Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / PageContentAsyncResult.cs / 2 / PageContentAsyncResult.cs
//---------------------------------------------------------------------------- //// Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // // Description: // Implements the PageContentAsyncResult // // History: // 02/25/2004 - [....] ([....]) - Created. // // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Diagnostics; using System.IO; using System.Windows.Threading; using System.Threading; using MS.Internal; using MS.Internal.AppModel; using MS.Internal.Utility; using MS.Internal.Navigation; using MS.Utility; using System.Reflection; using System.Windows.Controls; using System.Windows.Markup; using System.Net; using System.IO.Packaging; ////// IAsyncResult for GetPageAsync. This item is passed around and queued up during various /// phase of async call. /// internal sealed class PageContentAsyncResult : IAsyncResult { //------------------------------------------------------------------- // // Internal enum // //--------------------------------------------------------------------- internal enum GetPageStatus { Loading, Cancelled, Finished } //-------------------------------------------------------------------- // // Ctor // //--------------------------------------------------------------------- #region Ctor internal PageContentAsyncResult(AsyncCallback callback, object state, Dispatcher dispatcher, Uri baseUri, Uri source, FixedPage child) { this._dispatcher = dispatcher; this._isCompleted = false; this._completedSynchronously = false; this._callback = callback; this._asyncState = state; this._getpageStatus = GetPageStatus.Loading; this._child = child; this._baseUri = baseUri; Debug.Assert(source == null || source.IsAbsoluteUri); this._source = source; } #endregion Ctor //-------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- //------------------------------------------------------------------- // // Public Properties // //---------------------------------------------------------------------- #region IAsyncResult //--------------------------------------------------------------------- ////// Gets a user-defined object that contains information about /// this GetPageAsync call /// public object AsyncState { get { return _asyncState; } } //--------------------------------------------------------------------- ////// Gets a WaitHandle that is used to wait for the asynchrounus /// GetPageAsync to complete. We are not providing WaitHandle /// since this can be called on the main UIThread. /// public WaitHandle AsyncWaitHandle { get { Debug.Assert(false); return null; } } //--------------------------------------------------------------------- ////// Gets an indication of whether the asynchronous GetPage /// completed synchronously. /// public bool CompletedSynchronously { get { return _completedSynchronously; } } //---------------------------------------------------------------------- ////// Gets an indication whether the asynchronous GetPage has finished /// public bool IsCompleted { get { return _isCompleted; } } #endregion IAsyncResult //------------------------------------------------------------------- // // Internal Methods // //---------------------------------------------------------------------- #region DispatcherOperationCallback //---------------------------------------------------------------------- internal object Dispatch(object arg) { if (this._exception != null) { // force finish if there was any exception this._getpageStatus = GetPageStatus.Finished; } switch (this._getpageStatus) { case GetPageStatus.Loading: try { if (this._child != null) { this._completedSynchronously = true; this._result = this._child; _getpageStatus = GetPageStatus.Finished; goto case GetPageStatus.Finished; } // // Note if _source == null, exception will // be thrown. // Stream responseStream = null; object o = null; WebResponse response = WpfWebRequestHelper.CreateRequestAndGetResponse(this._source); responseStream = response.GetResponseStream(); ParserContext pc = new ParserContext(); pc.BaseUri = this._source; XpsValidatingLoader loader = new XpsValidatingLoader(); o = loader.Load(responseStream, _baseUri, pc, new ContentType(response.ContentType)); if (o == null) { throw new ApplicationException(SR.Get(SRID.PageContentUnsupportedMimeType)); } _result = o as FixedPage; if (_result == null) { throw new ApplicationException(SR.Get(SRID.PageContentUnsupportedPageType, o.GetType())); } if (_result.IsInitialized) { responseStream.Close(); } else { _pendingStream = responseStream; _result.Initialized += new EventHandler(_OnPaserFinished); } _getpageStatus = GetPageStatus.Finished; } catch (ApplicationException e) { this._exception = e; } goto case GetPageStatus.Finished; case GetPageStatus.Cancelled: // do nothing goto case GetPageStatus.Finished; case GetPageStatus.Finished: _isCompleted = true; if (_callback != null) { _callback(this); } break; } return null; } #endregion DispatcherOperationCallback //----------------------------------------------------------------- internal void Cancel() { _getpageStatus = GetPageStatus.Cancelled; // } internal void Wait() { _dispatcherOperation.Wait(); } //-------------------------------------------------------------------- // // Internal Properties // //--------------------------------------------------------------------- #region Internal Properties //--------------------------------------------------------------------- internal Exception Exception { get { return _exception; } } //----------------------------------------------------------------- internal bool IsCancelled { get { return _getpageStatus == GetPageStatus.Cancelled; } } internal DispatcherOperation DispatcherOperation { set { _dispatcherOperation = value; } } //------------------------------------------------------------------ internal FixedPage Result { get { return _result; } } #endregion Internal Properties //------------------------------------------------------------------- // // Private Methods // //---------------------------------------------------------------------- #region Private Methods private void _OnPaserFinished(object sender, EventArgs args) { if (_pendingStream != null) { _pendingStream.Close(); _pendingStream = null; } } #endregion Private Methods //-------------------------------------------------------------------- // // Private Fields // //--------------------------------------------------------------------- #region Private private object _asyncState; private bool _isCompleted; private bool _completedSynchronously; private AsyncCallback _callback; private Exception _exception; private GetPageStatus _getpageStatus; private Uri _baseUri; private Uri _source; private FixedPage _child; private Dispatcher _dispatcher; private FixedPage _result; private Stream _pendingStream; private DispatcherOperation _dispatcherOperation; #endregion Private } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
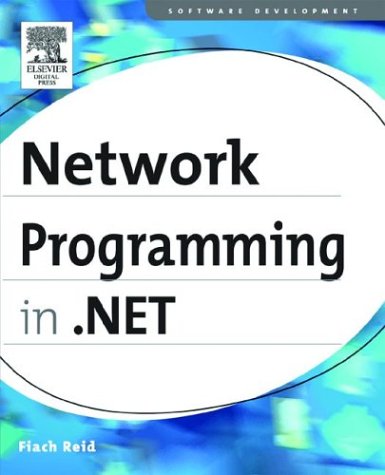
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ComNativeDescriptor.cs
- Hex.cs
- ContentPathSegment.cs
- StringUtil.cs
- ProvidePropertyAttribute.cs
- RemoteWebConfigurationHostStream.cs
- StreamedFramingRequestChannel.cs
- ProfilePropertyNameValidator.cs
- ResXFileRef.cs
- HelpEvent.cs
- DataGridViewRowStateChangedEventArgs.cs
- DNS.cs
- NativeMethods.cs
- ToolStripItemImageRenderEventArgs.cs
- EntryIndex.cs
- CompiledRegexRunner.cs
- NamespaceEmitter.cs
- TableStyle.cs
- RelationshipEndCollection.cs
- XmlSchemaFacet.cs
- XmlSchemaAppInfo.cs
- DataReceivedEventArgs.cs
- PrivilegeNotHeldException.cs
- XmlCollation.cs
- BitmapMetadataEnumerator.cs
- DocumentOrderQuery.cs
- XmlSchemaValidationException.cs
- GatewayDefinition.cs
- WindowsRebar.cs
- UserPreferenceChangingEventArgs.cs
- SchemaLookupTable.cs
- SuppressMergeCheckAttribute.cs
- HashCodeCombiner.cs
- ProtocolsConfigurationEntry.cs
- TraceProvider.cs
- HttpFileCollectionWrapper.cs
- XmlAutoDetectWriter.cs
- PlanCompiler.cs
- MetadataItemCollectionFactory.cs
- GridViewUpdatedEventArgs.cs
- AnonymousIdentificationSection.cs
- ProfileBuildProvider.cs
- ReadOnlyAttribute.cs
- NotSupportedException.cs
- DataServiceClientException.cs
- MiniLockedBorderGlyph.cs
- FilteredAttributeCollection.cs
- FamilyMap.cs
- MeasureItemEvent.cs
- CustomBindingElementCollection.cs
- ColumnMapVisitor.cs
- TraceSource.cs
- SortKey.cs
- ProfileGroupSettingsCollection.cs
- SizeChangedEventArgs.cs
- CanExecuteRoutedEventArgs.cs
- CellTreeNode.cs
- CollectionViewProxy.cs
- EventLogPermissionAttribute.cs
- BinaryUtilClasses.cs
- DictionaryBase.cs
- CipherData.cs
- Translator.cs
- DeadLetterQueue.cs
- SystemColorTracker.cs
- NativeMethods.cs
- ContentType.cs
- PageDeviceFont.cs
- AtomicFile.cs
- TableSectionStyle.cs
- CopyNamespacesAction.cs
- ActivityBuilderHelper.cs
- __Filters.cs
- ColumnMapCopier.cs
- ObjectDataSourceStatusEventArgs.cs
- ObjectDataSourceView.cs
- Crypto.cs
- SqlTopReducer.cs
- DataGridViewRowEventArgs.cs
- DescendentsWalkerBase.cs
- PrintPreviewDialog.cs
- DataGridPageChangedEventArgs.cs
- SubstitutionList.cs
- webbrowsersite.cs
- ToolBarDesigner.cs
- IdentityReference.cs
- BamlResourceSerializer.cs
- ManualResetEvent.cs
- TextEffectResolver.cs
- KeyInstance.cs
- WorkflowPrinting.cs
- TableLayoutSettings.cs
- SubpageParaClient.cs
- AspCompat.cs
- MimeMapping.cs
- ExpressionsCollectionConverter.cs
- RTLAwareMessageBox.cs
- CacheHelper.cs
- SessionState.cs
- SymbolDocumentGenerator.cs