Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ListViewInsertionMark.cs / 1 / ListViewInsertionMark.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Drawing; using System.Runtime.InteropServices; using System.Diagnostics; namespace System.Windows.Forms { ////// /// public sealed class ListViewInsertionMark { private ListView listView; private int index = 0; private Color color = Color.Empty; private bool appearsAfterItem = false; internal ListViewInsertionMark(ListView listView) { this.listView = listView; } ////// Encapsulates insertion-mark information /// ////// /// Specifies whether the insertion mark appears /// after the item - otherwise it appears /// before the item (the default). /// /// public bool AppearsAfterItem { get { return appearsAfterItem; } set { if (appearsAfterItem != value) { appearsAfterItem = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Returns bounds of the insertion-mark. /// /// public Rectangle Bounds { get { NativeMethods.RECT rect = new NativeMethods.RECT(); listView.SendMessage(NativeMethods.LVM_GETINSERTMARKRECT, 0, ref rect); return Rectangle.FromLTRB(rect.left, rect.top, rect.right, rect.bottom); } } ////// /// The color of the insertion-mark. /// /// public Color Color { get { if (color.IsEmpty) { color = SafeNativeMethods.ColorFromCOLORREF((int)listView.SendMessage(NativeMethods.LVM_GETINSERTMARKCOLOR, 0, 0)); } return color; } set { if (color != value) { color = value; if (listView.IsHandleCreated) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } ////// /// Item next to which the insertion-mark appears. /// /// public int Index { get { return index; } set { if (index != value) { index = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Performs a hit-test at the specified insertion point /// and returns the closest item. /// /// public int NearestIndex(Point pt) { NativeMethods.POINT point = new NativeMethods.POINT(); point.x = pt.X; point.y = pt.Y; NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_INSERTMARKHITTEST, point, lvInsertMark); return lvInsertMark.iItem; } internal void UpdateListView() { Debug.Assert(listView.IsHandleCreated, "ApplySavedState Precondition: List-view handle must be created"); NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); lvInsertMark.dwFlags = appearsAfterItem ? NativeMethods.LVIM_AFTER : 0; lvInsertMark.iItem = index; UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_SETINSERTMARK, 0, lvInsertMark); if (!color.IsEmpty) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
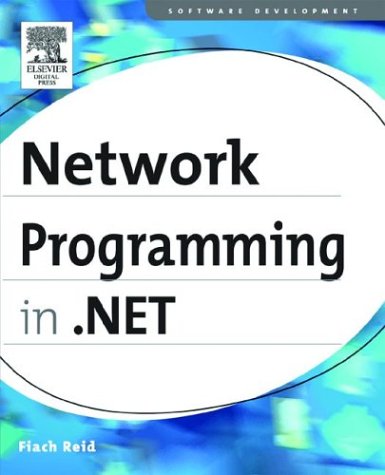
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmiEventSink_DeferedProcessing.cs
- IEnumerable.cs
- DetailsViewRowCollection.cs
- Encoder.cs
- XmlSchemaExporter.cs
- OdbcEnvironmentHandle.cs
- NullableIntSumAggregationOperator.cs
- DockAndAnchorLayout.cs
- DataGridViewColumnCollectionEditor.cs
- OracleRowUpdatedEventArgs.cs
- ChannelSinkStacks.cs
- ResourceAttributes.cs
- ProtocolsConfiguration.cs
- ArcSegment.cs
- LingerOption.cs
- SmiTypedGetterSetter.cs
- FamilyMapCollection.cs
- PreparingEnlistment.cs
- PageCache.cs
- XmlSchemaValidator.cs
- UInt32Storage.cs
- DiagnosticTrace.cs
- BuildManagerHost.cs
- BidPrivateBase.cs
- DLinqAssociationProvider.cs
- TagPrefixAttribute.cs
- AnimatedTypeHelpers.cs
- SiteMapDataSourceDesigner.cs
- XmlWellformedWriter.cs
- SchemaImporterExtensionElement.cs
- KnownTypeDataContractResolver.cs
- ChannelDispatcherCollection.cs
- EdmType.cs
- ComboBox.cs
- PageRequestManager.cs
- ClientSettingsSection.cs
- RectangleConverter.cs
- TabItem.cs
- CompositeDataBoundControl.cs
- ActivityExecutorSurrogate.cs
- FunctionDescription.cs
- TransformGroup.cs
- LogRecordSequence.cs
- ExpressionNode.cs
- ButtonFieldBase.cs
- UnsafeNativeMethods.cs
- CopyOnWriteList.cs
- CaseInsensitiveHashCodeProvider.cs
- IMembershipProvider.cs
- GlyphCache.cs
- IdentityReference.cs
- SQLMembershipProvider.cs
- FamilyCollection.cs
- IntranetCredentialPolicy.cs
- PixelShader.cs
- FullTextBreakpoint.cs
- EntityContainerAssociationSet.cs
- SelectionWordBreaker.cs
- MiniCustomAttributeInfo.cs
- PassportIdentity.cs
- StringReader.cs
- ServiceNameElementCollection.cs
- LayoutTableCell.cs
- NullReferenceException.cs
- CalculatedColumn.cs
- AttributeConverter.cs
- CultureInfoConverter.cs
- SqlClientWrapperSmiStreamChars.cs
- EtwTrackingParticipant.cs
- PersonalizationDictionary.cs
- DependencyObjectType.cs
- RequestUriProcessor.cs
- SingleSelectRootGridEntry.cs
- XmlSchemaAny.cs
- EntityConnectionStringBuilder.cs
- Decorator.cs
- ReadOnlyDictionary.cs
- UpdateTranslator.cs
- ProcessModuleCollection.cs
- EventLog.cs
- XmlSchemaImport.cs
- LingerOption.cs
- Keyboard.cs
- OLEDB_Enum.cs
- _NegoState.cs
- VisualProxy.cs
- WinEventQueueItem.cs
- ActivityXRefPropertyEditor.cs
- ObjectConverter.cs
- ExpanderAutomationPeer.cs
- shaperfactory.cs
- CodeStatementCollection.cs
- AllMembershipCondition.cs
- FragmentQuery.cs
- QEncodedStream.cs
- UndoUnit.cs
- BamlResourceContent.cs
- Light.cs
- DataGridViewTextBoxColumn.cs
- Process.cs