Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Map / Update / Internal / UpdateCommand.cs / 1 / UpdateCommand.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Data.Common; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Globalization; using System.Data.Common.Utils; using System.Data.Common.CommandTrees; using System.Data.Objects; using System.Linq; using System.Data.EntityClient; namespace System.Data.Mapping.Update.Internal { internal enum UpdateCommandKind { Dynamic, Function, } ////// Class storing the result of compiling an instance DML command. /// internal abstract class UpdateCommand : IComparable, IEquatable { /// /// Gets all identifiers (key values basically) generated by this command. For instance, /// @@IDENTITY values. /// internal abstract IEnumerableOutputIdentifiers { get; } /// /// Gets all identifiers required by this command. /// internal abstract IEnumerableInputIdentifiers { get; } /// /// Gets table (if any) associated with the current command. FunctionUpdateCommand has no table. /// internal virtual EntitySet Table { get { return null; } } ////// Gets type of command. /// internal abstract UpdateCommandKind Kind { get; } ////// Yields all state entries contributing to this command. Used for error reporting. /// /// Translator context. ///Related state entries. internal abstract ListGetStateEntries(UpdateTranslator translator); /// /// Determines model level dependencies for the current command. A command 'requires' an entity /// (in other words, it must follow commands 'producing' that entity) if it deletes the entity /// or inserts a relationship to that entity. A command 'produces' an entity (in other words, /// it must precede commands 'requiring' that entity) if it inserts the entity or deletes a relationship /// to that entity. /// internal void GetRequiredAndProducedEntities(UpdateTranslator translator, out Listrequired, out List produced) { List stateEntries = GetStateEntries(translator); required = new List (); produced = new List (); // determine parent entity (a command must handle at most one entity) EntityKey parent = null; foreach (IEntityStateEntry stateEntry in stateEntries) { if (!stateEntry.IsRelationship) { parent = stateEntry.EntityKey; Debug.Assert(stateEntries.Where(e => !e.IsRelationship).Select(e => e.EntityKey).Distinct().Count() <= 1, "more than one IEntityStateEntry is an Entity!"); if (stateEntry.State == EntityState.Added) { produced.Add(parent); } else if (stateEntry.State == EntityState.Deleted) { required.Add(parent); } break; } } // process relationships foreach (IEntityStateEntry stateEntry in stateEntries) { if (stateEntry.IsRelationship) { // only worry about the relationship if it is being added or deleted bool isAdded = stateEntry.State == EntityState.Added; if (isAdded || stateEntry.State == EntityState.Deleted) { DbDataRecord record = isAdded ? (DbDataRecord)stateEntry.CurrentValues : stateEntry.OriginalValues; Debug.Assert(2 == record.FieldCount, "non-binary relationship?"); EntityKey end1 = (EntityKey)record[0]; EntityKey end2 = (EntityKey)record[1]; // relationships require the entity when they're added and free the entity when they're deleted... List affected = isAdded ? required : produced; if (parent == null) { // both ends are being modified by the relationship affected.Add(end1); affected.Add(end2); } else { // only the end opposite the parent is being modified by the relationship... if (parent.Equals(end1)) { affected.Add(end2); } else { affected.Add(end1); } } } } } } /// /// Executes the current update command. /// /// Translator context. /// EntityConnection to use (and implicitly, the EntityTransaction to use). /// Aggregator for identifier values (read for InputIdentifiers; write for /// OutputIdentifiers /// Aggregator for server generated values. ///Number of rows affected by the command. internal abstract int Execute(UpdateTranslator translator, EntityConnection connection, DictionaryidentifierValues, List > generatedValues); internal abstract int CompareToType(UpdateCommand other); public int CompareTo(UpdateCommand other) { if (this.Equals(other)) { return 0; } Debug.Assert(null != other, "comparing to null UpdateCommand"); int result = (int)this.Kind - (int)other.Kind; if (0 != result) { return result; } // defer to specific type for other comparisons... result = CompareToType(other); return result; } #region IEquatable: note that we use reference equality public bool Equals(UpdateCommand other) { return base.Equals(other); } public override bool Equals(object obj) { return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Data.Common; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Globalization; using System.Data.Common.Utils; using System.Data.Common.CommandTrees; using System.Data.Objects; using System.Linq; using System.Data.EntityClient; namespace System.Data.Mapping.Update.Internal { internal enum UpdateCommandKind { Dynamic, Function, } ////// Class storing the result of compiling an instance DML command. /// internal abstract class UpdateCommand : IComparable, IEquatable { /// /// Gets all identifiers (key values basically) generated by this command. For instance, /// @@IDENTITY values. /// internal abstract IEnumerableOutputIdentifiers { get; } /// /// Gets all identifiers required by this command. /// internal abstract IEnumerableInputIdentifiers { get; } /// /// Gets table (if any) associated with the current command. FunctionUpdateCommand has no table. /// internal virtual EntitySet Table { get { return null; } } ////// Gets type of command. /// internal abstract UpdateCommandKind Kind { get; } ////// Yields all state entries contributing to this command. Used for error reporting. /// /// Translator context. ///Related state entries. internal abstract ListGetStateEntries(UpdateTranslator translator); /// /// Determines model level dependencies for the current command. A command 'requires' an entity /// (in other words, it must follow commands 'producing' that entity) if it deletes the entity /// or inserts a relationship to that entity. A command 'produces' an entity (in other words, /// it must precede commands 'requiring' that entity) if it inserts the entity or deletes a relationship /// to that entity. /// internal void GetRequiredAndProducedEntities(UpdateTranslator translator, out Listrequired, out List produced) { List stateEntries = GetStateEntries(translator); required = new List (); produced = new List (); // determine parent entity (a command must handle at most one entity) EntityKey parent = null; foreach (IEntityStateEntry stateEntry in stateEntries) { if (!stateEntry.IsRelationship) { parent = stateEntry.EntityKey; Debug.Assert(stateEntries.Where(e => !e.IsRelationship).Select(e => e.EntityKey).Distinct().Count() <= 1, "more than one IEntityStateEntry is an Entity!"); if (stateEntry.State == EntityState.Added) { produced.Add(parent); } else if (stateEntry.State == EntityState.Deleted) { required.Add(parent); } break; } } // process relationships foreach (IEntityStateEntry stateEntry in stateEntries) { if (stateEntry.IsRelationship) { // only worry about the relationship if it is being added or deleted bool isAdded = stateEntry.State == EntityState.Added; if (isAdded || stateEntry.State == EntityState.Deleted) { DbDataRecord record = isAdded ? (DbDataRecord)stateEntry.CurrentValues : stateEntry.OriginalValues; Debug.Assert(2 == record.FieldCount, "non-binary relationship?"); EntityKey end1 = (EntityKey)record[0]; EntityKey end2 = (EntityKey)record[1]; // relationships require the entity when they're added and free the entity when they're deleted... List affected = isAdded ? required : produced; if (parent == null) { // both ends are being modified by the relationship affected.Add(end1); affected.Add(end2); } else { // only the end opposite the parent is being modified by the relationship... if (parent.Equals(end1)) { affected.Add(end2); } else { affected.Add(end1); } } } } } } /// /// Executes the current update command. /// /// Translator context. /// EntityConnection to use (and implicitly, the EntityTransaction to use). /// Aggregator for identifier values (read for InputIdentifiers; write for /// OutputIdentifiers /// Aggregator for server generated values. ///Number of rows affected by the command. internal abstract int Execute(UpdateTranslator translator, EntityConnection connection, DictionaryidentifierValues, List > generatedValues); internal abstract int CompareToType(UpdateCommand other); public int CompareTo(UpdateCommand other) { if (this.Equals(other)) { return 0; } Debug.Assert(null != other, "comparing to null UpdateCommand"); int result = (int)this.Kind - (int)other.Kind; if (0 != result) { return result; } // defer to specific type for other comparisons... result = CompareToType(other); return result; } #region IEquatable: note that we use reference equality public bool Equals(UpdateCommand other) { return base.Equals(other); } public override bool Equals(object obj) { return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
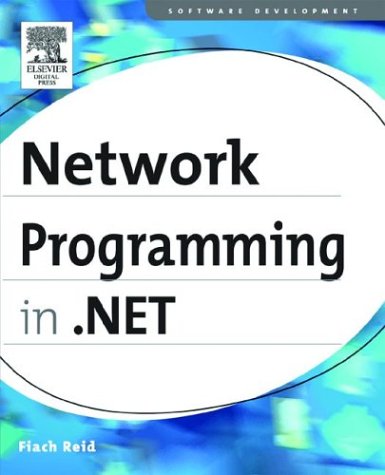
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HwndTarget.cs
- UndoManager.cs
- UidPropertyAttribute.cs
- MgmtConfigurationRecord.cs
- DbMetaDataFactory.cs
- ParserExtension.cs
- SectionUpdates.cs
- MemoryMappedFile.cs
- EventLogEntryCollection.cs
- XmlSchemaComplexContentRestriction.cs
- DeclaredTypeElementCollection.cs
- ResXResourceSet.cs
- DrawingImage.cs
- HtmlTextArea.cs
- ClassicBorderDecorator.cs
- BevelBitmapEffect.cs
- CacheDict.cs
- RegexMatch.cs
- ListViewGroupConverter.cs
- TemplateField.cs
- UpdatePanelControlTrigger.cs
- DependencyPropertyConverter.cs
- ExitEventArgs.cs
- RequestQueue.cs
- UriTemplateClientFormatter.cs
- LinkGrep.cs
- ScriptResourceInfo.cs
- DataGridTemplateColumn.cs
- PerformanceCounterManager.cs
- RestHandler.cs
- LinkArea.cs
- ConfigurationManagerHelperFactory.cs
- BitmapEffectGroup.cs
- Symbol.cs
- MenuDesigner.cs
- XmlUtil.cs
- TitleStyle.cs
- CollectionViewGroup.cs
- RepeaterItemCollection.cs
- SerialPinChanges.cs
- PeerCollaborationPermission.cs
- CompositeTypefaceMetrics.cs
- DependencyObjectPropertyDescriptor.cs
- PeerEndPoint.cs
- Geometry.cs
- XpsFilter.cs
- RepeaterItemCollection.cs
- Parsers.cs
- Publisher.cs
- SessionViewState.cs
- StatusBarDrawItemEvent.cs
- SubMenuStyleCollection.cs
- TargetPerspective.cs
- DataGridSortCommandEventArgs.cs
- TraceRecord.cs
- TabItemAutomationPeer.cs
- ImageDrawing.cs
- HiddenFieldPageStatePersister.cs
- XhtmlTextWriter.cs
- TextView.cs
- metadatamappinghashervisitor.cs
- SuppressMergeCheckAttribute.cs
- PackWebRequestFactory.cs
- SwitchLevelAttribute.cs
- AttributeEmitter.cs
- DataGridRow.cs
- HelpKeywordAttribute.cs
- NumberFormatInfo.cs
- SortedDictionary.cs
- ButtonField.cs
- PeerObject.cs
- SqlCharStream.cs
- ToolBar.cs
- Native.cs
- XmlSortKeyAccumulator.cs
- UrlPropertyAttribute.cs
- SqlWorkflowInstanceStoreLock.cs
- URLIdentityPermission.cs
- QueuePathDialog.cs
- HtmlMobileTextWriter.cs
- UriParserTemplates.cs
- DataBindingsDialog.cs
- _SslState.cs
- QilSortKey.cs
- OverflowException.cs
- TrustManager.cs
- KeyInfo.cs
- TransportConfigurationTypeElementCollection.cs
- Rotation3D.cs
- XpsImageSerializationService.cs
- AdapterSwitches.cs
- SymLanguageVendor.cs
- InputLangChangeRequestEvent.cs
- UrlRoutingModule.cs
- CleanUpVirtualizedItemEventArgs.cs
- PageOrientation.cs
- EncodingNLS.cs
- OdbcParameterCollection.cs
- AQNBuilder.cs
- SqlRemoveConstantOrderBy.cs