Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / DynamicResourceExtension.cs / 1 / DynamicResourceExtension.cs
/****************************************************************************\ * * File: DynamicResourceExtension.cs * * Class for Xaml markup extension for static resource references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.ComponentModel; using System.Windows; using System.Windows.Markup; using System.Reflection; using MS.Internal; // Helper namespace System.Windows { ////// Class for Xaml markup extension for static resource references. /// [TypeConverter(typeof(DynamicResourceExtensionConverter))] [MarkupExtensionReturnType(typeof(object))] public class DynamicResourceExtension : MarkupExtension { ////// Constructor that takes no parameters /// public DynamicResourceExtension() { } ////// Constructor that takes the resource key that this is a static reference to. /// public DynamicResourceExtension( object resourceKey) { if (resourceKey == null) { throw new ArgumentNullException("resourceKey"); } _resourceKey = resourceKey; } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For DynamicResourceExtension, this is the object found in /// a resource dictionary in the current parent chain that is keyed by ResourceKey /// ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (ResourceKey == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionResourceKey)); } if (serviceProvider != null) { IProvideValueTarget provideValueTarget = serviceProvider.GetService(typeof(IProvideValueTarget)) as IProvideValueTarget; // DynamicResourceExtensions are not allowed On CLR props except for Setter,Trigger,Condition (bugs 1183373,1572537) DependencyObject targetDependencyObject; DependencyProperty targetDependencyProperty; Helper.CheckCanReceiveMarkupExtension(this, provideValueTarget, out targetDependencyObject, out targetDependencyProperty); } return new ResourceReferenceExpression(ResourceKey); } ////// The key in a Resource Dictionary used to find the object refered to by this /// Markup Extension. /// [ConstructorArgument("resourceKey")] // Uses an instance descriptor public object ResourceKey { get { return _resourceKey; } set { if (value == null) { throw new ArgumentNullException("value"); } _resourceKey = value; } } private object _resourceKey; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: DynamicResourceExtension.cs * * Class for Xaml markup extension for static resource references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.ComponentModel; using System.Windows; using System.Windows.Markup; using System.Reflection; using MS.Internal; // Helper namespace System.Windows { ////// Class for Xaml markup extension for static resource references. /// [TypeConverter(typeof(DynamicResourceExtensionConverter))] [MarkupExtensionReturnType(typeof(object))] public class DynamicResourceExtension : MarkupExtension { ////// Constructor that takes no parameters /// public DynamicResourceExtension() { } ////// Constructor that takes the resource key that this is a static reference to. /// public DynamicResourceExtension( object resourceKey) { if (resourceKey == null) { throw new ArgumentNullException("resourceKey"); } _resourceKey = resourceKey; } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For DynamicResourceExtension, this is the object found in /// a resource dictionary in the current parent chain that is keyed by ResourceKey /// ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (ResourceKey == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionResourceKey)); } if (serviceProvider != null) { IProvideValueTarget provideValueTarget = serviceProvider.GetService(typeof(IProvideValueTarget)) as IProvideValueTarget; // DynamicResourceExtensions are not allowed On CLR props except for Setter,Trigger,Condition (bugs 1183373,1572537) DependencyObject targetDependencyObject; DependencyProperty targetDependencyProperty; Helper.CheckCanReceiveMarkupExtension(this, provideValueTarget, out targetDependencyObject, out targetDependencyProperty); } return new ResourceReferenceExpression(ResourceKey); } ////// The key in a Resource Dictionary used to find the object refered to by this /// Markup Extension. /// [ConstructorArgument("resourceKey")] // Uses an instance descriptor public object ResourceKey { get { return _resourceKey; } set { if (value == null) { throw new ArgumentNullException("value"); } _resourceKey = value; } } private object _resourceKey; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
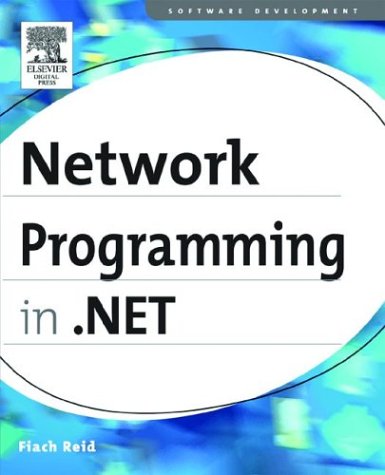
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Odbc32.cs
- SocketElement.cs
- CaseCqlBlock.cs
- TransactionTable.cs
- UriTemplateCompoundPathSegment.cs
- ScriptMethodAttribute.cs
- TemplateBamlTreeBuilder.cs
- IIS7UserPrincipal.cs
- XMLSyntaxException.cs
- InternalsVisibleToAttribute.cs
- FileDetails.cs
- String.cs
- HttpListenerRequest.cs
- CollectionAdapters.cs
- DaylightTime.cs
- ImageMetadata.cs
- Page.cs
- RsaSecurityTokenAuthenticator.cs
- __ConsoleStream.cs
- RuntimeCompatibilityAttribute.cs
- FrameworkTextComposition.cs
- ExportOptions.cs
- PcmConverter.cs
- ThrowHelper.cs
- Grid.cs
- DesigntimeLicenseContext.cs
- RegisteredExpandoAttribute.cs
- ToolStripLabel.cs
- EdmFunctions.cs
- Control.cs
- TdsParserSafeHandles.cs
- TogglePattern.cs
- ThaiBuddhistCalendar.cs
- UnsafeNativeMethods.cs
- DrawingAttributes.cs
- SymmetricAlgorithm.cs
- StylusTip.cs
- DefaultObjectMappingItemCollection.cs
- UserPersonalizationStateInfo.cs
- DependencyPropertyAttribute.cs
- ToolStrip.cs
- XPathSelectionIterator.cs
- MulticastOption.cs
- SapiRecoInterop.cs
- Primitive.cs
- SqlNotificationEventArgs.cs
- TextBoxAutomationPeer.cs
- objectquery_tresulttype.cs
- TextElementCollection.cs
- EntityCommandDefinition.cs
- ExtentJoinTreeNode.cs
- CharacterMetrics.cs
- WebSysDescriptionAttribute.cs
- CodeArrayIndexerExpression.cs
- CipherData.cs
- InfiniteTimeSpanConverter.cs
- HandleCollector.cs
- ConfigsHelper.cs
- httpserverutility.cs
- SoapDocumentServiceAttribute.cs
- ObjectSet.cs
- AssertSection.cs
- TextEffectCollection.cs
- AlternateViewCollection.cs
- COM2ExtendedTypeConverter.cs
- AdPostCacheSubstitution.cs
- DataGridCellsPresenter.cs
- InlineCategoriesDocument.cs
- TextDecorationCollectionConverter.cs
- iisPickupDirectory.cs
- BindingList.cs
- SafeMILHandleMemoryPressure.cs
- MaxValueConverter.cs
- formatter.cs
- PropertyChangingEventArgs.cs
- PathData.cs
- propertyentry.cs
- MultiSelectRootGridEntry.cs
- SQLBoolean.cs
- OdbcConnectionFactory.cs
- WFItemsToSpacerVisibility.cs
- Filter.cs
- TextBoxLine.cs
- WebDescriptionAttribute.cs
- Documentation.cs
- XsdBuildProvider.cs
- updateconfighost.cs
- LayoutManager.cs
- XmlNodeComparer.cs
- DataBoundControlHelper.cs
- DocComment.cs
- SafeFileMappingHandle.cs
- HtmlInputRadioButton.cs
- TransformProviderWrapper.cs
- DeflateEmulationStream.cs
- LockCookie.cs
- PrivateFontCollection.cs
- SyndicationElementExtensionCollection.cs
- BoolExpr.cs
- ZipIOCentralDirectoryDigitalSignature.cs