Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / TreeNodeCollection.cs / 1 / TreeNodeCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.InteropServices; using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing.Design; using System.Globalization; ////// /// [ Editor("System.Windows.Forms.Design.TreeNodeCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] public class TreeNodeCollection : IList { private TreeNode owner; /// A caching mechanism for key accessor /// We use an index here rather than control so that we don't have lifetime /// issues by holding on to extra references. private int lastAccessedIndex = -1; //this index is used to optimize performance of AddRange //items are added from last to first after this index //(to work around TV_INSertItem comctl32 perf issue with consecutive adds in the end of the list) private int fixedIndex = -1; internal TreeNodeCollection(TreeNode owner) { this.owner = owner; } ///[To be supplied.] ////// internal int FixedIndex { get { return fixedIndex; } set { fixedIndex = value; } } /// /// /// public virtual TreeNode this[int index] { get { if (index < 0 || index >= owner.childCount) { throw new ArgumentOutOfRangeException("index"); } return owner.children[index]; } set { if (index < 0 || index >= owner.childCount) throw new ArgumentOutOfRangeException("index", SR.GetString(SR.InvalidArgument, "index", (index).ToString(CultureInfo.CurrentCulture))); value.parent = owner; value.index = index; owner.children[index] = value; value.Realize(false); } } ///[To be supplied.] ////// object IList.this[int index] { get { return this[index]; } set { if (value is TreeNode) { this[index] = (TreeNode)value; } else { throw new ArgumentException(SR.GetString(SR.TreeNodeCollectionBadTreeNode), "value"); } } } /// /// /// public virtual TreeNode this[string key] { get { // We do not support null and empty string as valid keys. if (string.IsNullOrEmpty(key)){ return null; } // Search for the key in our collection int index = IndexOfKey(key); if (IsValidIndex(index)) { return this[index]; } else { return null; } } } ///Retrieves the child control with the specified key. ////// /// // VSWhidbey 152051: Make this property available to Intellisense. (Removed the EditorBrowsable attribute.) [Browsable(false)] public int Count { get { return owner.childCount; } } ///[To be supplied.] ////// object ICollection.SyncRoot { get { return this; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// bool IList.IsFixedSize { get { return false; } } /// /// /// public bool IsReadOnly { get { return false; } } ///[To be supplied.] ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string text) { TreeNode tn = new TreeNode(text); Add(tn); return tn; } // <-- NEW ADD OVERLOADS IN WHIDBEY ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text) { TreeNode tn = new TreeNode(text); tn.Name = key; Add(tn); return tn; } ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text, int imageIndex) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageIndex = imageIndex; Add(tn); return tn; } ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text, string imageKey) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageKey = imageKey; Add(tn); return tn; } ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text, int imageIndex, int selectedImageIndex) { TreeNode tn = new TreeNode(text, imageIndex, selectedImageIndex); tn.Name = key; Add(tn); return tn; } ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text, string imageKey, string selectedImageKey) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageKey = imageKey; tn.SelectedImageKey = selectedImageKey; Add(tn); return tn; } // END - NEW ADD OVERLOADS IN WHIDBEY --> ////// /// public virtual void AddRange(TreeNode[] nodes) { if (nodes == null) { throw new ArgumentNullException("nodes"); } if (nodes.Length == 0) return; TreeView tv = owner.TreeView; if (tv != null && nodes.Length > TreeNode.MAX_TREENODES_OPS) { tv.BeginUpdate(); } owner.Nodes.FixedIndex = owner.childCount; owner.EnsureCapacity(nodes.Length); for (int i = nodes.Length-1 ; i >= 0; i--) { AddInternal(nodes[i],i); } owner.Nodes.FixedIndex = -1; if (tv != null && nodes.Length > TreeNode.MAX_TREENODES_OPS) { tv.EndUpdate(); } } ///[To be supplied.] ////// /// public TreeNode[] Find (string key, bool searchAllChildren) { ArrayList foundNodes = FindInternal(key, searchAllChildren, this, new ArrayList()); // TreeNode[] stronglyTypedFoundNodes = new TreeNode[foundNodes.Count]; foundNodes.CopyTo(stronglyTypedFoundNodes, 0); return stronglyTypedFoundNodes; } private ArrayList FindInternal(string key, bool searchAllChildren, TreeNodeCollection treeNodeCollectionToLookIn, ArrayList foundTreeNodes) { if ((treeNodeCollectionToLookIn == null) || (foundTreeNodes == null)) { return null; } // Perform breadth first search - as it's likely people will want tree nodes belonging // to the same parent close to each other. for (int i = 0; i < treeNodeCollectionToLookIn.Count; i++) { if (treeNodeCollectionToLookIn[i] == null){ continue; } if (WindowsFormsUtils.SafeCompareStrings(treeNodeCollectionToLookIn[i].Name, key, /* ignoreCase = */ true)) { foundTreeNodes.Add(treeNodeCollectionToLookIn[i]); } } // Optional recurive search for controls in child collections. if (searchAllChildren){ for (int i = 0; i < treeNodeCollectionToLookIn.Count; i++) { if (treeNodeCollectionToLookIn[i] == null){ continue; } if ((treeNodeCollectionToLookIn[i].Nodes != null) && treeNodeCollectionToLookIn[i].Nodes.Count > 0){ // if it has a valid child collecion, append those results to our collection foundTreeNodes = FindInternal(key, searchAllChildren, treeNodeCollectionToLookIn[i].Nodes, foundTreeNodes); } } } return foundTreeNodes; } ///[To be supplied.] ////// /// Adds a new child node to this node. Child node is positioned after siblings. /// public virtual int Add(TreeNode node) { return AddInternal(node, 0); } private int AddInternal(TreeNode node, int delta) { if (node == null) { throw new ArgumentNullException("node"); } if (node.handle != IntPtr.Zero) throw new ArgumentException(SR.GetString(SR.OnlyOneControl, node.Text), "node"); // If the TreeView is sorted, index is ignored TreeView tv = owner.TreeView; if (tv != null && tv.Sorted) { return owner.AddSorted(node); } node.parent = owner; int fixedIndex = owner.Nodes.FixedIndex; if (fixedIndex != -1) { node.index = fixedIndex + delta; } else { //if fixedIndex != -1 capacity was ensured by AddRange Debug.Assert(delta == 0,"delta should be 0"); owner.EnsureCapacity(1); node.index = owner.childCount; } owner.children[node.index] = node; owner.childCount++; node.Realize(false); if (tv != null && node == tv.selectedNode) tv.SelectedNode = node; // communicate this to the handle if (tv != null && tv.TreeViewNodeSorter != null) { tv.Sort(); } return node.index; } ////// int IList.Add(object node) { if (node == null) { throw new ArgumentNullException("node"); } else if (node is TreeNode) { return Add((TreeNode)node); } else { return Add(node.ToString()).index; } } /// /// /// public bool Contains(TreeNode node) { return IndexOf(node) != -1; } ///[To be supplied.] ////// /// public virtual bool ContainsKey(string key) { return IsValidIndex(IndexOfKey(key)); } ///Returns true if the collection contains an item with the specified key, false otherwise. ////// bool IList.Contains(object node) { if (node is TreeNode) { return Contains((TreeNode)node); } else { return false; } } /// /// /// public int IndexOf(TreeNode node) { for(int index=0; index < Count; ++index) { if (this[index] == node) { return index; } } return -1; } ///[To be supplied.] ////// int IList.IndexOf(object node) { if (node is TreeNode) { return IndexOf((TreeNode)node); } else { return -1; } } /// /// /// public virtual int IndexOfKey(String key) { // Step 0 - Arg validation if (string.IsNullOrEmpty(key)){ return -1; // we dont support empty or null keys. } // step 1 - check the last cached item if (IsValidIndex(lastAccessedIndex)) { if (WindowsFormsUtils.SafeCompareStrings(this[lastAccessedIndex].Name, key, /* ignoreCase = */ true)) { return lastAccessedIndex; } } // step 2 - search for the item for (int i = 0; i < this.Count; i ++) { if (WindowsFormsUtils.SafeCompareStrings(this[i].Name, key, /* ignoreCase = */ true)) { lastAccessedIndex = i; return i; } } // step 3 - we didn't find it. Invalidate the last accessed index and return -1. lastAccessedIndex = -1; return -1; } ///The zero-based index of the first occurrence of value within the entire CollectionBase, if found; otherwise, -1. ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual void Insert(int index, TreeNode node) { if (node.handle != IntPtr.Zero) throw new ArgumentException(SR.GetString(SR.OnlyOneControl, node.Text), "node"); // If the TreeView is sorted, index is ignored TreeView tv = owner.TreeView; if (tv != null && tv.Sorted) { owner.AddSorted(node); return; } if (index < 0) index = 0; if (index > owner.childCount) index = owner.childCount; owner.InsertNodeAt(index, node); } ////// void IList.Insert(int index, object node) { if (node is TreeNode) { Insert(index, (TreeNode)node); } else { throw new ArgumentException(SR.GetString(SR.TreeNodeCollectionBadTreeNode), "node"); } } // <-- NEW INSERT OVERLOADS IN WHIDBEY /// /// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string text) { TreeNode tn = new TreeNode(text); Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text) { TreeNode tn = new TreeNode(text); tn.Name = key; Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text, int imageIndex) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageIndex = imageIndex; Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text, string imageKey) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageKey = imageKey; Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text, int imageIndex, int selectedImageIndex) { TreeNode tn = new TreeNode(text, imageIndex, selectedImageIndex); tn.Name = key; Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text, string imageKey, string selectedImageKey) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageKey = imageKey; tn.SelectedImageKey = selectedImageKey; Insert(index, tn); return tn; } // END - NEW INSERT OVERLOADS IN WHIDBEY --> ////// /// ///Determines if the index is valid for the collection. ///private bool IsValidIndex(int index) { return ((index >= 0) && (index < this.Count)); } /// /// /// Remove all nodes from the tree view. /// public virtual void Clear() { owner.Clear(); } ////// /// public void CopyTo(Array dest, int index) { if (owner.childCount > 0) { System.Array.Copy(owner.children, 0, dest, index, owner.childCount); } } ///[To be supplied.] ////// /// public void Remove(TreeNode node) { node.Remove(); } ///[To be supplied.] ////// void IList.Remove(object node) { if (node is TreeNode ) { Remove((TreeNode)node); } } /// /// /// public virtual void RemoveAt(int index) { this[index].Remove(); } ///[To be supplied.] ////// /// public virtual void RemoveByKey(string key) { int index = IndexOfKey(key); if (IsValidIndex(index)) { RemoveAt(index); } } ///Removes the child control with the specified key. ////// /// public IEnumerator GetEnumerator() { return new WindowsFormsUtils.ArraySubsetEnumerator(owner.children, owner.childCount); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.InteropServices; using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing.Design; using System.Globalization; ////// /// [ Editor("System.Windows.Forms.Design.TreeNodeCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] public class TreeNodeCollection : IList { private TreeNode owner; /// A caching mechanism for key accessor /// We use an index here rather than control so that we don't have lifetime /// issues by holding on to extra references. private int lastAccessedIndex = -1; //this index is used to optimize performance of AddRange //items are added from last to first after this index //(to work around TV_INSertItem comctl32 perf issue with consecutive adds in the end of the list) private int fixedIndex = -1; internal TreeNodeCollection(TreeNode owner) { this.owner = owner; } ///[To be supplied.] ////// internal int FixedIndex { get { return fixedIndex; } set { fixedIndex = value; } } /// /// /// public virtual TreeNode this[int index] { get { if (index < 0 || index >= owner.childCount) { throw new ArgumentOutOfRangeException("index"); } return owner.children[index]; } set { if (index < 0 || index >= owner.childCount) throw new ArgumentOutOfRangeException("index", SR.GetString(SR.InvalidArgument, "index", (index).ToString(CultureInfo.CurrentCulture))); value.parent = owner; value.index = index; owner.children[index] = value; value.Realize(false); } } ///[To be supplied.] ////// object IList.this[int index] { get { return this[index]; } set { if (value is TreeNode) { this[index] = (TreeNode)value; } else { throw new ArgumentException(SR.GetString(SR.TreeNodeCollectionBadTreeNode), "value"); } } } /// /// /// public virtual TreeNode this[string key] { get { // We do not support null and empty string as valid keys. if (string.IsNullOrEmpty(key)){ return null; } // Search for the key in our collection int index = IndexOfKey(key); if (IsValidIndex(index)) { return this[index]; } else { return null; } } } ///Retrieves the child control with the specified key. ////// /// // VSWhidbey 152051: Make this property available to Intellisense. (Removed the EditorBrowsable attribute.) [Browsable(false)] public int Count { get { return owner.childCount; } } ///[To be supplied.] ////// object ICollection.SyncRoot { get { return this; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// bool IList.IsFixedSize { get { return false; } } /// /// /// public bool IsReadOnly { get { return false; } } ///[To be supplied.] ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string text) { TreeNode tn = new TreeNode(text); Add(tn); return tn; } // <-- NEW ADD OVERLOADS IN WHIDBEY ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text) { TreeNode tn = new TreeNode(text); tn.Name = key; Add(tn); return tn; } ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text, int imageIndex) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageIndex = imageIndex; Add(tn); return tn; } ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text, string imageKey) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageKey = imageKey; Add(tn); return tn; } ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text, int imageIndex, int selectedImageIndex) { TreeNode tn = new TreeNode(text, imageIndex, selectedImageIndex); tn.Name = key; Add(tn); return tn; } ////// /// Creates a new child node under this node. Child node is positioned after siblings. /// public virtual TreeNode Add(string key, string text, string imageKey, string selectedImageKey) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageKey = imageKey; tn.SelectedImageKey = selectedImageKey; Add(tn); return tn; } // END - NEW ADD OVERLOADS IN WHIDBEY --> ////// /// public virtual void AddRange(TreeNode[] nodes) { if (nodes == null) { throw new ArgumentNullException("nodes"); } if (nodes.Length == 0) return; TreeView tv = owner.TreeView; if (tv != null && nodes.Length > TreeNode.MAX_TREENODES_OPS) { tv.BeginUpdate(); } owner.Nodes.FixedIndex = owner.childCount; owner.EnsureCapacity(nodes.Length); for (int i = nodes.Length-1 ; i >= 0; i--) { AddInternal(nodes[i],i); } owner.Nodes.FixedIndex = -1; if (tv != null && nodes.Length > TreeNode.MAX_TREENODES_OPS) { tv.EndUpdate(); } } ///[To be supplied.] ////// /// public TreeNode[] Find (string key, bool searchAllChildren) { ArrayList foundNodes = FindInternal(key, searchAllChildren, this, new ArrayList()); // TreeNode[] stronglyTypedFoundNodes = new TreeNode[foundNodes.Count]; foundNodes.CopyTo(stronglyTypedFoundNodes, 0); return stronglyTypedFoundNodes; } private ArrayList FindInternal(string key, bool searchAllChildren, TreeNodeCollection treeNodeCollectionToLookIn, ArrayList foundTreeNodes) { if ((treeNodeCollectionToLookIn == null) || (foundTreeNodes == null)) { return null; } // Perform breadth first search - as it's likely people will want tree nodes belonging // to the same parent close to each other. for (int i = 0; i < treeNodeCollectionToLookIn.Count; i++) { if (treeNodeCollectionToLookIn[i] == null){ continue; } if (WindowsFormsUtils.SafeCompareStrings(treeNodeCollectionToLookIn[i].Name, key, /* ignoreCase = */ true)) { foundTreeNodes.Add(treeNodeCollectionToLookIn[i]); } } // Optional recurive search for controls in child collections. if (searchAllChildren){ for (int i = 0; i < treeNodeCollectionToLookIn.Count; i++) { if (treeNodeCollectionToLookIn[i] == null){ continue; } if ((treeNodeCollectionToLookIn[i].Nodes != null) && treeNodeCollectionToLookIn[i].Nodes.Count > 0){ // if it has a valid child collecion, append those results to our collection foundTreeNodes = FindInternal(key, searchAllChildren, treeNodeCollectionToLookIn[i].Nodes, foundTreeNodes); } } } return foundTreeNodes; } ///[To be supplied.] ////// /// Adds a new child node to this node. Child node is positioned after siblings. /// public virtual int Add(TreeNode node) { return AddInternal(node, 0); } private int AddInternal(TreeNode node, int delta) { if (node == null) { throw new ArgumentNullException("node"); } if (node.handle != IntPtr.Zero) throw new ArgumentException(SR.GetString(SR.OnlyOneControl, node.Text), "node"); // If the TreeView is sorted, index is ignored TreeView tv = owner.TreeView; if (tv != null && tv.Sorted) { return owner.AddSorted(node); } node.parent = owner; int fixedIndex = owner.Nodes.FixedIndex; if (fixedIndex != -1) { node.index = fixedIndex + delta; } else { //if fixedIndex != -1 capacity was ensured by AddRange Debug.Assert(delta == 0,"delta should be 0"); owner.EnsureCapacity(1); node.index = owner.childCount; } owner.children[node.index] = node; owner.childCount++; node.Realize(false); if (tv != null && node == tv.selectedNode) tv.SelectedNode = node; // communicate this to the handle if (tv != null && tv.TreeViewNodeSorter != null) { tv.Sort(); } return node.index; } ////// int IList.Add(object node) { if (node == null) { throw new ArgumentNullException("node"); } else if (node is TreeNode) { return Add((TreeNode)node); } else { return Add(node.ToString()).index; } } /// /// /// public bool Contains(TreeNode node) { return IndexOf(node) != -1; } ///[To be supplied.] ////// /// public virtual bool ContainsKey(string key) { return IsValidIndex(IndexOfKey(key)); } ///Returns true if the collection contains an item with the specified key, false otherwise. ////// bool IList.Contains(object node) { if (node is TreeNode) { return Contains((TreeNode)node); } else { return false; } } /// /// /// public int IndexOf(TreeNode node) { for(int index=0; index < Count; ++index) { if (this[index] == node) { return index; } } return -1; } ///[To be supplied.] ////// int IList.IndexOf(object node) { if (node is TreeNode) { return IndexOf((TreeNode)node); } else { return -1; } } /// /// /// public virtual int IndexOfKey(String key) { // Step 0 - Arg validation if (string.IsNullOrEmpty(key)){ return -1; // we dont support empty or null keys. } // step 1 - check the last cached item if (IsValidIndex(lastAccessedIndex)) { if (WindowsFormsUtils.SafeCompareStrings(this[lastAccessedIndex].Name, key, /* ignoreCase = */ true)) { return lastAccessedIndex; } } // step 2 - search for the item for (int i = 0; i < this.Count; i ++) { if (WindowsFormsUtils.SafeCompareStrings(this[i].Name, key, /* ignoreCase = */ true)) { lastAccessedIndex = i; return i; } } // step 3 - we didn't find it. Invalidate the last accessed index and return -1. lastAccessedIndex = -1; return -1; } ///The zero-based index of the first occurrence of value within the entire CollectionBase, if found; otherwise, -1. ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual void Insert(int index, TreeNode node) { if (node.handle != IntPtr.Zero) throw new ArgumentException(SR.GetString(SR.OnlyOneControl, node.Text), "node"); // If the TreeView is sorted, index is ignored TreeView tv = owner.TreeView; if (tv != null && tv.Sorted) { owner.AddSorted(node); return; } if (index < 0) index = 0; if (index > owner.childCount) index = owner.childCount; owner.InsertNodeAt(index, node); } ////// void IList.Insert(int index, object node) { if (node is TreeNode) { Insert(index, (TreeNode)node); } else { throw new ArgumentException(SR.GetString(SR.TreeNodeCollectionBadTreeNode), "node"); } } // <-- NEW INSERT OVERLOADS IN WHIDBEY /// /// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string text) { TreeNode tn = new TreeNode(text); Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text) { TreeNode tn = new TreeNode(text); tn.Name = key; Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text, int imageIndex) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageIndex = imageIndex; Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text, string imageKey) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageKey = imageKey; Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text, int imageIndex, int selectedImageIndex) { TreeNode tn = new TreeNode(text, imageIndex, selectedImageIndex); tn.Name = key; Insert(index, tn); return tn; } ////// /// Inserts a new child node on this node. Child node is positioned as specified by index. /// public virtual TreeNode Insert(int index, string key, string text, string imageKey, string selectedImageKey) { TreeNode tn = new TreeNode(text); tn.Name = key; tn.ImageKey = imageKey; tn.SelectedImageKey = selectedImageKey; Insert(index, tn); return tn; } // END - NEW INSERT OVERLOADS IN WHIDBEY --> ////// /// ///Determines if the index is valid for the collection. ///private bool IsValidIndex(int index) { return ((index >= 0) && (index < this.Count)); } /// /// /// Remove all nodes from the tree view. /// public virtual void Clear() { owner.Clear(); } ////// /// public void CopyTo(Array dest, int index) { if (owner.childCount > 0) { System.Array.Copy(owner.children, 0, dest, index, owner.childCount); } } ///[To be supplied.] ////// /// public void Remove(TreeNode node) { node.Remove(); } ///[To be supplied.] ////// void IList.Remove(object node) { if (node is TreeNode ) { Remove((TreeNode)node); } } /// /// /// public virtual void RemoveAt(int index) { this[index].Remove(); } ///[To be supplied.] ////// /// public virtual void RemoveByKey(string key) { int index = IndexOfKey(key); if (IsValidIndex(index)) { RemoveAt(index); } } ///Removes the child control with the specified key. ////// /// public IEnumerator GetEnumerator() { return new WindowsFormsUtils.ArraySubsetEnumerator(owner.children, owner.childCount); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
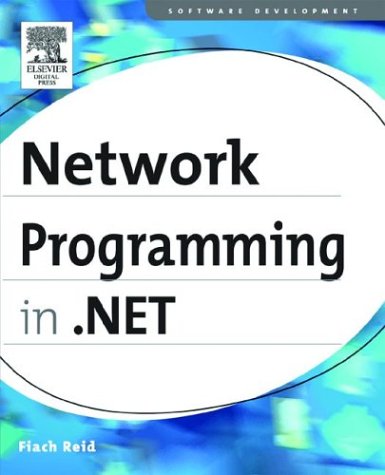
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewRow.cs
- SelectQueryOperator.cs
- RadioButtonList.cs
- NetMsmqBinding.cs
- UriTemplateDispatchFormatter.cs
- ObjectReferenceStack.cs
- EdmValidator.cs
- BinaryReader.cs
- PolyLineSegment.cs
- TextDpi.cs
- SystemResourceHost.cs
- ProcessExitedException.cs
- AnnotationHelper.cs
- ColumnMapVisitor.cs
- GridViewItemAutomationPeer.cs
- ToolStripDropDownClosedEventArgs.cs
- ClientApiGenerator.cs
- SynchronizationFilter.cs
- CodeAssignStatement.cs
- Ray3DHitTestResult.cs
- StringKeyFrameCollection.cs
- HtmlInputHidden.cs
- FileDetails.cs
- PnrpPeerResolver.cs
- ResourcesChangeInfo.cs
- DirectoryInfo.cs
- Double.cs
- TextUtf8RawTextWriter.cs
- RegisteredExpandoAttribute.cs
- XmlSerializerNamespaces.cs
- DynamicValueConverter.cs
- CommandBinding.cs
- CompleteWizardStep.cs
- Internal.cs
- GlyphCache.cs
- SecurityVerifiedMessage.cs
- ButtonAutomationPeer.cs
- XPathNodeIterator.cs
- FilterFactory.cs
- WSSecureConversationDec2005.cs
- InstanceOwnerException.cs
- FontCollection.cs
- Latin1Encoding.cs
- InteropBitmapSource.cs
- VisualSerializer.cs
- ImageBrush.cs
- UnsafeNativeMethods.cs
- input.cs
- XmlCharCheckingReader.cs
- EnvelopedSignatureTransform.cs
- SiteMapNodeItemEventArgs.cs
- RSACryptoServiceProvider.cs
- Converter.cs
- StringSorter.cs
- FormattedTextSymbols.cs
- EntityDataSourceUtil.cs
- EntityClassGenerator.cs
- ScriptControl.cs
- SymbolPair.cs
- ProfileGroupSettings.cs
- ObjectListCommandCollection.cs
- Expr.cs
- DocumentGridContextMenu.cs
- BamlLocalizationDictionary.cs
- CalendarKeyboardHelper.cs
- UInt64Converter.cs
- TextBoxAutomationPeer.cs
- HandleCollector.cs
- WinFormsSecurity.cs
- WebPartCollection.cs
- DataGridCaption.cs
- ObjectListItemCollection.cs
- ScaleTransform3D.cs
- OutputCacheProfileCollection.cs
- SafeMemoryMappedFileHandle.cs
- MethodCallConverter.cs
- EmptyStringExpandableObjectConverter.cs
- CachedBitmap.cs
- PeerPresenceInfo.cs
- StubHelpers.cs
- IndexedEnumerable.cs
- SessionEndedEventArgs.cs
- DataListItem.cs
- ResourceContainer.cs
- TreeView.cs
- MethodBuilder.cs
- TransactionScope.cs
- SlipBehavior.cs
- ActivityPreviewDesigner.cs
- GreenMethods.cs
- DynamicMethod.cs
- SamlAction.cs
- SQLDecimal.cs
- ColorTransformHelper.cs
- DocumentViewerBase.cs
- SystemTcpConnection.cs
- ChildTable.cs
- DetailsViewPageEventArgs.cs
- FullTextLine.cs
- Thumb.cs