Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / System.Runtime.DurableInstancing / System / Runtime / Ticks.cs / 1305376 / Ticks.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.Runtime { using System.Security; using System.Runtime.Interop; static class Ticks { public static long Now { [Fx.Tag.SecurityNote(Miscellaneous = "Why isn't the SuppressUnmanagedCodeSecurity attribute working in this case?")] [SecuritySafeCritical] get { long time; #pragma warning disable 1634 #pragma warning suppress 56523 // function has no error return value #pragma warning restore 1634 UnsafeNativeMethods.GetSystemTimeAsFileTime(out time); return time; } } public static long FromMilliseconds(int milliseconds) { return checked((long)milliseconds * TimeSpan.TicksPerMillisecond); } public static int ToMilliseconds(long ticks) { return checked((int)(ticks / TimeSpan.TicksPerMillisecond)); } public static long FromTimeSpan(TimeSpan duration) { return duration.Ticks; } public static TimeSpan ToTimeSpan(long ticks) { return new TimeSpan(ticks); } public static long Add(long firstTicks, long secondTicks) { if (firstTicks == long.MaxValue || firstTicks == long.MinValue) { return firstTicks; } if (secondTicks == long.MaxValue || secondTicks == long.MinValue) { return secondTicks; } if (firstTicks >= 0 && long.MaxValue - firstTicks <= secondTicks) { return long.MaxValue - 1; } if (firstTicks <= 0 && long.MinValue - firstTicks >= secondTicks) { return long.MinValue + 1; } return checked(firstTicks + secondTicks); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
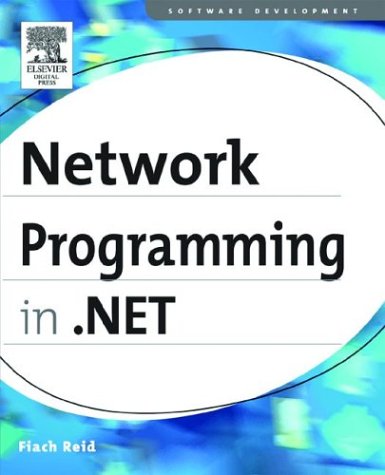
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ILGenerator.cs
- RegisteredHiddenField.cs
- xamlnodes.cs
- SimpleMailWebEventProvider.cs
- HttpInputStream.cs
- HttpContext.cs
- AsymmetricSignatureDeformatter.cs
- ListViewGroupItemCollection.cs
- SurrogateEncoder.cs
- IPEndPointCollection.cs
- DeviceContext.cs
- MenuItemCollectionEditorDialog.cs
- WeakReference.cs
- InvalidFilterCriteriaException.cs
- KerberosSecurityTokenAuthenticator.cs
- CustomErrorsSection.cs
- RowTypeElement.cs
- storepermission.cs
- ExtentCqlBlock.cs
- AlternateViewCollection.cs
- NamedElement.cs
- DataRowComparer.cs
- Token.cs
- unitconverter.cs
- EntityCommandDefinition.cs
- HandleScope.cs
- TableParaClient.cs
- SecurityMode.cs
- NetworkAddressChange.cs
- NativeMethods.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- ProtocolElementCollection.cs
- DirectoryRootQuery.cs
- EncodingTable.cs
- InboundActivityHelper.cs
- InheritanceAttribute.cs
- WebOperationContext.cs
- SqlParameterizer.cs
- TextContainer.cs
- QueryResultOp.cs
- GridViewRowCollection.cs
- FilteredDataSetHelper.cs
- Encoding.cs
- AudioFormatConverter.cs
- EventRecordWrittenEventArgs.cs
- IndependentAnimationStorage.cs
- UriSection.cs
- MultiSelectRootGridEntry.cs
- ArcSegment.cs
- PrivateFontCollection.cs
- ProxyWebPartConnectionCollection.cs
- DragDrop.cs
- sqlnorm.cs
- FormViewUpdatedEventArgs.cs
- ResourceProviderFactory.cs
- HttpListenerResponse.cs
- BooleanExpr.cs
- CacheOutputQuery.cs
- Pointer.cs
- InputScopeConverter.cs
- X509Utils.cs
- SafeCertificateContext.cs
- DesignerVerb.cs
- DataSysAttribute.cs
- XmlNodeChangedEventManager.cs
- DataGridViewColumnDividerDoubleClickEventArgs.cs
- PerfService.cs
- SeekStoryboard.cs
- ZipIOExtraField.cs
- ArgumentOutOfRangeException.cs
- DbConnectionFactory.cs
- TaiwanCalendar.cs
- ProgramPublisher.cs
- Color.cs
- CodeTypeMemberCollection.cs
- ObjectQueryState.cs
- ButtonColumn.cs
- BinaryCommonClasses.cs
- DynamicObject.cs
- PartialCachingControl.cs
- XamlReader.cs
- ReflectionTypeLoadException.cs
- TriggerBase.cs
- DrawingImage.cs
- XmlValidatingReaderImpl.cs
- GenericWebPart.cs
- WebPartAuthorizationEventArgs.cs
- ErrorWrapper.cs
- CacheDependency.cs
- DataRow.cs
- ClientFormsAuthenticationCredentials.cs
- LocalizationParserHooks.cs
- RelationshipSet.cs
- SerialStream.cs
- UnaryNode.cs
- DataGridViewCellLinkedList.cs
- PersonalizationStateQuery.cs
- ListDesigner.cs
- DataTablePropertyDescriptor.cs
- DefaultValueTypeConverter.cs