Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Markup / TemplateBamlTreeBuilder.cs / 1 / TemplateBamlTreeBuilder.cs
/****************************************************************************\ * * File: TemplateTreeBuilderBamlTranslator.cs * * Purpose: Class that builds a template object from BAML * * History: * 11/22/04: varsham Created * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Threading; using MS.Utility; namespace System.Windows.Markup { ////// TemplateTreeBuilderBamlTranslator is the TreeBuilder implementation that loads a Template object /// from BAML. /// internal class TemplateTreeBuilderBamlTranslator : TreeBuilderBamlTranslator { #region Constructors ////// Constructor. Set up associated baml reader to /// read the template baml records. /// public TemplateTreeBuilderBamlTranslator( ParserContext parserContext, // context Stream bamlStream, // baml stream, when reading from a file BamlRecord bamlStartRecord, // baml records start, when loading from a dictionary BamlRecord bamlIndexRecord, // Index Record in bamlRecords to start parsing at ParserStack bamlReaderStack, // reader stack ArrayList rootList, // List of root objects for overall parse. XamlParseMode parseMode, // [....] or async parse mode int maxAsyncRecords) // number to read in async mode before yielding { BamlStream = bamlStream; RecordReader = new TemplateBamlRecordReader(bamlStream, bamlStartRecord, bamlIndexRecord, parserContext, bamlReaderStack, rootList); XamlParseMode = parseMode; MaxAsyncRecords = maxAsyncRecords; // Set associated record reader async info RecordReader.XamlParseMode = XamlParseMode; RecordReader.MaxAsyncRecords = MaxAsyncRecords; } #endregion Constructors #region Overrides ////// Internal Avalon method. Used to parse template section of a BAML file. /// ///An array containing the root objects in the template portion of a BAML stream public override object ParseFragment() { // if in synchronous mode then just read the baml stream and then // return. if going into async mode then build up the first tag // synchronously and then post a queue item. if (XamlParseMode == XamlParseMode.Synchronous) { RecordReader.Read(); } else { // read in the first record since binder at present // needs this. bool moreData = true; // sit in synchronous read until get first root. Need this // until we get async binder support. while (GetRoot() == null && moreData) { moreData = RecordReader.Read(true /* single record mode*/); } if (moreData && GetRoot() != null) { // before going async want to switch to async stream interfaces // so we don't block on I/O. // setup stream Manager on Reader and kick of Async Writes StreamManager = new ReadWriteStreamManager(); // RecordReader no points to the ReaderWriter stream which // is different from our BAMLStream member. RecordReader.BamlStream = StreamManager.ReaderStream; // now spin a thread to read the BAML. This can change // once we get Read support that fails if contents isn't // available instead of blocks. ThreadStart threadStart = new ThreadStart(ReadBamlAsync); Thread thread = new Thread(threadStart); thread.Start(); // post a work item to do the rest. Post(); } } return GetRoot(); } #endregion Overrides } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: TemplateTreeBuilderBamlTranslator.cs * * Purpose: Class that builds a template object from BAML * * History: * 11/22/04: varsham Created * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Threading; using MS.Utility; namespace System.Windows.Markup { ////// TemplateTreeBuilderBamlTranslator is the TreeBuilder implementation that loads a Template object /// from BAML. /// internal class TemplateTreeBuilderBamlTranslator : TreeBuilderBamlTranslator { #region Constructors ////// Constructor. Set up associated baml reader to /// read the template baml records. /// public TemplateTreeBuilderBamlTranslator( ParserContext parserContext, // context Stream bamlStream, // baml stream, when reading from a file BamlRecord bamlStartRecord, // baml records start, when loading from a dictionary BamlRecord bamlIndexRecord, // Index Record in bamlRecords to start parsing at ParserStack bamlReaderStack, // reader stack ArrayList rootList, // List of root objects for overall parse. XamlParseMode parseMode, // [....] or async parse mode int maxAsyncRecords) // number to read in async mode before yielding { BamlStream = bamlStream; RecordReader = new TemplateBamlRecordReader(bamlStream, bamlStartRecord, bamlIndexRecord, parserContext, bamlReaderStack, rootList); XamlParseMode = parseMode; MaxAsyncRecords = maxAsyncRecords; // Set associated record reader async info RecordReader.XamlParseMode = XamlParseMode; RecordReader.MaxAsyncRecords = MaxAsyncRecords; } #endregion Constructors #region Overrides ////// Internal Avalon method. Used to parse template section of a BAML file. /// ///An array containing the root objects in the template portion of a BAML stream public override object ParseFragment() { // if in synchronous mode then just read the baml stream and then // return. if going into async mode then build up the first tag // synchronously and then post a queue item. if (XamlParseMode == XamlParseMode.Synchronous) { RecordReader.Read(); } else { // read in the first record since binder at present // needs this. bool moreData = true; // sit in synchronous read until get first root. Need this // until we get async binder support. while (GetRoot() == null && moreData) { moreData = RecordReader.Read(true /* single record mode*/); } if (moreData && GetRoot() != null) { // before going async want to switch to async stream interfaces // so we don't block on I/O. // setup stream Manager on Reader and kick of Async Writes StreamManager = new ReadWriteStreamManager(); // RecordReader no points to the ReaderWriter stream which // is different from our BAMLStream member. RecordReader.BamlStream = StreamManager.ReaderStream; // now spin a thread to read the BAML. This can change // once we get Read support that fails if contents isn't // available instead of blocks. ThreadStart threadStart = new ThreadStart(ReadBamlAsync); Thread thread = new Thread(threadStart); thread.Start(); // post a work item to do the rest. Post(); } } return GetRoot(); } #endregion Overrides } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
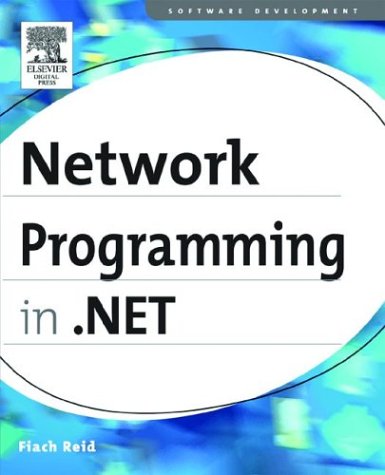
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlNamespaceMappingCollection.cs
- HTMLTagNameToTypeMapper.cs
- QfeChecker.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- RedirectionProxy.cs
- DataGridViewRowEventArgs.cs
- _SingleItemRequestCache.cs
- XmlDocumentType.cs
- XmlDocument.cs
- EmbeddedMailObject.cs
- WebScriptServiceHostFactory.cs
- WindowsTokenRoleProvider.cs
- ManifestSignedXml.cs
- SystemIPInterfaceProperties.cs
- WebPartTransformerAttribute.cs
- MetafileHeaderWmf.cs
- XmlTextReaderImpl.cs
- XappLauncher.cs
- ConfigurationPropertyAttribute.cs
- QilGenerator.cs
- CommandValueSerializer.cs
- MultipleViewProviderWrapper.cs
- ObjectQuery.cs
- DesignTimeVisibleAttribute.cs
- ComplexBindingPropertiesAttribute.cs
- Int32Storage.cs
- AvTrace.cs
- StreamInfo.cs
- GlobalizationSection.cs
- CheckedListBox.cs
- ManipulationBoundaryFeedbackEventArgs.cs
- WebPartEditorOkVerb.cs
- HttpServerChannel.cs
- IconHelper.cs
- BitmapEffect.cs
- BridgeDataReader.cs
- CollectionViewGroupRoot.cs
- ExpressionEditorSheet.cs
- CancelRequestedRecord.cs
- ScriptingProfileServiceSection.cs
- PrefixQName.cs
- XmlSubtreeReader.cs
- HashRepartitionStream.cs
- SelectionRangeConverter.cs
- DeferrableContentConverter.cs
- ScrollBar.cs
- RoleService.cs
- ChannelSinkStacks.cs
- ContainsRowNumberChecker.cs
- GlyphInfoList.cs
- HandlerBase.cs
- OleCmdHelper.cs
- WebServiceBindingAttribute.cs
- FileInfo.cs
- CellQuery.cs
- RemotingSurrogateSelector.cs
- DataBoundControlHelper.cs
- Wrapper.cs
- Helpers.cs
- RenderDataDrawingContext.cs
- TypeConverterHelper.cs
- XmlSchemaSimpleTypeRestriction.cs
- XsltLoader.cs
- EntityContainerEmitter.cs
- EpmCustomContentWriterNodeData.cs
- PathSegmentCollection.cs
- SQLDoubleStorage.cs
- ExpressionsCollectionEditor.cs
- AccessViolationException.cs
- CodeChecksumPragma.cs
- JsonDeserializer.cs
- ToolboxBitmapAttribute.cs
- SQLMembershipProvider.cs
- PageThemeCodeDomTreeGenerator.cs
- UnsafeNativeMethods.cs
- FileSystemWatcher.cs
- ContainsSearchOperator.cs
- Pens.cs
- LicenseContext.cs
- ListDictionary.cs
- Overlapped.cs
- Int32Converter.cs
- WebControlToolBoxItem.cs
- ContainerUtilities.cs
- ToolStripItemRenderEventArgs.cs
- Util.cs
- SspiHelper.cs
- QueryContinueDragEvent.cs
- XmlName.cs
- EntityViewGenerationAttribute.cs
- DataGridViewSelectedCellCollection.cs
- Descriptor.cs
- ConstraintManager.cs
- ISO2022Encoding.cs
- DecimalConstantAttribute.cs
- AnnotationHelper.cs
- DataServicePagingProviderWrapper.cs
- MultiTrigger.cs
- ResolveInfo.cs
- DBSqlParser.cs