Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / InternalDuplexChannelFactory.cs / 1 / InternalDuplexChannelFactory.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel.Dispatcher; using System.Runtime.Serialization; using System.Threading; sealed class InternalDuplexChannelFactory : LayeredChannelFactory{ static long channelCount = 0; InputChannelDemuxer channelDemuxer; IChannelFactory innerChannelFactory; IChannelListener innerChannelListener; LocalAddressProvider localAddressProvider; bool providesCorrelation; internal InternalDuplexChannelFactory(InternalDuplexBindingElement bindingElement, BindingContext context, InputChannelDemuxer channelDemuxer, IChannelFactory innerChannelFactory, LocalAddressProvider localAddressProvider) : base(context.Binding, innerChannelFactory) { this.channelDemuxer = channelDemuxer; this.innerChannelFactory = innerChannelFactory; ChannelDemuxerFilter demuxFilter = new ChannelDemuxerFilter(new MatchNoneMessageFilter(), int.MinValue); this.innerChannelListener = this.channelDemuxer.BuildChannelListener (demuxFilter); this.localAddressProvider = localAddressProvider; this.providesCorrelation = bindingElement.ProvidesCorrelation; } void CreateUniqueLocalAddress(out EndpointAddress address, out int priority) { long tempChannelCount = Interlocked.Increment(ref channelCount); if (tempChannelCount > 1) { AddressHeader uniqueEndpointHeader = AddressHeader.CreateAddressHeader(XD.UtilityDictionary.UniqueEndpointHeaderName, XD.UtilityDictionary.UniqueEndpointHeaderNamespace, tempChannelCount); address = new EndpointAddress(this.innerChannelListener.Uri, uniqueEndpointHeader); priority = 1; } else { address = new EndpointAddress(this.innerChannelListener.Uri); priority = 0; } } protected override IDuplexChannel OnCreateChannel(EndpointAddress address, Uri via) { EndpointAddress localAddress; int priority; MessageFilter filter; if (localAddressProvider != null) { localAddress = localAddressProvider.LocalAddress; filter = localAddressProvider.Filter; priority = localAddressProvider.Priority; } else { CreateUniqueLocalAddress(out localAddress, out priority); filter = new MatchAllMessageFilter(); } return this.CreateChannel(address, via, localAddress, filter, priority); } public IDuplexChannel CreateChannel(EndpointAddress address, Uri via, MessageFilter filter, int priority) { return this.CreateChannel(address, via, new EndpointAddress(this.innerChannelListener.Uri), filter, priority); } public IDuplexChannel CreateChannel(EndpointAddress remoteAddress, Uri via, EndpointAddress localAddress, MessageFilter filter, int priority) { ChannelDemuxerFilter demuxFilter = new ChannelDemuxerFilter(new AndMessageFilter(new EndpointAddressMessageFilter(localAddress, true), filter), priority); IDuplexChannel newChannel = null; IOutputChannel innerOutputChannel = null; IChannelListener innerInputListener = null; IInputChannel innerInputChannel = null; try { innerOutputChannel = this.innerChannelFactory.CreateChannel(remoteAddress, via); innerInputListener = this.channelDemuxer.BuildChannelListener (demuxFilter); innerInputListener.Open(); innerInputChannel = innerInputListener.AcceptChannel(); newChannel = new ClientCompositeDuplexChannel(this, innerInputChannel, innerInputListener, localAddress, innerOutputChannel); } finally { if (newChannel == null) // need to cleanup { if (innerOutputChannel != null) { innerOutputChannel.Close(); } if (innerInputListener != null) { innerInputListener.Close(); } if (innerInputChannel != null) { innerInputChannel.Close(); } } } return newChannel; } protected override void OnAbort() { base.OnAbort(); this.innerChannelListener.Abort(); } protected override void OnOpen(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnOpen(timeoutHelper.RemainingTime()); this.innerChannelListener.Open(timeoutHelper.RemainingTime()); } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedOpenAsyncResult(timeout, callback, state, base.OnBeginOpen, base.OnEndOpen, this.innerChannelListener); } protected override void OnEndOpen(IAsyncResult result) { ChainedOpenAsyncResult.End(result); } protected override void OnClose(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnClose(timeoutHelper.RemainingTime()); this.innerChannelListener.Close(timeoutHelper.RemainingTime()); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedCloseAsyncResult(timeout, callback, state, base.OnBeginClose, base.OnEndClose, this.innerChannelListener); } protected override void OnEndClose(IAsyncResult result) { ChainedCloseAsyncResult.End(result); } public override T GetProperty () { if (typeof(T) == typeof(IChannelListener)) { return (T)(object)innerChannelListener; } if (typeof(T) == typeof(ISecurityCapabilities) && !this.providesCorrelation) { return InternalDuplexBindingElement.GetSecurityCapabilities (base.GetProperty ()); } T baseProperty = base.GetProperty (); if (baseProperty != null) { return baseProperty; } IChannelListener channelListener = innerChannelListener; if (channelListener != null) { return channelListener.GetProperty (); } else { return default(T); } } class ClientCompositeDuplexChannel : LayeredDuplexChannel { IChannelListener innerInputListener; public ClientCompositeDuplexChannel(ChannelManagerBase channelManager, IInputChannel innerInputChannel, IChannelListener innerInputListener, EndpointAddress localAddress, IOutputChannel innerOutputChannel) : base(channelManager, innerInputChannel, localAddress, innerOutputChannel) { this.innerInputListener = innerInputListener; } protected override void OnAbort() { base.OnAbort(); this.innerInputListener.Abort(); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedAsyncResult(timeout, callback, state, base.OnBeginClose, base.OnEndClose, this.innerInputListener.BeginClose, this.innerInputListener.EndClose); } protected override void OnClose(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnClose(timeoutHelper.RemainingTime()); this.innerInputListener.Close(timeoutHelper.RemainingTime()); } protected override void OnEndClose(IAsyncResult result) { ChainedAsyncResult.End(result); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
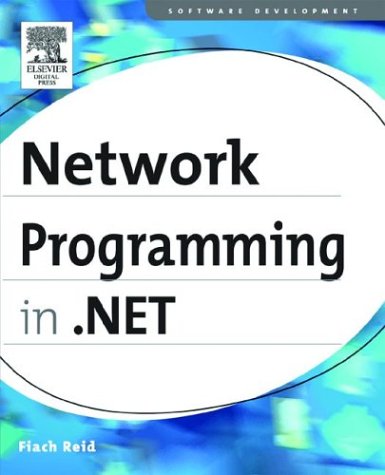
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _SafeNetHandles.cs
- PaperSource.cs
- CoTaskMemHandle.cs
- DiscoveryDocument.cs
- SimpleLine.cs
- ResourcesGenerator.cs
- InstanceData.cs
- AssociationTypeEmitter.cs
- _ChunkParse.cs
- SqlDataReader.cs
- BitmapEffectInput.cs
- ValuePattern.cs
- ResourceCategoryAttribute.cs
- MaterialCollection.cs
- Socket.cs
- InvalidOleVariantTypeException.cs
- Int32.cs
- BaseResourcesBuildProvider.cs
- AlternationConverter.cs
- HttpProtocolImporter.cs
- WebBrowser.cs
- PreProcessInputEventArgs.cs
- baseshape.cs
- ReferenceConverter.cs
- TrayIconDesigner.cs
- RemotingConfigParser.cs
- ResourcesGenerator.cs
- QueryCacheEntry.cs
- AppDomainUnloadedException.cs
- SafeReadContext.cs
- RowParagraph.cs
- Types.cs
- HttpRequestBase.cs
- ValidationErrorCollection.cs
- ThreadStartException.cs
- ReachSerializableProperties.cs
- TemplateXamlTreeBuilder.cs
- WindowsGraphics2.cs
- AssemblyInfo.cs
- DataKeyCollection.cs
- TextElementEnumerator.cs
- XmlSerializerVersionAttribute.cs
- SafeNativeMethods.cs
- COAUTHIDENTITY.cs
- Html32TextWriter.cs
- CodeDOMProvider.cs
- ConvertersCollection.cs
- TabControl.cs
- TraceInternal.cs
- DataGridHeaderBorder.cs
- TextSelectionHelper.cs
- TCPClient.cs
- MobileCategoryAttribute.cs
- DockEditor.cs
- DecodeHelper.cs
- EntityTemplateFactory.cs
- SafeFindHandle.cs
- TextServicesCompartmentEventSink.cs
- RecordConverter.cs
- ErrorFormatterPage.cs
- SrgsElementFactoryCompiler.cs
- _Connection.cs
- Schema.cs
- HostingEnvironmentException.cs
- CommandConverter.cs
- GridLengthConverter.cs
- MediaContext.cs
- DataError.cs
- NativeMethods.cs
- _PooledStream.cs
- TransformDescriptor.cs
- PropertyDescriptor.cs
- ConstrainedDataObject.cs
- TableLayoutRowStyleCollection.cs
- TextParagraphCache.cs
- WebPartUtil.cs
- dataobject.cs
- AuthenticatingEventArgs.cs
- TerminatorSinks.cs
- MediaCommands.cs
- ConnectionInterfaceCollection.cs
- TransformProviderWrapper.cs
- SudsCommon.cs
- FlowDocumentReader.cs
- SQLMembershipProvider.cs
- TextBlock.cs
- ParseNumbers.cs
- DrawingCollection.cs
- cookie.cs
- SpAudioStreamWrapper.cs
- TypeLibConverter.cs
- NTAccount.cs
- ReadOnlyPropertyMetadata.cs
- CreateUserWizard.cs
- PatternMatcher.cs
- TCPListener.cs
- ImageAnimator.cs
- ArgumentException.cs
- ExtendedTransformFactory.cs
- MimeTypePropertyAttribute.cs