Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Interaction / Model / ModelMemberCollection.cs / 1305376 / ModelMemberCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics.CodeAnalysis; using System.Activities.Presentation; ////// ModelMemberCollection is an abstract base class that /// ModelPropertyCollection and ModelEventCollection derive from. /// ///The type of item the collection represents. ///The type that should be used as a key in "Find" methods. public abstract class ModelMemberCollection: IEnumerable , IEnumerable { /// /// Internal constructor. Only our own collections can derive from this class. /// internal ModelMemberCollection() { } ////// Searches the collection for the given key and returns it /// if it is found. If not found, this throws an exception. /// /// ////// if name is null. ///if name is not found. public TItemType this[string name] { get { if (name == null) throw FxTrace.Exception.ArgumentNull("name"); return Find(name, true); } } ////// Searches the collection for the given key and returns it /// if it is found. If not found, this throws an exception. /// /// ////// if value is null. ///if value is not found. [SuppressMessage("Microsoft.Design", "CA1043:UseIntegralOrStringArgumentForIndexers")] public TItemType this[TFindType value] { get { if (value == null) throw FxTrace.Exception.ArgumentNull("value"); return Find(value, true); } } ////// Searches the collection for the given key and returns it if it is /// found. If not found, this returns null. /// /// ////// if name is null. public TItemType Find(string name) { if (name == null) throw FxTrace.Exception.ArgumentNull("name"); return Find(name, false); } ////// Searches the collection for the given key and returns it if it is /// found. If not found, this throws an exception or returns null, /// depending on the value passed to throwOnError. /// /// /// ////// if name is not found and throwOnError is true. protected abstract TItemType Find(string name, bool throwOnError); ////// Searches the collection for the given key and returns it if it is /// found. If not found, this returns null. /// /// ////// if value is null. public TItemType Find(TFindType value) { if (value == null) throw FxTrace.Exception.ArgumentNull("value"); return Find(value, false); } ////// Searches the collection for the given key and returns it if it is /// found. If not found, this throws an exception or returns null, /// depending on the value passed to throwOnError. /// /// /// ////// if value is not found and throwOnError is true. protected abstract TItemType Find(TFindType value, bool throwOnError); ////// Returns an enumerator to enumerate values. /// ///public abstract IEnumerator GetEnumerator(); #region IEnumerable Members /// /// IEnumerable Implementation. /// ///IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics.CodeAnalysis; using System.Activities.Presentation; ////// ModelMemberCollection is an abstract base class that /// ModelPropertyCollection and ModelEventCollection derive from. /// ///The type of item the collection represents. ///The type that should be used as a key in "Find" methods. public abstract class ModelMemberCollection: IEnumerable , IEnumerable { /// /// Internal constructor. Only our own collections can derive from this class. /// internal ModelMemberCollection() { } ////// Searches the collection for the given key and returns it /// if it is found. If not found, this throws an exception. /// /// ////// if name is null. ///if name is not found. public TItemType this[string name] { get { if (name == null) throw FxTrace.Exception.ArgumentNull("name"); return Find(name, true); } } ////// Searches the collection for the given key and returns it /// if it is found. If not found, this throws an exception. /// /// ////// if value is null. ///if value is not found. [SuppressMessage("Microsoft.Design", "CA1043:UseIntegralOrStringArgumentForIndexers")] public TItemType this[TFindType value] { get { if (value == null) throw FxTrace.Exception.ArgumentNull("value"); return Find(value, true); } } ////// Searches the collection for the given key and returns it if it is /// found. If not found, this returns null. /// /// ////// if name is null. public TItemType Find(string name) { if (name == null) throw FxTrace.Exception.ArgumentNull("name"); return Find(name, false); } ////// Searches the collection for the given key and returns it if it is /// found. If not found, this throws an exception or returns null, /// depending on the value passed to throwOnError. /// /// /// ////// if name is not found and throwOnError is true. protected abstract TItemType Find(string name, bool throwOnError); ////// Searches the collection for the given key and returns it if it is /// found. If not found, this returns null. /// /// ////// if value is null. public TItemType Find(TFindType value) { if (value == null) throw FxTrace.Exception.ArgumentNull("value"); return Find(value, false); } ////// Searches the collection for the given key and returns it if it is /// found. If not found, this throws an exception or returns null, /// depending on the value passed to throwOnError. /// /// /// ////// if value is not found and throwOnError is true. protected abstract TItemType Find(TFindType value, bool throwOnError); ////// Returns an enumerator to enumerate values. /// ///public abstract IEnumerator GetEnumerator(); #region IEnumerable Members /// /// IEnumerable Implementation. /// ///IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
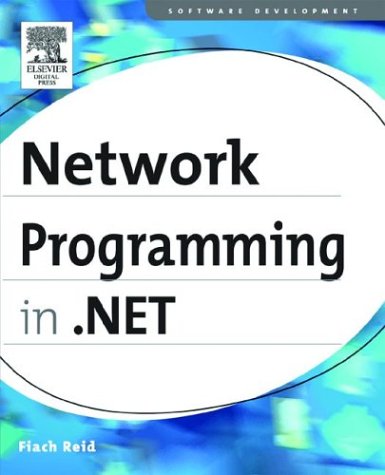
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AxHostDesigner.cs
- CompiledRegexRunnerFactory.cs
- ZipIOLocalFileBlock.cs
- CustomAttribute.cs
- FormViewDeletedEventArgs.cs
- SelectedPathEditor.cs
- WebBrowserContainer.cs
- TdsParameterSetter.cs
- XamlClipboardData.cs
- ManagementDateTime.cs
- WebResourceAttribute.cs
- dbdatarecord.cs
- WorkItem.cs
- PlainXmlWriter.cs
- ChannelServices.cs
- RangeValueProviderWrapper.cs
- Win32PrintDialog.cs
- SnapshotChangeTrackingStrategy.cs
- CurrentChangingEventManager.cs
- CompilerWrapper.cs
- _emptywebproxy.cs
- TemplateControlCodeDomTreeGenerator.cs
- ThaiBuddhistCalendar.cs
- ComponentCollection.cs
- TextSelectionProcessor.cs
- IdentityManager.cs
- ChildTable.cs
- TextServicesCompartmentContext.cs
- wgx_exports.cs
- CommunicationException.cs
- ScriptingWebServicesSectionGroup.cs
- UpdateCommand.cs
- TakeOrSkipWhileQueryOperator.cs
- Parameter.cs
- SqlGenericUtil.cs
- NegationPusher.cs
- WeakReferenceEnumerator.cs
- TailCallAnalyzer.cs
- UserInitiatedNavigationPermission.cs
- ComponentResourceKeyConverter.cs
- MbpInfo.cs
- InstanceCreationEditor.cs
- SelectedGridItemChangedEvent.cs
- ChannelFactory.cs
- EnumValidator.cs
- mda.cs
- HwndSubclass.cs
- ProcessModule.cs
- SqlDataSourceView.cs
- ChannelBinding.cs
- AnnotationResourceChangedEventArgs.cs
- XPathAncestorQuery.cs
- SqlBinder.cs
- XamlToRtfParser.cs
- latinshape.cs
- SQLDecimal.cs
- WindowVisualStateTracker.cs
- Pair.cs
- SHA1CryptoServiceProvider.cs
- EUCJPEncoding.cs
- LinkClickEvent.cs
- UiaCoreTypesApi.cs
- ObjectQueryExecutionPlan.cs
- DeobfuscatingStream.cs
- HttpConfigurationContext.cs
- WorkflowInstanceUnhandledExceptionRecord.cs
- UriTemplateMatchException.cs
- TransactionsSectionGroup.cs
- __Filters.cs
- StringWriter.cs
- VerificationAttribute.cs
- PersonalizationProviderCollection.cs
- XmlIlGenerator.cs
- TemplateBuilder.cs
- EditorZoneBase.cs
- Stroke.cs
- XPathExpr.cs
- PlainXmlWriter.cs
- Polygon.cs
- Hex.cs
- RepeatBehavior.cs
- DbModificationCommandTree.cs
- MsmqInputMessagePool.cs
- ControlUtil.cs
- StringKeyFrameCollection.cs
- FormViewAutoFormat.cs
- FileClassifier.cs
- ImageFormatConverter.cs
- ConfigPathUtility.cs
- StatusBarPanelClickEvent.cs
- ComponentChangingEvent.cs
- ResourceDescriptionAttribute.cs
- PropertyGeneratedEventArgs.cs
- SqlInternalConnection.cs
- rsa.cs
- SSmlParser.cs
- NullExtension.cs
- CompiledQuery.cs
- IconConverter.cs
- OptionalRstParameters.cs