Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / schema / XmlSchemaParticle.cs / 1 / XmlSchemaParticle.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Xml.Serialization; ////// /// public abstract class XmlSchemaParticle : XmlSchemaAnnotated { [Flags] enum Occurs { None, Min, Max }; decimal minOccurs = decimal.One; decimal maxOccurs = decimal.One; Occurs flags = Occurs.None; ///[To be supplied.] ////// /// [XmlAttribute("minOccurs")] public string MinOccursString { get { return (flags & Occurs.Min) == 0 ? null : XmlConvert.ToString(minOccurs); } set { if (value == null) { minOccurs = decimal.One; flags &= ~Occurs.Min; } else { minOccurs = XmlConvert.ToInteger(value); if (minOccurs < decimal.Zero) { throw new XmlSchemaException(Res.Sch_MinOccursInvalidXsd, string.Empty); } flags |= Occurs.Min; } } } ///[To be supplied.] ////// /// [XmlAttribute("maxOccurs")] public string MaxOccursString { get { return (flags & Occurs.Max) == 0 ? null : (maxOccurs == decimal.MaxValue) ? "unbounded" : XmlConvert.ToString(maxOccurs); } set { if (value == null) { maxOccurs = decimal.One; flags &= ~Occurs.Max; } else { if (value == "unbounded") { maxOccurs = decimal.MaxValue; } else { maxOccurs = XmlConvert.ToInteger(value); if (maxOccurs < decimal.Zero) { throw new XmlSchemaException(Res.Sch_MaxOccursInvalidXsd, string.Empty); } else if (maxOccurs == decimal.Zero && (flags & Occurs.Min) == 0) { minOccurs = decimal.Zero; } } flags |= Occurs.Max; } } } ///[To be supplied.] ////// /// [XmlIgnore] public decimal MinOccurs { get { return minOccurs; } set { if (value < decimal.Zero || value != decimal.Truncate(value)) { throw new XmlSchemaException(Res.Sch_MinOccursInvalidXsd, string.Empty); } minOccurs = value; flags |= Occurs.Min; } } ///[To be supplied.] ////// /// [XmlIgnore] public decimal MaxOccurs { get { return maxOccurs; } set { if (value < decimal.Zero || value != decimal.Truncate(value)) { throw new XmlSchemaException(Res.Sch_MaxOccursInvalidXsd, string.Empty); } maxOccurs = value; if (maxOccurs == decimal.Zero && (flags & Occurs.Min) == 0) { minOccurs = decimal.Zero; } flags |= Occurs.Max; } } internal virtual bool IsEmpty { get { return maxOccurs == decimal.Zero; } } internal bool IsMultipleOccurrence { get { return maxOccurs > decimal.One; } } internal virtual string NameString { get { return string.Empty; } } internal XmlQualifiedName GetQualifiedName() { XmlSchemaElement elem = this as XmlSchemaElement; if (elem != null) { return elem.QualifiedName; } else { XmlSchemaAny any = this as XmlSchemaAny; if (any != null) { string ns = any.Namespace; if (ns != null) { ns = ns.Trim(); } else { ns = string.Empty; } return new XmlQualifiedName("*", ns.Length == 0 ? "##any" : ns); } } return XmlQualifiedName.Empty; //If ever called on other particles } class EmptyParticle : XmlSchemaParticle { internal override bool IsEmpty { get { return true; } } } internal static readonly XmlSchemaParticle Empty = new EmptyParticle(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Xml.Serialization; ////// /// public abstract class XmlSchemaParticle : XmlSchemaAnnotated { [Flags] enum Occurs { None, Min, Max }; decimal minOccurs = decimal.One; decimal maxOccurs = decimal.One; Occurs flags = Occurs.None; ///[To be supplied.] ////// /// [XmlAttribute("minOccurs")] public string MinOccursString { get { return (flags & Occurs.Min) == 0 ? null : XmlConvert.ToString(minOccurs); } set { if (value == null) { minOccurs = decimal.One; flags &= ~Occurs.Min; } else { minOccurs = XmlConvert.ToInteger(value); if (minOccurs < decimal.Zero) { throw new XmlSchemaException(Res.Sch_MinOccursInvalidXsd, string.Empty); } flags |= Occurs.Min; } } } ///[To be supplied.] ////// /// [XmlAttribute("maxOccurs")] public string MaxOccursString { get { return (flags & Occurs.Max) == 0 ? null : (maxOccurs == decimal.MaxValue) ? "unbounded" : XmlConvert.ToString(maxOccurs); } set { if (value == null) { maxOccurs = decimal.One; flags &= ~Occurs.Max; } else { if (value == "unbounded") { maxOccurs = decimal.MaxValue; } else { maxOccurs = XmlConvert.ToInteger(value); if (maxOccurs < decimal.Zero) { throw new XmlSchemaException(Res.Sch_MaxOccursInvalidXsd, string.Empty); } else if (maxOccurs == decimal.Zero && (flags & Occurs.Min) == 0) { minOccurs = decimal.Zero; } } flags |= Occurs.Max; } } } ///[To be supplied.] ////// /// [XmlIgnore] public decimal MinOccurs { get { return minOccurs; } set { if (value < decimal.Zero || value != decimal.Truncate(value)) { throw new XmlSchemaException(Res.Sch_MinOccursInvalidXsd, string.Empty); } minOccurs = value; flags |= Occurs.Min; } } ///[To be supplied.] ////// /// [XmlIgnore] public decimal MaxOccurs { get { return maxOccurs; } set { if (value < decimal.Zero || value != decimal.Truncate(value)) { throw new XmlSchemaException(Res.Sch_MaxOccursInvalidXsd, string.Empty); } maxOccurs = value; if (maxOccurs == decimal.Zero && (flags & Occurs.Min) == 0) { minOccurs = decimal.Zero; } flags |= Occurs.Max; } } internal virtual bool IsEmpty { get { return maxOccurs == decimal.Zero; } } internal bool IsMultipleOccurrence { get { return maxOccurs > decimal.One; } } internal virtual string NameString { get { return string.Empty; } } internal XmlQualifiedName GetQualifiedName() { XmlSchemaElement elem = this as XmlSchemaElement; if (elem != null) { return elem.QualifiedName; } else { XmlSchemaAny any = this as XmlSchemaAny; if (any != null) { string ns = any.Namespace; if (ns != null) { ns = ns.Trim(); } else { ns = string.Empty; } return new XmlQualifiedName("*", ns.Length == 0 ? "##any" : ns); } } return XmlQualifiedName.Empty; //If ever called on other particles } class EmptyParticle : XmlSchemaParticle { internal override bool IsEmpty { get { return true; } } } internal static readonly XmlSchemaParticle Empty = new EmptyParticle(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
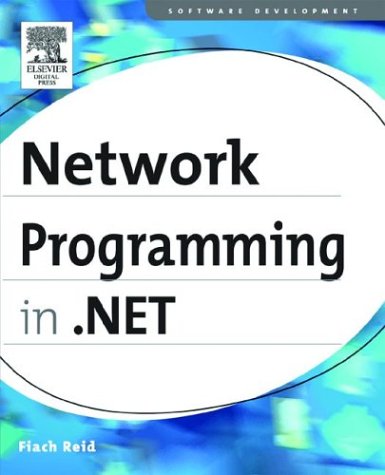
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WSHttpSecurityElement.cs
- TabControlCancelEvent.cs
- TemplatedAdorner.cs
- SqlUnionizer.cs
- HttpCapabilitiesSectionHandler.cs
- ColumnClickEvent.cs
- Types.cs
- CompilerLocalReference.cs
- HostedTransportConfigurationBase.cs
- RealizedColumnsBlock.cs
- DoubleCollectionConverter.cs
- ReadOnlyDataSource.cs
- CookieProtection.cs
- Action.cs
- RowBinding.cs
- SerTrace.cs
- ReferentialConstraintRoleElement.cs
- XhtmlTextWriter.cs
- PrintingPermissionAttribute.cs
- ExtendedPropertyInfo.cs
- TextBoxBase.cs
- SpellerInterop.cs
- ImageAnimator.cs
- ZipIOFileItemStream.cs
- NullableDecimalSumAggregationOperator.cs
- exports.cs
- QueryCursorEventArgs.cs
- MinMaxParagraphWidth.cs
- ComponentEvent.cs
- TableLayoutSettingsTypeConverter.cs
- DSASignatureDeformatter.cs
- AuthenticationModulesSection.cs
- SamlAttribute.cs
- webproxy.cs
- StylusPointPropertyId.cs
- URLMembershipCondition.cs
- x509utils.cs
- IPCCacheManager.cs
- COM2PropertyBuilderUITypeEditor.cs
- Selector.cs
- XmlSignatureProperties.cs
- MessageDescription.cs
- TraceRecord.cs
- ColorTransform.cs
- GlobalizationSection.cs
- ComponentEditorPage.cs
- OSEnvironmentHelper.cs
- EnumUnknown.cs
- NonVisualControlAttribute.cs
- PackWebRequestFactory.cs
- DataGridViewBindingCompleteEventArgs.cs
- QilUnary.cs
- TcpSocketManager.cs
- WebPartConnectionsEventArgs.cs
- XmlAtomicValue.cs
- SimpleHandlerBuildProvider.cs
- Timeline.cs
- WindowsScrollBar.cs
- TabRenderer.cs
- NativeMethods.cs
- XmlSchemaInferenceException.cs
- PanelDesigner.cs
- IApplicationTrustManager.cs
- SegmentInfo.cs
- SystemColorTracker.cs
- DataService.cs
- ExtendedPropertyInfo.cs
- QuadTree.cs
- XmlSchemaComplexContent.cs
- CompModSwitches.cs
- StatusBarPanel.cs
- XamlToRtfWriter.cs
- XmlAnyElementAttribute.cs
- DataRowCollection.cs
- PageCatalogPart.cs
- EmptyImpersonationContext.cs
- BooleanConverter.cs
- DesignerActionItem.cs
- NonVisualControlAttribute.cs
- RequestCachingSection.cs
- DateTimeConverter2.cs
- _NestedSingleAsyncResult.cs
- DateTimeAutomationPeer.cs
- ToolStripGripRenderEventArgs.cs
- Int16.cs
- ToolStripContainer.cs
- DependencyPropertyKey.cs
- UTF8Encoding.cs
- DataGridViewTextBoxCell.cs
- NamedObject.cs
- MultipartIdentifier.cs
- GC.cs
- RightNameExpirationInfoPair.cs
- GenericPrincipal.cs
- CombinedGeometry.cs
- ObjectDataSourceChooseMethodsPanel.cs
- ProviderUtil.cs
- Label.cs
- oledbconnectionstring.cs
- SchemaImporter.cs