Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / ImageSourceValueSerializer.cs / 2 / ImageSourceValueSerializer.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2005 // // File: ImageSourceValueSerializer.cs // // Contents: Value serializer for ImageSource instances // // Created: 06/21/2005 chuckj // //----------------------------------------------------------------------- #pragma warning disable 1634, 1691 // Allow suppression of certain presharp messages using System; using System.Collections.Generic; using System.Globalization; using System.Text; using System.Windows.Markup; using System.Windows.Media.Imaging; namespace System.Windows.Media { ////// Value serializer for Transform instances /// public class ImageSourceValueSerializer : ValueSerializer { ////// Returns true. /// public override bool CanConvertFromString(string value, IValueSerializerContext context) { return true; } ////// Returns true if the given transform can be converted into a string /// public override bool CanConvertToString(object value, IValueSerializerContext context) { ImageSource imageSource = value as ImageSource; #pragma warning disable 6506 return imageSource != null && imageSource.CanSerializeToString(); #pragma warning restore 6506 } ////// Converts a string into a transform. /// public override object ConvertFromString(string value, IValueSerializerContext context) { if (!string.IsNullOrEmpty(value)) { UriHolder uriHolder = TypeConverterHelper.GetUriFromUriContext(context, value); return BitmapFrame.CreateFromUriOrStream( uriHolder.BaseUri, uriHolder.OriginalUri, null, BitmapCreateOptions.None, BitmapCacheOption.Default, null ); } return base.ConvertFromString(value, context); } ////// Converts a transform into a string. /// public override string ConvertToString(object value, IValueSerializerContext context) { ImageSource imageSource = value as ImageSource; if (imageSource != null) return imageSource.ConvertToString(null, CultureInfo.GetCultureInfo("en-us")); else return base.ConvertToString(value, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
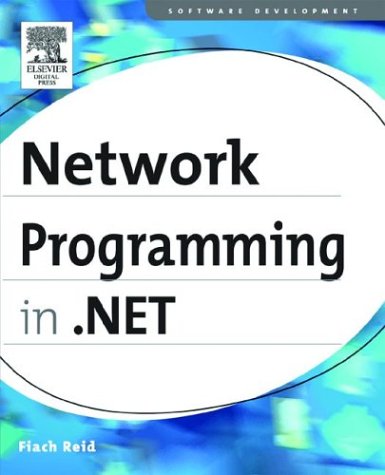
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegularExpressionValidator.cs
- QilVisitor.cs
- parserscommon.cs
- WebBrowserEvent.cs
- SimpleType.cs
- METAHEADER.cs
- LineSegment.cs
- RangeValueProviderWrapper.cs
- DataGridClipboardCellContent.cs
- ExplicitDiscriminatorMap.cs
- OperatingSystem.cs
- ClickablePoint.cs
- FontClient.cs
- DataFormat.cs
- ExpandSegmentCollection.cs
- PageCatalogPart.cs
- ReadOnlyDataSource.cs
- EraserBehavior.cs
- ConstraintCollection.cs
- SecurityTimestamp.cs
- DocumentApplication.cs
- FacetDescriptionElement.cs
- CompositeActivityTypeDescriptorProvider.cs
- TcpChannelHelper.cs
- LabelInfo.cs
- SiteMapNodeCollection.cs
- NamedPipeAppDomainProtocolHandler.cs
- SQLBoolean.cs
- ActivityDesignerLayoutSerializers.cs
- EventWaitHandleSecurity.cs
- EntityProxyTypeInfo.cs
- SmtpNegotiateAuthenticationModule.cs
- CompilerInfo.cs
- EpmSyndicationContentSerializer.cs
- StorageMappingItemLoader.cs
- InvalidComObjectException.cs
- FilteredDataSetHelper.cs
- Codec.cs
- TypeElement.cs
- OdbcConnectionStringbuilder.cs
- webclient.cs
- Cursors.cs
- ReceiveSecurityHeaderElementManager.cs
- TraceHwndHost.cs
- Page.cs
- MdiWindowListItemConverter.cs
- SubclassTypeValidator.cs
- DataObjectFieldAttribute.cs
- CheckableControlBaseAdapter.cs
- FragmentNavigationEventArgs.cs
- GridViewSortEventArgs.cs
- ObjectDataSourceStatusEventArgs.cs
- EdmEntityTypeAttribute.cs
- XmlHierarchicalDataSourceView.cs
- SoapTypeAttribute.cs
- Pair.cs
- WinEventWrap.cs
- StateMachineHelpers.cs
- X509IssuerSerialKeyIdentifierClause.cs
- EntityTypeBase.cs
- ElasticEase.cs
- ListBindableAttribute.cs
- SystemIPGlobalProperties.cs
- TextElement.cs
- Ray3DHitTestResult.cs
- RuntimeIdentifierPropertyAttribute.cs
- OutputCacheProfile.cs
- TextSelectionHighlightLayer.cs
- ExtensibleClassFactory.cs
- DuplicateDetector.cs
- DesignerActionPropertyItem.cs
- XhtmlBasicTextViewAdapter.cs
- TextTreeUndo.cs
- NamedElement.cs
- ApplicationActivator.cs
- HtmlControlPersistable.cs
- TextEffect.cs
- Message.cs
- ResourceWriter.cs
- BmpBitmapEncoder.cs
- Matrix3DStack.cs
- ISFTagAndGuidCache.cs
- HtmlContainerControl.cs
- TextElementEnumerator.cs
- TextLine.cs
- GradientBrush.cs
- AssociativeAggregationOperator.cs
- StylusShape.cs
- SolidColorBrush.cs
- SelectionChangedEventArgs.cs
- LiteralTextParser.cs
- Converter.cs
- ValueType.cs
- MessageHeaderInfoTraceRecord.cs
- XmlSchemaType.cs
- ReadOnlyCollectionBase.cs
- Helpers.cs
- InputMethodStateChangeEventArgs.cs
- PaperSize.cs
- StoreAnnotationsMap.cs