Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / ManipulationDeltaEventArgs.cs / 1305600 / ManipulationDeltaEventArgs.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; namespace System.Windows.Input { ////// Provides an update on an ocurring manipulation. /// public sealed class ManipulationDeltaEventArgs : InputEventArgs { ////// Instantiates a new instance of this class. /// internal ManipulationDeltaEventArgs( ManipulationDevice manipulationDevice, int timestamp, IInputElement manipulationContainer, Point origin, ManipulationDelta delta, ManipulationDelta cumulative, ManipulationVelocities velocities, bool isInertial) : base(manipulationDevice, timestamp) { if (delta == null) { throw new ArgumentNullException("delta"); } if (cumulative == null) { throw new ArgumentNullException("cumulative"); } if (velocities == null) { throw new ArgumentNullException("velocities"); } RoutedEvent = Manipulation.ManipulationDeltaEvent; ManipulationContainer = manipulationContainer; ManipulationOrigin = origin; DeltaManipulation = delta; CumulativeManipulation = cumulative; Velocities = velocities; IsInertial = isInertial; } ////// Invokes a handler of this event. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { if (genericHandler == null) { throw new ArgumentNullException("genericHandler"); } if (genericTarget == null) { throw new ArgumentNullException("genericTarget"); } if (RoutedEvent == Manipulation.ManipulationDeltaEvent) { ((EventHandler)genericHandler)(genericTarget, this); } else { base.InvokeEventHandler(genericHandler, genericTarget); } } /// /// Whether the event was generated due to inertia. /// public bool IsInertial { get; private set; } ////// Defines the coordinate space of the other properties. /// public IInputElement ManipulationContainer { get; private set; } ////// Returns the value of the origin. /// public Point ManipulationOrigin { get; private set; } ////// Returns the cumulative transformation associated with the manipulation. /// public ManipulationDelta CumulativeManipulation { get; private set; } ////// Returns the delta transformation associated with the manipulation. /// public ManipulationDelta DeltaManipulation { get; private set; } ////// Returns the current velocities associated with a manipulation. /// public ManipulationVelocities Velocities { get; private set; } ////// Allows a handler to specify that the manipulation has gone beyond certain boundaries. /// By default, this value will then be used to provide panning feedback on the window, but /// it can be change by handling the ManipulationBoundaryFeedback event. /// public void ReportBoundaryFeedback(ManipulationDelta unusedManipulation) { if (unusedManipulation == null) { throw new ArgumentNullException("unusedManipulation"); } UnusedManipulation = unusedManipulation; } ////// The value of the unused manipulation information in global coordinate space. /// internal ManipulationDelta UnusedManipulation { get; private set; } ////// Preempts further processing and completes the manipulation without any inertia. /// public void Complete() { RequestedComplete = true; RequestedInertia = false; RequestedCancel = false; } ////// Preempts further processing and completes the manipulation, allowing inertia to continue. /// public void StartInertia() { RequestedComplete = true; RequestedInertia = true; RequestedCancel = false; } ////// Method to cancel the Manipulation /// ///A bool indicating the success of Cancel public bool Cancel() { if (!IsInertial) { RequestedCancel = true; RequestedComplete = false; RequestedInertia = false; return true; } return false; } ////// A handler requested that the manipulation complete. /// internal bool RequestedComplete { get; private set; } ////// A handler requested that the manipulation complete with inertia. /// internal bool RequestedInertia { get; private set; } ////// A handler Requested to cancel the Manipulation /// internal bool RequestedCancel { get; private set; } ////// The Manipulators for this manipulation. /// public IEnumerableManipulators { get { if (_manipulators == null) { _manipulators = ((ManipulationDevice)Device).GetManipulatorsReadOnly(); } return _manipulators; } } private IEnumerable _manipulators; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
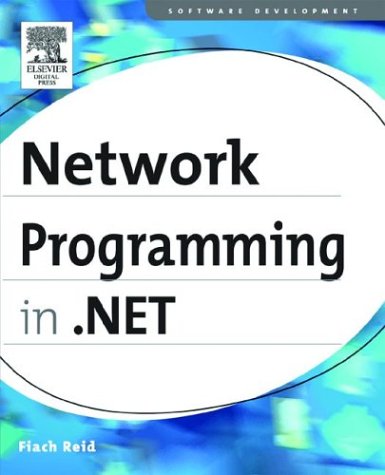
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HMACSHA384.cs
- DeobfuscatingStream.cs
- ModelPropertyCollectionImpl.cs
- EdmToObjectNamespaceMap.cs
- FixedStringLookup.cs
- OrderedHashRepartitionStream.cs
- SqlNode.cs
- InvalidProgramException.cs
- XmlChildNodes.cs
- Ipv6Element.cs
- SerializationStore.cs
- Image.cs
- UIElement3D.cs
- PersonalizationStateInfo.cs
- EarlyBoundInfo.cs
- DES.cs
- Condition.cs
- SafeNativeMethods.cs
- EDesignUtil.cs
- EntityModelSchemaGenerator.cs
- ScriptReferenceEventArgs.cs
- FrameworkName.cs
- PageThemeBuildProvider.cs
- DisplayInformation.cs
- SqlInfoMessageEvent.cs
- InputLangChangeRequestEvent.cs
- IdnElement.cs
- HttpContextServiceHost.cs
- OdbcConnectionFactory.cs
- MouseWheelEventArgs.cs
- X509RawDataKeyIdentifierClause.cs
- MenuAutomationPeer.cs
- TranslateTransform.cs
- Synchronization.cs
- StateInitialization.cs
- ObjectTag.cs
- OutputCacheProfileCollection.cs
- SmtpFailedRecipientsException.cs
- EventManager.cs
- ToolBarOverflowPanel.cs
- FormsAuthenticationEventArgs.cs
- SessionStateItemCollection.cs
- HandlerWithFactory.cs
- WindowsUpDown.cs
- XmlSiteMapProvider.cs
- SharedUtils.cs
- ObjectDataSourceEventArgs.cs
- BaseTemplateParser.cs
- UnionQueryOperator.cs
- CodeTypeReferenceExpression.cs
- TreeNodeMouseHoverEvent.cs
- DataGridViewComboBoxColumn.cs
- CalendarTable.cs
- StatusBarDrawItemEvent.cs
- SqlDataSourceCache.cs
- DataColumnChangeEvent.cs
- KnownIds.cs
- XmlSchemaComplexContentRestriction.cs
- StreamResourceInfo.cs
- StylusSystemGestureEventArgs.cs
- ImageCreator.cs
- QuestionEventArgs.cs
- ToolStripPanelDesigner.cs
- DebugView.cs
- Misc.cs
- EntityDataSourceSelectedEventArgs.cs
- URLMembershipCondition.cs
- ConfigViewGenerator.cs
- GeneratedContractType.cs
- MouseActionConverter.cs
- RawMouseInputReport.cs
- TextElementCollectionHelper.cs
- WebAdminConfigurationHelper.cs
- XmlProcessingInstruction.cs
- ReadContentAsBinaryHelper.cs
- DesigntimeLicenseContextSerializer.cs
- TextAdaptor.cs
- WindowsContainer.cs
- XmlWrappingReader.cs
- ApplicationActivator.cs
- VariableQuery.cs
- PlainXmlDeserializer.cs
- CodeBinaryOperatorExpression.cs
- NGCPageContentSerializerAsync.cs
- StrokeNode.cs
- SponsorHelper.cs
- ExpandCollapseProviderWrapper.cs
- Underline.cs
- MobileResource.cs
- _FixedSizeReader.cs
- HorizontalAlignConverter.cs
- IteratorFilter.cs
- HttpCapabilitiesSectionHandler.cs
- EdmFunction.cs
- LastQueryOperator.cs
- XmlWriterTraceListener.cs
- StrongNameIdentityPermission.cs
- PassportIdentity.cs
- LambdaCompiler.Logical.cs
- ProfileGroupSettings.cs