Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / Security / Permissions / AspNetHostingPermission.cs / 1305376 / AspNetHostingPermission.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Security; using System.Security.Permissions; using System.Globalization; //NOTE: While AspNetHostingPermissionAttribute resides in System.DLL, // no classes from that DLL are able to make declarative usage of AspNetHostingPermission. [Serializable] public enum AspNetHostingPermissionLevel { None = 100, Minimal = 200, Low = 300, Medium = 400, High = 500, Unrestricted = 600 } [AttributeUsage(AttributeTargets.All, AllowMultiple=true, Inherited=false )] [Serializable] sealed public class AspNetHostingPermissionAttribute : CodeAccessSecurityAttribute { AspNetHostingPermissionLevel _level; public AspNetHostingPermissionAttribute ( SecurityAction action ) : base( action ) { _level = AspNetHostingPermissionLevel.None; } public AspNetHostingPermissionLevel Level { get { return _level; } set { AspNetHostingPermission.VerifyAspNetHostingPermissionLevel(value, "Level"); _level = value; } } public override IPermission CreatePermission() { if (Unrestricted) { return new AspNetHostingPermission(PermissionState.Unrestricted); } else { return new AspNetHostingPermission(_level); } } } ////// [Serializable] public sealed class AspNetHostingPermission : CodeAccessPermission, IUnrestrictedPermission { AspNetHostingPermissionLevel _level; static internal void VerifyAspNetHostingPermissionLevel(AspNetHostingPermissionLevel level, string arg) { switch (level) { case AspNetHostingPermissionLevel.Unrestricted: case AspNetHostingPermissionLevel.High: case AspNetHostingPermissionLevel.Medium: case AspNetHostingPermissionLevel.Low: case AspNetHostingPermissionLevel.Minimal: case AspNetHostingPermissionLevel.None: break; default: throw new ArgumentException(arg); } } ////// ////// public AspNetHostingPermission(PermissionState state) { switch (state) { case PermissionState.Unrestricted: _level = AspNetHostingPermissionLevel.Unrestricted; break; case PermissionState.None: _level = AspNetHostingPermissionLevel.None; break; default: throw new ArgumentException(SR.GetString(SR.InvalidArgument, state.ToString(), "state")); } } public AspNetHostingPermission(AspNetHostingPermissionLevel level) { VerifyAspNetHostingPermissionLevel(level, "level"); _level = level; } public AspNetHostingPermissionLevel Level { get { return _level; } set { VerifyAspNetHostingPermissionLevel(value, "Level"); _level = value; } } // IUnrestrictedPermission interface methods ////// Creates a new instance of the System.Net.AspNetHostingPermission /// class that passes all demands or that fails all demands. /// ////// public bool IsUnrestricted() { return _level == AspNetHostingPermissionLevel.Unrestricted; } // IPermission interface methods ////// Checks the overall permission state of the object. /// ////// public override IPermission Copy () { return new AspNetHostingPermission(_level); } ////// Creates a copy of a System.Net.AspNetHostingPermission /// ////// public override IPermission Union(IPermission target) { if (target == null) { return Copy(); } if (target.GetType() != typeof(AspNetHostingPermission)) { throw new ArgumentException(SR.GetString(SR.InvalidArgument, target == null ? "null" : target.ToString(), "target")); } AspNetHostingPermission other = (AspNetHostingPermission) target; if (Level >= other.Level) { return new AspNetHostingPermission(Level); } else { return new AspNetHostingPermission(other.Level); } } ///Returns the logical union between two System.Net.AspNetHostingPermission instances. ////// public override IPermission Intersect(IPermission target) { if (target == null) { return null; } if (target.GetType() != typeof(AspNetHostingPermission)) { throw new ArgumentException(SR.GetString(SR.InvalidArgument, target == null ? "null" : target.ToString(), "target")); } AspNetHostingPermission other = (AspNetHostingPermission) target; if (Level <= other.Level) { return new AspNetHostingPermission(Level); } else { return new AspNetHostingPermission(other.Level); } } ///Returns the logical intersection between two System.Net.AspNetHostingPermission instances. ////// public override bool IsSubsetOf(IPermission target) { if (target == null) { return _level == AspNetHostingPermissionLevel.None; } if (target.GetType() != typeof(AspNetHostingPermission)) { throw new ArgumentException(SR.GetString(SR.InvalidArgument, target == null ? "null" : target.ToString(), "target")); } AspNetHostingPermission other = (AspNetHostingPermission) target; return Level <= other.Level; } ///Compares two System.Net.AspNetHostingPermission instances. ////// public override void FromXml(SecurityElement securityElement) { if (securityElement == null) { throw new ArgumentNullException(SR.GetString(SR.AspNetHostingPermissionBadXml,"securityElement")); } if (!securityElement.Tag.Equals("IPermission")) { throw new ArgumentException(SR.GetString(SR.AspNetHostingPermissionBadXml,"securityElement")); } string className = securityElement.Attribute("class"); if (className == null) { throw new ArgumentException(SR.GetString(SR.AspNetHostingPermissionBadXml,"securityElement")); } if (className.IndexOf(this.GetType().FullName, StringComparison.Ordinal) < 0) { throw new ArgumentException(SR.GetString(SR.AspNetHostingPermissionBadXml,"securityElement")); } string version = securityElement.Attribute("version"); if (string.Compare(version, "1", StringComparison.OrdinalIgnoreCase) != 0) { throw new ArgumentException(SR.GetString(SR.AspNetHostingPermissionBadXml,"version")); } string level = securityElement.Attribute("Level"); if (level == null) { _level = AspNetHostingPermissionLevel.None; } else { _level = (AspNetHostingPermissionLevel) Enum.Parse(typeof(AspNetHostingPermissionLevel), level); } } ////// public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement("IPermission"); securityElement.AddAttribute("class", this.GetType().FullName + ", " + this.GetType().Module.Assembly.FullName.Replace( '\"', '\'' )); securityElement.AddAttribute("version", "1" ); securityElement.AddAttribute("Level", Enum.GetName(typeof(AspNetHostingPermissionLevel), _level)); if (IsUnrestricted()) { securityElement.AddAttribute("Unrestricted", "true"); } return securityElement; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Security; using System.Security.Permissions; using System.Globalization; //NOTE: While AspNetHostingPermissionAttribute resides in System.DLL, // no classes from that DLL are able to make declarative usage of AspNetHostingPermission. [Serializable] public enum AspNetHostingPermissionLevel { None = 100, Minimal = 200, Low = 300, Medium = 400, High = 500, Unrestricted = 600 } [AttributeUsage(AttributeTargets.All, AllowMultiple=true, Inherited=false )] [Serializable] sealed public class AspNetHostingPermissionAttribute : CodeAccessSecurityAttribute { AspNetHostingPermissionLevel _level; public AspNetHostingPermissionAttribute ( SecurityAction action ) : base( action ) { _level = AspNetHostingPermissionLevel.None; } public AspNetHostingPermissionLevel Level { get { return _level; } set { AspNetHostingPermission.VerifyAspNetHostingPermissionLevel(value, "Level"); _level = value; } } public override IPermission CreatePermission() { if (Unrestricted) { return new AspNetHostingPermission(PermissionState.Unrestricted); } else { return new AspNetHostingPermission(_level); } } } ////// [Serializable] public sealed class AspNetHostingPermission : CodeAccessPermission, IUnrestrictedPermission { AspNetHostingPermissionLevel _level; static internal void VerifyAspNetHostingPermissionLevel(AspNetHostingPermissionLevel level, string arg) { switch (level) { case AspNetHostingPermissionLevel.Unrestricted: case AspNetHostingPermissionLevel.High: case AspNetHostingPermissionLevel.Medium: case AspNetHostingPermissionLevel.Low: case AspNetHostingPermissionLevel.Minimal: case AspNetHostingPermissionLevel.None: break; default: throw new ArgumentException(arg); } } ////// ////// public AspNetHostingPermission(PermissionState state) { switch (state) { case PermissionState.Unrestricted: _level = AspNetHostingPermissionLevel.Unrestricted; break; case PermissionState.None: _level = AspNetHostingPermissionLevel.None; break; default: throw new ArgumentException(SR.GetString(SR.InvalidArgument, state.ToString(), "state")); } } public AspNetHostingPermission(AspNetHostingPermissionLevel level) { VerifyAspNetHostingPermissionLevel(level, "level"); _level = level; } public AspNetHostingPermissionLevel Level { get { return _level; } set { VerifyAspNetHostingPermissionLevel(value, "Level"); _level = value; } } // IUnrestrictedPermission interface methods ////// Creates a new instance of the System.Net.AspNetHostingPermission /// class that passes all demands or that fails all demands. /// ////// public bool IsUnrestricted() { return _level == AspNetHostingPermissionLevel.Unrestricted; } // IPermission interface methods ////// Checks the overall permission state of the object. /// ////// public override IPermission Copy () { return new AspNetHostingPermission(_level); } ////// Creates a copy of a System.Net.AspNetHostingPermission /// ////// public override IPermission Union(IPermission target) { if (target == null) { return Copy(); } if (target.GetType() != typeof(AspNetHostingPermission)) { throw new ArgumentException(SR.GetString(SR.InvalidArgument, target == null ? "null" : target.ToString(), "target")); } AspNetHostingPermission other = (AspNetHostingPermission) target; if (Level >= other.Level) { return new AspNetHostingPermission(Level); } else { return new AspNetHostingPermission(other.Level); } } ///Returns the logical union between two System.Net.AspNetHostingPermission instances. ////// public override IPermission Intersect(IPermission target) { if (target == null) { return null; } if (target.GetType() != typeof(AspNetHostingPermission)) { throw new ArgumentException(SR.GetString(SR.InvalidArgument, target == null ? "null" : target.ToString(), "target")); } AspNetHostingPermission other = (AspNetHostingPermission) target; if (Level <= other.Level) { return new AspNetHostingPermission(Level); } else { return new AspNetHostingPermission(other.Level); } } ///Returns the logical intersection between two System.Net.AspNetHostingPermission instances. ////// public override bool IsSubsetOf(IPermission target) { if (target == null) { return _level == AspNetHostingPermissionLevel.None; } if (target.GetType() != typeof(AspNetHostingPermission)) { throw new ArgumentException(SR.GetString(SR.InvalidArgument, target == null ? "null" : target.ToString(), "target")); } AspNetHostingPermission other = (AspNetHostingPermission) target; return Level <= other.Level; } ///Compares two System.Net.AspNetHostingPermission instances. ////// public override void FromXml(SecurityElement securityElement) { if (securityElement == null) { throw new ArgumentNullException(SR.GetString(SR.AspNetHostingPermissionBadXml,"securityElement")); } if (!securityElement.Tag.Equals("IPermission")) { throw new ArgumentException(SR.GetString(SR.AspNetHostingPermissionBadXml,"securityElement")); } string className = securityElement.Attribute("class"); if (className == null) { throw new ArgumentException(SR.GetString(SR.AspNetHostingPermissionBadXml,"securityElement")); } if (className.IndexOf(this.GetType().FullName, StringComparison.Ordinal) < 0) { throw new ArgumentException(SR.GetString(SR.AspNetHostingPermissionBadXml,"securityElement")); } string version = securityElement.Attribute("version"); if (string.Compare(version, "1", StringComparison.OrdinalIgnoreCase) != 0) { throw new ArgumentException(SR.GetString(SR.AspNetHostingPermissionBadXml,"version")); } string level = securityElement.Attribute("Level"); if (level == null) { _level = AspNetHostingPermissionLevel.None; } else { _level = (AspNetHostingPermissionLevel) Enum.Parse(typeof(AspNetHostingPermissionLevel), level); } } ////// public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement("IPermission"); securityElement.AddAttribute("class", this.GetType().FullName + ", " + this.GetType().Module.Assembly.FullName.Replace( '\"', '\'' )); securityElement.AddAttribute("version", "1" ); securityElement.AddAttribute("Level", Enum.GetName(typeof(AspNetHostingPermissionLevel), _level)); if (IsUnrestricted()) { securityElement.AddAttribute("Unrestricted", "true"); } return securityElement; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
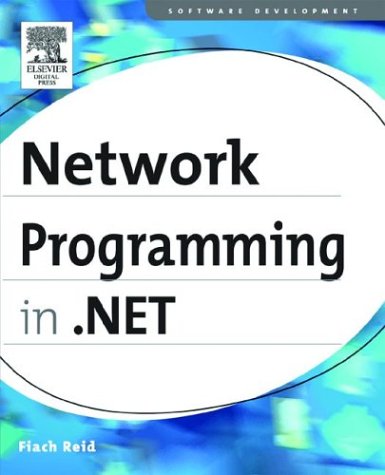
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SoapExtensionTypeElement.cs
- DetailsViewInsertEventArgs.cs
- FloaterParagraph.cs
- ToolBarPanel.cs
- PriorityQueue.cs
- DragEvent.cs
- PrimaryKeyTypeConverter.cs
- ConstructorExpr.cs
- NativeActivity.cs
- SynchronizationLockException.cs
- ToolStripGrip.cs
- NullableFloatAverageAggregationOperator.cs
- TemplateBamlRecordReader.cs
- VarRefManager.cs
- BitmapData.cs
- MimeFormatter.cs
- ResolvedKeyFrameEntry.cs
- SpeechRecognitionEngine.cs
- StaticFileHandler.cs
- AsmxEndpointPickerExtension.cs
- ResolvedKeyFrameEntry.cs
- GetPageNumberCompletedEventArgs.cs
- While.cs
- LassoSelectionBehavior.cs
- BoundField.cs
- SingletonInstanceContextProvider.cs
- DataGridViewDataErrorEventArgs.cs
- GroupItem.cs
- GroupLabel.cs
- TrustSection.cs
- BooleanStorage.cs
- NameGenerator.cs
- LineVisual.cs
- TagMapInfo.cs
- UpdateProgress.cs
- ProtocolsSection.cs
- XamlVector3DCollectionSerializer.cs
- OneOfElement.cs
- ILGen.cs
- StringUtil.cs
- NumericUpDownAcceleration.cs
- RelatedView.cs
- ServiceManagerHandle.cs
- Paragraph.cs
- ConfigurationLockCollection.cs
- CurrentChangingEventArgs.cs
- PinnedBufferMemoryStream.cs
- FlowNode.cs
- SystemResourceHost.cs
- AsnEncodedData.cs
- AgileSafeNativeMemoryHandle.cs
- AutomationPropertyInfo.cs
- StylusButtonEventArgs.cs
- ObjectPersistData.cs
- HebrewCalendar.cs
- DecimalConverter.cs
- GraphicsPathIterator.cs
- TcpServerChannel.cs
- ColumnPropertiesGroup.cs
- ResolvedKeyFrameEntry.cs
- EncryptedKey.cs
- InstanceHandleConflictException.cs
- WindowsSlider.cs
- Inflater.cs
- ResourceSet.cs
- SAPIEngineTypes.cs
- EventMap.cs
- cryptoapiTransform.cs
- WeakReferenceKey.cs
- ViewPort3D.cs
- TraceHandler.cs
- SplitContainerDesigner.cs
- RequestCachingSection.cs
- Configuration.cs
- DATA_BLOB.cs
- SqlRewriteScalarSubqueries.cs
- IDQuery.cs
- BasePattern.cs
- PerformanceCounter.cs
- Stopwatch.cs
- XmlKeywords.cs
- CultureInfoConverter.cs
- AnnotationResourceCollection.cs
- StyleCollection.cs
- XmlSchemaExporter.cs
- DelayLoadType.cs
- XmlQueryContext.cs
- ConnectionPointGlyph.cs
- PrimaryKeyTypeConverter.cs
- WebBrowserSiteBase.cs
- LinkedList.cs
- ManagementEventArgs.cs
- DbConnectionStringBuilder.cs
- DrawingVisualDrawingContext.cs
- CaseInsensitiveHashCodeProvider.cs
- StateChangeEvent.cs
- DisplayInformation.cs
- XmlSchema.cs
- Label.cs
- QueryStringHandler.cs