Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Tracking / EtwTrackingParticipant.cs / 1305376 / EtwTrackingParticipant.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Tracking { using System; using System.Activities; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Globalization; using System.IO; using System.Runtime; using System.Runtime.Diagnostics; using System.Runtime.Serialization.Formatters.Binary; using System.Text; using System.Runtime.Serialization; using System.Security; using System.Diagnostics.CodeAnalysis; using System.Xml; public sealed class EtwTrackingParticipant : TrackingParticipant { const string truncatedItemsTag = "... "; const string emptyItemsTag = ""; const string itemsTag = "items"; const string itemTag = "item"; const string nameAttribute = "name"; const string typeAttribute = "type"; static Hashtable diagnosticTraceCache = new Hashtable(); NetDataContractSerializer variableSerializer; DiagnosticTrace diagnosticTrace; Guid etwProviderId; public EtwTrackingParticipant() { this.EtwProviderId = DiagnosticTrace.DefaultEtwProviderId; this.ApplicationReference = string.Empty; } [Fx.Tag.KnownXamlExternal] public Guid EtwProviderId { get { return this.etwProviderId; } set { if (value == Guid.Empty) { throw FxTrace.Exception.ArgumentNullOrEmpty("value"); } InitializeEtwTrackingProvider(value); } } public string ApplicationReference { get; set; } protected internal override IAsyncResult BeginTrack(TrackingRecord record, TimeSpan timeout, AsyncCallback callback, object state) { Track(record, timeout); return new CompletedAsyncResult(callback, state); } protected internal override void EndTrack(IAsyncResult result) { CompletedAsyncResult.End(result); } protected internal override void Track(TrackingRecord record, TimeSpan timeout) { if (this.diagnosticTrace.IsEtwProviderEnabled) { if (record is ActivityStateRecord) { TrackActivityRecord((ActivityStateRecord)record); } else if (record is WorkflowInstanceRecord) { TrackWorkflowRecord((WorkflowInstanceRecord)record); } else if (record is BookmarkResumptionRecord) { TrackBookmarkRecord((BookmarkResumptionRecord)record); } else if (record is ActivityScheduledRecord) { TrackActivityScheduledRecord((ActivityScheduledRecord)record); } else if (record is CancelRequestedRecord) { TrackCancelRequestedRecord((CancelRequestedRecord)record); } else if (record is FaultPropagationRecord) { TrackFaultPropagationRecord((FaultPropagationRecord)record); } else { Fx.Assert(record is CustomTrackingRecord, "Expected only CustomTrackingRecord"); TrackCustomRecord((CustomTrackingRecord)record); } } } void InitializeEtwTrackingProvider(Guid providerId) { this.diagnosticTrace = (DiagnosticTrace)diagnosticTraceCache[providerId]; if (this.diagnosticTrace == null) { lock (diagnosticTraceCache) { this.diagnosticTrace = (DiagnosticTrace)diagnosticTraceCache[providerId]; if (this.diagnosticTrace == null) { this.diagnosticTrace = new DiagnosticTrace(null, providerId); diagnosticTraceCache.Add(providerId, this.diagnosticTrace); } } } this.etwProviderId = providerId; } string PrepareDictionary(IDictionary data) { StringBuilder builder = new StringBuilder(); XmlWriterSettings settings = new XmlWriterSettings() { OmitXmlDeclaration = true }; using (XmlWriter writer = XmlWriter.Create(builder, settings)) { writer.WriteStartElement(itemsTag); if (data != null) { foreach (KeyValuePair item in data) { writer.WriteStartElement(itemTag); writer.WriteAttributeString(nameAttribute, item.Key); if (item.Value == null) { writer.WriteAttributeString(typeAttribute, string.Empty); writer.WriteValue(string.Empty); } else { Type valueType = item.Value.GetType(); writer.WriteAttributeString(typeAttribute, valueType.FullName); if (valueType == typeof(int) || valueType == typeof(float) || valueType == typeof(double) || valueType == typeof(long) || valueType == typeof(bool) || valueType == typeof(uint) || valueType == typeof(ushort) || valueType == typeof(short) || valueType == typeof(ulong) || valueType == typeof(string) || valueType == typeof(DateTimeOffset)) { writer.WriteValue(item.Value); } else if (valueType == typeof(Guid)) { Guid value = (Guid)item.Value; writer.WriteValue(value.ToString()); } else if (valueType == typeof(DateTime)) { DateTime date = ((DateTime)item.Value).ToUniversalTime(); writer.WriteValue(date); } else { if (this.variableSerializer == null) { this.variableSerializer = new NetDataContractSerializer(); } try { this.variableSerializer.WriteObject(writer, item.Value); } catch (Exception e) { if (Fx.IsFatal(e)) { throw; } TraceItemNotSerializable(item.Key, e); } } } writer.WriteEndElement(); } } writer.WriteEndElement(); writer.Flush(); return builder.ToString(); } } static string PrepareAnnotations(IDictionary data) { string stringTypeName = typeof(string).FullName; StringBuilder builder = new StringBuilder(); XmlWriterSettings settings = new XmlWriterSettings() { OmitXmlDeclaration = true }; using (XmlWriter writer = XmlWriter.Create(builder, settings)) { writer.WriteStartElement(itemsTag); if (data != null) { foreach (KeyValuePair item in data) { writer.WriteStartElement(itemTag); writer.WriteAttributeString(nameAttribute, item.Key); writer.WriteAttributeString(typeAttribute, stringTypeName); if (item.Value == null) { writer.WriteValue(string.Empty); } else { writer.WriteValue(item.Value); } writer.WriteEndElement(); } } writer.WriteEndElement(); writer.Flush(); return builder.ToString(); } } static void TraceItemNotSerializable(string item, Exception e) { //trace the exception. FxTrace.Exception.AsInformation(e); if (TD.TrackingValueNotSerializableIsEnabled()) { TD.TrackingValueNotSerializable(item); } } void TraceTrackingRecordDropped(long recordNumber) { if (TD.TrackingRecordDroppedIsEnabled()) { TD.TrackingRecordDropped(recordNumber, this.EtwProviderId); } } void TraceTrackingRecordTruncated(long recordNumber) { if (TD.TrackingRecordTruncatedIsEnabled()) { TD.TrackingRecordTruncated(recordNumber, this.EtwProviderId); } } void TrackActivityRecord(ActivityStateRecord record) { if (EtwTrackingParticipantTrackRecords.ActivityStateRecordIsEnabled(this.diagnosticTrace)) { if (!EtwTrackingParticipantTrackRecords.ActivityStateRecord(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.State, record.Activity.Name, record.Activity.Id, record.Activity.InstanceId, record.Activity.TypeName, record.Arguments.Count > 0 ? PrepareDictionary(record.Arguments) : emptyItemsTag, record.Variables.Count > 0 ? PrepareDictionary(record.Variables) : emptyItemsTag, record.HasAnnotations ? PrepareAnnotations(record.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.ActivityStateRecord(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.State, record.Activity.Name, record.Activity.Id, record.Activity.InstanceId, record.Activity.TypeName, truncatedItemsTag, truncatedItemsTag, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(record.RecordNumber); } else { TraceTrackingRecordDropped(record.RecordNumber); } } } } void TrackActivityScheduledRecord(ActivityScheduledRecord scheduledRecord) { if (EtwTrackingParticipantTrackRecords.ActivityScheduledRecordIsEnabled(this.diagnosticTrace)) { if (!EtwTrackingParticipantTrackRecords.ActivityScheduledRecord(this.diagnosticTrace, scheduledRecord.InstanceId, scheduledRecord.RecordNumber, scheduledRecord.EventTime.ToFileTime(), scheduledRecord.Activity == null ? string.Empty : scheduledRecord.Activity.Name, scheduledRecord.Activity == null ? string.Empty : scheduledRecord.Activity.Id, scheduledRecord.Activity == null ? string.Empty : scheduledRecord.Activity.InstanceId, scheduledRecord.Activity == null ? string.Empty : scheduledRecord.Activity.TypeName, scheduledRecord.Child.Name, scheduledRecord.Child.Id, scheduledRecord.Child.InstanceId, scheduledRecord.Child.TypeName, scheduledRecord.HasAnnotations ? PrepareAnnotations(scheduledRecord.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.ActivityScheduledRecord(this.diagnosticTrace, scheduledRecord.InstanceId, scheduledRecord.RecordNumber, scheduledRecord.EventTime.ToFileTime(), scheduledRecord.Activity == null ? string.Empty : scheduledRecord.Activity.Name, scheduledRecord.Activity == null ? string.Empty : scheduledRecord.Activity.Id, scheduledRecord.Activity == null ? string.Empty : scheduledRecord.Activity.InstanceId, scheduledRecord.Activity == null ? string.Empty : scheduledRecord.Activity.TypeName, scheduledRecord.Child.Name, scheduledRecord.Child.Id, scheduledRecord.Child.InstanceId, scheduledRecord.Child.TypeName, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(scheduledRecord.RecordNumber); } else { TraceTrackingRecordDropped(scheduledRecord.RecordNumber); } } } } void TrackCancelRequestedRecord(CancelRequestedRecord cancelRecord) { if (EtwTrackingParticipantTrackRecords.CancelRequestedRecordIsEnabled(this.diagnosticTrace)) { if (!EtwTrackingParticipantTrackRecords.CancelRequestedRecord(this.diagnosticTrace, cancelRecord.InstanceId, cancelRecord.RecordNumber, cancelRecord.EventTime.ToFileTime(), cancelRecord.Activity == null ? string.Empty : cancelRecord.Activity.Name, cancelRecord.Activity == null ? string.Empty : cancelRecord.Activity.Id, cancelRecord.Activity == null ? string.Empty : cancelRecord.Activity.InstanceId, cancelRecord.Activity == null ? string.Empty : cancelRecord.Activity.TypeName, cancelRecord.Child.Name, cancelRecord.Child.Id, cancelRecord.Child.InstanceId, cancelRecord.Child.TypeName, cancelRecord.HasAnnotations ? PrepareAnnotations(cancelRecord.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.CancelRequestedRecord(this.diagnosticTrace, cancelRecord.InstanceId, cancelRecord.RecordNumber, cancelRecord.EventTime.ToFileTime(), cancelRecord.Activity == null ? string.Empty : cancelRecord.Activity.Name, cancelRecord.Activity == null ? string.Empty : cancelRecord.Activity.Id, cancelRecord.Activity == null ? string.Empty : cancelRecord.Activity.InstanceId, cancelRecord.Activity == null ? string.Empty : cancelRecord.Activity.TypeName, cancelRecord.Child.Name, cancelRecord.Child.Id, cancelRecord.Child.InstanceId, cancelRecord.Child.TypeName, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(cancelRecord.RecordNumber); } else { TraceTrackingRecordDropped(cancelRecord.RecordNumber); } } } } void TrackFaultPropagationRecord(FaultPropagationRecord faultRecord) { if (EtwTrackingParticipantTrackRecords.FaultPropagationRecordIsEnabled(this.diagnosticTrace)) { if (!EtwTrackingParticipantTrackRecords.FaultPropagationRecord(this.diagnosticTrace, faultRecord.InstanceId, faultRecord.RecordNumber, faultRecord.EventTime.ToFileTime(), faultRecord.FaultSource.Name, faultRecord.FaultSource.Id, faultRecord.FaultSource.InstanceId, faultRecord.FaultSource.TypeName, faultRecord.FaultHandler != null ? faultRecord.FaultHandler.Name : string.Empty, faultRecord.FaultHandler != null ? faultRecord.FaultHandler.Id : string.Empty, faultRecord.FaultHandler != null ? faultRecord.FaultHandler.InstanceId : string.Empty, faultRecord.FaultHandler != null ? faultRecord.FaultHandler.TypeName : string.Empty, faultRecord.Fault.ToString(), faultRecord.IsFaultSource, faultRecord.HasAnnotations ? PrepareAnnotations(faultRecord.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.FaultPropagationRecord(this.diagnosticTrace, faultRecord.InstanceId, faultRecord.RecordNumber, faultRecord.EventTime.ToFileTime(), faultRecord.FaultSource.Name, faultRecord.FaultSource.Id, faultRecord.FaultSource.InstanceId, faultRecord.FaultSource.TypeName, faultRecord.FaultHandler != null ? faultRecord.FaultHandler.Name : string.Empty, faultRecord.FaultHandler != null ? faultRecord.FaultHandler.Id : string.Empty, faultRecord.FaultHandler != null ? faultRecord.FaultHandler.InstanceId : string.Empty, faultRecord.FaultHandler != null ? faultRecord.FaultHandler.TypeName : string.Empty, faultRecord.Fault.ToString(), faultRecord.IsFaultSource, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(faultRecord.RecordNumber); } else { TraceTrackingRecordDropped(faultRecord.RecordNumber); } } } } void TrackBookmarkRecord(BookmarkResumptionRecord record) { if (EtwTrackingParticipantTrackRecords.BookmarkResumptionRecordIsEnabled(this.diagnosticTrace)) { if (!EtwTrackingParticipantTrackRecords.BookmarkResumptionRecord(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.BookmarkName, record.BookmarkScope, record.Owner.Name, record.Owner.Id, record.Owner.InstanceId, record.Owner.TypeName, record.HasAnnotations ? PrepareAnnotations(record.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.BookmarkResumptionRecord(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.BookmarkName, record.BookmarkScope, record.Owner.Name, record.Owner.Id, record.Owner.InstanceId, record.Owner.TypeName, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(record.RecordNumber); } else { TraceTrackingRecordDropped(record.RecordNumber); } } } } void TrackCustomRecord(CustomTrackingRecord record) { switch (record.Level) { case TraceLevel.Error: if (EtwTrackingParticipantTrackRecords.CustomTrackingRecordErrorIsEnabled(this.diagnosticTrace)) { if (!EtwTrackingParticipantTrackRecords.CustomTrackingRecordError(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.Name, record.Activity.Name, record.Activity.Id, record.Activity.InstanceId, record.Activity.TypeName, PrepareDictionary(record.Data), record.HasAnnotations ? PrepareAnnotations(record.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.CustomTrackingRecordError(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.Name, record.Activity.Name, record.Activity.Id, record.Activity.InstanceId, record.Activity.TypeName, truncatedItemsTag, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(record.RecordNumber); } else { TraceTrackingRecordDropped(record.RecordNumber); } } } break; case TraceLevel.Warning: if (EtwTrackingParticipantTrackRecords.CustomTrackingRecordWarningIsEnabled(this.diagnosticTrace)) { if (!EtwTrackingParticipantTrackRecords.CustomTrackingRecordWarning(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.Name, record.Activity.Name, record.Activity.Id, record.Activity.InstanceId, record.Activity.TypeName, PrepareDictionary(record.Data), record.HasAnnotations ? PrepareAnnotations(record.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.CustomTrackingRecordWarning(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.Name, record.Activity.Name, record.Activity.Id, record.Activity.InstanceId, record.Activity.TypeName, truncatedItemsTag, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(record.RecordNumber); } else { TraceTrackingRecordDropped(record.RecordNumber); } } } break; default: if (EtwTrackingParticipantTrackRecords.CustomTrackingRecordInfoIsEnabled(this.diagnosticTrace)) { if (!EtwTrackingParticipantTrackRecords.CustomTrackingRecordInfo(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.Name, record.Activity.Name, record.Activity.Id, record.Activity.InstanceId, record.Activity.TypeName, PrepareDictionary(record.Data), record.HasAnnotations ? PrepareAnnotations(record.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.CustomTrackingRecordInfo(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.Name, record.Activity.Name, record.Activity.Id, record.Activity.InstanceId, record.Activity.TypeName, truncatedItemsTag, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(record.RecordNumber); } else { TraceTrackingRecordDropped(record.RecordNumber); } } } break; } } void TrackWorkflowRecord(WorkflowInstanceRecord record) { if (record is WorkflowInstanceUnhandledExceptionRecord) { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceUnhandledExceptionRecordIsEnabled(this.diagnosticTrace)) { WorkflowInstanceUnhandledExceptionRecord unhandled = record as WorkflowInstanceUnhandledExceptionRecord; if (!EtwTrackingParticipantTrackRecords.WorkflowInstanceUnhandledExceptionRecord(this.diagnosticTrace, unhandled.InstanceId, unhandled.RecordNumber, unhandled.EventTime.ToFileTime(),unhandled.ActivityDefinitionId, unhandled.FaultSource.Name, unhandled.FaultSource.Id, unhandled.FaultSource.InstanceId, unhandled.FaultSource.TypeName, unhandled.UnhandledException == null ? string.Empty : unhandled.UnhandledException.ToString(), unhandled.HasAnnotations ? PrepareAnnotations(unhandled.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceUnhandledExceptionRecord(this.diagnosticTrace, unhandled.InstanceId, unhandled.RecordNumber, unhandled.EventTime.ToFileTime(), unhandled.ActivityDefinitionId, unhandled.FaultSource.Name, unhandled.FaultSource.Id, unhandled.FaultSource.InstanceId, unhandled.FaultSource.TypeName, unhandled.UnhandledException == null ? string.Empty : unhandled.UnhandledException.ToString(), truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(unhandled.RecordNumber); } else { TraceTrackingRecordDropped(unhandled.RecordNumber); } } } } else if (record is WorkflowInstanceAbortedRecord) { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceAbortedRecordIsEnabled(this.diagnosticTrace)) { WorkflowInstanceAbortedRecord aborted = record as WorkflowInstanceAbortedRecord; if (!EtwTrackingParticipantTrackRecords.WorkflowInstanceAbortedRecord(this.diagnosticTrace, aborted.InstanceId, aborted.RecordNumber, aborted.EventTime.ToFileTime(), aborted.ActivityDefinitionId, aborted.Reason, aborted.HasAnnotations ? PrepareAnnotations(aborted.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceAbortedRecord(this.diagnosticTrace, aborted.InstanceId, aborted.RecordNumber, aborted.EventTime.ToFileTime(), aborted.ActivityDefinitionId, aborted.Reason, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(aborted.RecordNumber); } else { TraceTrackingRecordDropped(aborted.RecordNumber); } } } } else if (record is WorkflowInstanceSuspendedRecord) { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceSuspendedRecordIsEnabled(this.diagnosticTrace)) { WorkflowInstanceSuspendedRecord suspended = record as WorkflowInstanceSuspendedRecord; if (!EtwTrackingParticipantTrackRecords.WorkflowInstanceSuspendedRecord(this.diagnosticTrace, suspended.InstanceId, suspended.RecordNumber, suspended.EventTime.ToFileTime(), suspended.ActivityDefinitionId, suspended.Reason, suspended.HasAnnotations ? PrepareAnnotations(suspended.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceSuspendedRecord(this.diagnosticTrace, suspended.InstanceId, suspended.RecordNumber, suspended.EventTime.ToFileTime(), suspended.ActivityDefinitionId, suspended.Reason, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(suspended.RecordNumber); } else { TraceTrackingRecordDropped(suspended.RecordNumber); } } } } else if (record is WorkflowInstanceTerminatedRecord) { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceTerminatedRecordIsEnabled(this.diagnosticTrace)) { WorkflowInstanceTerminatedRecord terminated = record as WorkflowInstanceTerminatedRecord; if (!EtwTrackingParticipantTrackRecords.WorkflowInstanceTerminatedRecord(this.diagnosticTrace, terminated.InstanceId, terminated.RecordNumber, terminated.EventTime.ToFileTime(), terminated.ActivityDefinitionId, terminated.Reason, terminated.HasAnnotations ? PrepareAnnotations(terminated.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceTerminatedRecord(this.diagnosticTrace, terminated.InstanceId, terminated.RecordNumber, terminated.EventTime.ToFileTime(), terminated.ActivityDefinitionId, terminated.Reason, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(terminated.RecordNumber); } else { TraceTrackingRecordDropped(terminated.RecordNumber); } } } } else { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceRecordIsEnabled(this.diagnosticTrace)) { if (!EtwTrackingParticipantTrackRecords.WorkflowInstanceRecord(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.ActivityDefinitionId, record.State, record.HasAnnotations ? PrepareAnnotations(record.Annotations) : emptyItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { if (EtwTrackingParticipantTrackRecords.WorkflowInstanceRecord(this.diagnosticTrace, record.InstanceId, record.RecordNumber, record.EventTime.ToFileTime(), record.ActivityDefinitionId, record.State, truncatedItemsTag, this.TrackingProfile == null ? string.Empty : this.TrackingProfile.Name, this.ApplicationReference)) { TraceTrackingRecordTruncated(record.RecordNumber); } else { TraceTrackingRecordDropped(record.RecordNumber); } } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
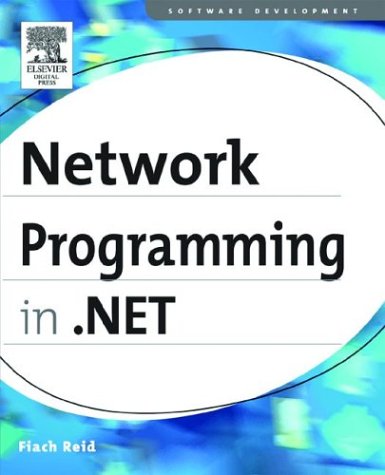
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SparseMemoryStream.cs
- TraceContextEventArgs.cs
- FileIOPermission.cs
- IfJoinedCondition.cs
- AdapterDictionary.cs
- SoapSchemaImporter.cs
- ContentTextAutomationPeer.cs
- KeyInstance.cs
- PackWebRequest.cs
- TabPanel.cs
- FieldNameLookup.cs
- XmlElementList.cs
- ToolStripItem.cs
- GC.cs
- BindToObject.cs
- followingquery.cs
- NameValueSectionHandler.cs
- SizeChangedInfo.cs
- TextureBrush.cs
- ObjectListGeneralPage.cs
- InkCanvasSelection.cs
- ValueType.cs
- MonthCalendar.cs
- FragmentNavigationEventArgs.cs
- HttpGetProtocolImporter.cs
- CounterCreationData.cs
- IdentityManager.cs
- ConstraintStruct.cs
- SoapSchemaExporter.cs
- PatternMatcher.cs
- IfElseDesigner.xaml.cs
- Internal.cs
- MimePart.cs
- ProfilePropertySettingsCollection.cs
- WizardStepBase.cs
- FrameworkElement.cs
- SignatureHelper.cs
- RowCache.cs
- ProviderCollection.cs
- FacetDescription.cs
- DataGridViewTextBoxColumn.cs
- ActivityCodeDomSerializationManager.cs
- MessageAction.cs
- QueryResults.cs
- FixUp.cs
- FileLogRecordStream.cs
- Rotation3DAnimationBase.cs
- InstanceNameConverter.cs
- SByteConverter.cs
- TextTreeTextElementNode.cs
- XamlFigureLengthSerializer.cs
- Switch.cs
- MulticastNotSupportedException.cs
- HttpResponseInternalBase.cs
- ServicePointManagerElement.cs
- DisplayNameAttribute.cs
- Missing.cs
- RowsCopiedEventArgs.cs
- StateManagedCollection.cs
- ComPlusTypeValidator.cs
- DrawToolTipEventArgs.cs
- ListItemCollection.cs
- LineGeometry.cs
- InfoCardArgumentException.cs
- BaseParser.cs
- MimeFormReflector.cs
- DropAnimation.xaml.cs
- QilChoice.cs
- NetworkStream.cs
- BaseDataBoundControl.cs
- Binding.cs
- UDPClient.cs
- MasterPageParser.cs
- TabControlEvent.cs
- Action.cs
- SessionEndedEventArgs.cs
- SynchronizedReadOnlyCollection.cs
- Dynamic.cs
- SynchronousSendBindingElement.cs
- AspNetHostingPermission.cs
- TableRowCollection.cs
- SQLDateTime.cs
- ItemContainerGenerator.cs
- PreviewControlDesigner.cs
- SafeWaitHandle.cs
- StorageModelBuildProvider.cs
- CodePrimitiveExpression.cs
- OleDbConnectionInternal.cs
- WebConfigurationHostFileChange.cs
- Attributes.cs
- StylusPointPropertyUnit.cs
- DrawingAttributesDefaultValueFactory.cs
- ExecutionPropertyManager.cs
- MethodBuilder.cs
- ImageSource.cs
- SafeTokenHandle.cs
- ImageFormat.cs
- SecurityException.cs
- TreeNodeBindingCollection.cs
- EffectiveValueEntry.cs