Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / AutoGeneratedField.cs / 3 / AutoGeneratedField.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [EditorBrowsable(EditorBrowsableState.Never)] public sealed class AutoGeneratedField : BoundField { private bool _suppressPropertyThrows = false; private bool _useCheckBox; private bool _useCheckBoxValid; ///Creates a field bounded to a data field in a ///. /// public AutoGeneratedField(string dataField) { DataField = dataField; } ///Initializes a new instance of a ///class. /// public override string DataFormatString { get { return base.DataFormatString; } set { if (!_suppressPropertyThrows) { throw new NotSupportedException(); } } } ///Gets or sets the display format of data in this /// field. ////// public Type DataType { get { object o = ViewState["DataType"]; if (o != null) { return (Type)o; } return typeof(string); } set { ViewState["DataType"] = value; } } ///Gets or sets the type of data being displayed by the field. ////// public override bool InsertVisible { get { return base.InsertVisible; } set { if (!_suppressPropertyThrows) { throw new NotSupportedException(); } } } ///Gets or sets whether the field is visible in Insert mode. Turn off for auto-gen'd db fields ////// public override bool ConvertEmptyStringToNull { get { return base.ConvertEmptyStringToNull; } set { if (!_suppressPropertyThrows) { throw new NotSupportedException(); } } } ///Gets or sets the property that determines whether the BoundField treats empty string as /// null when the field values are extracted. ////// private bool UseCheckBox { get { if (!_useCheckBoxValid) { _useCheckBox = (DataType == typeof(bool) || DataType == typeof(bool?)); _useCheckBoxValid = true; } return _useCheckBox; } } protected override void CopyProperties(DataControlField newField) { ((AutoGeneratedField)newField).DataType = DataType; _suppressPropertyThrows = true; ((AutoGeneratedField)newField)._suppressPropertyThrows = true; base.CopyProperties(newField); _suppressPropertyThrows = false; ((AutoGeneratedField)newField)._suppressPropertyThrows = false; } protected override DataControlField CreateField() { return new AutoGeneratedField(DataField); } ///Determines whether the field should display a text box. ////// Returns a value to be used for design-time rendering /// protected override object GetDesignTimeValue() { if (UseCheckBox) { return true; } return base.GetDesignTimeValue(); } ////// Extracts the value of the databound cell and inserts the value into the given dictionary /// public override void ExtractValuesFromCell(IOrderedDictionary dictionary, DataControlFieldCell cell, DataControlRowState rowState, bool includeReadOnly) { if (UseCheckBox) { Control childControl = null; string dataField = DataField; object value = null; if (cell.Controls.Count > 0) { childControl = cell.Controls[0]; CheckBox checkBox = childControl as CheckBox; if (checkBox != null) { if (includeReadOnly || checkBox.Enabled) { value = checkBox.Checked; } } } if (value != null) { if (dictionary.Contains(dataField)) { dictionary[dataField] = value; } else { dictionary.Add(dataField, value); } } } else { base.ExtractValuesFromCell(dictionary, cell, rowState, includeReadOnly); } } protected override void InitializeDataCell(DataControlFieldCell cell, DataControlRowState rowState) { if (UseCheckBox) { CheckBox childControl = null; CheckBox boundControl = null; if (((rowState & DataControlRowState.Edit) != 0 && ReadOnly == false) || (rowState & DataControlRowState.Insert) != 0) { CheckBox editor = new CheckBox(); childControl = editor; if (DataField.Length != 0 && (rowState & DataControlRowState.Edit) != 0) { boundControl = editor; } } else if (DataField.Length != 0) { CheckBox editor = new CheckBox(); editor.Enabled = false; childControl = editor; boundControl = editor; } if (childControl != null) { childControl.ToolTip = HeaderText; cell.Controls.Add(childControl); } if (boundControl != null) { boundControl.DataBinding += new EventHandler(this.OnDataBindField); } } else { base.InitializeDataCell(cell, rowState); } } ////// protected override void OnDataBindField(object sender, EventArgs e) { if (UseCheckBox) { Control boundControl = (Control)sender; Control controlContainer = boundControl.NamingContainer; object data = GetValue(controlContainer); if (!(boundControl is CheckBox)) { throw new HttpException(SR.GetString(SR.CheckBoxField_WrongControlType, DataField)); } if (DataBinder.IsNull(data)) { ((CheckBox)boundControl).Checked = false; } else { if (data is Boolean) { ((CheckBox)boundControl).Checked = (Boolean)data; } else { try { ((CheckBox)boundControl).Checked = Boolean.Parse(data.ToString()); } catch (FormatException fe) { throw new HttpException(SR.GetString(SR.CheckBoxField_CouldntParseAsBoolean, DataField), fe); } } } } else { base.OnDataBindField(sender, e); } } ////// public override void ValidateSupportsCallback() { } } }Override with an empty body if the field's controls all support callback. /// Otherwise, override and throw a useful error message about why the field can't support callbacks. ///
Link Menu
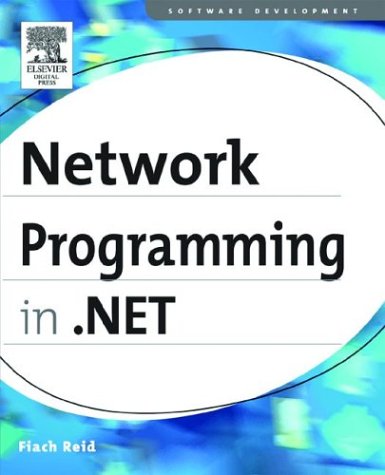
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventMappingSettings.cs
- DurationConverter.cs
- CollectionConverter.cs
- KeyedPriorityQueue.cs
- WindowCollection.cs
- HtmlElementCollection.cs
- WorkflowQueueInfo.cs
- ResponseBodyWriter.cs
- WebBrowserContainer.cs
- BoundField.cs
- CodeSubDirectoriesCollection.cs
- ShaderEffect.cs
- EmbeddedObject.cs
- ExternalException.cs
- WebPartConnectionsConnectVerb.cs
- LinqDataSourceContextEventArgs.cs
- InternalTypeHelper.cs
- BasePropertyDescriptor.cs
- Propagator.Evaluator.cs
- SQLDateTime.cs
- ActionItem.cs
- PiiTraceSource.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- CompiledRegexRunner.cs
- DesignerActionKeyboardBehavior.cs
- FilterEventArgs.cs
- UrlPath.cs
- BamlBinaryReader.cs
- AlphabeticalEnumConverter.cs
- ExitEventArgs.cs
- ScrollProviderWrapper.cs
- CommandBinding.cs
- WindowsBrush.cs
- XmlElementCollection.cs
- PermissionListSet.cs
- ResourceWriter.cs
- SqlCharStream.cs
- PersonalizableAttribute.cs
- COSERVERINFO.cs
- Ticks.cs
- Transactions.cs
- LinqDataSourceStatusEventArgs.cs
- Size3D.cs
- TripleDESCryptoServiceProvider.cs
- SqlTriggerAttribute.cs
- HostExecutionContextManager.cs
- CollectionExtensions.cs
- IIS7ConfigurationLoader.cs
- MetadataArtifactLoader.cs
- XmlNotation.cs
- _OverlappedAsyncResult.cs
- FixedPageStructure.cs
- Atom10FormatterFactory.cs
- BoundPropertyEntry.cs
- BindingList.cs
- MembershipValidatePasswordEventArgs.cs
- DataGridViewCellStyle.cs
- AnimationStorage.cs
- HttpValueCollection.cs
- MailWebEventProvider.cs
- DrawItemEvent.cs
- RtfToken.cs
- XmlTextAttribute.cs
- DefaultValueTypeConverter.cs
- HandlerMappingMemo.cs
- HtmlInputReset.cs
- FrameworkTextComposition.cs
- LoginName.cs
- unitconverter.cs
- Empty.cs
- XsltException.cs
- WebPartCloseVerb.cs
- MeasureItemEvent.cs
- ArgIterator.cs
- FindCriteriaApril2005.cs
- IndicShape.cs
- Propagator.JoinPropagator.cs
- Parameter.cs
- InvalidEnumArgumentException.cs
- PathSegmentCollection.cs
- XhtmlStyleClass.cs
- TcpHostedTransportConfiguration.cs
- DataServiceCollectionOfT.cs
- EncryptedXml.cs
- ReverseQueryOperator.cs
- cryptoapiTransform.cs
- CloudCollection.cs
- EntityCollection.cs
- ObjectListTitleAttribute.cs
- ConfigurationPropertyAttribute.cs
- NegatedCellConstant.cs
- OleStrCAMarshaler.cs
- SiteOfOriginPart.cs
- TransformCollection.cs
- ParameterRefs.cs
- ThicknessConverter.cs
- InputLanguageProfileNotifySink.cs
- XmlNamedNodeMap.cs
- UnsafeNetInfoNativeMethods.cs
- BasicCellRelation.cs