Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / ExtentCqlBlock.cs / 1305376 / ExtentCqlBlock.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Text; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.Structures; using System.Data.Common.Utils; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class that corresponds to the leaf CQL Blocks in the CqlBlock tree internal class ExtentCqlBlock : CqlBlock { private static readonly ListEmptyChildren = new List (); #region Constructors // effects: Creates an Extent CqlBlock corresponding to // "extent" (the FROM part). SELECT is given by slots, WHERE by // whereClause and AS by blockAliasNum internal ExtentCqlBlock(EntitySetBase extent, CellQuery.SelectDistinct selectDistinct, SlotInfo[] slots, BoolExpression whereClause, CqlIdentifiers identifiers, int blockAliasNum) : base(slots, EmptyChildren, whereClause, identifiers, blockAliasNum) { m_extent = extent; m_nodeTableAlias = identifiers.GetBlockAlias(); m_selectDistinct = selectDistinct; } #endregion #region Fields private EntitySetBase m_extent; // The extent for which we have this block private string m_nodeTableAlias; CellQuery.SelectDistinct m_selectDistinct; #endregion #region Methods // effects: See CqlBlock.AsCql internal override StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel) { // The SELECT part GenerateProjectedtList(builder, indentLevel, m_nodeTableAlias, m_selectDistinct, false); // Get the FROM part builder.Append("FROM "); // Get the extent object in C-Space (if m_extent is an S space object) CqlWriter.AppendEscapedQualifiedName(builder, m_extent.EntityContainer.Name, m_extent.Name); builder.Append(" AS ") .Append(m_nodeTableAlias); // Get the WHERE part only when the expression is not simply TRUE if (false == BoolExpression.EqualityComparer.Equals(WhereClause, BoolExpression.True)) { StringUtil.IndentNewLine(builder, indentLevel); builder.Append("WHERE "); WhereClause.AsCql(builder, m_nodeTableAlias); } return builder; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
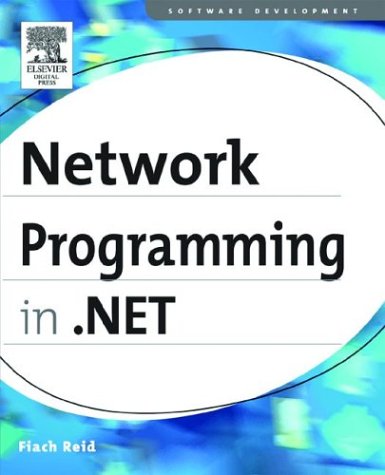
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReachUIElementCollectionSerializer.cs
- BrushValueSerializer.cs
- StrokeRenderer.cs
- DesignOnlyAttribute.cs
- CodeMethodInvokeExpression.cs
- RuleSet.cs
- ISessionStateStore.cs
- CounterCreationData.cs
- Matrix.cs
- ZoomPercentageConverter.cs
- ToolStripPanelRow.cs
- AppDomainProtocolHandler.cs
- RoutedCommand.cs
- TreeViewImageKeyConverter.cs
- LogExtent.cs
- NotSupportedException.cs
- MetadataProperty.cs
- RegexWorker.cs
- ApplicationInfo.cs
- HandledEventArgs.cs
- Single.cs
- ScrollViewer.cs
- GroupItemAutomationPeer.cs
- Utilities.cs
- MenuEventArgs.cs
- _CacheStreams.cs
- TrustLevel.cs
- TextHidden.cs
- BitmapEffectGeneralTransform.cs
- ChameleonKey.cs
- WindowPatternIdentifiers.cs
- InfoCardTrace.cs
- EntityContainerEntitySetDefiningQuery.cs
- TraceListeners.cs
- SqlDelegatedTransaction.cs
- ComAdminInterfaces.cs
- FileVersionInfo.cs
- AppSecurityManager.cs
- FacetValueContainer.cs
- SelectionProcessor.cs
- ConnectionPoolRegistry.cs
- FixedSOMContainer.cs
- GenericTextProperties.cs
- DependencyPropertyDescriptor.cs
- DefaultTextStore.cs
- DataSourceCache.cs
- MainMenu.cs
- SoapRpcServiceAttribute.cs
- ClickablePoint.cs
- DataGridColumnCollection.cs
- InkSerializer.cs
- PermissionSetTriple.cs
- ZipPackagePart.cs
- StringReader.cs
- SmtpNtlmAuthenticationModule.cs
- WebPartAuthorizationEventArgs.cs
- ProfileSection.cs
- BinaryObjectWriter.cs
- BooleanStorage.cs
- HandlerFactoryWrapper.cs
- RemotingConfiguration.cs
- EventLogRecord.cs
- ItemChangedEventArgs.cs
- MultiSelector.cs
- ContentPlaceHolder.cs
- sqlser.cs
- ColorContextHelper.cs
- InvalidEnumArgumentException.cs
- ContentElementAutomationPeer.cs
- TypedRowHandler.cs
- Vertex.cs
- SettingsPropertyNotFoundException.cs
- QilCloneVisitor.cs
- NameValueSectionHandler.cs
- Attributes.cs
- StandardOleMarshalObject.cs
- DelegateOutArgument.cs
- InvalidateEvent.cs
- Path.cs
- SymmetricCryptoHandle.cs
- HttpDictionary.cs
- FormView.cs
- NameTable.cs
- ColorConverter.cs
- EmptyImpersonationContext.cs
- RegexParser.cs
- ThrowHelper.cs
- BinHexDecoder.cs
- HttpCacheVaryByContentEncodings.cs
- HttpModuleAction.cs
- MemberInfoSerializationHolder.cs
- PasswordTextNavigator.cs
- HasRunnableWorkflowEvent.cs
- SafeRightsManagementEnvironmentHandle.cs
- Attributes.cs
- AppDomain.cs
- HttpProfileGroupBase.cs
- TabPanel.cs
- StylusTip.cs
- ScrollItemPattern.cs