Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / xsp / System / Web / Extensions / ui / ScriptResourceInfo.cs / 3 / ScriptResourceInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Reflection; using System.Text; using System.Web.Resources; using System.Web.Util; internal class ScriptResourceInfo { private string _contentType; private bool _performSubstitution; private string _scriptName; private string _scriptResourceName; private string _typeName; private bool _isDebug; private static readonly IDictionary _scriptCache = Hashtable.Synchronized(new Hashtable()); private static readonly IDictionary _duplicateScriptAttributesChecked = Hashtable.Synchronized(new Hashtable()); public static readonly ScriptResourceInfo Empty = new ScriptResourceInfo(); private ScriptResourceInfo() { } public ScriptResourceInfo(ScriptResourceAttribute attr) : this() { _scriptName = attr.ScriptName; _scriptResourceName = attr.ScriptResourceName; _typeName = attr.TypeName; } public string ContentType { get { return _contentType; } set { _contentType = value; } } public bool IsDebug { get { return _isDebug; } set { _isDebug = value; } } public bool PerformSubstitution { get { return _performSubstitution; } set { _performSubstitution = value; } } public string ScriptName { get { return _scriptName; } set { _scriptName = value; } } public string ScriptResourceName { get { return _scriptResourceName; } } public string TypeName { get { return _typeName; } } public static ScriptResourceInfo GetInstance(Assembly assembly, string resourceName) { // The first time this API is called, check for attributes that point to the same script if (!_duplicateScriptAttributesChecked.Contains(assembly)) { Dictionaryscripts = new Dictionary (); foreach (ScriptResourceAttribute attr in assembly.GetCustomAttributes(typeof(ScriptResourceAttribute), false)) { string scriptName = attr.ScriptName; if (scripts.ContainsKey(scriptName)) { throw new InvalidOperationException( String.Format(CultureInfo.CurrentCulture, AtlasWeb.ScriptResourceHandler_DuplicateScriptResources, scriptName, assembly.GetName())); } scripts.Add(scriptName, true); } _duplicateScriptAttributesChecked[assembly] = true; } Pair cacheKey = new Pair (assembly, resourceName); ScriptResourceInfo resourceInfo = (ScriptResourceInfo)_scriptCache[cacheKey]; if (resourceInfo == null) { resourceInfo = ScriptResourceInfo.Empty; object[] attrs = assembly.GetCustomAttributes(typeof(ScriptResourceAttribute), false); // First look for a script resource attribute with that name for (int i = 0; i < attrs.Length; i++) { ScriptResourceAttribute sra = (ScriptResourceAttribute)attrs[i]; if (String.Equals(sra.ScriptName, resourceName, StringComparison.Ordinal)) { resourceInfo = new ScriptResourceInfo(sra); break; } } // Look for a web resource with that name, to get additional info if any attrs = assembly.GetCustomAttributes(typeof(WebResourceAttribute), false); for (int i = 0; i < attrs.Length; i++) { WebResourceAttribute wra = (WebResourceAttribute)attrs[i]; if (String.Equals(wra.WebResource, resourceName, StringComparison.Ordinal)) { if (resourceInfo == ScriptResourceInfo.Empty) { resourceInfo = new ScriptResourceInfo(); resourceInfo.ScriptName = resourceName; } resourceInfo.ContentType = wra.ContentType; resourceInfo.PerformSubstitution = wra.PerformSubstitution; break; } } resourceInfo.IsDebug = resourceName.EndsWith(".debug.js", StringComparison.OrdinalIgnoreCase); // Cache the results so we don't have to do this again _scriptCache[cacheKey] = resourceInfo; } return resourceInfo; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Reflection; using System.Text; using System.Web.Resources; using System.Web.Util; internal class ScriptResourceInfo { private string _contentType; private bool _performSubstitution; private string _scriptName; private string _scriptResourceName; private string _typeName; private bool _isDebug; private static readonly IDictionary _scriptCache = Hashtable.Synchronized(new Hashtable()); private static readonly IDictionary _duplicateScriptAttributesChecked = Hashtable.Synchronized(new Hashtable()); public static readonly ScriptResourceInfo Empty = new ScriptResourceInfo(); private ScriptResourceInfo() { } public ScriptResourceInfo(ScriptResourceAttribute attr) : this() { _scriptName = attr.ScriptName; _scriptResourceName = attr.ScriptResourceName; _typeName = attr.TypeName; } public string ContentType { get { return _contentType; } set { _contentType = value; } } public bool IsDebug { get { return _isDebug; } set { _isDebug = value; } } public bool PerformSubstitution { get { return _performSubstitution; } set { _performSubstitution = value; } } public string ScriptName { get { return _scriptName; } set { _scriptName = value; } } public string ScriptResourceName { get { return _scriptResourceName; } } public string TypeName { get { return _typeName; } } public static ScriptResourceInfo GetInstance(Assembly assembly, string resourceName) { // The first time this API is called, check for attributes that point to the same script if (!_duplicateScriptAttributesChecked.Contains(assembly)) { Dictionaryscripts = new Dictionary (); foreach (ScriptResourceAttribute attr in assembly.GetCustomAttributes(typeof(ScriptResourceAttribute), false)) { string scriptName = attr.ScriptName; if (scripts.ContainsKey(scriptName)) { throw new InvalidOperationException( String.Format(CultureInfo.CurrentCulture, AtlasWeb.ScriptResourceHandler_DuplicateScriptResources, scriptName, assembly.GetName())); } scripts.Add(scriptName, true); } _duplicateScriptAttributesChecked[assembly] = true; } Pair cacheKey = new Pair (assembly, resourceName); ScriptResourceInfo resourceInfo = (ScriptResourceInfo)_scriptCache[cacheKey]; if (resourceInfo == null) { resourceInfo = ScriptResourceInfo.Empty; object[] attrs = assembly.GetCustomAttributes(typeof(ScriptResourceAttribute), false); // First look for a script resource attribute with that name for (int i = 0; i < attrs.Length; i++) { ScriptResourceAttribute sra = (ScriptResourceAttribute)attrs[i]; if (String.Equals(sra.ScriptName, resourceName, StringComparison.Ordinal)) { resourceInfo = new ScriptResourceInfo(sra); break; } } // Look for a web resource with that name, to get additional info if any attrs = assembly.GetCustomAttributes(typeof(WebResourceAttribute), false); for (int i = 0; i < attrs.Length; i++) { WebResourceAttribute wra = (WebResourceAttribute)attrs[i]; if (String.Equals(wra.WebResource, resourceName, StringComparison.Ordinal)) { if (resourceInfo == ScriptResourceInfo.Empty) { resourceInfo = new ScriptResourceInfo(); resourceInfo.ScriptName = resourceName; } resourceInfo.ContentType = wra.ContentType; resourceInfo.PerformSubstitution = wra.PerformSubstitution; break; } } resourceInfo.IsDebug = resourceName.EndsWith(".debug.js", StringComparison.OrdinalIgnoreCase); // Cache the results so we don't have to do this again _scriptCache[cacheKey] = resourceInfo; } return resourceInfo; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
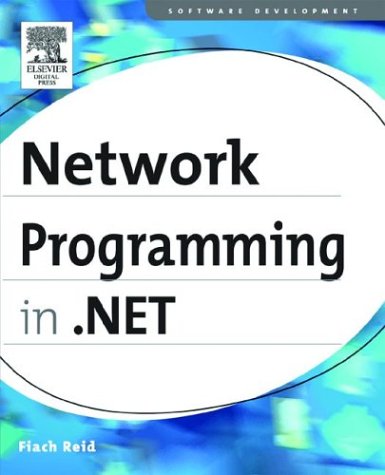
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HMACSHA384.cs
- DataGridViewMethods.cs
- DataObjectPastingEventArgs.cs
- XamlBrushSerializer.cs
- PanelStyle.cs
- WebPermission.cs
- FileLevelControlBuilderAttribute.cs
- ContentElement.cs
- ParagraphResult.cs
- BasicCellRelation.cs
- Button.cs
- ParameterCollection.cs
- SqlUserDefinedAggregateAttribute.cs
- Fonts.cs
- WebBrowserNavigatedEventHandler.cs
- _BasicClient.cs
- SafeBitVector32.cs
- SafeHandles.cs
- StateBag.cs
- RootBuilder.cs
- DataBinding.cs
- SynchronizedDispatch.cs
- CustomSignedXml.cs
- ScrollData.cs
- DateTimeSerializationSection.cs
- DecimalKeyFrameCollection.cs
- OleDbRowUpdatedEvent.cs
- ReadingWritingEntityEventArgs.cs
- RequestFactory.cs
- CodeNamespace.cs
- TableCellAutomationPeer.cs
- COM2ColorConverter.cs
- ExceptionUtil.cs
- DotExpr.cs
- XmlDataCollection.cs
- RuntimeCompatibilityAttribute.cs
- BmpBitmapEncoder.cs
- MimePart.cs
- SerializationFieldInfo.cs
- ThicknessConverter.cs
- FlowDocument.cs
- SrgsSubset.cs
- PixelFormats.cs
- MouseButton.cs
- ReferenceSchema.cs
- SourceFileBuildProvider.cs
- TransportConfigurationTypeElement.cs
- EntityViewGenerationAttribute.cs
- ActivityStatusChangeEventArgs.cs
- WriteFileContext.cs
- MouseActionValueSerializer.cs
- CodeGenerator.cs
- InvalidComObjectException.cs
- CultureInfoConverter.cs
- XmlMapping.cs
- DisplayMemberTemplateSelector.cs
- QuaternionValueSerializer.cs
- EntityDataSourceDataSelection.cs
- IProvider.cs
- SecureUICommand.cs
- UIElementHelper.cs
- OdbcConnection.cs
- StreamWithDictionary.cs
- WebSysDescriptionAttribute.cs
- TemplateManager.cs
- ClassValidator.cs
- XamlStyleSerializer.cs
- MailMessageEventArgs.cs
- IndexedString.cs
- RequiredAttributeAttribute.cs
- CharUnicodeInfo.cs
- Model3DGroup.cs
- DrawingContext.cs
- RegexReplacement.cs
- XmlDocument.cs
- ConstantCheck.cs
- MultiBinding.cs
- ActivityCodeDomSerializer.cs
- MasterPageBuildProvider.cs
- HandlerBase.cs
- HtmlAnchor.cs
- PageHandlerFactory.cs
- RawStylusActions.cs
- TargetConverter.cs
- DataGridViewTopLeftHeaderCell.cs
- MessageQueueEnumerator.cs
- SR.cs
- ContentDisposition.cs
- BaseParagraph.cs
- SqlCommandSet.cs
- SecureUICommand.cs
- HandledMouseEvent.cs
- HwndProxyElementProvider.cs
- _TLSstream.cs
- XmlSchemaAnnotated.cs
- EpmSyndicationContentDeSerializer.cs
- TrackingProfileCache.cs
- GeneratedContractType.cs
- RouteValueDictionary.cs
- UIElementHelper.cs