Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / DataStreamFromComStream.cs / 1 / DataStreamFromComStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.IO; ////// /// /// internal class DataStreamFromComStream : Stream { private UnsafeNativeMethods.IStream comStream; public DataStreamFromComStream(UnsafeNativeMethods.IStream comStream) : base() { this.comStream = comStream; } public override long Position { get { return Seek(0, SeekOrigin.Current); } set { Seek(value, SeekOrigin.Begin); } } public override bool CanWrite { get { return true; } } public override bool CanSeek { get { return true; } } public override bool CanRead { get { return true; } } public override long Length { get { long curPos = this.Position; long endPos = Seek(0, SeekOrigin.End); this.Position = curPos; return endPos - curPos; } } /* private void _NotImpl(string message) { NotSupportedException ex = new NotSupportedException(message, new ExternalException(SR.GetString(SR.ExternalException), NativeMethods.E_NOTIMPL)); throw ex; } */ private unsafe int _Read(void* handle, int bytes) { return comStream.Read((IntPtr)handle, bytes); } private unsafe int _Write(void* handle, int bytes) { return comStream.Write((IntPtr)handle, bytes); } public override void Flush() { } public unsafe override int Read(byte[] buffer, int index, int count) { int bytesRead = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { fixed (byte* ch = buffer) { bytesRead = _Read((void*)(ch + index), count); } } return bytesRead; } public override void SetLength(long value) { comStream.SetSize(value); } public override long Seek(long offset, SeekOrigin origin) { return comStream.Seek(offset, (int)origin); } public unsafe override void Write(byte[] buffer, int index, int count) { int bytesWritten = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { try { fixed (byte* b = buffer) { bytesWritten = _Write((void*)(b + index), count); } } catch { } } if (bytesWritten < count) { throw new IOException(SR.GetString(SR.DataStreamWrite)); } } protected override void Dispose(bool disposing) { try { if (disposing && comStream != null) { try { comStream.Commit(NativeMethods.STGC_DEFAULT); } catch(Exception) { } } // Can't release a COM stream from the finalizer thread. comStream = null; } finally { base.Dispose(disposing); } } ~DataStreamFromComStream() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.IO; ////// /// /// internal class DataStreamFromComStream : Stream { private UnsafeNativeMethods.IStream comStream; public DataStreamFromComStream(UnsafeNativeMethods.IStream comStream) : base() { this.comStream = comStream; } public override long Position { get { return Seek(0, SeekOrigin.Current); } set { Seek(value, SeekOrigin.Begin); } } public override bool CanWrite { get { return true; } } public override bool CanSeek { get { return true; } } public override bool CanRead { get { return true; } } public override long Length { get { long curPos = this.Position; long endPos = Seek(0, SeekOrigin.End); this.Position = curPos; return endPos - curPos; } } /* private void _NotImpl(string message) { NotSupportedException ex = new NotSupportedException(message, new ExternalException(SR.GetString(SR.ExternalException), NativeMethods.E_NOTIMPL)); throw ex; } */ private unsafe int _Read(void* handle, int bytes) { return comStream.Read((IntPtr)handle, bytes); } private unsafe int _Write(void* handle, int bytes) { return comStream.Write((IntPtr)handle, bytes); } public override void Flush() { } public unsafe override int Read(byte[] buffer, int index, int count) { int bytesRead = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { fixed (byte* ch = buffer) { bytesRead = _Read((void*)(ch + index), count); } } return bytesRead; } public override void SetLength(long value) { comStream.SetSize(value); } public override long Seek(long offset, SeekOrigin origin) { return comStream.Seek(offset, (int)origin); } public unsafe override void Write(byte[] buffer, int index, int count) { int bytesWritten = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { try { fixed (byte* b = buffer) { bytesWritten = _Write((void*)(b + index), count); } } catch { } } if (bytesWritten < count) { throw new IOException(SR.GetString(SR.DataStreamWrite)); } } protected override void Dispose(bool disposing) { try { if (disposing && comStream != null) { try { comStream.Commit(NativeMethods.STGC_DEFAULT); } catch(Exception) { } } // Can't release a COM stream from the finalizer thread. comStream = null; } finally { base.Dispose(disposing); } } ~DataStreamFromComStream() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
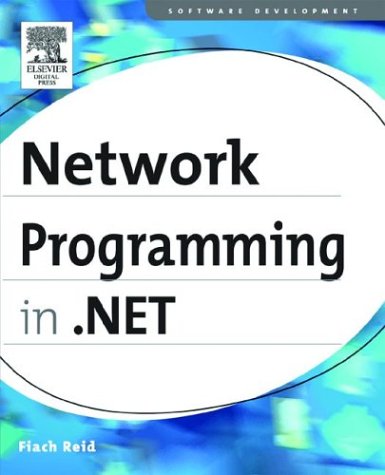
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaMapping.cs
- TransactionChannelListener.cs
- GridToolTip.cs
- DockingAttribute.cs
- OptimizerPatterns.cs
- EndpointAddressMessageFilter.cs
- CrossSiteScriptingValidation.cs
- NavigationProgressEventArgs.cs
- CustomCredentialPolicy.cs
- TextTreeDeleteContentUndoUnit.cs
- HitTestFilterBehavior.cs
- WindowsFormsSectionHandler.cs
- DataGridViewCellValidatingEventArgs.cs
- SqlMethods.cs
- HandlerBase.cs
- AppModelKnownContentFactory.cs
- ListViewTableCell.cs
- Lazy.cs
- GeometryDrawing.cs
- EntitySet.cs
- CmsInterop.cs
- WebResourceAttribute.cs
- SecurityUtils.cs
- UnsafeNetInfoNativeMethods.cs
- EventRouteFactory.cs
- MimeWriter.cs
- DataBinding.cs
- DesignerAttributeInfo.cs
- MediaScriptCommandRoutedEventArgs.cs
- XamlStackWriter.cs
- ToolboxComponentsCreatingEventArgs.cs
- BulletedList.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- AppliedDeviceFiltersEditor.cs
- RegionData.cs
- DetailsViewRowCollection.cs
- UIElementHelper.cs
- WebPartUserCapability.cs
- XPathConvert.cs
- KernelTypeValidation.cs
- PersonalizablePropertyEntry.cs
- ChangeTracker.cs
- ParagraphVisual.cs
- TargetInvocationException.cs
- ButtonRenderer.cs
- OdbcRowUpdatingEvent.cs
- CultureTable.cs
- CommunicationObjectFaultedException.cs
- FontClient.cs
- WindowsRebar.cs
- AsmxEndpointPickerExtension.cs
- HttpBrowserCapabilitiesBase.cs
- OneOfElement.cs
- ReadContentAsBinaryHelper.cs
- EntitySetBase.cs
- TargetException.cs
- HandleTable.cs
- VerificationAttribute.cs
- OdbcParameterCollection.cs
- ServiceObjectContainer.cs
- XmlDataSourceView.cs
- ListBoxChrome.cs
- BitVector32.cs
- ImageInfo.cs
- AddInControllerImpl.cs
- ResourcePool.cs
- TreeNodeMouseHoverEvent.cs
- NumericUpDownAccelerationCollection.cs
- CaretElement.cs
- SimpleTextLine.cs
- PropertyValueUIItem.cs
- SvcMapFile.cs
- MaskedTextProvider.cs
- LayoutSettings.cs
- Nodes.cs
- TimelineGroup.cs
- DataServiceContext.cs
- RoleService.cs
- ImageMapEventArgs.cs
- WorkflowIdleBehavior.cs
- SupportingTokenAuthenticatorSpecification.cs
- XmlMembersMapping.cs
- DynamicControl.cs
- _IPv4Address.cs
- SimpleApplicationHost.cs
- PrintPreviewControl.cs
- Rfc4050KeyFormatter.cs
- ConfigurationProperty.cs
- ServiceControllerDesigner.cs
- HandleExceptionArgs.cs
- RegisteredDisposeScript.cs
- GcSettings.cs
- RegexReplacement.cs
- ArgumentElement.cs
- RangeValuePatternIdentifiers.cs
- GB18030Encoding.cs
- FocusManager.cs
- ArrangedElementCollection.cs
- OrderByExpression.cs
- ImageListStreamer.cs