Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / ManagedLibraries / Remoting / Channels / TCP / TcpChannelHelper.cs / 1305376 / TcpChannelHelper.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //========================================================================== // File: TcpChannelHelper.cs // // Summary: Implements helper methods for tcp client and server channels. // //========================================================================= using System; using System.Text; using System.Runtime.Remoting.Channels; namespace System.Runtime.Remoting.Channels.Tcp { internal static class TcpChannelHelper { private const String _tcp = "tcp://"; // Used by tcp channels to implement IChannel::Parse. // It returns the channel uri and places object uri into out parameter. internal static String ParseURL(String url, out String objectURI) { // Set the out parameters objectURI = null; int separator; // Find the starting point of tcp:// // NOTE: We are using this version of String.Compare to ensure // that string operations are case-insensitive!! if (StringHelper.StartsWithAsciiIgnoreCasePrefixLower(url, _tcp)) { separator = _tcp.Length; } else { return null; } // find next slash (after end of scheme) separator = url.IndexOf('/', separator); if (-1 == separator) { return url; // means that the url is just "tcp://foo:90" or something like that } // Extract the channel URI which is the prefix String channelURI = url.Substring(0, separator); // Extract the object URI which is the suffix objectURI = url.Substring(separator); // leave the slash return channelURI; } // ParseURL } // class TcpChannelHelper } // namespace System.Runtime.Remoting.Channels.Tcp // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
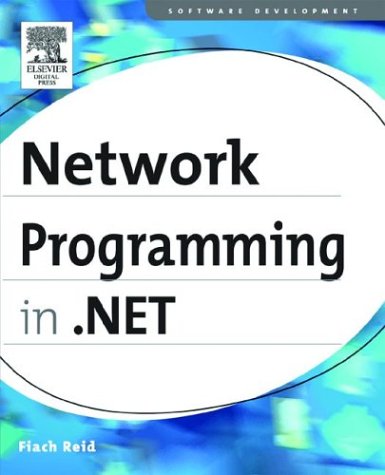
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputLanguageSource.cs
- EncodingNLS.cs
- UniqueConstraint.cs
- EmptyQuery.cs
- ClipboardData.cs
- EasingQuaternionKeyFrame.cs
- BindingsCollection.cs
- CustomAttribute.cs
- TextAdaptor.cs
- GenericPrincipal.cs
- PopupRoot.cs
- TraceSource.cs
- _ConnectStream.cs
- SqlError.cs
- XmlComplianceUtil.cs
- LoginUtil.cs
- LinkDescriptor.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- DesignerFrame.cs
- XmlQueryOutput.cs
- XmlDataCollection.cs
- DesignerDataView.cs
- ExpressionBuilder.cs
- ResourceReader.cs
- indexingfiltermarshaler.cs
- DataTableMappingCollection.cs
- MatrixConverter.cs
- NullReferenceException.cs
- ReadContentAsBinaryHelper.cs
- NameObjectCollectionBase.cs
- BaseDataList.cs
- ColumnReorderedEventArgs.cs
- LeftCellWrapper.cs
- CommentEmitter.cs
- StrongNameUtility.cs
- FileDialog_Vista.cs
- SchemaHelper.cs
- SendMessageChannelCache.cs
- SkewTransform.cs
- OdbcRowUpdatingEvent.cs
- ExpressionPrefixAttribute.cs
- FormsAuthenticationConfiguration.cs
- InvalidFilterCriteriaException.cs
- Operand.cs
- RectangleHotSpot.cs
- DynamicResourceExtension.cs
- ConfigPathUtility.cs
- EdmType.cs
- DecimalAnimationBase.cs
- hwndwrapper.cs
- WebPartChrome.cs
- BehaviorEditorPart.cs
- XmlIterators.cs
- Stopwatch.cs
- SecurityDocument.cs
- WebColorConverter.cs
- BoundField.cs
- RoutingSection.cs
- XPathDocumentIterator.cs
- XmlDataLoader.cs
- AdapterUtil.cs
- GridLength.cs
- MethodCallExpression.cs
- EventData.cs
- WindowsStreamSecurityBindingElement.cs
- ConfigurationElementCollection.cs
- XmlnsCache.cs
- TypedTableHandler.cs
- XPathNodeList.cs
- DbSetClause.cs
- FileDialog_Vista.cs
- CodeAttributeArgument.cs
- SQLGuid.cs
- BodyWriter.cs
- DataGridViewButtonCell.cs
- WindowsFont.cs
- Buffer.cs
- DEREncoding.cs
- PerformanceCountersElement.cs
- XmlFormatExtensionPrefixAttribute.cs
- Win32.cs
- DataGridRow.cs
- SecurityElement.cs
- BasicViewGenerator.cs
- DataGridState.cs
- Underline.cs
- TextRunTypographyProperties.cs
- ScaleTransform3D.cs
- DataProtection.cs
- Encoder.cs
- NamespaceList.cs
- XmlAtomicValue.cs
- PagesChangedEventArgs.cs
- ReaderWriterLock.cs
- CodeCompiler.cs
- TableParagraph.cs
- GAC.cs
- NamespaceDisplay.xaml.cs
- MulticastOption.cs
- StatusInfoItem.cs