Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / Xml / System / Xml / Core / CharEntityEncoderFallback.cs / 2 / CharEntityEncoderFallback.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Text; using System.Diagnostics; using System.Globalization; namespace System.Xml { // // CharEntityEncoderFallback // internal class CharEntityEncoderFallback : EncoderFallback { private CharEntityEncoderFallbackBuffer fallbackBuffer; private int[] textContentMarks; private int endMarkPos; private int curMarkPos; private int startOffset; internal CharEntityEncoderFallback() { } public override EncoderFallbackBuffer CreateFallbackBuffer() { if ( fallbackBuffer == null ) { fallbackBuffer = new CharEntityEncoderFallbackBuffer( this ); } return fallbackBuffer; } public override int MaxCharCount { get { return 12; } } internal int StartOffset { get { return startOffset; } set { startOffset = value; } } internal void Reset( int[] textContentMarks, int endMarkPos ) { this.textContentMarks = textContentMarks; this.endMarkPos = endMarkPos; curMarkPos = 0; } internal bool CanReplaceAt( int index ) { int mPos = curMarkPos; int charPos = startOffset + index; while ( mPos < endMarkPos && charPos >= textContentMarks[mPos+1] ) { mPos++; } curMarkPos = mPos; return (mPos & 1) != 0; } } // // CharEntityFallbackBuffer // internal class CharEntityEncoderFallbackBuffer : EncoderFallbackBuffer { private CharEntityEncoderFallback parent; private string charEntity = string.Empty; private int charEntityIndex = -1; internal CharEntityEncoderFallbackBuffer( CharEntityEncoderFallback parent ) { this.parent = parent; } public override bool Fallback( char charUnknown, int index ) { // If we are already in fallback, throw, it's probably at the suspect character in charEntity if ( charEntityIndex >= 0 ) { (new EncoderExceptionFallbackBuffer()).Fallback( charUnknown, index ); } // find out if we can replace the character with entity if ( parent.CanReplaceAt( index ) ) { // Create the replacement character entity charEntity = string.Format( CultureInfo.InvariantCulture, "{0:X};", new object[] { (int)charUnknown } ); charEntityIndex = 0; return true; } else { EncoderFallbackBuffer errorFallbackBuffer = ( new EncoderExceptionFallback() ).CreateFallbackBuffer(); errorFallbackBuffer.Fallback( charUnknown, index ); return false; } } public override bool Fallback( char charUnknownHigh, char charUnknownLow, int index ) { // if ( !char.IsSurrogatePair( charUnknownHigh, charUnknownLow ) ) { throw XmlConvert.CreateInvalidSurrogatePairException( charUnknownHigh, charUnknownLow ); } // If we are already in fallback, throw, it's probably at the suspect character in charEntity if ( charEntityIndex >= 0 ) { (new EncoderExceptionFallbackBuffer()).Fallback( charUnknownHigh, charUnknownLow, index ); } if ( parent.CanReplaceAt( index ) ) { // Create the replacement character entity charEntity = string.Format( CultureInfo.InvariantCulture, "{0:X};", new object[] { char.ConvertToUtf32( charUnknownHigh, charUnknownLow ) } ); charEntityIndex = 0; return true; } else { EncoderFallbackBuffer errorFallbackBuffer = ( new EncoderExceptionFallback() ).CreateFallbackBuffer(); errorFallbackBuffer.Fallback( charUnknownHigh, charUnknownLow, index ); return false; } } public override char GetNextChar() { if ( charEntityIndex == -1 ) { return (char)0; } else { Debug.Assert( charEntityIndex < charEntity.Length ); char ch = charEntity[charEntityIndex++]; if ( charEntityIndex == charEntity.Length ) { charEntityIndex = -1; } return ch; } } public override bool MovePrevious() { if ( charEntityIndex == -1 ) { return false; } else { Debug.Assert( charEntityIndex < charEntity.Length ); if ( charEntityIndex > 0 ) { charEntityIndex--; return true; } else { return false; } } } public override int Remaining { get { if ( charEntityIndex == -1 ) { return 0; } else { return charEntity.Length - charEntityIndex; } } } public override void Reset() { charEntityIndex = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
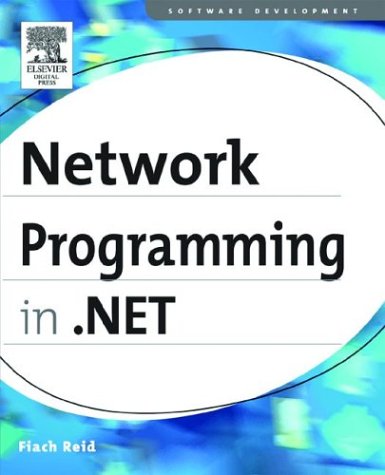
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartDisplayModeCollection.cs
- Rect.cs
- webbrowsersite.cs
- StickyNote.cs
- CompositeControl.cs
- SmiXetterAccessMap.cs
- UnauthorizedWebPart.cs
- StringInfo.cs
- XmlAttributeOverrides.cs
- DictionaryEntry.cs
- FolderNameEditor.cs
- Timer.cs
- ReadOnlyHierarchicalDataSourceView.cs
- CodeTypeDeclarationCollection.cs
- ControlIdConverter.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- MediaPlayerState.cs
- OrElse.cs
- DataPagerFieldItem.cs
- _WinHttpWebProxyDataBuilder.cs
- RuntimeHandles.cs
- XmlDataImplementation.cs
- BitmapMetadata.cs
- CodeTypeReferenceExpression.cs
- GuidelineSet.cs
- OutputCacheSettingsSection.cs
- XmlDeclaration.cs
- SecurityManager.cs
- MeasureItemEvent.cs
- CopyEncoder.cs
- ObjectDataSourceDisposingEventArgs.cs
- TypedServiceChannelBuilder.cs
- ResumeStoryboard.cs
- Point3DValueSerializer.cs
- IntSecurity.cs
- IisTraceListener.cs
- MultiSelectRootGridEntry.cs
- HttpRuntime.cs
- Ipv6Element.cs
- ListItemConverter.cs
- CodeTypeMember.cs
- transactioncontext.cs
- BypassElement.cs
- OleDbError.cs
- AttributeProviderAttribute.cs
- Vector3DKeyFrameCollection.cs
- EventNotify.cs
- WebPartAuthorizationEventArgs.cs
- PanelStyle.cs
- SecondaryViewProvider.cs
- VectorConverter.cs
- AppDomainAttributes.cs
- TextEndOfSegment.cs
- ListSortDescription.cs
- VariableModifiersHelper.cs
- WsatServiceAddress.cs
- RegexWorker.cs
- ConfigXmlSignificantWhitespace.cs
- ObjectDataProvider.cs
- CacheMemory.cs
- RecordsAffectedEventArgs.cs
- DefaultBindingPropertyAttribute.cs
- ProgressBarBrushConverter.cs
- securitycriticaldataClass.cs
- ObjectItemCachedAssemblyLoader.cs
- PropertyTabAttribute.cs
- ImageMap.cs
- ViewgenGatekeeper.cs
- PrivilegeNotHeldException.cs
- WebChannelFactory.cs
- PropertyEmitterBase.cs
- SafeIUnknown.cs
- ObjectQueryState.cs
- FieldNameLookup.cs
- XamlSerializationHelper.cs
- WinFormsUtils.cs
- HttpApplicationFactory.cs
- QuestionEventArgs.cs
- Border.cs
- NavigationExpr.cs
- DefaultSection.cs
- DataControlCommands.cs
- DSGeneratorProblem.cs
- ExeContext.cs
- SqlRowUpdatedEvent.cs
- LoginUtil.cs
- TimelineGroup.cs
- webproxy.cs
- DataGridToolTip.cs
- ImageSourceValueSerializer.cs
- WebMessageBodyStyleHelper.cs
- InfocardExtendedInformationCollection.cs
- InlineUIContainer.cs
- SqlDataSourceConfigureFilterForm.cs
- DropShadowEffect.cs
- WmpBitmapDecoder.cs
- GetReadStreamResult.cs
- AggregateException.cs
- XmlWrappingWriter.cs
- UrlMappingsModule.cs