Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Data / XmlDataCollection.cs / 1305600 / XmlDataCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Data collection produced by an XmlDataProvider // // Specs: http://avalon/connecteddata/M5%20Specs/IDataCollection.mht // //--------------------------------------------------------------------------- using System.Xml; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Windows.Data; using System.Windows.Markup; namespace MS.Internal.Data { ////// Implementation of a data collection based on ArrayList, /// implementing INotifyCollectionChanged to notify listeners /// when items get added, removed or the whole list is refreshed. /// internal class XmlDataCollection : ReadOnlyObservableCollection{ //----------------------------------------------------- // // Constructors // //----------------------------------------------------- /// /// Initializes a new instance of XmlDataCollection that is empty and has the specified initial capacity. /// /// Parent Xml Data Source internal XmlDataCollection(XmlDataProvider xmlDataProvider) : base(new ObservableCollection()) { _xds = xmlDataProvider; } //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- /// /// XmlNamespaceManager property, XmlNamespaceManager used for executing XPath queries. /// internal XmlNamespaceManager XmlNamespaceManager { get { if (_nsMgr == null && _xds != null) _nsMgr = _xds.XmlNamespaceManager; return _nsMgr; } set { _nsMgr = value; } } internal XmlDataProvider ParentXmlDataProvider { get { return _xds; } } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ // return true if the counts are different or the identity of the nodes have changed internal bool CollectionHasChanged(XmlNodeList nodes) { int count = this.Count; if (count != nodes.Count) return true; for (int i = 0; i < count; ++i) { if (this[i] != nodes[i]) return true; } return false; } // Update the collection using new query results internal void SynchronizeCollection(XmlNodeList nodes) { if (nodes == null) { Items.Clear(); return; } int i = 0, j; while (i < this.Count && i < nodes.Count) { if (this[i] != nodes[i]) { // starting after current node, see if the old node is still in the new list. for (j = i + 1; j < nodes.Count; ++j) { if (this[i] == nodes[j]) { break; } } if (j < nodes.Count) { // the node from existing collection is found at [j] in the new collection; // this means the node(s) [i ~ j-1] in new collection should be inserted. while (i < j) { Items.Insert(i, nodes[i]); ++i; } ++i; // advance to next node } else { // the node from existing collection is no longer in // the new collection, delete it. Items.RemoveAt(i); // do not advance to the next node } } else { // nodes are the same; advance to the next node. ++i; } } // Remove any extra nodes left over in the old collection while (i < this.Count) { Items.RemoveAt(i); } // Add any extra new nodes from the new collection while (i < nodes.Count) { Items.Insert(i, nodes[i]); ++i; } } private XmlDataProvider _xds; private XmlNamespaceManager _nsMgr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Data collection produced by an XmlDataProvider // // Specs: http://avalon/connecteddata/M5%20Specs/IDataCollection.mht // //--------------------------------------------------------------------------- using System.Xml; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Windows.Data; using System.Windows.Markup; namespace MS.Internal.Data { ////// Implementation of a data collection based on ArrayList, /// implementing INotifyCollectionChanged to notify listeners /// when items get added, removed or the whole list is refreshed. /// internal class XmlDataCollection : ReadOnlyObservableCollection{ //----------------------------------------------------- // // Constructors // //----------------------------------------------------- /// /// Initializes a new instance of XmlDataCollection that is empty and has the specified initial capacity. /// /// Parent Xml Data Source internal XmlDataCollection(XmlDataProvider xmlDataProvider) : base(new ObservableCollection()) { _xds = xmlDataProvider; } //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- /// /// XmlNamespaceManager property, XmlNamespaceManager used for executing XPath queries. /// internal XmlNamespaceManager XmlNamespaceManager { get { if (_nsMgr == null && _xds != null) _nsMgr = _xds.XmlNamespaceManager; return _nsMgr; } set { _nsMgr = value; } } internal XmlDataProvider ParentXmlDataProvider { get { return _xds; } } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ // return true if the counts are different or the identity of the nodes have changed internal bool CollectionHasChanged(XmlNodeList nodes) { int count = this.Count; if (count != nodes.Count) return true; for (int i = 0; i < count; ++i) { if (this[i] != nodes[i]) return true; } return false; } // Update the collection using new query results internal void SynchronizeCollection(XmlNodeList nodes) { if (nodes == null) { Items.Clear(); return; } int i = 0, j; while (i < this.Count && i < nodes.Count) { if (this[i] != nodes[i]) { // starting after current node, see if the old node is still in the new list. for (j = i + 1; j < nodes.Count; ++j) { if (this[i] == nodes[j]) { break; } } if (j < nodes.Count) { // the node from existing collection is found at [j] in the new collection; // this means the node(s) [i ~ j-1] in new collection should be inserted. while (i < j) { Items.Insert(i, nodes[i]); ++i; } ++i; // advance to next node } else { // the node from existing collection is no longer in // the new collection, delete it. Items.RemoveAt(i); // do not advance to the next node } } else { // nodes are the same; advance to the next node. ++i; } } // Remove any extra nodes left over in the old collection while (i < this.Count) { Items.RemoveAt(i); } // Add any extra new nodes from the new collection while (i < nodes.Count) { Items.Insert(i, nodes[i]); ++i; } } private XmlDataProvider _xds; private XmlNamespaceManager _nsMgr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
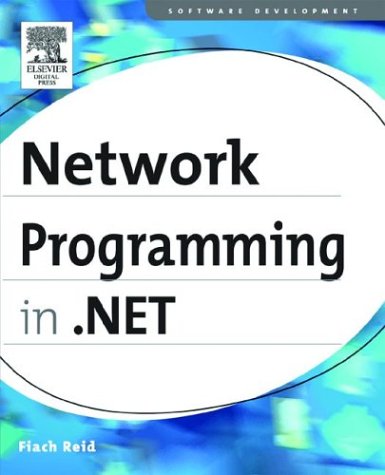
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ViewValidator.cs
- FlowLayout.cs
- ConfigurationSettings.cs
- ValidatingReaderNodeData.cs
- RemoteWebConfigurationHostServer.cs
- TiffBitmapDecoder.cs
- BitmapData.cs
- SamlAttributeStatement.cs
- ReadOnlyHierarchicalDataSource.cs
- FunctionNode.cs
- PaperSource.cs
- UrlEncodedParameterWriter.cs
- BasicKeyConstraint.cs
- AnnotationService.cs
- ComponentChangedEvent.cs
- PaperSize.cs
- GridViewDeletedEventArgs.cs
- XsdBuildProvider.cs
- CodeDomConfigurationHandler.cs
- Brush.cs
- Directory.cs
- RotateTransform.cs
- TreeNodeClickEventArgs.cs
- DesignerActionUIService.cs
- Stream.cs
- HwndMouseInputProvider.cs
- Page.cs
- ProcessHostFactoryHelper.cs
- RelatedCurrencyManager.cs
- ResourcesBuildProvider.cs
- ObjectDataSourceMethodEventArgs.cs
- CallContext.cs
- ContentTextAutomationPeer.cs
- AppDomainManager.cs
- XpsFixedPageReaderWriter.cs
- BamlRecordReader.cs
- Error.cs
- WindowsAuthenticationModule.cs
- TreeNodeStyle.cs
- SecurityResources.cs
- BamlRecordHelper.cs
- DependencyPropertyChangedEventArgs.cs
- BamlLocalizationDictionary.cs
- SqlDependencyUtils.cs
- BaseValidator.cs
- ProfileSettings.cs
- MouseActionConverter.cs
- JoinGraph.cs
- SQLDateTime.cs
- PropertyValue.cs
- StaticSiteMapProvider.cs
- AdornerDecorator.cs
- QilIterator.cs
- CharConverter.cs
- PersistencePipeline.cs
- Translator.cs
- TableItemStyle.cs
- TabItemWrapperAutomationPeer.cs
- ErrorProvider.cs
- SpellerStatusTable.cs
- AsnEncodedData.cs
- ColumnResizeUndoUnit.cs
- PropertyCondition.cs
- ITreeGenerator.cs
- SafeSystemMetrics.cs
- VarRemapper.cs
- AppDomainProtocolHandler.cs
- AvtEvent.cs
- TableLayoutPanelCodeDomSerializer.cs
- LinkedList.cs
- ComplexTypeEmitter.cs
- InheritanceContextHelper.cs
- SHA512Managed.cs
- AuthenticationSection.cs
- CurrentChangingEventManager.cs
- ObjectListTitleAttribute.cs
- WriterOutput.cs
- URLIdentityPermission.cs
- SecurityResources.cs
- COM2TypeInfoProcessor.cs
- InkCanvasAutomationPeer.cs
- ValidationService.cs
- errorpatternmatcher.cs
- DataGridTable.cs
- SharedDp.cs
- SafeViewOfFileHandle.cs
- _KerberosClient.cs
- TableAdapterManagerNameHandler.cs
- SharedPersonalizationStateInfo.cs
- UserPreferenceChangingEventArgs.cs
- PackagingUtilities.cs
- CodeSnippetTypeMember.cs
- OperationCanceledException.cs
- LongValidator.cs
- SoapIgnoreAttribute.cs
- ZipIOExtraFieldElement.cs
- NativeMethods.cs
- LogRecordSequence.cs
- ColorAnimationBase.cs
- BrowserCapabilitiesCompiler.cs