Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Epm / EpmCustomContentWriterNodeData.cs / 1305376 / EpmCustomContentWriterNodeData.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Data held by each node in the EpmTargetTree containing information used // by the EpmCustomContentWriter visitor // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { using System; using System.IO; using System.Xml; #if !ASTORIA_CLIENT using System.ServiceModel.Syndication; using System.Data.Services.Serializers; using System.Data.Services.Providers; #else using System.Data.Services.Client; #endif ////// Data held by each node in the EpmTargetTree containing information used by the /// EpmCustomContentWriter visitor /// internal sealed class EpmCustomContentWriterNodeData : IDisposable { ////// IDisposable helper state /// private bool disposed; #if ASTORIA_CLIENT ///Initializes the per node data for custom serializer /// Segment in target tree corresponding to this node /// Object from which to read properties internal EpmCustomContentWriterNodeData(EpmTargetPathSegment segment, object element) #else ///Initializes the per node data for custom serializer /// Segment in target tree corresponding to this node /// Object from which to read properties /// Null valued properties found during serialization /// Data Service provider used for rights verification. internal EpmCustomContentWriterNodeData(EpmTargetPathSegment segment, object element, EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties, DataServiceProviderWrapper provider) #endif { this.XmlContentStream = new MemoryStream(); XmlWriterSettings customContentWriterSettings = new XmlWriterSettings(); customContentWriterSettings.OmitXmlDeclaration = true; customContentWriterSettings.ConformanceLevel = ConformanceLevel.Fragment; this.XmlContentWriter = XmlWriter.Create(this.XmlContentStream, customContentWriterSettings); #if ASTORIA_CLIENT this.PopulateData(segment, element); #else this.PopulateData(segment, element, nullValuedProperties, provider); #endif } #if ASTORIA_CLIENT ///Initializes the per node data for custom serializer /// Parent node whose xml writer we are going to reuse /// Segment in target tree corresponding to this node /// Object from which to read properties internal EpmCustomContentWriterNodeData(EpmCustomContentWriterNodeData parentData, EpmTargetPathSegment segment, object element) #else ///Initializes the per node data for custom serializer /// Parent node whose xml writer we are going to reuse /// Segment in target tree corresponding to this node /// Object from which to read properties /// Null valued properties found during serialization /// Data Service provider used for rights verification. internal EpmCustomContentWriterNodeData(EpmCustomContentWriterNodeData parentData, EpmTargetPathSegment segment, object element, EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties, DataServiceProviderWrapper provider) #endif { this.XmlContentStream = parentData.XmlContentStream; this.XmlContentWriter = parentData.XmlContentWriter; #if ASTORIA_CLIENT this.PopulateData(segment, element); #else this.PopulateData(segment, element, nullValuedProperties, provider); #endif } ////// Memory stream on top of which XmlWriter works /// internal MemoryStream XmlContentStream { get; private set; } ////// Xml writer used for holding custom content fragment /// internal XmlWriter XmlContentWriter { get; private set; } ///Data for current node internal String Data { get; private set; } ////// Closes XmlWriter and disposes the MemoryStream /// public void Dispose() { if (!this.disposed) { if (this.XmlContentWriter != null) { this.XmlContentWriter.Close(); this.XmlContentWriter = null; } if (this.XmlContentStream != null) { this.XmlContentStream.Dispose(); this.XmlContentStream = null; } this.disposed = true; } } ////// Adds the content generated through custom serialization to the SyndicationItem or XmlWriter /// /// SyndicationItem or XmlWriter being serialized [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2000:Dispose objects before losing scope", Justification = "XmlReader on MemoryStream does not require disposal")] #if ASTORIA_CLIENT internal void AddContentToTarget(XmlWriter target) #else internal void AddContentToTarget(SyndicationItem target) #endif { #if ASTORIA_CLIENT Util.CheckArgumentNull(target, "target"); #else WebUtil.CheckArgumentNull(target, "target"); #endif this.XmlContentWriter.Close(); this.XmlContentWriter = null; this.XmlContentStream.Seek(0, SeekOrigin.Begin); XmlReaderSettings customContentReaderSettings = new XmlReaderSettings(); customContentReaderSettings.ConformanceLevel = ConformanceLevel.Fragment; XmlReader reader = XmlReader.Create(this.XmlContentStream, customContentReaderSettings); this.XmlContentStream = null; #if ASTORIA_CLIENT target.WriteNode(reader, false); #else target.ElementExtensions.Add(reader); #endif } #if ASTORIA_CLIENT ////// Populates the data value corresponding to this node, also updates the list of null attributes /// in the parent null attribute list if current node is attribute with null value /// /// Segment being populated /// Object whose property will be read private void PopulateData(EpmTargetPathSegment segment, object element) #else ////// Populates the data value corresponding to this node, also updates the list of null attributes /// in the parent null attribute list if current node is attribute with null value /// /// Segment being populated /// Object whose property will be read /// Null valued properties found during serialization /// Data Service provider used for rights verification. private void PopulateData(EpmTargetPathSegment segment, object element, EpmContentSerializer.EpmNullValuedPropertyTree nullValuedProperties, DataServiceProviderWrapper provider) #endif { if (segment.EpmInfo != null) { Object propertyValue; try { #if ASTORIA_CLIENT propertyValue = segment.EpmInfo.PropValReader.DynamicInvoke(element); #else propertyValue = segment.EpmInfo.PropValReader.DynamicInvoke(element, provider); #endif } catch #if ASTORIA_CLIENT (System.Reflection.TargetInvocationException) #else (System.Reflection.TargetInvocationException e) #endif { #if !ASTORIA_CLIENT ErrorHandler.HandleTargetInvocationException(e); #endif throw; } #if ASTORIA_CLIENT this.Data = propertyValue == null ? String.Empty : ClientConvert.ToString(propertyValue, false /* atomDateConstruct */); #else if (propertyValue == null || propertyValue == DBNull.Value) { this.Data = String.Empty; nullValuedProperties.Add(segment.EpmInfo); } else { this.Data = PlainXmlSerializer.PrimitiveToString(propertyValue); } #endif } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
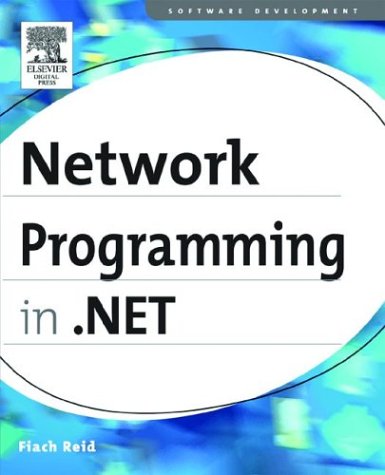
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ModuleConfigurationInfo.cs
- DefaultEvaluationContext.cs
- FocusChangedEventArgs.cs
- SelectionBorderGlyph.cs
- ListItemCollection.cs
- smtppermission.cs
- Vector3DAnimationBase.cs
- PackUriHelper.cs
- ToolStripDropDownClosedEventArgs.cs
- Mutex.cs
- HttpPostedFile.cs
- ButtonField.cs
- XmlSignatureManifest.cs
- FileFormatException.cs
- WindowAutomationPeer.cs
- HandleExceptionArgs.cs
- BuildProvider.cs
- InputLanguageManager.cs
- FixedHighlight.cs
- AnonymousIdentificationSection.cs
- UrlAuthorizationModule.cs
- ToolStripPanelCell.cs
- PageRequestManager.cs
- AnimationClockResource.cs
- Label.cs
- ListBindingHelper.cs
- ListDictionary.cs
- DataGridRowAutomationPeer.cs
- ReceiveActivity.cs
- ToolStripContainer.cs
- NameValuePair.cs
- DataGridViewCellParsingEventArgs.cs
- MsmqSecureHashAlgorithm.cs
- IntegerValidator.cs
- CodePageEncoding.cs
- ReadOnlyHierarchicalDataSourceView.cs
- Single.cs
- AttributeCollection.cs
- DynamicFilter.cs
- NavigationProgressEventArgs.cs
- CookielessHelper.cs
- MLangCodePageEncoding.cs
- CheckBoxField.cs
- WebPartMinimizeVerb.cs
- QueryFunctions.cs
- PropertyChangedEventManager.cs
- AspNetSynchronizationContext.cs
- MergablePropertyAttribute.cs
- LocalFileSettingsProvider.cs
- WebCategoryAttribute.cs
- AutomationElement.cs
- VerificationException.cs
- SmiMetaDataProperty.cs
- JournalNavigationScope.cs
- RefreshEventArgs.cs
- RectangleF.cs
- HttpRequest.cs
- WebPartCollection.cs
- ClientTarget.cs
- ButtonBase.cs
- AppLevelCompilationSectionCache.cs
- SqlMethods.cs
- MetaColumn.cs
- CookieParameter.cs
- CfgRule.cs
- InvokeFunc.cs
- HuffmanTree.cs
- InvokeAction.cs
- SizeConverter.cs
- RootNamespaceAttribute.cs
- ListControl.cs
- TextHidden.cs
- SqlServer2KCompatibilityAnnotation.cs
- CircleEase.cs
- ItemPager.cs
- TickBar.cs
- HierarchicalDataBoundControl.cs
- SoapCodeExporter.cs
- LeftCellWrapper.cs
- DoubleLinkListEnumerator.cs
- XhtmlConformanceSection.cs
- BindingMAnagerBase.cs
- NativeObjectSecurity.cs
- FormViewRow.cs
- Ipv6Element.cs
- FontInfo.cs
- BuildResult.cs
- SubpageParaClient.cs
- TextRangeEditLists.cs
- SecurityCriticalDataForSet.cs
- AssociationEndMember.cs
- UInt64.cs
- FormatVersion.cs
- AnnotationHelper.cs
- ContentIterators.cs
- PartBasedPackageProperties.cs
- ButtonChrome.cs
- PersistenceTypeAttribute.cs
- BitmapEffectInput.cs
- ControlBuilder.cs