Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / TextEffectResolver.cs / 1 / TextEffectResolver.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Text Effect Setter // // History: // 3/24/2004 garyyang Created // 10/28/2004 garyyang rewrite the helper class // //--------------------------------------------------------------------------- using System.Collections.Generic; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.Text; namespace System.Windows.Documents { ////// Helper class to help set text effects into the Text container /// public static class TextEffectResolver { //--------------------------- // public static methods //--------------------------- ////// resolves text effect on a text range to a list of text effect targets. /// The method will walk the text and perform the following task: /// 1) For each continous block of text, create a text effect targeting the scoping element /// 2) For the text effect created, calculate the starting cp index and cp count for the effect /// /// The method will create freezable copy of the TextEffect passed in and fill in /// CharacterIndex and Count for the range. /// /// starting text pointer /// end text pointer /// effect that is apply on the text public static TextEffectTarget[] Resolve( TextPointer startPosition, TextPointer endPosition, TextEffect effect ) { if (effect == null) throw new ArgumentNullException("effect"); ValidationHelper.VerifyPositionPair(startPosition, endPosition); TextPointer effectStart = new TextPointer(startPosition); // move to the first character symbol at or after Start position MoveToFirstCharacterSymbol(effectStart); TextEffect effectCopy; Listlist = new List (); // we will now traverse the TOM and resolve text effects to the immediate parent // of the characters. We are effectively applying the text effect onto // block of continous text. while (effectStart.CompareTo(endPosition) < 0) { // create a copy of the text effect // so that we can set the CharacterIndex and Count effectCopy = effect.Clone(); // create a position TextPointer continuousTextEnd = new TextPointer(effectStart); // move the position to the end of the continuous text block MoveToFirstNonCharacterSymbol(continuousTextEnd, endPosition); // make sure we are not out of the range continuousTextEnd = (TextPointer)TextPointerBase.Min(continuousTextEnd, endPosition); // set the character index to be the distance from the Start // of this text block to the Start of the text container effectCopy.PositionStart = effectStart.TextContainer.Start.GetOffsetToPosition(effectStart); // count is the distance from the text block start to end effectCopy.PositionCount = effectStart.GetOffsetToPosition(continuousTextEnd); list.Add( new TextEffectTarget( effectStart.Parent, effectCopy ) ); // move the effectStart to the beginning of the next text block. effectStart = continuousTextEnd; MoveToFirstCharacterSymbol(effectStart); } return list.ToArray(); } //--------------------------- // Private static methods //--------------------------- // move to the first character symbol private static void MoveToFirstCharacterSymbol(TextPointer navigator) { while (navigator.GetPointerContext(LogicalDirection.Forward) != TextPointerContext.Text && navigator.MoveToNextContextPosition(LogicalDirection.Forward)) ; } // move to the first non-character symbol, but not pass beyond the limit private static void MoveToFirstNonCharacterSymbol( TextPointer navigator, // navigator to move TextPointer stopHint // don't move further if we already pass beyond this point ) { while (navigator.GetPointerContext(LogicalDirection.Forward) == TextPointerContext.Text && navigator.CompareTo(stopHint) < 0 && navigator.MoveToNextContextPosition(LogicalDirection.Forward)) ; } } /// /// result from TextEffectSetter which contains the TextEffect created and the DependencyObject /// to which the TextEffect should be set. /// public class TextEffectTarget { private DependencyObject _element; private TextEffect _effect; internal TextEffectTarget( DependencyObject element, TextEffect effect ) { if (element == null) throw new ArgumentNullException("element"); if (effect == null) throw new ArgumentNullException("effect"); _element = element; _effect = effect; } ////// The DependencyObject that the TextEffect is targetting. /// public DependencyObject Element { get { return _element; } } ////// The TextEffect /// public TextEffect TextEffect { get { return _effect; } } ////// Enable the TextEffect on the target. If the texteffect is /// already enabled, this will be a no-op. /// public void Enable() { TextEffectCollection textEffects = DynamicPropertyReader.GetTextEffects(_element); if (textEffects == null) { textEffects = new TextEffectCollection(); // use it as reference to avoid creating a copy (Freezable pattern) _element.SetValue(TextElement.TextEffectsProperty, textEffects); } // check whether this instance is already enabled for (int i = 0; i < textEffects.Count; i++) { if (textEffects[i] == _effect) return; // no-op } // use this as reference. textEffects.Add(_effect); } ////// Disable TextEffect on the target. If the texteffect is /// already disabled, this will be a no-op. /// public void Disable() { TextEffectCollection textEffects = DynamicPropertyReader.GetTextEffects(_element); if (textEffects != null) { for (int i = 0; i < textEffects.Count; i++) { if (textEffects[i] == _effect) { // remove the exact instance of the effect from the collection textEffects.RemoveAt(i); return; } } } } ////// Return whether the text effect is enabled on the target element /// public bool IsEnabled { get { TextEffectCollection textEffects = DynamicPropertyReader.GetTextEffects(_element); if (textEffects != null) { for (int i = 0; i < textEffects.Count; i++) { if (textEffects[i] == _effect) { return true; } } } return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Text Effect Setter // // History: // 3/24/2004 garyyang Created // 10/28/2004 garyyang rewrite the helper class // //--------------------------------------------------------------------------- using System.Collections.Generic; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.Text; namespace System.Windows.Documents { ////// Helper class to help set text effects into the Text container /// public static class TextEffectResolver { //--------------------------- // public static methods //--------------------------- ////// resolves text effect on a text range to a list of text effect targets. /// The method will walk the text and perform the following task: /// 1) For each continous block of text, create a text effect targeting the scoping element /// 2) For the text effect created, calculate the starting cp index and cp count for the effect /// /// The method will create freezable copy of the TextEffect passed in and fill in /// CharacterIndex and Count for the range. /// /// starting text pointer /// end text pointer /// effect that is apply on the text public static TextEffectTarget[] Resolve( TextPointer startPosition, TextPointer endPosition, TextEffect effect ) { if (effect == null) throw new ArgumentNullException("effect"); ValidationHelper.VerifyPositionPair(startPosition, endPosition); TextPointer effectStart = new TextPointer(startPosition); // move to the first character symbol at or after Start position MoveToFirstCharacterSymbol(effectStart); TextEffect effectCopy; Listlist = new List (); // we will now traverse the TOM and resolve text effects to the immediate parent // of the characters. We are effectively applying the text effect onto // block of continous text. while (effectStart.CompareTo(endPosition) < 0) { // create a copy of the text effect // so that we can set the CharacterIndex and Count effectCopy = effect.Clone(); // create a position TextPointer continuousTextEnd = new TextPointer(effectStart); // move the position to the end of the continuous text block MoveToFirstNonCharacterSymbol(continuousTextEnd, endPosition); // make sure we are not out of the range continuousTextEnd = (TextPointer)TextPointerBase.Min(continuousTextEnd, endPosition); // set the character index to be the distance from the Start // of this text block to the Start of the text container effectCopy.PositionStart = effectStart.TextContainer.Start.GetOffsetToPosition(effectStart); // count is the distance from the text block start to end effectCopy.PositionCount = effectStart.GetOffsetToPosition(continuousTextEnd); list.Add( new TextEffectTarget( effectStart.Parent, effectCopy ) ); // move the effectStart to the beginning of the next text block. effectStart = continuousTextEnd; MoveToFirstCharacterSymbol(effectStart); } return list.ToArray(); } //--------------------------- // Private static methods //--------------------------- // move to the first character symbol private static void MoveToFirstCharacterSymbol(TextPointer navigator) { while (navigator.GetPointerContext(LogicalDirection.Forward) != TextPointerContext.Text && navigator.MoveToNextContextPosition(LogicalDirection.Forward)) ; } // move to the first non-character symbol, but not pass beyond the limit private static void MoveToFirstNonCharacterSymbol( TextPointer navigator, // navigator to move TextPointer stopHint // don't move further if we already pass beyond this point ) { while (navigator.GetPointerContext(LogicalDirection.Forward) == TextPointerContext.Text && navigator.CompareTo(stopHint) < 0 && navigator.MoveToNextContextPosition(LogicalDirection.Forward)) ; } } /// /// result from TextEffectSetter which contains the TextEffect created and the DependencyObject /// to which the TextEffect should be set. /// public class TextEffectTarget { private DependencyObject _element; private TextEffect _effect; internal TextEffectTarget( DependencyObject element, TextEffect effect ) { if (element == null) throw new ArgumentNullException("element"); if (effect == null) throw new ArgumentNullException("effect"); _element = element; _effect = effect; } ////// The DependencyObject that the TextEffect is targetting. /// public DependencyObject Element { get { return _element; } } ////// The TextEffect /// public TextEffect TextEffect { get { return _effect; } } ////// Enable the TextEffect on the target. If the texteffect is /// already enabled, this will be a no-op. /// public void Enable() { TextEffectCollection textEffects = DynamicPropertyReader.GetTextEffects(_element); if (textEffects == null) { textEffects = new TextEffectCollection(); // use it as reference to avoid creating a copy (Freezable pattern) _element.SetValue(TextElement.TextEffectsProperty, textEffects); } // check whether this instance is already enabled for (int i = 0; i < textEffects.Count; i++) { if (textEffects[i] == _effect) return; // no-op } // use this as reference. textEffects.Add(_effect); } ////// Disable TextEffect on the target. If the texteffect is /// already disabled, this will be a no-op. /// public void Disable() { TextEffectCollection textEffects = DynamicPropertyReader.GetTextEffects(_element); if (textEffects != null) { for (int i = 0; i < textEffects.Count; i++) { if (textEffects[i] == _effect) { // remove the exact instance of the effect from the collection textEffects.RemoveAt(i); return; } } } } ////// Return whether the text effect is enabled on the target element /// public bool IsEnabled { get { TextEffectCollection textEffects = DynamicPropertyReader.GetTextEffects(_element); if (textEffects != null) { for (int i = 0; i < textEffects.Count; i++) { if (textEffects[i] == _effect) { return true; } } } return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
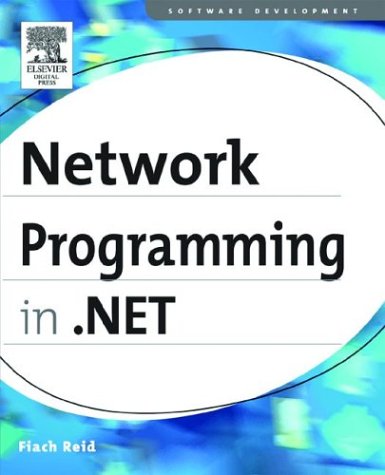
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Control.cs
- RootAction.cs
- AttributeAction.cs
- ListSourceHelper.cs
- TypedTableBase.cs
- OdbcEnvironmentHandle.cs
- Bind.cs
- CompilerErrorCollection.cs
- MobileControlPersister.cs
- EventProvider.cs
- InlineUIContainer.cs
- HttpWebRequest.cs
- ContentType.cs
- SmtpFailedRecipientException.cs
- AsymmetricSignatureFormatter.cs
- TreeNodeMouseHoverEvent.cs
- RTLAwareMessageBox.cs
- DependencyObjectCodeDomSerializer.cs
- QuerySelectOp.cs
- TagPrefixInfo.cs
- TextRenderer.cs
- NetworkInformationPermission.cs
- BamlTreeNode.cs
- WebPageTraceListener.cs
- PropertyPathWorker.cs
- HwndSource.cs
- ManipulationStartingEventArgs.cs
- NodeFunctions.cs
- SQLResource.cs
- NativeMethods.cs
- SqlFormatter.cs
- NativeWrapper.cs
- Rethrow.cs
- WebPartConnectionCollection.cs
- StateElementCollection.cs
- WorkflowTransactionOptions.cs
- WhitespaceRuleReader.cs
- hresults.cs
- _BaseOverlappedAsyncResult.cs
- DataDesignUtil.cs
- MergeFilterQuery.cs
- DataGridViewTopLeftHeaderCell.cs
- PropertyEntry.cs
- StrongNameMembershipCondition.cs
- CharConverter.cs
- EntityDataSourceUtil.cs
- EndpointDiscoveryMetadata.cs
- FaultPropagationQuery.cs
- PanelStyle.cs
- BitmapImage.cs
- TextEditorCharacters.cs
- IndentedWriter.cs
- CapiSafeHandles.cs
- MetadataFile.cs
- XmlSchemaType.cs
- COM2Enum.cs
- StylusPointProperty.cs
- RC2CryptoServiceProvider.cs
- StylusDevice.cs
- Keywords.cs
- mediaeventargs.cs
- ValidationError.cs
- UidManager.cs
- TextEffectCollection.cs
- GenericTextProperties.cs
- StylusEventArgs.cs
- DataRecordInternal.cs
- DbParameterCollectionHelper.cs
- FontWeights.cs
- WebBrowserPermission.cs
- TableCellCollection.cs
- SqlConnection.cs
- XmlSchemaNotation.cs
- UserControlCodeDomTreeGenerator.cs
- DrawingState.cs
- OdbcConnectionStringbuilder.cs
- XamlSerializerUtil.cs
- EntityDataReader.cs
- DynamicActionMessageFilter.cs
- CheckBoxFlatAdapter.cs
- Assembly.cs
- AuthenticationServiceManager.cs
- PieceNameHelper.cs
- CodeSubDirectoriesCollection.cs
- ClientEventManager.cs
- SizeConverter.cs
- AutoGeneratedField.cs
- RemotingAttributes.cs
- ToolStripItemCollection.cs
- ConstructorExpr.cs
- AssemblyAttributesGoHere.cs
- BrowserTree.cs
- HtmlCalendarAdapter.cs
- HttpRuntime.cs
- IProducerConsumerCollection.cs
- WindowsBrush.cs
- WindowsComboBox.cs
- ConsoleCancelEventArgs.cs
- RuleSetDialog.Designer.cs
- WindowsRegion.cs