Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / DateTimeAutomationPeer.cs / 1305600 / DateTimeAutomationPeer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Input; using System.Windows.Threading; namespace System.Windows.Automation.Peers { ////// AutomationPeer for CalendarDayButton and CalendarButton /// public sealed class DateTimeAutomationPeer : AutomationPeer, IGridItemProvider, ISelectionItemProvider, ITableItemProvider, IInvokeProvider , IVirtualizedItemProvider { ////// Initializes a new instance of the DateTimeAutomationPeer class. /// /// /// /// internal DateTimeAutomationPeer(DateTime date, Calendar owningCalendar, CalendarMode buttonMode) : base() { if (date == null) { throw new ArgumentNullException("date"); } if (owningCalendar == null) { throw new ArgumentNullException("owningCalendar"); } Date = date; ButtonMode = buttonMode; OwningCalendar = owningCalendar; } /// internal override bool AncestorsInvalid { get { return base.AncestorsInvalid; } set { base.AncestorsInvalid = value; if (value) return; AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { wrapperPeer.AncestorsInvalid = false; } } } #region Private Properties private Calendar OwningCalendar { get; set; } internal DateTime Date { get; private set; } internal CalendarMode ButtonMode { get; private set; } internal bool IsDayButton { get { return ButtonMode == CalendarMode.Month; } } private IRawElementProviderSimple OwningCalendarProvider { get { if (this.OwningCalendar != null) { AutomationPeer peer = FrameworkElementAutomationPeer.CreatePeerForElement(this.OwningCalendar); if (peer != null) { return ProviderFromPeer(peer); } } return null; } } internal Button OwningButton { get { if (OwningCalendar.DisplayMode != ButtonMode) { return null; } if (IsDayButton) { return OwningCalendar.MonthControl.GetCalendarDayButton(this.Date); } else { return OwningCalendar.MonthControl.GetCalendarButton(this.Date, ButtonMode); } } } internal FrameworkElementAutomationPeer WrapperPeer { get { Button owningButton = OwningButton; if (owningButton != null) { return FrameworkElementAutomationPeer.CreatePeerForElement(owningButton) as FrameworkElementAutomationPeer; } return null; } } #endregion Private Properties #region AutomationPeer override Methods protected override string GetAcceleratorKeyCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetAcceleratorKey(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override string GetAccessKeyCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetAccessKey(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Button; } protected override string GetAutomationIdCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetAutomationId(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override Rect GetBoundingRectangleCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetBoundingRectangle(); } else { ThrowElementNotAvailableException(); } return new Rect(); } protected override ListGetChildrenCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetChildren(); } else { ThrowElementNotAvailableException(); } return null; } protected override string GetClassNameCore() { AutomationPeer wrapperPeer = WrapperPeer; return (wrapperPeer != null) ? wrapperPeer.GetClassName() : (IsDayButton)? "CalendarDayButton" : "CalendarButton"; } protected override Point GetClickablePointCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetClickablePoint(); } else { ThrowElementNotAvailableException(); } return new Point(double.NaN, double.NaN); } protected override string GetHelpTextCore() { string dateString = DateTimeHelper.ToLongDateString(Date, DateTimeHelper.GetCulture(OwningCalendar)); if (IsDayButton && this.OwningCalendar.BlackoutDates.Contains(Date)) { return string.Format(DateTimeHelper.GetCurrentDateFormat(), SR.Get(SRID.CalendarAutomationPeer_BlackoutDayHelpText), dateString); } return dateString; } protected override string GetItemStatusCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetItemStatus(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override string GetItemTypeCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetItemType(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override AutomationPeer GetLabeledByCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetLabeledBy(); } else { ThrowElementNotAvailableException(); } return null; } protected override string GetLocalizedControlTypeCore() { return IsDayButton ? SR.Get(SRID.CalendarAutomationPeer_DayButtonLocalizedControlType) : SR.Get(SRID.CalendarAutomationPeer_CalendarButtonLocalizedControlType); } protected override string GetNameCore() { string dateString = ""; switch (ButtonMode) { case CalendarMode.Month: dateString = DateTimeHelper.ToLongDateString(Date, DateTimeHelper.GetCulture(OwningCalendar)); break; case CalendarMode.Year: dateString = DateTimeHelper.ToYearMonthPatternString(Date, DateTimeHelper.GetCulture(OwningCalendar)); break; case CalendarMode.Decade: dateString = DateTimeHelper.ToYearString(Date, DateTimeHelper.GetCulture(OwningCalendar)); break; } return dateString; } protected override AutomationOrientation GetOrientationCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetOrientation(); } else { ThrowElementNotAvailableException(); } return AutomationOrientation.None; } /// /// Gets the control pattern that is associated with the specified System.Windows.Automation.Peers.PatternInterface. /// /// A value from the System.Windows.Automation.Peers.PatternInterface enumeration. ///The object that supports the specified pattern, or null if unsupported. public override object GetPattern(PatternInterface patternInterface) { object result = null; Button owningButton = OwningButton; switch (patternInterface) { case PatternInterface.Invoke: case PatternInterface.GridItem: { if (owningButton != null) { result = this; } break; } case PatternInterface.TableItem: { if (IsDayButton && owningButton != null) { result = this; } break; } case PatternInterface.SelectionItem: { result = this; break; } case PatternInterface.VirtualizedItem: if (VirtualizedItemPatternIdentifiers.Pattern != null) { if (owningButton == null) { result = this; } else { // If the Item is in Automation Tree we consider it Realized and need not return VirtualizedItem pattern. if (!IsItemInAutomationTree()) { return this; } } } break; default: break; } return result; } internal override Rect GetVisibleBoundingRectCore() { AutomationPeer wrapperPeer = WrapperPeer; return (wrapperPeer != null) ? wrapperPeer.GetVisibleBoundingRect() : GetBoundingRectangle(); } protected override bool HasKeyboardFocusCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.HasKeyboardFocus(); } else { ThrowElementNotAvailableException(); } return false; } protected override bool IsContentElementCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsContentElement(); } else { ThrowElementNotAvailableException(); } return true; } protected override bool IsControlElementCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsControlElement(); } else { ThrowElementNotAvailableException(); } return true; } protected override bool IsEnabledCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsEnabled(); } else { ThrowElementNotAvailableException(); } return false; } protected override bool IsKeyboardFocusableCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsKeyboardFocusable(); } else { ThrowElementNotAvailableException(); } return false; } protected override bool IsOffscreenCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsOffscreen(); } else { ThrowElementNotAvailableException(); } return true; } protected override bool IsPasswordCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsPassword(); } else { ThrowElementNotAvailableException(); } return false; } protected override bool IsRequiredForFormCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsRequiredForForm(); } else { ThrowElementNotAvailableException(); } return false; } protected override void SetFocusCore() { UIElementAutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { wrapperPeer.SetFocus(); } else { ThrowElementNotAvailableException(); } } #endregion AutomationPeer override Methods #region IGridItemProvider ////// Grid item column. /// int IGridItemProvider.Column { get { Button owningButton = OwningButton; if (owningButton != null) { return (int)owningButton.GetValue(Grid.ColumnProperty); } else { throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } } } ////// Grid item column span. /// int IGridItemProvider.ColumnSpan { get { Button owningButton = OwningButton; if (owningButton != null) { return (int)owningButton.GetValue(Grid.ColumnSpanProperty); } else { throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } } } ////// Grid item's containing grid. /// IRawElementProviderSimple IGridItemProvider.ContainingGrid { get { return this.OwningCalendarProvider; } } ////// Grid item row. /// int IGridItemProvider.Row { get { Button owningButton = OwningButton; if (owningButton != null) { if (IsDayButton) { Debug.Assert((int)owningButton.GetValue(Grid.RowProperty) > 0); // we decrement the Row value by one since the first row is composed of DayTitles return (int)owningButton.GetValue(Grid.RowProperty) - 1; } else { return (int)owningButton.GetValue(Grid.RowProperty); } } else { throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } } } ////// Grid item row span. /// int IGridItemProvider.RowSpan { get { Button owningButton = OwningButton; if (owningButton != null) { if (IsDayButton) { return (int)owningButton.GetValue(Grid.RowSpanProperty); } else { return 1; } } else { throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } } } #endregion IGridItemProvider #region ISelectionItemProvider ////// True if the owning CalendarDayButton is selected. /// bool ISelectionItemProvider.IsSelected { get { if (IsDayButton) { return this.OwningCalendar.SelectedDates.Contains(Date); } return false; } } ////// Selection items selection container. /// IRawElementProviderSimple ISelectionItemProvider.SelectionContainer { get { return this.OwningCalendarProvider; } } ////// Adds selection item to selection. /// void ISelectionItemProvider.AddToSelection() { // Return if the day is already selected or if it is a BlackoutDate if (((ISelectionItemProvider)this).IsSelected) { return; } if (IsDayButton && EnsureSelection()) { if (this.OwningCalendar.SelectionMode == CalendarSelectionMode.SingleDate) { this.OwningCalendar.SelectedDate = Date; } else { this.OwningCalendar.SelectedDates.Add(Date); } } return; } ////// Removes selection item from selection. /// void ISelectionItemProvider.RemoveFromSelection() { // Return if the item is not already selected. if (!((ISelectionItemProvider)this).IsSelected) { return; } if (IsDayButton) { this.OwningCalendar.SelectedDates.Remove(Date); } return; } ////// Selects this item. /// void ISelectionItemProvider.Select() { Button owningButton = OwningButton; if (IsDayButton) { if (EnsureSelection() && this.OwningCalendar.SelectionMode == CalendarSelectionMode.SingleDate) { this.OwningCalendar.SelectedDate = Date; } } else if (owningButton != null && owningButton.IsEnabled) { owningButton.Focus(); } } #endregion ISelectionItemProvider #region ITableItemProvider ////// Gets the table item's column headers. /// ///The table item's column headers IRawElementProviderSimple[] ITableItemProvider.GetColumnHeaderItems() { if (IsDayButton && OwningButton != null) { if (this.OwningCalendar != null && this.OwningCalendarProvider != null) { IRawElementProviderSimple[] headers = ((ITableProvider)FrameworkElementAutomationPeer.CreatePeerForElement(this.OwningCalendar)).GetColumnHeaders(); if (headers != null) { int column = ((IGridItemProvider)this).Column; return new IRawElementProviderSimple[] { headers[column] }; } } } return null; } ////// Get's the table item's row headers. /// ///The table item's row headers IRawElementProviderSimple[] ITableItemProvider.GetRowHeaderItems() { return null; } #endregion ITableItemProvider #region IInvokeProvider void IInvokeProvider.Invoke() { Button owningButton = OwningButton; if (owningButton == null || !this.IsEnabled()) throw new ElementNotEnabledException(); // Async call of click event // In ClickHandler opens a dialog and suspend the execution we don't want to block this thread Dispatcher.BeginInvoke(DispatcherPriority.Input, new DispatcherOperationCallback(delegate(object param) { owningButton.AutomationButtonBaseClick(); return null; }), null); } #endregion IInvokeProvider # region IVirtualizedItemProvider void IVirtualizedItemProvider.Realize() { // Change Display mode if (OwningCalendar.DisplayMode != ButtonMode) { OwningCalendar.DisplayMode = ButtonMode; } // Bring into view OwningCalendar.DisplayDate = this.Date; } #endregion IVirtualizedItemProvider override internal bool IsDataItemAutomationPeer() { return true; } override internal void AddToParentProxyWeakRefCache() { CalendarAutomationPeer owningCalendarPeer = FrameworkElementAutomationPeer.CreatePeerForElement(OwningCalendar) as CalendarAutomationPeer; if (owningCalendarPeer != null) { owningCalendarPeer.AddProxyToWeakRefStorage(this.ElementProxyWeakReference, this); } } #region Private Methods private bool EnsureSelection() { // If the day is a blackout day or the SelectionMode is None, selection is not allowed if (this.OwningCalendar.BlackoutDates.Contains(Date) || this.OwningCalendar.SelectionMode == CalendarSelectionMode.None) { return false; } return true; } private bool IsItemInAutomationTree() { AutomationPeer parent = this.GetParent(); if (this.Index != -1 && parent != null && parent.Children != null && this.Index < parent.Children.Count && parent.Children[this.Index] == this) return true; else return false; } private void ThrowElementNotAvailableException() { // To avoid the situation on legacy systems which may not have new unmanaged core. this check with old unmanaged core // avoids throwing exception and provide older behavior returning default values for items which are virtualized rather than throwing exception. if (VirtualizedItemPatternIdentifiers.Pattern != null) throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Input; using System.Windows.Threading; namespace System.Windows.Automation.Peers { ////// AutomationPeer for CalendarDayButton and CalendarButton /// public sealed class DateTimeAutomationPeer : AutomationPeer, IGridItemProvider, ISelectionItemProvider, ITableItemProvider, IInvokeProvider , IVirtualizedItemProvider { ////// Initializes a new instance of the DateTimeAutomationPeer class. /// /// /// /// internal DateTimeAutomationPeer(DateTime date, Calendar owningCalendar, CalendarMode buttonMode) : base() { if (date == null) { throw new ArgumentNullException("date"); } if (owningCalendar == null) { throw new ArgumentNullException("owningCalendar"); } Date = date; ButtonMode = buttonMode; OwningCalendar = owningCalendar; } /// internal override bool AncestorsInvalid { get { return base.AncestorsInvalid; } set { base.AncestorsInvalid = value; if (value) return; AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { wrapperPeer.AncestorsInvalid = false; } } } #region Private Properties private Calendar OwningCalendar { get; set; } internal DateTime Date { get; private set; } internal CalendarMode ButtonMode { get; private set; } internal bool IsDayButton { get { return ButtonMode == CalendarMode.Month; } } private IRawElementProviderSimple OwningCalendarProvider { get { if (this.OwningCalendar != null) { AutomationPeer peer = FrameworkElementAutomationPeer.CreatePeerForElement(this.OwningCalendar); if (peer != null) { return ProviderFromPeer(peer); } } return null; } } internal Button OwningButton { get { if (OwningCalendar.DisplayMode != ButtonMode) { return null; } if (IsDayButton) { return OwningCalendar.MonthControl.GetCalendarDayButton(this.Date); } else { return OwningCalendar.MonthControl.GetCalendarButton(this.Date, ButtonMode); } } } internal FrameworkElementAutomationPeer WrapperPeer { get { Button owningButton = OwningButton; if (owningButton != null) { return FrameworkElementAutomationPeer.CreatePeerForElement(owningButton) as FrameworkElementAutomationPeer; } return null; } } #endregion Private Properties #region AutomationPeer override Methods protected override string GetAcceleratorKeyCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetAcceleratorKey(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override string GetAccessKeyCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetAccessKey(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Button; } protected override string GetAutomationIdCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetAutomationId(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override Rect GetBoundingRectangleCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetBoundingRectangle(); } else { ThrowElementNotAvailableException(); } return new Rect(); } protected override ListGetChildrenCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetChildren(); } else { ThrowElementNotAvailableException(); } return null; } protected override string GetClassNameCore() { AutomationPeer wrapperPeer = WrapperPeer; return (wrapperPeer != null) ? wrapperPeer.GetClassName() : (IsDayButton)? "CalendarDayButton" : "CalendarButton"; } protected override Point GetClickablePointCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetClickablePoint(); } else { ThrowElementNotAvailableException(); } return new Point(double.NaN, double.NaN); } protected override string GetHelpTextCore() { string dateString = DateTimeHelper.ToLongDateString(Date, DateTimeHelper.GetCulture(OwningCalendar)); if (IsDayButton && this.OwningCalendar.BlackoutDates.Contains(Date)) { return string.Format(DateTimeHelper.GetCurrentDateFormat(), SR.Get(SRID.CalendarAutomationPeer_BlackoutDayHelpText), dateString); } return dateString; } protected override string GetItemStatusCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetItemStatus(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override string GetItemTypeCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetItemType(); } else { ThrowElementNotAvailableException(); } return String.Empty; } protected override AutomationPeer GetLabeledByCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetLabeledBy(); } else { ThrowElementNotAvailableException(); } return null; } protected override string GetLocalizedControlTypeCore() { return IsDayButton ? SR.Get(SRID.CalendarAutomationPeer_DayButtonLocalizedControlType) : SR.Get(SRID.CalendarAutomationPeer_CalendarButtonLocalizedControlType); } protected override string GetNameCore() { string dateString = ""; switch (ButtonMode) { case CalendarMode.Month: dateString = DateTimeHelper.ToLongDateString(Date, DateTimeHelper.GetCulture(OwningCalendar)); break; case CalendarMode.Year: dateString = DateTimeHelper.ToYearMonthPatternString(Date, DateTimeHelper.GetCulture(OwningCalendar)); break; case CalendarMode.Decade: dateString = DateTimeHelper.ToYearString(Date, DateTimeHelper.GetCulture(OwningCalendar)); break; } return dateString; } protected override AutomationOrientation GetOrientationCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.GetOrientation(); } else { ThrowElementNotAvailableException(); } return AutomationOrientation.None; } /// /// Gets the control pattern that is associated with the specified System.Windows.Automation.Peers.PatternInterface. /// /// A value from the System.Windows.Automation.Peers.PatternInterface enumeration. ///The object that supports the specified pattern, or null if unsupported. public override object GetPattern(PatternInterface patternInterface) { object result = null; Button owningButton = OwningButton; switch (patternInterface) { case PatternInterface.Invoke: case PatternInterface.GridItem: { if (owningButton != null) { result = this; } break; } case PatternInterface.TableItem: { if (IsDayButton && owningButton != null) { result = this; } break; } case PatternInterface.SelectionItem: { result = this; break; } case PatternInterface.VirtualizedItem: if (VirtualizedItemPatternIdentifiers.Pattern != null) { if (owningButton == null) { result = this; } else { // If the Item is in Automation Tree we consider it Realized and need not return VirtualizedItem pattern. if (!IsItemInAutomationTree()) { return this; } } } break; default: break; } return result; } internal override Rect GetVisibleBoundingRectCore() { AutomationPeer wrapperPeer = WrapperPeer; return (wrapperPeer != null) ? wrapperPeer.GetVisibleBoundingRect() : GetBoundingRectangle(); } protected override bool HasKeyboardFocusCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.HasKeyboardFocus(); } else { ThrowElementNotAvailableException(); } return false; } protected override bool IsContentElementCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsContentElement(); } else { ThrowElementNotAvailableException(); } return true; } protected override bool IsControlElementCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsControlElement(); } else { ThrowElementNotAvailableException(); } return true; } protected override bool IsEnabledCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsEnabled(); } else { ThrowElementNotAvailableException(); } return false; } protected override bool IsKeyboardFocusableCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsKeyboardFocusable(); } else { ThrowElementNotAvailableException(); } return false; } protected override bool IsOffscreenCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsOffscreen(); } else { ThrowElementNotAvailableException(); } return true; } protected override bool IsPasswordCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsPassword(); } else { ThrowElementNotAvailableException(); } return false; } protected override bool IsRequiredForFormCore() { AutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { return wrapperPeer.IsRequiredForForm(); } else { ThrowElementNotAvailableException(); } return false; } protected override void SetFocusCore() { UIElementAutomationPeer wrapperPeer = WrapperPeer; if (wrapperPeer != null) { wrapperPeer.SetFocus(); } else { ThrowElementNotAvailableException(); } } #endregion AutomationPeer override Methods #region IGridItemProvider ////// Grid item column. /// int IGridItemProvider.Column { get { Button owningButton = OwningButton; if (owningButton != null) { return (int)owningButton.GetValue(Grid.ColumnProperty); } else { throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } } } ////// Grid item column span. /// int IGridItemProvider.ColumnSpan { get { Button owningButton = OwningButton; if (owningButton != null) { return (int)owningButton.GetValue(Grid.ColumnSpanProperty); } else { throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } } } ////// Grid item's containing grid. /// IRawElementProviderSimple IGridItemProvider.ContainingGrid { get { return this.OwningCalendarProvider; } } ////// Grid item row. /// int IGridItemProvider.Row { get { Button owningButton = OwningButton; if (owningButton != null) { if (IsDayButton) { Debug.Assert((int)owningButton.GetValue(Grid.RowProperty) > 0); // we decrement the Row value by one since the first row is composed of DayTitles return (int)owningButton.GetValue(Grid.RowProperty) - 1; } else { return (int)owningButton.GetValue(Grid.RowProperty); } } else { throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } } } ////// Grid item row span. /// int IGridItemProvider.RowSpan { get { Button owningButton = OwningButton; if (owningButton != null) { if (IsDayButton) { return (int)owningButton.GetValue(Grid.RowSpanProperty); } else { return 1; } } else { throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } } } #endregion IGridItemProvider #region ISelectionItemProvider ////// True if the owning CalendarDayButton is selected. /// bool ISelectionItemProvider.IsSelected { get { if (IsDayButton) { return this.OwningCalendar.SelectedDates.Contains(Date); } return false; } } ////// Selection items selection container. /// IRawElementProviderSimple ISelectionItemProvider.SelectionContainer { get { return this.OwningCalendarProvider; } } ////// Adds selection item to selection. /// void ISelectionItemProvider.AddToSelection() { // Return if the day is already selected or if it is a BlackoutDate if (((ISelectionItemProvider)this).IsSelected) { return; } if (IsDayButton && EnsureSelection()) { if (this.OwningCalendar.SelectionMode == CalendarSelectionMode.SingleDate) { this.OwningCalendar.SelectedDate = Date; } else { this.OwningCalendar.SelectedDates.Add(Date); } } return; } ////// Removes selection item from selection. /// void ISelectionItemProvider.RemoveFromSelection() { // Return if the item is not already selected. if (!((ISelectionItemProvider)this).IsSelected) { return; } if (IsDayButton) { this.OwningCalendar.SelectedDates.Remove(Date); } return; } ////// Selects this item. /// void ISelectionItemProvider.Select() { Button owningButton = OwningButton; if (IsDayButton) { if (EnsureSelection() && this.OwningCalendar.SelectionMode == CalendarSelectionMode.SingleDate) { this.OwningCalendar.SelectedDate = Date; } } else if (owningButton != null && owningButton.IsEnabled) { owningButton.Focus(); } } #endregion ISelectionItemProvider #region ITableItemProvider ////// Gets the table item's column headers. /// ///The table item's column headers IRawElementProviderSimple[] ITableItemProvider.GetColumnHeaderItems() { if (IsDayButton && OwningButton != null) { if (this.OwningCalendar != null && this.OwningCalendarProvider != null) { IRawElementProviderSimple[] headers = ((ITableProvider)FrameworkElementAutomationPeer.CreatePeerForElement(this.OwningCalendar)).GetColumnHeaders(); if (headers != null) { int column = ((IGridItemProvider)this).Column; return new IRawElementProviderSimple[] { headers[column] }; } } } return null; } ////// Get's the table item's row headers. /// ///The table item's row headers IRawElementProviderSimple[] ITableItemProvider.GetRowHeaderItems() { return null; } #endregion ITableItemProvider #region IInvokeProvider void IInvokeProvider.Invoke() { Button owningButton = OwningButton; if (owningButton == null || !this.IsEnabled()) throw new ElementNotEnabledException(); // Async call of click event // In ClickHandler opens a dialog and suspend the execution we don't want to block this thread Dispatcher.BeginInvoke(DispatcherPriority.Input, new DispatcherOperationCallback(delegate(object param) { owningButton.AutomationButtonBaseClick(); return null; }), null); } #endregion IInvokeProvider # region IVirtualizedItemProvider void IVirtualizedItemProvider.Realize() { // Change Display mode if (OwningCalendar.DisplayMode != ButtonMode) { OwningCalendar.DisplayMode = ButtonMode; } // Bring into view OwningCalendar.DisplayDate = this.Date; } #endregion IVirtualizedItemProvider override internal bool IsDataItemAutomationPeer() { return true; } override internal void AddToParentProxyWeakRefCache() { CalendarAutomationPeer owningCalendarPeer = FrameworkElementAutomationPeer.CreatePeerForElement(OwningCalendar) as CalendarAutomationPeer; if (owningCalendarPeer != null) { owningCalendarPeer.AddProxyToWeakRefStorage(this.ElementProxyWeakReference, this); } } #region Private Methods private bool EnsureSelection() { // If the day is a blackout day or the SelectionMode is None, selection is not allowed if (this.OwningCalendar.BlackoutDates.Contains(Date) || this.OwningCalendar.SelectionMode == CalendarSelectionMode.None) { return false; } return true; } private bool IsItemInAutomationTree() { AutomationPeer parent = this.GetParent(); if (this.Index != -1 && parent != null && parent.Children != null && this.Index < parent.Children.Count && parent.Children[this.Index] == this) return true; else return false; } private void ThrowElementNotAvailableException() { // To avoid the situation on legacy systems which may not have new unmanaged core. this check with old unmanaged core // avoids throwing exception and provide older behavior returning default values for items which are virtualized rather than throwing exception. if (VirtualizedItemPatternIdentifiers.Pattern != null) throw new ElementNotAvailableException(SR.Get(SRID.VirtualizedElement)); } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
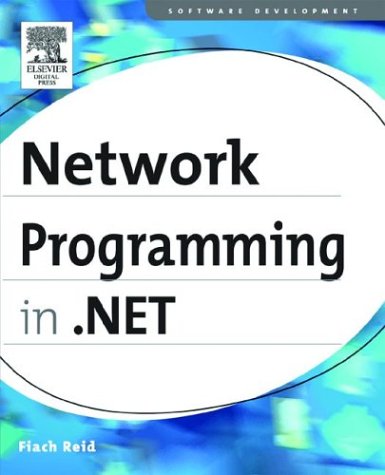
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HostingPreferredMapPath.cs
- EnvelopedPkcs7.cs
- GridSplitter.cs
- DataAdapter.cs
- TypeNameHelper.cs
- Subtree.cs
- TextHidden.cs
- ContractsBCL.cs
- CodeVariableDeclarationStatement.cs
- CodeExpressionStatement.cs
- DetailsViewDesigner.cs
- XamlReaderHelper.cs
- QueryMatcher.cs
- PageRequestManager.cs
- CachedResourceDictionaryExtension.cs
- VisualStyleRenderer.cs
- ConnectionStringEditor.cs
- TaskResultSetter.cs
- PreviewPrintController.cs
- SamlSubjectStatement.cs
- NameTable.cs
- FormViewModeEventArgs.cs
- XmlReader.cs
- InternalRelationshipCollection.cs
- LinqDataSourceSelectEventArgs.cs
- SafeProcessHandle.cs
- selecteditemcollection.cs
- HtmlElementErrorEventArgs.cs
- JoinQueryOperator.cs
- ClientSponsor.cs
- File.cs
- Utility.cs
- InvokeGenerator.cs
- ResourceExpressionBuilder.cs
- EastAsianLunisolarCalendar.cs
- MobileFormsAuthentication.cs
- SchemaImporterExtensionsSection.cs
- TextBounds.cs
- DispatchWrapper.cs
- BufferedStream2.cs
- ClientRoleProvider.cs
- TextSelection.cs
- TextLineResult.cs
- OneToOneMappingSerializer.cs
- HttpContextWrapper.cs
- HostProtectionPermission.cs
- PatternMatcher.cs
- IPHostEntry.cs
- ToggleButton.cs
- MarkupProperty.cs
- Preprocessor.cs
- ListCardsInFileRequest.cs
- EntityType.cs
- ValidatorCollection.cs
- OrderByBuilder.cs
- XamlToRtfParser.cs
- ExecutionContext.cs
- StrokeCollection.cs
- ApplicationSecurityInfo.cs
- TargetInvocationException.cs
- HotCommands.cs
- DesignSurfaceEvent.cs
- TableLayoutStyleCollection.cs
- CommonXSendMessage.cs
- MetadataPropertyCollection.cs
- RemoteWebConfigurationHostServer.cs
- XmlILConstructAnalyzer.cs
- DatasetMethodGenerator.cs
- FormatterConverter.cs
- MenuItemBinding.cs
- TableSectionStyle.cs
- List.cs
- InfoCard.cs
- EndEvent.cs
- ApplicationCommands.cs
- BaseInfoTable.cs
- DownloadProgressEventArgs.cs
- SqlDataRecord.cs
- PermissionListSet.cs
- HtmlMeta.cs
- WebPartChrome.cs
- CacheAxisQuery.cs
- AppSettingsReader.cs
- SubpageParagraph.cs
- CodeIndexerExpression.cs
- SecurityHelper.cs
- CompilerLocalReference.cs
- TagElement.cs
- DropTarget.cs
- LocatorPart.cs
- PropertyFilterAttribute.cs
- GetMemberBinder.cs
- filewebresponse.cs
- SoapHeaders.cs
- CompoundFileDeflateTransform.cs
- WebBrowserNavigatedEventHandler.cs
- CodeTryCatchFinallyStatement.cs
- PolyQuadraticBezierSegment.cs
- _HelperAsyncResults.cs
- SqlClientWrapperSmiStreamChars.cs