Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / PtsHost / MbpInfo.cs / 1 / MbpInfo.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: MbpInfo.cs // // Description: Provides paragraph level margin collapsing support. // //--------------------------------------------------------------------------- using System; using System.Windows; // DependencyObject using System.Windows.Documents; // Block using MS.Internal.Text; // TextDpi using System.Windows.Media; // Brush using MS.Internal.PtsHost.UnsafeNativeMethods; // Pts namespace MS.Internal.PtsHost { ////// Provides services for MBP handling. /// internal sealed class MbpInfo { ////// Get MbpInfo from DependencyObject. /// /// DependencyObject for which MBP properties are retrieved. internal static MbpInfo FromElement(DependencyObject o) { if (o is Block || o is AnchoredBlock || o is TableCell || o is ListItem) { MbpInfo mbp = new MbpInfo((TextElement)o); double lineHeight = DynamicPropertyReader.GetLineHeightValue(o); if (mbp.IsMarginAuto) { ResolveAutoMargin(mbp, o, lineHeight); } if (mbp.IsPaddingAuto) { ResolveAutoPadding(mbp, o, lineHeight); } return mbp; } return _empty; } ////// Mirrors margin /// internal void MirrorMargin() { ReverseFlowDirection(ref _margin); } ////// Mirrors border and padding /// internal void MirrorBP() { ReverseFlowDirection(ref _border); ReverseFlowDirection(ref _padding); } ////// Reverses flow direction for a given thickness /// private static void ReverseFlowDirection(ref Thickness thickness) { double temp = thickness.Left; thickness.Left = thickness.Right; thickness.Right = temp; } ////// Static constructor. /// static MbpInfo() { _empty = new MbpInfo(); } ////// Private constructor. /// private MbpInfo() { _margin = new Thickness(); _border = new Thickness(); _padding = new Thickness(); _borderBrush = new SolidColorBrush(); } ////// Constructor. /// /// Block for which MBP properties are retrieved. private MbpInfo(TextElement block) { _margin = (Thickness)block.GetValue(Block.MarginProperty); _border = (Thickness)block.GetValue(Block.BorderThicknessProperty); _padding = (Thickness)block.GetValue(Block.PaddingProperty); _borderBrush = (Brush)block.GetValue(Block.BorderBrushProperty); } ////// Resolve Auto values for Margin. /// private static void ResolveAutoMargin(MbpInfo mbp, DependencyObject o, double lineHeight) { Thickness defaultMargin; if (o is Paragraph) { DependencyObject parent = ((Paragraph)o).Parent; if (parent is ListItem || parent is TableCell || parent is AnchoredBlock) { defaultMargin = new Thickness(0); } else { defaultMargin = new Thickness(0, lineHeight, 0, lineHeight); } } else if (o is Table || o is List) { defaultMargin = new Thickness(0, lineHeight, 0, lineHeight); } else if (o is Figure || o is Floater) { defaultMargin = new Thickness(0.5 * lineHeight); } else { defaultMargin = new Thickness(0); } mbp.Margin = new Thickness( Double.IsNaN(mbp.Margin.Left) ? defaultMargin.Left : mbp.Margin.Left, Double.IsNaN(mbp.Margin.Top) ? defaultMargin.Top : mbp.Margin.Top, Double.IsNaN(mbp.Margin.Right) ? defaultMargin.Right : mbp.Margin.Right, Double.IsNaN(mbp.Margin.Bottom) ? defaultMargin.Bottom : mbp.Margin.Bottom); } ////// Resolve Auto values for Padding. /// private static void ResolveAutoPadding(MbpInfo mbp, DependencyObject o, double lineHeight) { Thickness defaultPadding; if (o is Figure || o is Floater) { defaultPadding = new Thickness(0.5 * lineHeight); } else if (o is List) { defaultPadding = ListMarkerSourceInfo.CalculatePadding((List)o, lineHeight); } else { defaultPadding = new Thickness(0); } mbp.Padding = new Thickness( Double.IsNaN(mbp.Padding.Left) ? defaultPadding.Left : mbp.Padding.Left, Double.IsNaN(mbp.Padding.Top) ? defaultPadding.Top : mbp.Padding.Top, Double.IsNaN(mbp.Padding.Right) ? defaultPadding.Right : mbp.Padding.Right, Double.IsNaN(mbp.Padding.Bottom) ? defaultPadding.Bottom : mbp.Padding.Bottom); } ////// Combined value of left Margin, Border and Padding. /// internal int MBPLeft { get { return TextDpi.ToTextDpi(_margin.Left) + TextDpi.ToTextDpi(_border.Left) + TextDpi.ToTextDpi(_padding.Left); } } ////// Combined value of right Margin, Border and Padding. /// internal int MBPRight { get { return TextDpi.ToTextDpi(_margin.Right) + TextDpi.ToTextDpi(_border.Right) + TextDpi.ToTextDpi(_padding.Right); } } ////// Combined value of top Margin, Border and Padding. /// internal int MBPTop { get { return TextDpi.ToTextDpi(_margin.Top) + TextDpi.ToTextDpi(_border.Top) + TextDpi.ToTextDpi(_padding.Top); } } ////// Combined value of top Margin, Border and Padding. /// internal int MBPBottom { get { return TextDpi.ToTextDpi(_margin.Bottom) + TextDpi.ToTextDpi(_border.Bottom) + TextDpi.ToTextDpi(_padding.Bottom); } } ////// Combined value of left Border and Padding. /// internal int BPLeft { get { return TextDpi.ToTextDpi(_border.Left) + TextDpi.ToTextDpi(_padding.Left); } } ////// Combined value of right Border and Padding. /// internal int BPRight { get { return TextDpi.ToTextDpi(_border.Right) + TextDpi.ToTextDpi(_padding.Right); } } ////// Combined value of top Border and Padding. /// internal int BPTop { get { return TextDpi.ToTextDpi(_border.Top) + TextDpi.ToTextDpi(_padding.Top); } } ////// Combined value of bottom Border and Padding. /// internal int BPBottom { get { return TextDpi.ToTextDpi(_border.Bottom) + TextDpi.ToTextDpi(_padding.Bottom); } } ////// Left Border /// internal int BorderLeft { get { return TextDpi.ToTextDpi(_border.Left); } } ////// Right Border /// internal int BorderRight { get { return TextDpi.ToTextDpi(_border.Right); } } ////// Top Border /// internal int BorderTop { get { return TextDpi.ToTextDpi(_border.Top); } } ////// Bottom Border /// internal int BorderBottom { get { return TextDpi.ToTextDpi(_border.Bottom); } } ////// Left margin. /// internal int MarginLeft { get { return TextDpi.ToTextDpi(_margin.Left); } } ////// Right margin. /// internal int MarginRight { get { return TextDpi.ToTextDpi(_margin.Right); } } ////// top margin. /// internal int MarginTop { get { return TextDpi.ToTextDpi(_margin.Top); } } ////// Bottom margin. /// internal int MarginBottom { get { return TextDpi.ToTextDpi(_margin.Bottom); } } ////// Margin thickness. /// internal Thickness Margin { get { return _margin; } set { _margin = value; } } ////// Border thickness. /// internal Thickness Border { get { return _border; } set { _border = value; } } internal Thickness Padding { get { return _padding; } set { _padding = value; } } ////// Border brush. /// internal Brush BorderBrush { get { return _borderBrush; } } ////// Whether any padding value is Auto. /// private bool IsPaddingAuto { get { return ( Double.IsNaN(_padding.Left) || Double.IsNaN(_padding.Right) || Double.IsNaN(_padding.Top) || Double.IsNaN(_padding.Bottom)); } } ////// Whether any margin value is Auto. /// private bool IsMarginAuto { get { return ( Double.IsNaN(_margin.Left) || Double.IsNaN(_margin.Right) || Double.IsNaN(_margin.Top) || Double.IsNaN(_margin.Bottom)); } } ////// Margin thickness. /// private Thickness _margin; ////// Border thickness. /// private Thickness _border; ////// Padding thickness. /// private Thickness _padding; ////// Border brush. /// private Brush _borderBrush; ////// Empty MBPInfo instance. /// private static MbpInfo _empty; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
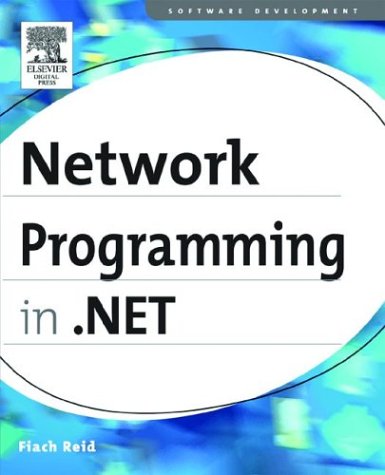
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Wizard.cs
- _ConnectOverlappedAsyncResult.cs
- ObjectDataSourceStatusEventArgs.cs
- StringResourceManager.cs
- WindowsTokenRoleProvider.cs
- QueryOutputWriter.cs
- CreateRefExpr.cs
- Point4D.cs
- WebExceptionStatus.cs
- FixedSOMContainer.cs
- HttpFileCollection.cs
- EllipticalNodeOperations.cs
- HelpEvent.cs
- XPathArrayIterator.cs
- TextTreeInsertElementUndoUnit.cs
- AnimatedTypeHelpers.cs
- RPIdentityRequirement.cs
- OdbcConnectionFactory.cs
- TreeNodeClickEventArgs.cs
- Size3D.cs
- RadioButton.cs
- CodeCompileUnit.cs
- TopClause.cs
- CommentAction.cs
- BamlReader.cs
- ServiceContractViewControl.Designer.cs
- RTTypeWrapper.cs
- figurelengthconverter.cs
- FontFaceLayoutInfo.cs
- SiteIdentityPermission.cs
- HandlerBase.cs
- WebServiceParameterData.cs
- ComponentResourceManager.cs
- InputReferenceExpression.cs
- IPeerNeighbor.cs
- ImageButton.cs
- PackageFilter.cs
- ClientTargetCollection.cs
- PaintEvent.cs
- PartitionerStatic.cs
- ParseNumbers.cs
- XmlnsDictionary.cs
- DataGridCell.cs
- KeyManager.cs
- CodeValidator.cs
- ContentTextAutomationPeer.cs
- MonitoringDescriptionAttribute.cs
- UriParserTemplates.cs
- ObjectKeyFrameCollection.cs
- PropertyGeneratedEventArgs.cs
- RuntimeConfig.cs
- DocumentPage.cs
- LabelLiteral.cs
- _HeaderInfoTable.cs
- DatagridviewDisplayedBandsData.cs
- DataGridViewCellMouseEventArgs.cs
- TextModifierScope.cs
- IssuedTokenClientBehaviorsElementCollection.cs
- SqlVersion.cs
- ComponentCodeDomSerializer.cs
- CompilerError.cs
- TraceInternal.cs
- FontConverter.cs
- ServiceHost.cs
- ViewCellRelation.cs
- EntityWrapper.cs
- SystemException.cs
- IImplicitResourceProvider.cs
- BindToObject.cs
- Model3DCollection.cs
- FontDifferentiator.cs
- ProfileSettings.cs
- GuidelineCollection.cs
- ThreadExceptionEvent.cs
- DesignColumn.cs
- Page.cs
- UnionCodeGroup.cs
- CodeCommentStatementCollection.cs
- TabControlAutomationPeer.cs
- HandlerFactoryCache.cs
- CheckPair.cs
- TreeViewImageIndexConverter.cs
- FaultDescription.cs
- ConfigurationException.cs
- ClientScriptItemCollection.cs
- handlecollector.cs
- wgx_sdk_version.cs
- Point3DAnimationBase.cs
- WindowsRichEdit.cs
- VSWCFServiceContractGenerator.cs
- ResourceReferenceExpression.cs
- LZCodec.cs
- Helper.cs
- AdornedElementPlaceholder.cs
- PolyLineSegment.cs
- xdrvalidator.cs
- ClientBuildManagerCallback.cs
- LeafCellTreeNode.cs
- VisualBrush.cs
- DragCompletedEventArgs.cs