Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / IO / compression / CopyEncoder.cs / 1305376 / CopyEncoder.cs
namespace System.IO.Compression { using System.Diagnostics; internal class CopyEncoder { // padding for copy encoder formatting // - 1 byte for header // - 4 bytes for len, nlen private const int PaddingSize = 5; // max uncompressed deflate block size is 64K. private const int MaxUncompressedBlockSize = 65536; // null input means write an empty payload with formatting info. This is needed for the final block. public void GetBlock(DeflateInput input, OutputBuffer output, bool isFinal) { Debug.Assert(output != null); Debug.Assert(output.FreeBytes >= PaddingSize); // determine number of bytes to write int count = 0; if (input != null) { // allow space for padding and bits not yet flushed to buffer count = Math.Min(input.Count, output.FreeBytes - PaddingSize - output.BitsInBuffer); // we don't expect the output buffer to ever be this big (currently 4K), but we'll check this // just in case that changes. if (count > MaxUncompressedBlockSize - PaddingSize) { count = MaxUncompressedBlockSize - PaddingSize; } } // write header and flush bits if (isFinal) { output.WriteBits(FastEncoderStatics.BFinalNoCompressionHeaderBitCount, FastEncoderStatics.BFinalNoCompressionHeader); } else { output.WriteBits(FastEncoderStatics.NoCompressionHeaderBitCount, FastEncoderStatics.NoCompressionHeader); } // now we're aligned output.FlushBits(); // write len, nlen WriteLenNLen((ushort)count, output); // write uncompressed bytes if (input != null && count > 0) { output.WriteBytes(input.Buffer, input.StartIndex, count); input.ConsumeBytes(count); } } private void WriteLenNLen(ushort len, OutputBuffer output) { // len output.WriteUInt16(len); // nlen ushort onesComp = (ushort)(~(ushort)len); output.WriteUInt16(onesComp); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
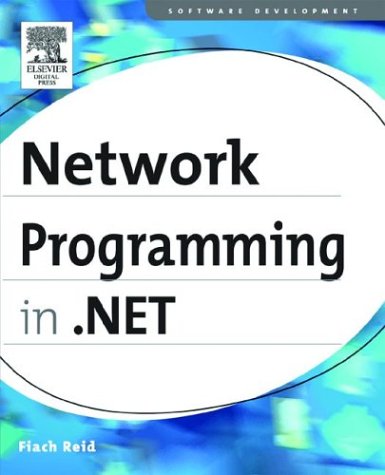
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TaskHelper.cs
- SelectedDatesCollection.cs
- ValidationHelpers.cs
- ObjectListField.cs
- GridView.cs
- TextServicesContext.cs
- dsa.cs
- WrappedReader.cs
- ListViewInsertionMark.cs
- WebBrowserUriTypeConverter.cs
- RawAppCommandInputReport.cs
- LayoutExceptionEventArgs.cs
- DebuggerService.cs
- WinEventTracker.cs
- CodeAccessSecurityEngine.cs
- NamedPipeProcessProtocolHandler.cs
- DrawListViewSubItemEventArgs.cs
- ResourceProperty.cs
- LightweightCodeGenerator.cs
- DbDataReader.cs
- XmlAttributeAttribute.cs
- UIElementPropertyUndoUnit.cs
- InstanceCollisionException.cs
- PreviewPageInfo.cs
- ComponentDispatcher.cs
- JulianCalendar.cs
- DataGridViewControlCollection.cs
- handlecollector.cs
- StandardOleMarshalObject.cs
- DataListDesigner.cs
- XPathSelfQuery.cs
- ToolStripItemClickedEventArgs.cs
- CodeMemberMethod.cs
- DataStreamFromComStream.cs
- EventLogWatcher.cs
- KeyedCollection.cs
- DBCommand.cs
- ProfileBuildProvider.cs
- Hashtable.cs
- StickyNoteAnnotations.cs
- SmtpNtlmAuthenticationModule.cs
- SmtpFailedRecipientException.cs
- Scanner.cs
- EtwTrackingBehaviorElement.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- TableCellAutomationPeer.cs
- CardSpacePolicyElement.cs
- DataObjectPastingEventArgs.cs
- QueryContinueDragEvent.cs
- DynamicDataRouteHandler.cs
- DnsElement.cs
- BoolLiteral.cs
- SqlReorderer.cs
- CompModSwitches.cs
- SymLanguageType.cs
- RuntimeResourceSet.cs
- ListSourceHelper.cs
- EventSourceCreationData.cs
- PermissionSetTriple.cs
- TextTreeFixupNode.cs
- EntityDataSourceViewSchema.cs
- Msmq4SubqueuePoisonHandler.cs
- AsyncStreamReader.cs
- BindingsSection.cs
- ConditionCollection.cs
- CodeLabeledStatement.cs
- _KerberosClient.cs
- ConstraintStruct.cs
- Rect3D.cs
- TableColumnCollection.cs
- RightsManagementEncryptedStream.cs
- UnsafeNativeMethods.cs
- ReferenceEqualityComparer.cs
- AttributeSetAction.cs
- InternalDispatchObject.cs
- _LocalDataStore.cs
- AliasGenerator.cs
- AssemblyInfo.cs
- Calendar.cs
- TextProperties.cs
- PlaceHolder.cs
- GatewayDefinition.cs
- FunctionNode.cs
- SoapProtocolReflector.cs
- AdjustableArrowCap.cs
- XmlReaderSettings.cs
- QueryModel.cs
- ProjectionRewriter.cs
- ModelItemDictionaryImpl.cs
- WorkflowRuntimeServiceElement.cs
- IDictionary.cs
- DesignerWebPartChrome.cs
- HotSpotCollectionEditor.cs
- FontCollection.cs
- ModuleBuilderData.cs
- SQLString.cs
- FullTrustAssemblyCollection.cs
- WSDualHttpSecurity.cs
- AppDomain.cs
- AssertUtility.cs