Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / FixedHighlight.cs / 1 / FixedHighlight.cs
//---------------------------------------------------------------------------- //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // Description: // Implements FixedHighlight // // History: // 1/11/2004 - Zhenbin Xu (ZhenbinX) - Created. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal.Documents; using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows.Media; // Brush using System.Windows.Media.TextFormatting; // CharacterHit using System.Windows.Shapes; // Glyphs using System.Windows.Controls; // Image //===================================================================== ////// FixedHighlight represents partial glyph run that is highlighted on a fixed document. /// internal sealed class FixedHighlight { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors ////// Create a new FixedHighlight for a Glyphs with character offset of /// beginOffset to endOffset, to be rendered with a given brush. /// internal FixedHighlight(UIElement element, int beginOffset, int endOffset, FixedHighlightType t, Brush foreground, Brush background) { Debug.Assert(element != null && beginOffset >= 0 && endOffset >= 0); _element = element; _gBeginOffset = beginOffset; _gEndOffset = endOffset; _type = t; _foregroundBrush = foreground; _backgroundBrush = background; } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods ////// Compares 2 FixedHighlight /// /// the FixedHighlight to compare with ///true if this FixedHighlight is on the same element with the same offset, and brush override public bool Equals(object oCompare) { FixedHighlight fh = oCompare as FixedHighlight; if (fh == null) { return false; } return (fh._element == _element) && (fh._gBeginOffset == _gBeginOffset) && (fh._gEndOffset == _gEndOffset) && (fh._type == _type); } ////// Overloaded method from object /// ///Hash code public override int GetHashCode() { return _element == null ? 0 : _element.GetHashCode() + _gBeginOffset + _gEndOffset + (int)_type; } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- //-------------------------------------------------------------------- // // Public Events // //--------------------------------------------------------------------- //------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods // Compute the rectangle that covers this highlight internal Rect ComputeDesignRect() { Glyphs g = _element as Glyphs; if (g == null) { Image im = _element as Image; if (im != null && im.Source != null) { return new Rect(0, 0, im.Width, im.Height); } else { Path p = _element as Path; if (p != null) { return p.Data.Bounds; } } return Rect.Empty; } GlyphRun run = g.MeasurementGlyphRun; // g.ToGlyphRun(); if (run == null || _gBeginOffset >= _gEndOffset) { return Rect.Empty; } Rect designRect = run.ComputeAlignmentBox(); designRect.Offset(g.OriginX, g.OriginY); int chrct = (run.Characters == null ? 0 : run.Characters.Count); Debug.Assert(_gBeginOffset >= 0); Debug.Assert(_gEndOffset <= chrct); double x1, x2, width; x1 = run.GetDistanceFromCaretCharacterHit(new CharacterHit(_gBeginOffset, 0)); if (_gEndOffset == chrct) { x2 = run.GetDistanceFromCaretCharacterHit(new CharacterHit(chrct - 1, 1)); } else { x2 = run.GetDistanceFromCaretCharacterHit(new CharacterHit(_gEndOffset, 0)); } if (x2 < x1) { double temp = x1; x1 = x2; x2 = temp; } width = x2 - x1; if ((run.BidiLevel & 1) != 0) { // right to left designRect.X = g.OriginX - x2; } else { designRect.X = g.OriginX + x1; } designRect.Width = width; #if DEBUG DocumentsTrace.FixedTextOM.Highlight.Trace(string.Format("DesignBound {0}", designRect)); #endif return designRect; } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //--------------------------------------------------------------------- #region Internal Properties internal FixedHighlightType HighlightType { get { return _type; } } internal Glyphs Glyphs { get { return _element as Glyphs; } } internal UIElement Element { get { return _element; } } internal Brush ForegroundBrush { get { return _foregroundBrush; } } internal Brush BackgroundBrush { get { return _backgroundBrush; } } #endregion Internal Properties //-------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields private readonly UIElement _element; // the Glyphs element, or possibly an image private readonly int _gBeginOffset; // Begin character offset with Glyphs private readonly int _gEndOffset; // End character offset with Glyphs private readonly FixedHighlightType _type; // Type of highlight private readonly Brush _backgroundBrush; // highlight background brush private readonly Brush _foregroundBrush; // highlight foreground brush #endregion Private Fields } ////// Flags determine type of highlight /// internal enum FixedHighlightType { None = 0, TextSelection = 1, AnnotationHighlight = 2 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // Description: // Implements FixedHighlight // // History: // 1/11/2004 - Zhenbin Xu (ZhenbinX) - Created. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal.Documents; using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows.Media; // Brush using System.Windows.Media.TextFormatting; // CharacterHit using System.Windows.Shapes; // Glyphs using System.Windows.Controls; // Image //===================================================================== ////// FixedHighlight represents partial glyph run that is highlighted on a fixed document. /// internal sealed class FixedHighlight { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors ////// Create a new FixedHighlight for a Glyphs with character offset of /// beginOffset to endOffset, to be rendered with a given brush. /// internal FixedHighlight(UIElement element, int beginOffset, int endOffset, FixedHighlightType t, Brush foreground, Brush background) { Debug.Assert(element != null && beginOffset >= 0 && endOffset >= 0); _element = element; _gBeginOffset = beginOffset; _gEndOffset = endOffset; _type = t; _foregroundBrush = foreground; _backgroundBrush = background; } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods ////// Compares 2 FixedHighlight /// /// the FixedHighlight to compare with ///true if this FixedHighlight is on the same element with the same offset, and brush override public bool Equals(object oCompare) { FixedHighlight fh = oCompare as FixedHighlight; if (fh == null) { return false; } return (fh._element == _element) && (fh._gBeginOffset == _gBeginOffset) && (fh._gEndOffset == _gEndOffset) && (fh._type == _type); } ////// Overloaded method from object /// ///Hash code public override int GetHashCode() { return _element == null ? 0 : _element.GetHashCode() + _gBeginOffset + _gEndOffset + (int)_type; } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- //-------------------------------------------------------------------- // // Public Events // //--------------------------------------------------------------------- //------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods // Compute the rectangle that covers this highlight internal Rect ComputeDesignRect() { Glyphs g = _element as Glyphs; if (g == null) { Image im = _element as Image; if (im != null && im.Source != null) { return new Rect(0, 0, im.Width, im.Height); } else { Path p = _element as Path; if (p != null) { return p.Data.Bounds; } } return Rect.Empty; } GlyphRun run = g.MeasurementGlyphRun; // g.ToGlyphRun(); if (run == null || _gBeginOffset >= _gEndOffset) { return Rect.Empty; } Rect designRect = run.ComputeAlignmentBox(); designRect.Offset(g.OriginX, g.OriginY); int chrct = (run.Characters == null ? 0 : run.Characters.Count); Debug.Assert(_gBeginOffset >= 0); Debug.Assert(_gEndOffset <= chrct); double x1, x2, width; x1 = run.GetDistanceFromCaretCharacterHit(new CharacterHit(_gBeginOffset, 0)); if (_gEndOffset == chrct) { x2 = run.GetDistanceFromCaretCharacterHit(new CharacterHit(chrct - 1, 1)); } else { x2 = run.GetDistanceFromCaretCharacterHit(new CharacterHit(_gEndOffset, 0)); } if (x2 < x1) { double temp = x1; x1 = x2; x2 = temp; } width = x2 - x1; if ((run.BidiLevel & 1) != 0) { // right to left designRect.X = g.OriginX - x2; } else { designRect.X = g.OriginX + x1; } designRect.Width = width; #if DEBUG DocumentsTrace.FixedTextOM.Highlight.Trace(string.Format("DesignBound {0}", designRect)); #endif return designRect; } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //--------------------------------------------------------------------- #region Internal Properties internal FixedHighlightType HighlightType { get { return _type; } } internal Glyphs Glyphs { get { return _element as Glyphs; } } internal UIElement Element { get { return _element; } } internal Brush ForegroundBrush { get { return _foregroundBrush; } } internal Brush BackgroundBrush { get { return _backgroundBrush; } } #endregion Internal Properties //-------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields private readonly UIElement _element; // the Glyphs element, or possibly an image private readonly int _gBeginOffset; // Begin character offset with Glyphs private readonly int _gEndOffset; // End character offset with Glyphs private readonly FixedHighlightType _type; // Type of highlight private readonly Brush _backgroundBrush; // highlight background brush private readonly Brush _foregroundBrush; // highlight foreground brush #endregion Private Fields } ////// Flags determine type of highlight /// internal enum FixedHighlightType { None = 0, TextSelection = 1, AnnotationHighlight = 2 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
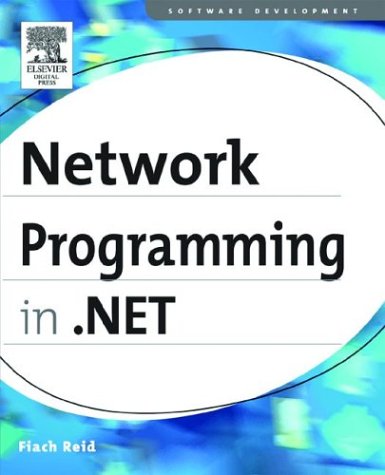
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DirectionalLight.cs
- LoginView.cs
- ConfigsHelper.cs
- DependencyObjectType.cs
- IntegerValidatorAttribute.cs
- ToolCreatedEventArgs.cs
- linebase.cs
- TimelineGroup.cs
- SlotInfo.cs
- BinaryFormatter.cs
- CurrentTimeZone.cs
- ContentFileHelper.cs
- DoubleAnimationUsingKeyFrames.cs
- ListViewEditEventArgs.cs
- Token.cs
- XPathNodePointer.cs
- SystemMulticastIPAddressInformation.cs
- ObjectItemAssemblyLoader.cs
- ExitEventArgs.cs
- RightsManagementManager.cs
- LineGeometry.cs
- JoinCqlBlock.cs
- LeaseManager.cs
- FigureParaClient.cs
- PageThemeParser.cs
- JsonByteArrayDataContract.cs
- SingleKeyFrameCollection.cs
- WorkflowTraceTransfer.cs
- DesignTimeTemplateParser.cs
- SecUtil.cs
- HtmlControl.cs
- DependencyPropertyDescriptor.cs
- CustomCredentialPolicy.cs
- DigitShape.cs
- ListViewHitTestInfo.cs
- ResourceExpression.cs
- InternalDuplexChannelFactory.cs
- Setter.cs
- KerberosTicketHashIdentifierClause.cs
- SQLString.cs
- XmlCharCheckingWriter.cs
- ConstantSlot.cs
- FormatConvertedBitmap.cs
- BitSet.cs
- AuthorizationContext.cs
- FormatterServices.cs
- GlobalProxySelection.cs
- HttpNamespaceReservationInstallComponent.cs
- ToolBarButtonClickEvent.cs
- SettingsPropertyValueCollection.cs
- Attribute.cs
- SymbolUsageManager.cs
- ConstraintEnumerator.cs
- __ConsoleStream.cs
- CapiSymmetricAlgorithm.cs
- HTMLTagNameToTypeMapper.cs
- ValidationError.cs
- ListViewInsertionMark.cs
- WebBrowserPermission.cs
- DecoderBestFitFallback.cs
- webeventbuffer.cs
- XmlUnspecifiedAttribute.cs
- WsdlImporter.cs
- ListBox.cs
- SvcMapFile.cs
- ColorAnimation.cs
- XNodeValidator.cs
- Base64Decoder.cs
- WorkflowService.cs
- UrlRoutingModule.cs
- ColorBlend.cs
- Version.cs
- UndirectedGraph.cs
- TypeDescriptorFilterService.cs
- ThreadPool.cs
- SecurityUtils.cs
- SiteMapProvider.cs
- VectorCollection.cs
- ShellProvider.cs
- __ConsoleStream.cs
- NullableFloatSumAggregationOperator.cs
- ClientRoleProvider.cs
- RouteUrlExpressionBuilder.cs
- HttpModuleActionCollection.cs
- FixedFlowMap.cs
- RadioButton.cs
- XmlAttributeProperties.cs
- AuthenticationModulesSection.cs
- NullableLongSumAggregationOperator.cs
- ObjectDataProvider.cs
- MessageOperationFormatter.cs
- ReceiveContent.cs
- ButtonBase.cs
- DataSourceSelectArguments.cs
- BinaryObjectInfo.cs
- DataGridViewRowPrePaintEventArgs.cs
- CacheDependency.cs
- KeyEventArgs.cs
- HtmlHead.cs
- QuaternionAnimationBase.cs