Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Misc / GDI / DeviceContexts.cs / 1305376 / DeviceContexts.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #if WGCM_TEST_SUITE // Enable tracking when built for the test suites. #define TRACK_HDC #define GDI_FONT_CACHE_TRACK #endif #if [....]_NAMESPACE namespace System.Windows.Forms.Internal #elif DRAWING_NAMESPACE namespace System.Drawing.Internal #else namespace System.Experimental.Gdi #endif { using System; using System.Collections.Generic; using System.Internal; using System.Diagnostics; using System.Drawing; using System.Threading; using Microsoft.Win32; ////// Keeps a cache of some graphics primitives. /// Created to improve performance of TextRenderer.MeasureText methods that don't receive a WindowsGraphics. /// This class mantains a cache of MRU WindowsFont objects in the process. (See VSWhidbey#301492). /// #if [....]_PUBLIC_GRAPHICS_LIBRARY public #else internal #endif static class DeviceContexts { [ThreadStatic] private static ClientUtils.WeakRefCollection activeDeviceContexts; ////// WindowsGraphicsCacheManager needs to track DeviceContext /// objects so it can ask them if a font is in use before they /// it's deleted. internal static void AddDeviceContext(DeviceContext dc) { if (activeDeviceContexts == null) { activeDeviceContexts = new ClientUtils.WeakRefCollection(); activeDeviceContexts.RefCheckThreshold = 20; // See DevDiv#82664 (the repro app attached to this bug was used to determine this number). } if (!activeDeviceContexts.Contains(dc)) { dc.Disposing += new EventHandler(OnDcDisposing); activeDeviceContexts.Add(dc); } } private static void OnDcDisposing(object sender, EventArgs e) { DeviceContext dc = sender as DeviceContext; if (dc != null) { dc.Disposing -= new EventHandler(OnDcDisposing); RemoveDeviceContext(dc); } } internal static void RemoveDeviceContext(DeviceContext dc) { if (activeDeviceContexts == null) { return; } activeDeviceContexts.RemoveByHashCode(dc); } #if !DRAWING_NAMESPACE internal static bool IsFontInUse(WindowsFont wf) { if (wf == null) { return false; } for (int i = 0; i < activeDeviceContexts.Count; i++) { DeviceContext dc = activeDeviceContexts[i] as DeviceContext; if (dc != null && (dc.ActiveFont == wf || dc.IsFontOnContextStack(wf))) { return true; } } return false; } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
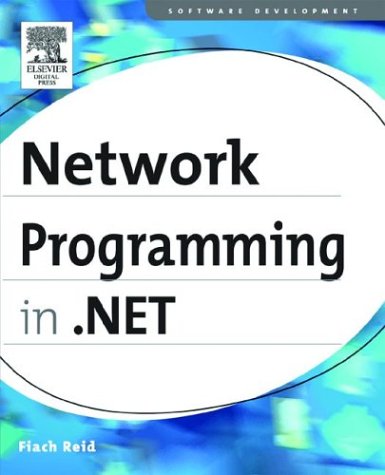
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataRowChangeEvent.cs
- RecognitionResult.cs
- RequestQueue.cs
- PageAsyncTaskManager.cs
- CreateRefExpr.cs
- ProtocolElement.cs
- DrawingContext.cs
- HtmlInputControl.cs
- EventWaitHandleSecurity.cs
- XmlUrlResolver.cs
- HybridDictionary.cs
- ProjectedSlot.cs
- SizeLimitedCache.cs
- XamlSerializationHelper.cs
- RegexCompiler.cs
- UpDownBase.cs
- DateTimeFormat.cs
- SqlInternalConnectionTds.cs
- Win32Native.cs
- AmbiguousMatchException.cs
- EditorPartCollection.cs
- Attributes.cs
- TextSelectionHelper.cs
- DataObjectPastingEventArgs.cs
- PersistenceIOParticipant.cs
- DataObject.cs
- ScaleTransform.cs
- ProjectionCamera.cs
- OracleMonthSpan.cs
- StyleXamlTreeBuilder.cs
- AmbientValueAttribute.cs
- MenuItemCollection.cs
- DBDataPermission.cs
- ExpressionConverter.cs
- MemberRelationshipService.cs
- DetailsViewPageEventArgs.cs
- ButtonColumn.cs
- LogConverter.cs
- StackOverflowException.cs
- DbInsertCommandTree.cs
- StagingAreaInputItem.cs
- SubMenuStyle.cs
- FormsAuthenticationConfiguration.cs
- UpdatePanelControlTrigger.cs
- FaultBookmark.cs
- EraserBehavior.cs
- SynchronizationContextHelper.cs
- XamlParser.cs
- XpsFilter.cs
- IApplicationTrustManager.cs
- ServiceContractAttribute.cs
- DesignerToolboxInfo.cs
- XmlTextReader.cs
- isolationinterop.cs
- CommonGetThemePartSize.cs
- CodeCatchClauseCollection.cs
- SqlException.cs
- InputLanguage.cs
- EventMappingSettingsCollection.cs
- Tag.cs
- Internal.cs
- UserControlCodeDomTreeGenerator.cs
- PeerCollaborationPermission.cs
- UnionExpr.cs
- HandlerFactoryCache.cs
- OAVariantLib.cs
- XsltCompileContext.cs
- RectAnimationUsingKeyFrames.cs
- DesignerTransaction.cs
- DrawListViewColumnHeaderEventArgs.cs
- GCHandleCookieTable.cs
- DeferredReference.cs
- DrawingGroup.cs
- HwndKeyboardInputProvider.cs
- DesignerImageAdapter.cs
- ImageIndexConverter.cs
- BidPrivateBase.cs
- DetailsViewUpdatedEventArgs.cs
- ScriptResourceHandler.cs
- DirectionalLight.cs
- ClaimSet.cs
- MDIControlStrip.cs
- SelectedDatesCollection.cs
- ComponentEvent.cs
- WeakEventManager.cs
- RealizationDrawingContextWalker.cs
- Codec.cs
- EventManager.cs
- DoubleLinkList.cs
- ValidationHelper.cs
- DbSource.cs
- GraphicsPathIterator.cs
- FolderBrowserDialog.cs
- SqlDataSourceCache.cs
- RolePrincipal.cs
- InfocardChannelParameter.cs
- MemberRelationshipService.cs
- Authorization.cs
- FontResourceCache.cs
- AspProxy.cs