPaintAdornments(pe); } ////// /// Retrieves a set of rules concerning the movement capabilities of a component. /// This should be one or more flags from the SelectionRules class. If no designer /// provides rules for a component, the component will not get any UI services. /// public override SelectionRules SelectionRules { get { SelectionRules rules = base.SelectionRules; object component = Component; PropertyDescriptor propSizeMode = TypeDescriptor.GetProperties(Component)["SizeMode"]; if (propSizeMode != null) { PictureBoxSizeMode sizeMode = (PictureBoxSizeMode)propSizeMode.GetValue(component); if (sizeMode == PictureBoxSizeMode.AutoSize) { rules &= ~SelectionRules.AllSizeable; } } return rules; } } public override DesignerActionListCollection ActionLists { get { if (_actionLists == null) { _actionLists = new DesignerActionListCollection(); _actionLists.Add(new PictureBoxActionList(this)); } return _actionLists; } } } internal class PictureBoxActionList : DesignerActionList { private PictureBoxDesigner _designer; public PictureBoxActionList(PictureBoxDesigner designer) : base(designer.Component) { _designer = designer; } public PictureBoxSizeMode SizeMode { get { return ((PictureBox)Component).SizeMode; } set { TypeDescriptor.GetProperties(Component)["SizeMode"].SetValue(Component, value); } } public void ChooseImage() { EditorServiceContext.EditValue(_designer, Component, "Image"); } public override DesignerActionItemCollection GetSortedActionItems() { DesignerActionItemCollection items = new DesignerActionItemCollection(); items.Add(new DesignerActionMethodItem(this, "ChooseImage", SR.GetString(SR.ChooseImageDisplayName), SR.GetString(SR.PropertiesCategoryName), SR.GetString(SR.ChooseImageDescription), true)); items.Add(new DesignerActionPropertyItem("SizeMode", SR.GetString(SR.SizeModeDisplayName), SR.GetString(SR.PropertiesCategoryName), SR.GetString(SR.SizeModeDescription))); return items; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
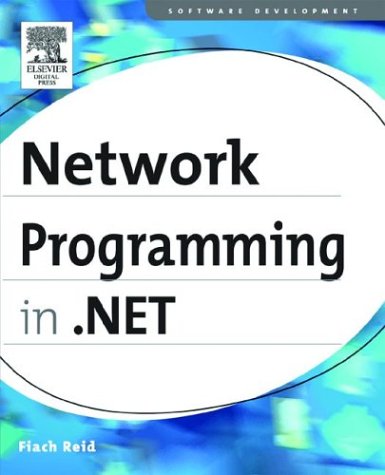
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- arabicshape.cs
- SHA256Cng.cs
- RewritingValidator.cs
- ToolStripDropTargetManager.cs
- FixedSOMContainer.cs
- BeginCreateSecurityTokenRequest.cs
- WebPartManagerInternals.cs
- DataSourceXmlAttributeAttribute.cs
- LocalServiceSecuritySettings.cs
- EventItfInfo.cs
- UnitySerializationHolder.cs
- Package.cs
- ReferenceSchema.cs
- DrawListViewColumnHeaderEventArgs.cs
- ObjectFullSpanRewriter.cs
- ScrollPatternIdentifiers.cs
- UserValidatedEventArgs.cs
- SecurityUtils.cs
- UInt64.cs
- SpAudioStreamWrapper.cs
- FrameSecurityDescriptor.cs
- RangeValuePattern.cs
- PrivilegedConfigurationManager.cs
- ApplicationServiceManager.cs
- AlignmentXValidation.cs
- SHA1.cs
- LineInfo.cs
- AsymmetricAlgorithm.cs
- WebPartDisplayModeEventArgs.cs
- CommandLineParser.cs
- DBConnection.cs
- CodeConstructor.cs
- XamlSerializer.cs
- BamlLocalizabilityResolver.cs
- DateTimeUtil.cs
- LogEntrySerializer.cs
- RenderingBiasValidation.cs
- FlowPosition.cs
- ServicePoint.cs
- JpegBitmapEncoder.cs
- DesignerSerializationVisibilityAttribute.cs
- UnrecognizedAssertionsBindingElement.cs
- CommaDelimitedStringAttributeCollectionConverter.cs
- RegexFCD.cs
- LambdaCompiler.Address.cs
- ThaiBuddhistCalendar.cs
- MediaSystem.cs
- Operators.cs
- TextPatternIdentifiers.cs
- Scanner.cs
- ClientWindowsAuthenticationMembershipProvider.cs
- HtmlInputCheckBox.cs
- StateFinalizationActivity.cs
- SplashScreenNativeMethods.cs
- BitArray.cs
- ViewRendering.cs
- RubberbandSelector.cs
- ResourcePermissionBase.cs
- StateInitializationDesigner.cs
- ToolStripRenderer.cs
- WebPartEditorApplyVerb.cs
- UTF32Encoding.cs
- RectangleF.cs
- IteratorDescriptor.cs
- Group.cs
- FrameSecurityDescriptor.cs
- ProcessThread.cs
- ListViewUpdatedEventArgs.cs
- KeysConverter.cs
- EventSinkHelperWriter.cs
- TextEffectResolver.cs
- PageAdapter.cs
- IntegerFacetDescriptionElement.cs
- RuleInfoComparer.cs
- ToolBar.cs
- FormViewPagerRow.cs
- WaitForChangedResult.cs
- _BasicClient.cs
- ReflectionPermission.cs
- ListParagraph.cs
- XmlWrappingReader.cs
- CssClassPropertyAttribute.cs
- oledbmetadatacolumnnames.cs
- AssemblyInfo.cs
- PropertyInfoSet.cs
- RtfToXamlReader.cs
- IndicShape.cs
- AlphaSortedEnumConverter.cs
- BindToObject.cs
- WebSysDefaultValueAttribute.cs
- BitmapInitialize.cs
- HandleCollector.cs
- DataRecord.cs
- VirtualPathProvider.cs
- IndexedGlyphRun.cs
- ListCollectionView.cs
- ConfigUtil.cs
- ObjectComplexPropertyMapping.cs
- LocalBuilder.cs
- WsrmFault.cs