Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / textformatting / TextModifierScope.cs / 1 / TextModifierScope.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: TextModifierScope.cs // // Contents: Text modification API // // Spec: http://avalon/text/DesignDocsAndSpecs/Text%20Formatting%20API.doc // // Created: 12-5-2004 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Media; namespace System.Windows.Media.TextFormatting { ////// Represents a single "frame" in the stack of text modifiers. The stack /// is represented not as an array, but as a linked structure in which each /// frame points to its parent. /// internal sealed class TextModifierScope { private TextModifierScope _parentScope; private TextModifier _modifier; private int _cp; ////// Constructs a new text modification state object. /// /// Parent scope, i.e., the previous top of the stack. /// Text modifier run fetched from the client. /// Text source character index of the run. internal TextModifierScope(TextModifierScope parentScope, TextModifier modifier, int cp) { _parentScope = parentScope; _modifier = modifier; _cp = cp; } ////// Next item in the stack of text modifiers. /// public TextModifierScope ParentScope { get { return _parentScope; } } ////// Text modifier run fetched from the client. /// public TextModifier TextModifier { get { return _modifier; } } ////// Character index of the text modifier run. /// public int TextSourceCharacterIndex { get { return _cp; } } ////// Modifies the specified text run properties by invoking the modifier at /// the current scope and all containing scopes. /// /// Properties to modify. ///Returns the text run properties after modification. internal TextRunProperties ModifyProperties(TextRunProperties properties) { for (TextModifierScope scope = this; scope != null; scope = scope._parentScope) { properties = scope._modifier.ModifyProperties(properties); } return properties; } ////// Performs a deep copy of the stack of TextModifierScope objects. /// ///Returns the top of the new stack. internal TextModifierScope CloneStack() { TextModifierScope top = new TextModifierScope(null, _modifier, _cp); TextModifierScope scope = top; for (TextModifierScope source = _parentScope; source != null; source = source._parentScope) { scope._parentScope = new TextModifierScope(null, source._modifier, source._cp); scope = scope._parentScope; } return top; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: TextModifierScope.cs // // Contents: Text modification API // // Spec: http://avalon/text/DesignDocsAndSpecs/Text%20Formatting%20API.doc // // Created: 12-5-2004 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Media; namespace System.Windows.Media.TextFormatting { ////// Represents a single "frame" in the stack of text modifiers. The stack /// is represented not as an array, but as a linked structure in which each /// frame points to its parent. /// internal sealed class TextModifierScope { private TextModifierScope _parentScope; private TextModifier _modifier; private int _cp; ////// Constructs a new text modification state object. /// /// Parent scope, i.e., the previous top of the stack. /// Text modifier run fetched from the client. /// Text source character index of the run. internal TextModifierScope(TextModifierScope parentScope, TextModifier modifier, int cp) { _parentScope = parentScope; _modifier = modifier; _cp = cp; } ////// Next item in the stack of text modifiers. /// public TextModifierScope ParentScope { get { return _parentScope; } } ////// Text modifier run fetched from the client. /// public TextModifier TextModifier { get { return _modifier; } } ////// Character index of the text modifier run. /// public int TextSourceCharacterIndex { get { return _cp; } } ////// Modifies the specified text run properties by invoking the modifier at /// the current scope and all containing scopes. /// /// Properties to modify. ///Returns the text run properties after modification. internal TextRunProperties ModifyProperties(TextRunProperties properties) { for (TextModifierScope scope = this; scope != null; scope = scope._parentScope) { properties = scope._modifier.ModifyProperties(properties); } return properties; } ////// Performs a deep copy of the stack of TextModifierScope objects. /// ///Returns the top of the new stack. internal TextModifierScope CloneStack() { TextModifierScope top = new TextModifierScope(null, _modifier, _cp); TextModifierScope scope = top; for (TextModifierScope source = _parentScope; source != null; source = source._parentScope) { scope._parentScope = new TextModifierScope(null, source._modifier, source._cp); scope = scope._parentScope; } return top; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
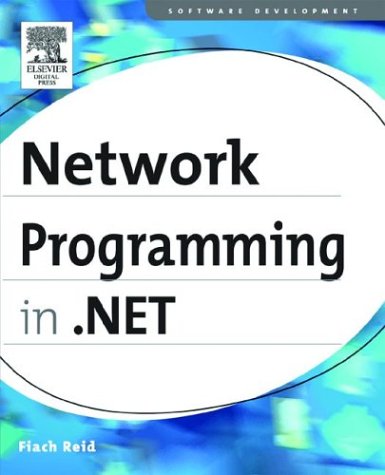
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseAddressPrefixFilterElementCollection.cs
- Peer.cs
- ViewStateException.cs
- SeekStoryboard.cs
- SingleKeyFrameCollection.cs
- SolidBrush.cs
- ConfigurationValues.cs
- OutputCacheSettingsSection.cs
- WebExceptionStatus.cs
- XmlSchemaAll.cs
- XmlQueryRuntime.cs
- Point3DAnimationUsingKeyFrames.cs
- HtmlHistory.cs
- GeometryCombineModeValidation.cs
- KeyValueInternalCollection.cs
- PackageStore.cs
- ParameterCollection.cs
- TextParagraphProperties.cs
- NonSerializedAttribute.cs
- TargetException.cs
- LinqDataSourceStatusEventArgs.cs
- UInt16Converter.cs
- ToolStripItem.cs
- DetailsViewRowCollection.cs
- MouseActionValueSerializer.cs
- XmlIlGenerator.cs
- MessageHeaderInfoTraceRecord.cs
- ControlCodeDomSerializer.cs
- XmlSchemaInferenceException.cs
- AutomationEventArgs.cs
- OdbcEnvironmentHandle.cs
- TypeValidationEventArgs.cs
- TableParaClient.cs
- IPAddress.cs
- SHA512Managed.cs
- CompositionDesigner.cs
- ResourcesChangeInfo.cs
- TdsRecordBufferSetter.cs
- XmlSchemaAnnotation.cs
- SecurityDocument.cs
- UrlMappingsSection.cs
- Filter.cs
- ByteConverter.cs
- SamlAuthorizationDecisionStatement.cs
- ToolStripRenderer.cs
- HtmlInputReset.cs
- XomlCompiler.cs
- ManipulationCompletedEventArgs.cs
- TextPatternIdentifiers.cs
- OleDbCommand.cs
- DetailsViewUpdatedEventArgs.cs
- DataGridViewComboBoxColumn.cs
- ImageSourceValueSerializer.cs
- SortKey.cs
- DynamicDocumentPaginator.cs
- BinaryUtilClasses.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- DataGridViewRowsRemovedEventArgs.cs
- TreeNodeCollection.cs
- TraceContext.cs
- Exceptions.cs
- InteropAutomationProvider.cs
- InternalConfigRoot.cs
- CustomServiceCredentials.cs
- InvokeMethodActivity.cs
- SocketAddress.cs
- CalendarTable.cs
- XamlReader.cs
- SubqueryRules.cs
- ConfigurationSectionHelper.cs
- EnumerableValidator.cs
- LinqDataSourceHelper.cs
- PropertyMap.cs
- DataBoundControlHelper.cs
- EnumConverter.cs
- NameValueCache.cs
- StreamResourceInfo.cs
- EqualityComparer.cs
- PropertyIDSet.cs
- NoneExcludedImageIndexConverter.cs
- SkipStoryboardToFill.cs
- WaitHandleCannotBeOpenedException.cs
- OutputCacheProfileCollection.cs
- JavaScriptSerializer.cs
- DynamicActionMessageFilter.cs
- DeviceContexts.cs
- CodeConditionStatement.cs
- DataBindingList.cs
- EncoderExceptionFallback.cs
- MappingException.cs
- XamlSerializerUtil.cs
- DesignerLoader.cs
- DataStreams.cs
- ValidationHelpers.cs
- WebPartDisplayMode.cs
- Stack.cs
- __FastResourceComparer.cs
- EditingCoordinator.cs
- CommandEventArgs.cs
- ListenerChannelContext.cs