Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / Typography.cs / 1 / Typography.cs
//---------------------------------------------------------------------------- // // Copyright(C) Microsoft Corporation. All rights reserved. // // File: Typography.cs // // Description: Element representing text typogrpahy properties for // Text, FlowDocument, TextRange // // History: // 06/12/2003 : [....] - created. // //--------------------------------------------------------------------------- using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Documents; using System.Windows.Controls; using System.ComponentModel; using MS.Internal.Text; using MS.Utility; using System; namespace System.Windows.Documents { ////// Provide access to typography porperties of element in syntax of Typography.xxx = yyy; /// Actual data is stored in the owner. /// public sealed class Typography { #region Constructors ////// Typography constructor. /// internal Typography(DependencyObject owner) { //there should be actual owner if(owner == null) { throw new ArgumentNullException("owner"); } _owner = owner; } // ----------------------------------------------------------------- // Static ctor. // ----------------------------------------------------------------- static Typography() { // Set typography properties to values that match those in property // definitions in Typography class. Default.SetStandardLigatures(true); Default.SetContextualAlternates(true); Default.SetContextualLigatures(true); Default.SetKerning(true); } #endregion Constructors #region Public properties ///StandardLigatures property public bool StandardLigatures { get { return (bool) _owner.GetValue(StandardLigaturesProperty); } set { _owner.SetValue(StandardLigaturesProperty, value); } } ///ContextualLigatures Property public bool ContextualLigatures { get { return (bool) _owner.GetValue(ContextualLigaturesProperty); } set { _owner.SetValue(ContextualLigaturesProperty, value); } } ///DiscretionaryLigatures Property public bool DiscretionaryLigatures { get { return (bool) _owner.GetValue(DiscretionaryLigaturesProperty); } set { _owner.SetValue(DiscretionaryLigaturesProperty, value); } } ///HistoricalLigatures Property public bool HistoricalLigatures { get { return (bool) _owner.GetValue(HistoricalLigaturesProperty); } set { _owner.SetValue(HistoricalLigaturesProperty, value); } } ///AnnotationAlternates Property public int AnnotationAlternates { get { return (int) _owner.GetValue(AnnotationAlternatesProperty); } set { _owner.SetValue(AnnotationAlternatesProperty, value); } } ///ContextualAlternates Property public bool ContextualAlternates { get { return (bool) _owner.GetValue(ContextualAlternatesProperty); } set { _owner.SetValue(ContextualAlternatesProperty, value); } } ///HistoricalForms Property public bool HistoricalForms { get { return (bool) _owner.GetValue(HistoricalFormsProperty); } set { _owner.SetValue(HistoricalFormsProperty, value); } } ///Kerning Property public bool Kerning { get { return (bool) _owner.GetValue(KerningProperty); } set { _owner.SetValue(KerningProperty, value); } } ///CapitalSpacing Property public bool CapitalSpacing { get { return (bool) _owner.GetValue(CapitalSpacingProperty); } set { _owner.SetValue(CapitalSpacingProperty, value); } } ///CaseSensitiveForms Property public bool CaseSensitiveForms { get { return (bool) _owner.GetValue(CaseSensitiveFormsProperty); } set { _owner.SetValue(CaseSensitiveFormsProperty, value); } } ///StylisticSet1 Property public bool StylisticSet1 { get { return (bool) _owner.GetValue(StylisticSet1Property); } set { _owner.SetValue(StylisticSet1Property, value); } } ///StylisticSet2 Property public bool StylisticSet2 { get { return (bool) _owner.GetValue(StylisticSet2Property); } set { _owner.SetValue(StylisticSet2Property, value); } } ///StylisticSet3 Property public bool StylisticSet3 { get { return (bool) _owner.GetValue(StylisticSet3Property); } set { _owner.SetValue(StylisticSet3Property, value); } } ///StylisticSet4 Property public bool StylisticSet4 { get { return (bool) _owner.GetValue(StylisticSet4Property); } set { _owner.SetValue(StylisticSet4Property, value); } } ///StylisticSet5 Property public bool StylisticSet5 { get { return (bool) _owner.GetValue(StylisticSet5Property); } set { _owner.SetValue(StylisticSet5Property, value); } } ///StylisticSet6 Property public bool StylisticSet6 { get { return (bool) _owner.GetValue(StylisticSet6Property); } set { _owner.SetValue(StylisticSet6Property, value); } } ///StylisticSet7 Property public bool StylisticSet7 { get { return (bool) _owner.GetValue(StylisticSet7Property); } set { _owner.SetValue(StylisticSet7Property, value); } } ///StylisticSet8 Property public bool StylisticSet8 { get { return (bool) _owner.GetValue(StylisticSet8Property); } set { _owner.SetValue(StylisticSet8Property, value); } } ///StylisticSet9 Property public bool StylisticSet9 { get { return (bool) _owner.GetValue(StylisticSet9Property); } set { _owner.SetValue(StylisticSet9Property, value); } } ///StylisticSet10 Property public bool StylisticSet10 { get { return (bool) _owner.GetValue(StylisticSet10Property); } set { _owner.SetValue(StylisticSet10Property, value); } } ///StylisticSet11 Property public bool StylisticSet11 { get { return (bool) _owner.GetValue(StylisticSet11Property); } set { _owner.SetValue(StylisticSet11Property, value); } } ///StylisticSet12 Property public bool StylisticSet12 { get { return (bool) _owner.GetValue(StylisticSet12Property); } set { _owner.SetValue(StylisticSet12Property, value); } } ///StylisticSet13 Property public bool StylisticSet13 { get { return (bool) _owner.GetValue(StylisticSet13Property); } set { _owner.SetValue(StylisticSet13Property, value); } } ///StylisticSet14 Property public bool StylisticSet14 { get { return (bool) _owner.GetValue(StylisticSet14Property); } set { _owner.SetValue(StylisticSet14Property, value); } } ///StylisticSet15 Property public bool StylisticSet15 { get { return (bool) _owner.GetValue(StylisticSet15Property); } set { _owner.SetValue(StylisticSet15Property, value); } } ///StylisticSet16 Property public bool StylisticSet16 { get { return (bool) _owner.GetValue(StylisticSet16Property); } set { _owner.SetValue(StylisticSet16Property, value); } } ///StylisticSet17 Property public bool StylisticSet17 { get { return (bool) _owner.GetValue(StylisticSet17Property); } set { _owner.SetValue(StylisticSet17Property, value); } } ///StylisticSet18 Property public bool StylisticSet18 { get { return (bool) _owner.GetValue(StylisticSet18Property); } set { _owner.SetValue(StylisticSet18Property, value); } } ///StylisticSet19 Property public bool StylisticSet19 { get { return (bool) _owner.GetValue(StylisticSet19Property); } set { _owner.SetValue(StylisticSet19Property, value); } } ///StylisticSet20 Property public bool StylisticSet20 { get { return (bool) _owner.GetValue(StylisticSet20Property); } set { _owner.SetValue(StylisticSet20Property, value); } } ///Fraction Property public FontFraction Fraction { get { return (FontFraction) _owner.GetValue(FractionProperty); } set { _owner.SetValue(FractionProperty, value); } } ///SlashedZero Property public bool SlashedZero { get { return (bool) _owner.GetValue(SlashedZeroProperty); } set { _owner.SetValue(SlashedZeroProperty, value); } } ///MathematicalGreek Property public bool MathematicalGreek { get { return (bool) _owner.GetValue(MathematicalGreekProperty); } set { _owner.SetValue(MathematicalGreekProperty, value); } } ///EastAsianExpertForms Property public bool EastAsianExpertForms { get { return (bool) _owner.GetValue(EastAsianExpertFormsProperty); } set { _owner.SetValue(EastAsianExpertFormsProperty, value); } } ///Variants Property public FontVariants Variants { get { return (FontVariants) _owner.GetValue(VariantsProperty); } set { _owner.SetValue(VariantsProperty, value); } } ///Capitals Property public FontCapitals Capitals { get { return (FontCapitals) _owner.GetValue(CapitalsProperty); } set { _owner.SetValue(CapitalsProperty, value); } } ///NumeralStyle Property public FontNumeralStyle NumeralStyle { get { return (FontNumeralStyle) _owner.GetValue(NumeralStyleProperty); } set { _owner.SetValue(NumeralStyleProperty, value); } } ///NumeralAlignment Property public FontNumeralAlignment NumeralAlignment { get { return (FontNumeralAlignment) _owner.GetValue(NumeralAlignmentProperty); } set { _owner.SetValue(NumeralAlignmentProperty, value); } } ///EastAsianWidths Property public FontEastAsianWidths EastAsianWidths { get { return (FontEastAsianWidths) _owner.GetValue(EastAsianWidthsProperty); } set { _owner.SetValue(EastAsianWidthsProperty, value); } } ///EastAsianLanguage Property public FontEastAsianLanguage EastAsianLanguage { get { return (FontEastAsianLanguage) _owner.GetValue(EastAsianLanguageProperty); } set { _owner.SetValue(EastAsianLanguageProperty, value); } } ///StandardSwashes Property public int StandardSwashes { get { return (int) _owner.GetValue(StandardSwashesProperty); } set { _owner.SetValue(StandardSwashesProperty, value); } } ///ContextualSwashes Property public int ContextualSwashes { get { return (int) _owner.GetValue(ContextualSwashesProperty); } set { _owner.SetValue(ContextualSwashesProperty, value); } } ///StylisticAlternates Property public int StylisticAlternates { get { return (int) _owner.GetValue(StylisticAlternatesProperty); } set { _owner.SetValue(StylisticAlternatesProperty, value); } } #endregion Public properties #region Attached Properties Setters ////// Writes the attached property StandardLigatures to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStandardLigatures(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StandardLigaturesProperty, value); } /// /// Returns the attached property StandardLigatures value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStandardLigatures(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StandardLigaturesProperty); } /// /// Writes the attached property ContextualLigatures to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetContextualLigatures(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ContextualLigaturesProperty, value); } /// /// Returns the attached property ContextualLigatures value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetContextualLigatures(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(ContextualLigaturesProperty); } /// /// Writes the attached property DiscretionaryLigatures to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetDiscretionaryLigatures(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(DiscretionaryLigaturesProperty, value); } /// /// Returns the attached property DiscretionaryLigatures value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetDiscretionaryLigatures(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(DiscretionaryLigaturesProperty); } /// /// Writes the attached property HistoricalLigatures to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetHistoricalLigatures(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HistoricalLigaturesProperty, value); } /// /// Returns the attached property HistoricalLigatures value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetHistoricalLigatures(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(HistoricalLigaturesProperty); } /// /// Writes the attached property AnnotationAlternates to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetAnnotationAlternates(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AnnotationAlternatesProperty, value); } /// /// Returns the attached property AnnotationAlternates value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetAnnotationAlternates(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(AnnotationAlternatesProperty); } /// /// Writes the attached property ContextualAlternates to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetContextualAlternates(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ContextualAlternatesProperty, value); } /// /// Returns the attached property ContextualAlternates value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetContextualAlternates(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(ContextualAlternatesProperty); } /// /// Writes the attached property HistoricalForms to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetHistoricalForms(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HistoricalFormsProperty, value); } /// /// Returns the attached property HistoricalForms value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetHistoricalForms(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(HistoricalFormsProperty); } /// /// Writes the attached property Kerning to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetKerning(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(KerningProperty, value); } /// /// Returns the attached property Kerning value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetKerning(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(KerningProperty); } /// /// Writes the attached property CapitalSpacing to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetCapitalSpacing(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(CapitalSpacingProperty, value); } /// /// Returns the attached property CapitalSpacing value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetCapitalSpacing(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(CapitalSpacingProperty); } /// /// Writes the attached property CaseSensitiveForms to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetCaseSensitiveForms(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(CaseSensitiveFormsProperty, value); } /// /// Returns the attached property CaseSensitiveForms value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetCaseSensitiveForms(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(CaseSensitiveFormsProperty); } /// /// Writes the attached property StylisticSet1 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet1(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet1Property, value); } /// /// Returns the attached property StylisticSet1 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet1(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet1Property); } /// /// Writes the attached property StylisticSet2 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet2(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet2Property, value); } /// /// Returns the attached property StylisticSet2 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet2(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet2Property); } /// /// Writes the attached property StylisticSet3 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet3(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet3Property, value); } /// /// Returns the attached property StylisticSet3 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet3(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet3Property); } /// /// Writes the attached property StylisticSet4 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet4(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet4Property, value); } /// /// Returns the attached property StylisticSet4 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet4(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet4Property); } /// /// Writes the attached property StylisticSet5 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet5(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet5Property, value); } /// /// Returns the attached property StylisticSet5 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet5(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet5Property); } /// /// Writes the attached property StylisticSet6 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet6(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet6Property, value); } /// /// Returns the attached property StylisticSet6 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet6(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet6Property); } /// /// Writes the attached property StylisticSet7 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet7(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet7Property, value); } /// /// Returns the attached property StylisticSet7 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet7(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet7Property); } /// /// Writes the attached property StylisticSet8 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet8(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet8Property, value); } /// /// Returns the attached property StylisticSet8 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet8(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet8Property); } /// /// Writes the attached property StylisticSet9 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet9(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet9Property, value); } /// /// Returns the attached property StylisticSet9 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet9(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet9Property); } /// /// Writes the attached property StylisticSet10 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet10(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet10Property, value); } /// /// Returns the attached property StylisticSet10 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet10(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet10Property); } /// /// Writes the attached property StylisticSet11 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet11(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet11Property, value); } /// /// Returns the attached property StylisticSet11 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet11(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet11Property); } /// /// Writes the attached property StylisticSet12 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet12(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet12Property, value); } /// /// Returns the attached property StylisticSet12 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet12(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet12Property); } /// /// Writes the attached property StylisticSet13 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet13(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet13Property, value); } /// /// Returns the attached property StylisticSet13 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet13(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet13Property); } /// /// Writes the attached property StylisticSet14 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet14(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet14Property, value); } /// /// Returns the attached property StylisticSet14 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet14(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet14Property); } /// /// Writes the attached property StylisticSet15 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet15(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet15Property, value); } /// /// Returns the attached property StylisticSet15 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet15(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet15Property); } /// /// Writes the attached property StylisticSet16 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet16(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet16Property, value); } /// /// Returns the attached property StylisticSet16 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet16(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet16Property); } /// /// Writes the attached property StylisticSet17 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet17(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet17Property, value); } /// /// Returns the attached property StylisticSet17 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet17(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet17Property); } /// /// Writes the attached property StylisticSet18 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet18(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet18Property, value); } /// /// Returns the attached property StylisticSet18 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet18(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet18Property); } /// /// Writes the attached property StylisticSet19 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet19(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet19Property, value); } /// /// Returns the attached property StylisticSet19 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet19(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet19Property); } /// /// Writes the attached property StylisticSet20 to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticSet20(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticSet20Property, value); } /// /// Returns the attached property StylisticSet20 value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetStylisticSet20(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(StylisticSet20Property); } /// /// Writes the attached property Fraction to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetFraction(DependencyObject element, FontFraction value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(FractionProperty, value); } /// /// Returns the attached property Fraction value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static FontFraction GetFraction(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (FontFraction)element.GetValue(FractionProperty); } /// /// Writes the attached property SlashedZero to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetSlashedZero(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(SlashedZeroProperty, value); } /// /// Returns the attached property SlashedZero value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetSlashedZero(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(SlashedZeroProperty); } /// /// Writes the attached property MathematicalGreek to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetMathematicalGreek(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(MathematicalGreekProperty, value); } /// /// Returns the attached property MathematicalGreek value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetMathematicalGreek(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(MathematicalGreekProperty); } /// /// Writes the attached property EastAsianExpertForms to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetEastAsianExpertForms(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(EastAsianExpertFormsProperty, value); } /// /// Returns the attached property EastAsianExpertForms value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetEastAsianExpertForms(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(EastAsianExpertFormsProperty); } /// /// Writes the attached property Variants to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetVariants(DependencyObject element, FontVariants value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(VariantsProperty, value); } /// /// Returns the attached property Variants value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static FontVariants GetVariants(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (FontVariants)element.GetValue(VariantsProperty); } /// /// Writes the attached property Capitals to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetCapitals(DependencyObject element, FontCapitals value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(CapitalsProperty, value); } /// /// Returns the attached property Capitals value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static FontCapitals GetCapitals(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (FontCapitals)element.GetValue(CapitalsProperty); } /// /// Writes the attached property NumeralStyle to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetNumeralStyle(DependencyObject element, FontNumeralStyle value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(NumeralStyleProperty, value); } /// /// Returns the attached property NumeralStyle value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static FontNumeralStyle GetNumeralStyle(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (FontNumeralStyle)element.GetValue(NumeralStyleProperty); } /// /// Writes the attached property NumeralAlignment to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetNumeralAlignment(DependencyObject element, FontNumeralAlignment value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(NumeralAlignmentProperty, value); } /// /// Returns the attached property NumeralAlignment value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static FontNumeralAlignment GetNumeralAlignment(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (FontNumeralAlignment)element.GetValue(NumeralAlignmentProperty); } /// /// Writes the attached property EastAsianWidths to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetEastAsianWidths(DependencyObject element, FontEastAsianWidths value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(EastAsianWidthsProperty, value); } /// /// Returns the attached property EastAsianWidths value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static FontEastAsianWidths GetEastAsianWidths(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (FontEastAsianWidths)element.GetValue(EastAsianWidthsProperty); } /// /// Writes the attached property EastAsianLanguage to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetEastAsianLanguage(DependencyObject element, FontEastAsianLanguage value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(EastAsianLanguageProperty, value); } /// /// Returns the attached property EastAsianLanguage value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static FontEastAsianLanguage GetEastAsianLanguage(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (FontEastAsianLanguage)element.GetValue(EastAsianLanguageProperty); } /// /// Writes the attached property StandardSwashes to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStandardSwashes(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StandardSwashesProperty, value); } /// /// Returns the attached property StandardSwashes value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetStandardSwashes(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(StandardSwashesProperty); } /// /// Writes the attached property ContextualSwashes to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetContextualSwashes(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ContextualSwashesProperty, value); } /// /// Returns the attached property ContextualSwashes value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetContextualSwashes(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(ContextualSwashesProperty); } /// /// Writes the attached property StylisticAlternates to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetStylisticAlternates(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(StylisticAlternatesProperty, value); } /// /// Returns the attached property StylisticAlternates value for the given element. /// /// The element from which to read the attached property. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetStylisticAlternates(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(StylisticAlternatesProperty); } #endregion Attached Groperties Getters and Setters #region Dependency Properties /// StandardLigatures Property public static readonly DependencyProperty StandardLigaturesProperty = DependencyProperty.RegisterAttached( "StandardLigatures", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( true, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///ContextualLigatures Property public static readonly DependencyProperty ContextualLigaturesProperty = DependencyProperty.RegisterAttached( "ContextualLigatures", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( true, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///DiscretionaryLigatures Property public static readonly DependencyProperty DiscretionaryLigaturesProperty = DependencyProperty.RegisterAttached( "DiscretionaryLigatures", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///HistoricalLigatures Property public static readonly DependencyProperty HistoricalLigaturesProperty = DependencyProperty.RegisterAttached( "HistoricalLigatures", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///AnnotationAlternates Property public static readonly DependencyProperty AnnotationAlternatesProperty = DependencyProperty.RegisterAttached( "AnnotationAlternates", typeof(int), typeof(Typography), new FrameworkPropertyMetadata( 0, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///ContextualAlternates Property public static readonly DependencyProperty ContextualAlternatesProperty = DependencyProperty.RegisterAttached( "ContextualAlternates", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( true, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///HistoricalForms Property public static readonly DependencyProperty HistoricalFormsProperty = DependencyProperty.RegisterAttached( "HistoricalForms", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///Kerning Property public static readonly DependencyProperty KerningProperty = DependencyProperty.RegisterAttached( "Kerning", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( true, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///CapitalSpacing Property public static readonly DependencyProperty CapitalSpacingProperty = DependencyProperty.RegisterAttached( "CapitalSpacing", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///CaseSensitiveForms Property public static readonly DependencyProperty CaseSensitiveFormsProperty = DependencyProperty.RegisterAttached( "CaseSensitiveForms", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet1 Property public static readonly DependencyProperty StylisticSet1Property = DependencyProperty.RegisterAttached( "StylisticSet1", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet2 Property public static readonly DependencyProperty StylisticSet2Property = DependencyProperty.RegisterAttached( "StylisticSet2", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet3 Property public static readonly DependencyProperty StylisticSet3Property = DependencyProperty.RegisterAttached( "StylisticSet3", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet4 Property public static readonly DependencyProperty StylisticSet4Property = DependencyProperty.RegisterAttached( "StylisticSet4", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet5 Property public static readonly DependencyProperty StylisticSet5Property = DependencyProperty.RegisterAttached( "StylisticSet5", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet6 Property public static readonly DependencyProperty StylisticSet6Property = DependencyProperty.RegisterAttached( "StylisticSet6", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet7 Property public static readonly DependencyProperty StylisticSet7Property = DependencyProperty.RegisterAttached( "StylisticSet7", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet8 Property public static readonly DependencyProperty StylisticSet8Property = DependencyProperty.RegisterAttached( "StylisticSet8", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet9 Property public static readonly DependencyProperty StylisticSet9Property = DependencyProperty.RegisterAttached( "StylisticSet9", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet10 Property public static readonly DependencyProperty StylisticSet10Property = DependencyProperty.RegisterAttached( "StylisticSet10", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet11 Property public static readonly DependencyProperty StylisticSet11Property = DependencyProperty.RegisterAttached( "StylisticSet11", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet12 Property public static readonly DependencyProperty StylisticSet12Property = DependencyProperty.RegisterAttached( "StylisticSet12", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet13 Property public static readonly DependencyProperty StylisticSet13Property = DependencyProperty.RegisterAttached( "StylisticSet13", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet14 Property public static readonly DependencyProperty StylisticSet14Property = DependencyProperty.RegisterAttached( "StylisticSet14", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet15 Property public static readonly DependencyProperty StylisticSet15Property = DependencyProperty.RegisterAttached( "StylisticSet15", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet16 Property public static readonly DependencyProperty StylisticSet16Property = DependencyProperty.RegisterAttached( "StylisticSet16", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet17 Property public static readonly DependencyProperty StylisticSet17Property = DependencyProperty.RegisterAttached( "StylisticSet17", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet18 Property public static readonly DependencyProperty StylisticSet18Property = DependencyProperty.RegisterAttached( "StylisticSet18", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet19 Property public static readonly DependencyProperty StylisticSet19Property = DependencyProperty.RegisterAttached( "StylisticSet19", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticSet20 Property public static readonly DependencyProperty StylisticSet20Property = DependencyProperty.RegisterAttached( "StylisticSet20", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///Fraction Property public static readonly DependencyProperty FractionProperty = DependencyProperty.RegisterAttached( "Fraction", typeof(FontFraction), typeof(Typography), new FrameworkPropertyMetadata( FontFraction.Normal, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///SlashedZero Property public static readonly DependencyProperty SlashedZeroProperty = DependencyProperty.RegisterAttached( "SlashedZero", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///MathematicalGreek Property public static readonly DependencyProperty MathematicalGreekProperty = DependencyProperty.RegisterAttached( "MathematicalGreek", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///EastAsianExpertForms Property public static readonly DependencyProperty EastAsianExpertFormsProperty = DependencyProperty.RegisterAttached( "EastAsianExpertForms", typeof(bool), typeof(Typography), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///Variants Property public static readonly DependencyProperty VariantsProperty = DependencyProperty.RegisterAttached( "Variants", typeof(FontVariants), typeof(Typography), new FrameworkPropertyMetadata( FontVariants.Normal, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///Capitals Property public static readonly DependencyProperty CapitalsProperty = DependencyProperty.RegisterAttached( "Capitals", typeof(FontCapitals), typeof(Typography), new FrameworkPropertyMetadata( FontCapitals.Normal, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///NumeralStyle Property public static readonly DependencyProperty NumeralStyleProperty = DependencyProperty.RegisterAttached( "NumeralStyle", typeof(FontNumeralStyle), typeof(Typography), new FrameworkPropertyMetadata( FontNumeralStyle.Normal, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///NumeralAlignment Property public static readonly DependencyProperty NumeralAlignmentProperty = DependencyProperty.RegisterAttached( "NumeralAlignment", typeof(FontNumeralAlignment), typeof(Typography), new FrameworkPropertyMetadata( FontNumeralAlignment.Normal, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///EastAsianWidths Property public static readonly DependencyProperty EastAsianWidthsProperty = DependencyProperty.RegisterAttached( "EastAsianWidths", typeof(FontEastAsianWidths), typeof(Typography), new FrameworkPropertyMetadata( FontEastAsianWidths.Normal, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///EastAsianLanguage Property public static readonly DependencyProperty EastAsianLanguageProperty = DependencyProperty.RegisterAttached( "EastAsianLanguage", typeof(FontEastAsianLanguage), typeof(Typography), new FrameworkPropertyMetadata( FontEastAsianLanguage.Normal, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StandardSwashes Property public static readonly DependencyProperty StandardSwashesProperty = DependencyProperty.RegisterAttached( "StandardSwashes", typeof(int), typeof(Typography), new FrameworkPropertyMetadata( 0, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///ContextualSwashes Property public static readonly DependencyProperty ContextualSwashesProperty = DependencyProperty.RegisterAttached( "ContextualSwashes", typeof(int), typeof(Typography), new FrameworkPropertyMetadata( 0, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ///StylisticAlternates Property public static readonly DependencyProperty StylisticAlternatesProperty = DependencyProperty.RegisterAttached( "StylisticAlternates", typeof(int), typeof(Typography), new FrameworkPropertyMetadata( 0, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ////// List of properties in TypographyProperties group cache /// Actual metadata registered in FlowDocument, TextRange, Text elements /// internal static DependencyProperty[] TypographyPropertiesList = new DependencyProperty[] { StandardLigaturesProperty, ContextualLigaturesProperty, DiscretionaryLigaturesProperty, HistoricalLigaturesProperty, AnnotationAlternatesProperty, ContextualAlternatesProperty, HistoricalFormsProperty, KerningProperty, CapitalSpacingProperty, CaseSensitiveFormsProperty, StylisticSet1Property, StylisticSet2Property, StylisticSet3Property, StylisticSet4Property, StylisticSet5Property, StylisticSet6Property, StylisticSet7Property, StylisticSet8Property, StylisticSet9Property, StylisticSet10Property, StylisticSet11Property, StylisticSet12Property, StylisticSet13Property, StylisticSet14Property, StylisticSet15Property, StylisticSet16Property, StylisticSet17Property, StylisticSet18Property, StylisticSet19Property, StylisticSet20Property, FractionProperty, SlashedZeroProperty, MathematicalGreekProperty, EastAsianExpertFormsProperty, VariantsProperty, CapitalsProperty, NumeralStyleProperty, NumeralAlignmentProperty, EastAsianWidthsProperty, EastAsianLanguageProperty, StandardSwashesProperty, ContextualSwashesProperty, StylisticAlternatesProperty }; #endregion Dependency Properties // ------------------------------------------------------------------ // Internal cache for default property groups. // ----------------------------------------------------------------- internal static readonly TypographyProperties Default = new TypographyProperties(); private DependencyObject _owner; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
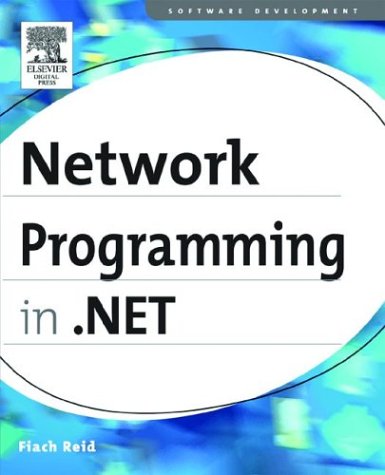
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetroSerializationManager.cs
- ScriptResourceAttribute.cs
- XmlSchemaElement.cs
- SafeSystemMetrics.cs
- EllipticalNodeOperations.cs
- TransactionManager.cs
- XslTransformFileEditor.cs
- SqlReferenceCollection.cs
- X509Certificate2.cs
- SHA512Cng.cs
- Crypto.cs
- AddInProcess.cs
- XmlSyndicationContent.cs
- NativeMethods.cs
- ClrPerspective.cs
- MetadataArtifactLoaderCompositeFile.cs
- CompressedStack.cs
- DatasetMethodGenerator.cs
- OdbcUtils.cs
- ListSortDescription.cs
- CodeAccessPermission.cs
- DictionaryEditChange.cs
- XPathExpr.cs
- InvalidCommandTreeException.cs
- DiscoveryRequestHandler.cs
- ProxyGenerationError.cs
- ToolboxItem.cs
- DataGridViewButtonColumn.cs
- printdlgexmarshaler.cs
- ScriptResourceAttribute.cs
- XmlCollation.cs
- IdentityReference.cs
- XhtmlCssHandler.cs
- ControlPropertyNameConverter.cs
- SubMenuStyleCollection.cs
- TreeBuilderXamlTranslator.cs
- ExpressionNode.cs
- ExitEventArgs.cs
- RuleProcessor.cs
- XmlDictionaryReaderQuotas.cs
- ElementAction.cs
- ComponentDesigner.cs
- PersonalizationDictionary.cs
- SafeCoTaskMem.cs
- BinHexDecoder.cs
- AccessText.cs
- IFlowDocumentViewer.cs
- WindowsClientElement.cs
- Activation.cs
- InputLanguageProfileNotifySink.cs
- Drawing.cs
- SimpleMailWebEventProvider.cs
- InstanceCreationEditor.cs
- Timeline.cs
- TiffBitmapEncoder.cs
- ZipIOExtraFieldZip64Element.cs
- Queue.cs
- Evaluator.cs
- SchemaInfo.cs
- TemplateColumn.cs
- PolyBezierSegmentFigureLogic.cs
- ConnectionPointCookie.cs
- GeometryGroup.cs
- CodeTypeParameterCollection.cs
- MultiPropertyDescriptorGridEntry.cs
- WebPartEditorApplyVerb.cs
- DesignerDataColumn.cs
- ServiceParser.cs
- Rotation3D.cs
- DateTimeStorage.cs
- BamlRecordReader.cs
- ResourceSet.cs
- GridPattern.cs
- SessionEndingEventArgs.cs
- TraceLevelStore.cs
- FacetChecker.cs
- ReaderContextStackData.cs
- ManipulationDevice.cs
- StylusButtonEventArgs.cs
- AssemblyResourceLoader.cs
- ReadWriteSpinLock.cs
- OptimizedTemplateContent.cs
- coordinator.cs
- ReliableChannelListener.cs
- NamespaceInfo.cs
- LineInfo.cs
- TabletCollection.cs
- SchemaComplexType.cs
- StrongNameUtility.cs
- SqlBulkCopyColumnMapping.cs
- WizardForm.cs
- SoapTypeAttribute.cs
- webproxy.cs
- TypeConstant.cs
- EventHandlersStore.cs
- SplitterPanel.cs
- ObsoleteAttribute.cs
- ListViewSelectEventArgs.cs
- DataKeyPropertyAttribute.cs
- XmlObjectSerializerWriteContextComplex.cs