Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Controls / GroupItem.cs / 2 / GroupItem.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: GroupItem object - root of the UI subtree generated for a CollectionViewGroup // // Specs: http://avalon/connecteddata/M5%20General%20Docs/Data%20Styling.mht // //--------------------------------------------------------------------------- using System; using System.Collections; namespace System.Windows.Controls { ////// A GroupItem appears as the root of the visual subtree generated for a CollectionViewGroup. /// public class GroupItem : ContentControl { static GroupItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(GroupItem), new FrameworkPropertyMetadata(typeof(GroupItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(GroupItem)); // GroupItems should not be focusable by default FocusableProperty.OverrideMetadata(typeof(GroupItem), new FrameworkPropertyMetadata(false)); } ////// Creates AutomationPeer ( protected override System.Windows.Automation.Peers.AutomationPeer OnCreateAutomationPeer() { return new System.Windows.Automation.Peers.GroupItemAutomationPeer(this); } ///) /// /// Gives a string representation of this object. /// ///internal override string GetPlainText() { System.Windows.Data.CollectionViewGroup cvg = Content as System.Windows.Data.CollectionViewGroup; if (cvg != null && cvg.Name != null) { return cvg.Name.ToString(); } return base.GetPlainText(); } //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- internal ItemContainerGenerator Generator { get { return _generator; } set { _generator = value; } } //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- internal void PrepareItemContainer(object item) { if (Generator == null) return; // user-declared GroupItem - ignore (bug 108423) ItemContainerGenerator generator = Generator.Parent; GroupStyle groupStyle = generator.GroupStyle; // apply the container style Style style = groupStyle.ContainerStyle; // no ContainerStyle set, try ContainerStyleSelector if (style == null) { if (groupStyle.ContainerStyleSelector != null) { style = groupStyle.ContainerStyleSelector.SelectStyle(item, this); } } // apply the style, if found if (style != null) { // verify style is appropriate before applying it if (!style.TargetType.IsInstanceOfType(this)) throw new InvalidOperationException(SR.Get(SRID.StyleForWrongType, style.TargetType.Name, this.GetType().Name)); this.Style = style; this.WriteInternalFlag2(InternalFlags2.IsStyleSetFromGenerator, true); } // forward the header template information if (!HasNonDefaultValue(ContentProperty)) this.Content = item; if (!HasNonDefaultValue(ContentTemplateProperty)) this.ContentTemplate = groupStyle.HeaderTemplate; if (!HasNonDefaultValue(ContentTemplateSelectorProperty)) this.ContentTemplateSelector = groupStyle.HeaderTemplateSelector; if (!HasNonDefaultValue(ContentStringFormatProperty)) this.ContentStringFormat = groupStyle.HeaderStringFormat; } internal void ClearContainerForItem(object item) { if (Generator == null) return; // user-declared GroupItem - ignore (bug 108423) ItemContainerGenerator generator = Generator.Parent; GroupStyle groupStyle = generator.GroupStyle; if (Object.Equals(this.Content, item)) ClearValue(ContentProperty); if (this.ContentTemplate == groupStyle.HeaderTemplate) ClearValue(ContentTemplateProperty); if (this.ContentTemplateSelector == groupStyle.HeaderTemplateSelector) ClearValue(ContentTemplateSelectorProperty); if (this.ContentStringFormat == groupStyle.HeaderStringFormat) ClearValue(ContentStringFormatProperty); Generator.Release(); } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ ItemContainerGenerator _generator; #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: GroupItem object - root of the UI subtree generated for a CollectionViewGroup // // Specs: http://avalon/connecteddata/M5%20General%20Docs/Data%20Styling.mht // //--------------------------------------------------------------------------- using System; using System.Collections; namespace System.Windows.Controls { ////// A GroupItem appears as the root of the visual subtree generated for a CollectionViewGroup. /// public class GroupItem : ContentControl { static GroupItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(GroupItem), new FrameworkPropertyMetadata(typeof(GroupItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(GroupItem)); // GroupItems should not be focusable by default FocusableProperty.OverrideMetadata(typeof(GroupItem), new FrameworkPropertyMetadata(false)); } ////// Creates AutomationPeer ( protected override System.Windows.Automation.Peers.AutomationPeer OnCreateAutomationPeer() { return new System.Windows.Automation.Peers.GroupItemAutomationPeer(this); } ///) /// /// Gives a string representation of this object. /// ///internal override string GetPlainText() { System.Windows.Data.CollectionViewGroup cvg = Content as System.Windows.Data.CollectionViewGroup; if (cvg != null && cvg.Name != null) { return cvg.Name.ToString(); } return base.GetPlainText(); } //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- internal ItemContainerGenerator Generator { get { return _generator; } set { _generator = value; } } //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- internal void PrepareItemContainer(object item) { if (Generator == null) return; // user-declared GroupItem - ignore (bug 108423) ItemContainerGenerator generator = Generator.Parent; GroupStyle groupStyle = generator.GroupStyle; // apply the container style Style style = groupStyle.ContainerStyle; // no ContainerStyle set, try ContainerStyleSelector if (style == null) { if (groupStyle.ContainerStyleSelector != null) { style = groupStyle.ContainerStyleSelector.SelectStyle(item, this); } } // apply the style, if found if (style != null) { // verify style is appropriate before applying it if (!style.TargetType.IsInstanceOfType(this)) throw new InvalidOperationException(SR.Get(SRID.StyleForWrongType, style.TargetType.Name, this.GetType().Name)); this.Style = style; this.WriteInternalFlag2(InternalFlags2.IsStyleSetFromGenerator, true); } // forward the header template information if (!HasNonDefaultValue(ContentProperty)) this.Content = item; if (!HasNonDefaultValue(ContentTemplateProperty)) this.ContentTemplate = groupStyle.HeaderTemplate; if (!HasNonDefaultValue(ContentTemplateSelectorProperty)) this.ContentTemplateSelector = groupStyle.HeaderTemplateSelector; if (!HasNonDefaultValue(ContentStringFormatProperty)) this.ContentStringFormat = groupStyle.HeaderStringFormat; } internal void ClearContainerForItem(object item) { if (Generator == null) return; // user-declared GroupItem - ignore (bug 108423) ItemContainerGenerator generator = Generator.Parent; GroupStyle groupStyle = generator.GroupStyle; if (Object.Equals(this.Content, item)) ClearValue(ContentProperty); if (this.ContentTemplate == groupStyle.HeaderTemplate) ClearValue(ContentTemplateProperty); if (this.ContentTemplateSelector == groupStyle.HeaderTemplateSelector) ClearValue(ContentTemplateSelectorProperty); if (this.ContentStringFormat == groupStyle.HeaderStringFormat) ClearValue(ContentStringFormatProperty); Generator.Release(); } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ ItemContainerGenerator _generator; #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
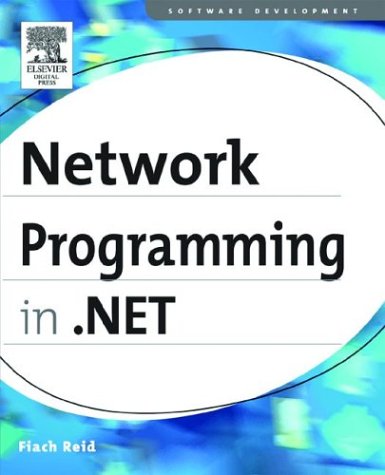
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Renderer.cs
- TabPageDesigner.cs
- ToolStrip.cs
- NullRuntimeConfig.cs
- Model3D.cs
- MetadataItemSerializer.cs
- SkipStoryboardToFill.cs
- ValidatorCompatibilityHelper.cs
- Rotation3D.cs
- BindableTemplateBuilder.cs
- DataGridAutoGeneratingColumnEventArgs.cs
- Roles.cs
- WebAdminConfigurationHelper.cs
- ImageDrawing.cs
- DecoderBestFitFallback.cs
- newinstructionaction.cs
- DATA_BLOB.cs
- SapiAttributeParser.cs
- FormViewDeleteEventArgs.cs
- FontEmbeddingManager.cs
- SolidColorBrush.cs
- HScrollProperties.cs
- PrintDialog.cs
- _HelperAsyncResults.cs
- X509CertificateInitiatorServiceCredential.cs
- _UncName.cs
- CounterCreationData.cs
- StructuralType.cs
- ThreadStartException.cs
- SRGSCompiler.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- Logging.cs
- ContentTextAutomationPeer.cs
- AudioLevelUpdatedEventArgs.cs
- RecordManager.cs
- UrlMappingCollection.cs
- SqlBuilder.cs
- CodeLinePragma.cs
- SQLInt16.cs
- Select.cs
- RC2.cs
- XmlNotation.cs
- CrossAppDomainChannel.cs
- DataTemplateSelector.cs
- SqlEnums.cs
- CodeNamespaceImport.cs
- MethodBody.cs
- CLSCompliantAttribute.cs
- TextStore.cs
- ParseElementCollection.cs
- SwitchDesigner.xaml.cs
- ScrollItemPatternIdentifiers.cs
- MailMessage.cs
- XmlNode.cs
- EntityClassGenerator.cs
- SoapFault.cs
- _SslState.cs
- XmlUTF8TextWriter.cs
- RoleManagerEventArgs.cs
- InheritedPropertyChangedEventArgs.cs
- ZipIORawDataFileBlock.cs
- AnimationStorage.cs
- ResolveNameEventArgs.cs
- ProfileGroupSettings.cs
- DataPagerCommandEventArgs.cs
- AdornedElementPlaceholder.cs
- TableLayoutPanelBehavior.cs
- ToolStripDropDownButton.cs
- HtmlElement.cs
- NominalTypeEliminator.cs
- PropertyDescriptor.cs
- CodeNamespaceCollection.cs
- IgnoreFlushAndCloseStream.cs
- Rectangle.cs
- DbDataSourceEnumerator.cs
- TCEAdapterGenerator.cs
- VisualCollection.cs
- FormViewUpdateEventArgs.cs
- CqlIdentifiers.cs
- TypeExtensions.cs
- Parsers.cs
- DynamicUpdateCommand.cs
- ComPlusDiagnosticTraceSchemas.cs
- DataGridPagerStyle.cs
- EntityDataSourceDesignerHelper.cs
- WebPartTransformerAttribute.cs
- LinkLabel.cs
- RSAPKCS1SignatureDeformatter.cs
- sortedlist.cs
- KeyboardEventArgs.cs
- ModelMemberCollection.cs
- WebPartCloseVerb.cs
- DataControlImageButton.cs
- ArgumentsParser.cs
- SqlGatherConsumedAliases.cs
- LogStore.cs
- ExpiredSecurityTokenException.cs
- FreezableOperations.cs
- VectorConverter.cs
- SecurityUtils.cs