Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / Common / SQLTypes / SQLDateTimeStorage.cs / 1 / SQLDateTimeStorage.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.Xml; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.IO; using System.Xml.Serialization; using System.Collections; internal sealed class SqlDateTimeStorage : DataStorage { private SqlDateTime[] values; public SqlDateTimeStorage(DataColumn column) : base(column, typeof(SqlDateTime), SqlDateTime.Null, SqlDateTime.Null) { } override public Object Aggregate(int[] records, AggregateType kind) { bool hasData = false; try { switch (kind) { case AggregateType.Min: SqlDateTime min = SqlDateTime.MaxValue; for (int i = 0; i < records.Length; i++) { int record = records[i]; if (IsNull(record)) continue; if ((SqlDateTime.LessThan(values[record], min)).IsTrue) min = values[record]; hasData = true; } if (hasData) { return min; } return NullValue; case AggregateType.Max: SqlDateTime max = SqlDateTime.MinValue; for (int i = 0; i < records.Length; i++) { int record = records[i]; if (IsNull(record)) continue; if ((SqlDateTime.GreaterThan(values[record], max)).IsTrue) max = values[record]; hasData = true; } if (hasData) { return max; } return NullValue; case AggregateType.First: if (records.Length > 0) { return values[records[0]]; } return null;// no data => null case AggregateType.Count: int count = 0; for (int i = 0; i < records.Length; i++) { if (!IsNull(records[i])) count++; } return count; } } catch (OverflowException) { throw ExprException.Overflow(typeof(SqlDateTime)); } throw ExceptionBuilder.AggregateException(kind, DataType); } override public int Compare(int recordNo1, int recordNo2) { return values[recordNo1].CompareTo(values[recordNo2]); } override public int CompareValueTo(int recordNo, Object value) { return values[recordNo].CompareTo((SqlDateTime)value); } override public object ConvertValue(object value) { if (null != value) { return SqlConvert.ConvertToSqlDateTime(value); } return NullValue; } override public void Copy(int recordNo1, int recordNo2) { values[recordNo2] = values[recordNo1]; } override public Object Get(int record) { return values[record]; } override public bool IsNull(int record) { return (values[record].IsNull); } override public void Set(int record, Object value) { values[record] = SqlConvert.ConvertToSqlDateTime(value); } override public void SetCapacity(int capacity) { SqlDateTime[] newValues = new SqlDateTime[capacity]; if (null != values) { Array.Copy(values, 0, newValues, 0, Math.Min(capacity, values.Length)); } values = newValues; } override public object ConvertXmlToObject(string s) { SqlDateTime newValue = new SqlDateTime(); string tempStr =string.Concat("", s, ""); // this is done since you can give fragmet to reader, bug 98767 StringReader strReader = new StringReader(tempStr); IXmlSerializable tmp = newValue; using (XmlTextReader xmlTextReader = new XmlTextReader(strReader)) { tmp.ReadXml(xmlTextReader); } return ((SqlDateTime)tmp); } override public string ConvertObjectToXml(object value) { Debug.Assert(!DataStorage.IsObjectNull(value), "we shouldn't have null here"); Debug.Assert((value.GetType() == typeof(SqlDateTime)), "wrong input type"); StringWriter strwriter = new StringWriter(FormatProvider); using (XmlTextWriter xmlTextWriter = new XmlTextWriter (strwriter)) { ((IXmlSerializable)value).WriteXml(xmlTextWriter); } return (strwriter.ToString ()); } override protected object GetEmptyStorage(int recordCount) { return new SqlDateTime[recordCount]; } override protected void CopyValue(int record, object store, BitArray nullbits, int storeIndex) { SqlDateTime[] typedStore = (SqlDateTime[]) store; typedStore[storeIndex] = values[record]; nullbits.Set(record, IsNull(record)); } override protected void SetStorage(object store, BitArray nullbits) { values = (SqlDateTime[]) store; //SetNullStorage(nullbits); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.Xml; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.IO; using System.Xml.Serialization; using System.Collections; internal sealed class SqlDateTimeStorage : DataStorage { private SqlDateTime[] values; public SqlDateTimeStorage(DataColumn column) : base(column, typeof(SqlDateTime), SqlDateTime.Null, SqlDateTime.Null) { } override public Object Aggregate(int[] records, AggregateType kind) { bool hasData = false; try { switch (kind) { case AggregateType.Min: SqlDateTime min = SqlDateTime.MaxValue; for (int i = 0; i < records.Length; i++) { int record = records[i]; if (IsNull(record)) continue; if ((SqlDateTime.LessThan(values[record], min)).IsTrue) min = values[record]; hasData = true; } if (hasData) { return min; } return NullValue; case AggregateType.Max: SqlDateTime max = SqlDateTime.MinValue; for (int i = 0; i < records.Length; i++) { int record = records[i]; if (IsNull(record)) continue; if ((SqlDateTime.GreaterThan(values[record], max)).IsTrue) max = values[record]; hasData = true; } if (hasData) { return max; } return NullValue; case AggregateType.First: if (records.Length > 0) { return values[records[0]]; } return null;// no data => null case AggregateType.Count: int count = 0; for (int i = 0; i < records.Length; i++) { if (!IsNull(records[i])) count++; } return count; } } catch (OverflowException) { throw ExprException.Overflow(typeof(SqlDateTime)); } throw ExceptionBuilder.AggregateException(kind, DataType); } override public int Compare(int recordNo1, int recordNo2) { return values[recordNo1].CompareTo(values[recordNo2]); } override public int CompareValueTo(int recordNo, Object value) { return values[recordNo].CompareTo((SqlDateTime)value); } override public object ConvertValue(object value) { if (null != value) { return SqlConvert.ConvertToSqlDateTime(value); } return NullValue; } override public void Copy(int recordNo1, int recordNo2) { values[recordNo2] = values[recordNo1]; } override public Object Get(int record) { return values[record]; } override public bool IsNull(int record) { return (values[record].IsNull); } override public void Set(int record, Object value) { values[record] = SqlConvert.ConvertToSqlDateTime(value); } override public void SetCapacity(int capacity) { SqlDateTime[] newValues = new SqlDateTime[capacity]; if (null != values) { Array.Copy(values, 0, newValues, 0, Math.Min(capacity, values.Length)); } values = newValues; } override public object ConvertXmlToObject(string s) { SqlDateTime newValue = new SqlDateTime(); string tempStr =string.Concat("", s, ""); // this is done since you can give fragmet to reader, bug 98767 StringReader strReader = new StringReader(tempStr); IXmlSerializable tmp = newValue; using (XmlTextReader xmlTextReader = new XmlTextReader(strReader)) { tmp.ReadXml(xmlTextReader); } return ((SqlDateTime)tmp); } override public string ConvertObjectToXml(object value) { Debug.Assert(!DataStorage.IsObjectNull(value), "we shouldn't have null here"); Debug.Assert((value.GetType() == typeof(SqlDateTime)), "wrong input type"); StringWriter strwriter = new StringWriter(FormatProvider); using (XmlTextWriter xmlTextWriter = new XmlTextWriter (strwriter)) { ((IXmlSerializable)value).WriteXml(xmlTextWriter); } return (strwriter.ToString ()); } override protected object GetEmptyStorage(int recordCount) { return new SqlDateTime[recordCount]; } override protected void CopyValue(int record, object store, BitArray nullbits, int storeIndex) { SqlDateTime[] typedStore = (SqlDateTime[]) store; typedStore[storeIndex] = values[record]; nullbits.Set(record, IsNull(record)); } override protected void SetStorage(object store, BitArray nullbits) { values = (SqlDateTime[]) store; //SetNullStorage(nullbits); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
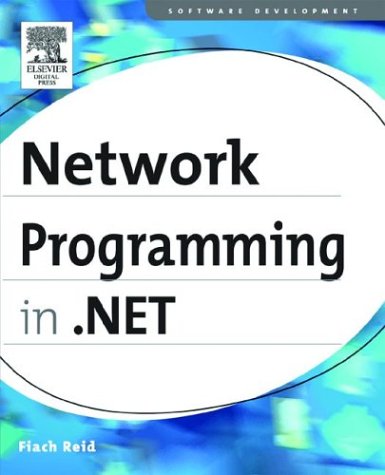
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QueryExpression.cs
- XmlIgnoreAttribute.cs
- CFGGrammar.cs
- ConditionalAttribute.cs
- FolderLevelBuildProvider.cs
- XmlElementAttribute.cs
- XmlDataContract.cs
- PropertyTabAttribute.cs
- IPEndPoint.cs
- SessionStateUtil.cs
- BitmapSourceSafeMILHandle.cs
- TrustLevelCollection.cs
- SoapException.cs
- LongAverageAggregationOperator.cs
- MetadataWorkspace.cs
- UnsafeNativeMethodsPenimc.cs
- ActivationArguments.cs
- HealthMonitoringSection.cs
- ArrayList.cs
- DiagnosticTraceSource.cs
- DataSourceControl.cs
- RelationshipFixer.cs
- Int64Animation.cs
- SQLBinary.cs
- RoleGroupCollectionEditor.cs
- DynamicMethod.cs
- ChannelFactoryRefCache.cs
- Timer.cs
- EventManager.cs
- XamlVector3DCollectionSerializer.cs
- DebugController.cs
- ImportStoreException.cs
- ProjectionCamera.cs
- ISessionStateStore.cs
- StructureChangedEventArgs.cs
- Popup.cs
- XmlValueConverter.cs
- PrintingPermissionAttribute.cs
- Utilities.cs
- ThicknessConverter.cs
- MimeImporter.cs
- NodeLabelEditEvent.cs
- AnimatedTypeHelpers.cs
- UrlPath.cs
- ColorBlend.cs
- WsatServiceAddress.cs
- EventLogPermissionAttribute.cs
- RequestCachePolicy.cs
- DocumentViewer.cs
- Logging.cs
- DataGridTablesFactory.cs
- CharEntityEncoderFallback.cs
- WorkflowLayouts.cs
- KoreanCalendar.cs
- ActiveXHost.cs
- CodeDOMUtility.cs
- ConfigurationCollectionAttribute.cs
- UntypedNullExpression.cs
- StylusButtonEventArgs.cs
- Comparer.cs
- Control.cs
- StringValidator.cs
- ObjectKeyFrameCollection.cs
- HttpCapabilitiesEvaluator.cs
- LocalizeDesigner.cs
- TabletCollection.cs
- HandlerBase.cs
- CultureInfoConverter.cs
- UTF7Encoding.cs
- ParentControlDesigner.cs
- Typography.cs
- ListBoxAutomationPeer.cs
- HttpValueCollection.cs
- XmlSchemaInfo.cs
- StyleHelper.cs
- httpstaticobjectscollection.cs
- InteropDesigner.xaml.cs
- EncoderParameters.cs
- GlyphElement.cs
- arc.cs
- SystemUnicastIPAddressInformation.cs
- Themes.cs
- Model3DGroup.cs
- ArithmeticException.cs
- ListContractAdapter.cs
- ClockController.cs
- Constraint.cs
- VerbConverter.cs
- TextParaClient.cs
- DispatchChannelSink.cs
- DesignColumnCollection.cs
- __ComObject.cs
- HtmlGenericControl.cs
- ListControlBuilder.cs
- StreamInfo.cs
- X509CertificateValidator.cs
- ClientScriptManager.cs
- ResourceDictionaryCollection.cs
- BitmapEffectCollection.cs
- LabelDesigner.cs