Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / GraphicsContext.cs / 1305376 / GraphicsContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System; using System.Drawing.Drawing2D; using System.Diagnostics; using System.Runtime.Versioning; ////// Contains information about the context of a Graphics object. /// internal class GraphicsContext : IDisposable { ////// The state that identifies the context. /// private int contextState; ////// The context's translate transform. /// private PointF transformOffset; ////// The context's clip region. /// private Region clipRegion; ////// The next context up the stack. /// private GraphicsContext nextContext; ////// The previous context down the stack. /// private GraphicsContext prevContext; ////// Flags that determines whether the context was created for a Graphics.Save() operation. /// This kind of contexts are cumulative across subsequent Save() calls so the top context /// info is cumulative. This is not the same for contexts created for a Graphics.BeginContainer() /// operation, in this case the new context information is reset. See Graphics.BeginContainer() /// and Graphics.Save() for more information. /// bool isCumulative; ////// Private constructor disallowed. /// private GraphicsContext() { } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public GraphicsContext(Graphics g) { Matrix transform = g.Transform; if (!transform.IsIdentity) { float[] elements = transform.Elements; this.transformOffset.X = elements[4]; this.transformOffset.Y = elements[5]; } transform.Dispose(); Region clip = g.Clip; if (clip.IsInfinite(g)) { clip.Dispose(); } else { this.clipRegion = clip; } } ////// Disposes this and all contexts up the stack. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Disposes this and all contexts up the stack. /// public void Dispose(bool disposing) { if (this.nextContext != null) { // Dispose all contexts up the stack since they are relative to this one and its state will be invalid. this.nextContext.Dispose(); this.nextContext = null; } if (this.clipRegion != null) { this.clipRegion.Dispose(); this.clipRegion = null; } } ////// The state id representing the GraphicsContext. /// public int State { get { return this.contextState; } set { this.contextState = value; } } ////// The translate transform in the GraphicsContext. /// public PointF TransformOffset { get { return this.transformOffset; } } ////// The clipping region the GraphicsContext. /// public Region Clip { get { return this.clipRegion; } } ////// The next GraphicsContext object in the stack. /// public GraphicsContext Next { get { return this.nextContext; } set { this.nextContext = value; } } ////// The previous GraphicsContext object in the stack. /// public GraphicsContext Previous { get { return this.prevContext; } set { this.prevContext = value; } } ////// Determines whether this context is cumulative or not. See filed for more info. /// public bool IsCumulative { get { return this.isCumulative; } set { this.isCumulative = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
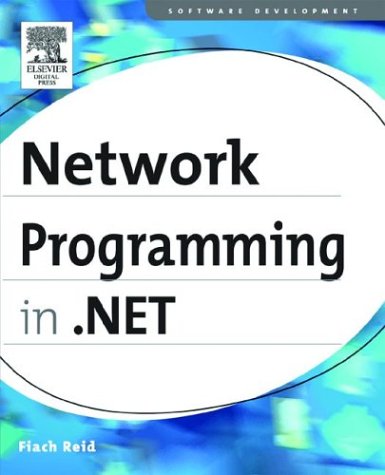
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityProviderServices.cs
- TextEditorSpelling.cs
- XmlTypeMapping.cs
- TransactionManager.cs
- ItemCollection.cs
- MainMenu.cs
- TransactionManager.cs
- DataGridViewComboBoxCell.cs
- FormatException.cs
- LocalServiceSecuritySettings.cs
- RemotingAttributes.cs
- XPathNodePointer.cs
- Schema.cs
- MatrixValueSerializer.cs
- ToolStripItem.cs
- DetailsViewInsertEventArgs.cs
- Parameter.cs
- CommittableTransaction.cs
- ScriptRegistrationManager.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- HWStack.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ControlUtil.cs
- SizeChangedInfo.cs
- SecurityAttributeGenerationHelper.cs
- ColorKeyFrameCollection.cs
- GridItemProviderWrapper.cs
- HostTimeoutsElement.cs
- PolicyReader.cs
- COAUTHIDENTITY.cs
- TriggerCollection.cs
- WebPartEditVerb.cs
- ResourceProperty.cs
- httpserverutility.cs
- DataColumnChangeEvent.cs
- LookupBindingPropertiesAttribute.cs
- MemoryStream.cs
- MediaContextNotificationWindow.cs
- DbParameterHelper.cs
- SQLDecimalStorage.cs
- TempEnvironment.cs
- ResourcePart.cs
- PrincipalPermission.cs
- JobPageOrder.cs
- CapabilitiesUse.cs
- RootDesignerSerializerAttribute.cs
- EntityWrapper.cs
- InkPresenterAutomationPeer.cs
- InvalidPropValue.cs
- DrawListViewColumnHeaderEventArgs.cs
- IntSumAggregationOperator.cs
- DifferencingCollection.cs
- RecoverInstanceLocksCommand.cs
- SystemResourceHost.cs
- TransformerInfoCollection.cs
- VerificationAttribute.cs
- WorkflowTransactionService.cs
- CompilerScope.Storage.cs
- ExtensionElement.cs
- ClaimTypeElementCollection.cs
- Base64Encoder.cs
- LambdaCompiler.Logical.cs
- DataServiceProviderMethods.cs
- TransportChannelListener.cs
- UnaryNode.cs
- HttpCacheVary.cs
- BindingManagerDataErrorEventArgs.cs
- WebBrowserHelper.cs
- HttpInputStream.cs
- MinMaxParagraphWidth.cs
- QuerySubExprEliminator.cs
- SqlBooleanMismatchVisitor.cs
- _ListenerAsyncResult.cs
- Lazy.cs
- LinqExpressionNormalizer.cs
- OdbcDataAdapter.cs
- ProtocolImporter.cs
- WebSysDisplayNameAttribute.cs
- NativeDirectoryServicesQueryAPIs.cs
- CustomErrorsSection.cs
- CallbackHandler.cs
- ReadOnlyHierarchicalDataSourceView.cs
- PolicyException.cs
- SmtpDigestAuthenticationModule.cs
- Header.cs
- TagPrefixCollection.cs
- TextAnchor.cs
- WeakRefEnumerator.cs
- RecordManager.cs
- ClientOptions.cs
- GeneralTransform3DTo2DTo3D.cs
- GPPOINT.cs
- CompositeScriptReference.cs
- DES.cs
- TreeView.cs
- RowType.cs
- TriggerCollection.cs
- ArrayExtension.cs
- CodeDomSerializerException.cs
- CertificateManager.cs