Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Hosting / SimpleApplicationHost.cs / 1305376 / SimpleApplicationHost.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Hosting { using System; using System.Collections; using System.Configuration; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Web; using System.Web.Configuration; using System.Web.UI; using System.Web.Util; using Microsoft.Win32; using Debug = System.Web.Util.Debug; internal class SimpleApplicationHost : MarshalByRefObject, IApplicationHost { private VirtualPath _appVirtualPath; private String _appPhysicalPath; internal SimpleApplicationHost(VirtualPath virtualPath, string physicalPath) { if (String.IsNullOrEmpty(physicalPath)) throw ExceptionUtil.ParameterNullOrEmpty("physicalPath"); // Throw if the physical path is not canonical, to prevent potential // security issues (VSWhidbey 418125) if (FileUtil.IsSuspiciousPhysicalPath(physicalPath)) { throw ExceptionUtil.ParameterInvalid(physicalPath); } _appVirtualPath = virtualPath; _appPhysicalPath = StringUtil.StringEndsWith(physicalPath, "\\") ? physicalPath : physicalPath + "\\"; } public override Object InitializeLifetimeService() { return null; // never expire lease } // IApplicationHost implementation public string GetVirtualPath() { return _appVirtualPath.VirtualPathString; } String IApplicationHost.GetPhysicalPath() { return _appPhysicalPath; } IConfigMapPathFactory IApplicationHost.GetConfigMapPathFactory() { return new SimpleConfigMapPathFactory(); } IntPtr IApplicationHost.GetConfigToken() { return IntPtr.Zero; } String IApplicationHost.GetSiteName() { return WebConfigurationHost.DefaultSiteName; } String IApplicationHost.GetSiteID() { return WebConfigurationHost.DefaultSiteID; } public void MessageReceived() { // nothing } } [Serializable()] internal class SimpleConfigMapPathFactory : IConfigMapPathFactory { IConfigMapPath IConfigMapPathFactory.Create(string virtualPath, string physicalPath) { WebConfigurationFileMap webFileMap = new WebConfigurationFileMap(); VirtualPath vpath = VirtualPath.Create(virtualPath); // Application path webFileMap.VirtualDirectories.Add(vpath.VirtualPathStringNoTrailingSlash, new VirtualDirectoryMapping(physicalPath, true)); // Client script file path webFileMap.VirtualDirectories.Add( HttpRuntime.AspClientScriptVirtualPath, new VirtualDirectoryMapping(HttpRuntime.AspClientScriptPhysicalPathInternal, false)); return new UserMapPath(webFileMap); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
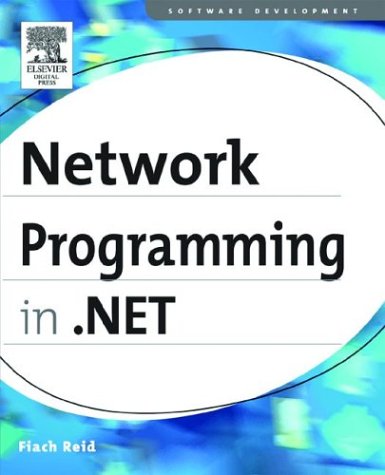
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XsltLoader.cs
- SubpageParaClient.cs
- OSFeature.cs
- SecurityHeader.cs
- JsonReaderDelegator.cs
- ListViewItemEventArgs.cs
- DESCryptoServiceProvider.cs
- AtomContentProperty.cs
- GlyphsSerializer.cs
- XmlAttributes.cs
- FormsAuthenticationCredentials.cs
- ApplicationInfo.cs
- ValidationErrorEventArgs.cs
- ECDsa.cs
- GroupItem.cs
- FixedPage.cs
- BinaryQueryOperator.cs
- PageHandlerFactory.cs
- XmlSchemaAnnotation.cs
- HtmlElementEventArgs.cs
- XmlDictionaryString.cs
- TextProperties.cs
- FormViewModeEventArgs.cs
- XmlValidatingReader.cs
- XmlSchemaRedefine.cs
- dbdatarecord.cs
- PropertyOrder.cs
- unsafenativemethodsother.cs
- TextServicesCompartmentEventSink.cs
- EntityStoreSchemaGenerator.cs
- StreamSecurityUpgradeAcceptor.cs
- ErrorEventArgs.cs
- KeySplineConverter.cs
- VectorCollectionConverter.cs
- SQLDecimal.cs
- LinqDataSourceStatusEventArgs.cs
- XmlSchemaSubstitutionGroup.cs
- DependencyPropertyConverter.cs
- XmlSchemaSimpleTypeUnion.cs
- StaticExtension.cs
- Single.cs
- ComplexTypeEmitter.cs
- WinEventQueueItem.cs
- TabControl.cs
- Point3DIndependentAnimationStorage.cs
- SQLBinary.cs
- CutCopyPasteHelper.cs
- Button.cs
- DataReceivedEventArgs.cs
- ObjectCloneHelper.cs
- MD5CryptoServiceProvider.cs
- SoapFault.cs
- UriScheme.cs
- TransformCollection.cs
- TranslateTransform3D.cs
- LockRecursionException.cs
- HtmlElement.cs
- ResXResourceWriter.cs
- HttpCachePolicyElement.cs
- CheckableControlBaseAdapter.cs
- Button.cs
- RelationshipEndCollection.cs
- HttpCapabilitiesSectionHandler.cs
- XmlSerializer.cs
- MulticastOption.cs
- DocComment.cs
- WebPartDescriptionCollection.cs
- StateItem.cs
- XpsFixedPageReaderWriter.cs
- CoreSwitches.cs
- Lease.cs
- HotSpot.cs
- VectorCollectionValueSerializer.cs
- DependencyPropertyConverter.cs
- SourceCollection.cs
- GAC.cs
- ProtocolsSection.cs
- HttpRawResponse.cs
- ConditionalBranch.cs
- PenThreadWorker.cs
- BulletDecorator.cs
- RegistryPermission.cs
- ObjectDataSourceEventArgs.cs
- ProtocolViolationException.cs
- FormParameter.cs
- Positioning.cs
- ValidationHelper.cs
- FontFamilyValueSerializer.cs
- WebConfigurationHostFileChange.cs
- PriorityQueue.cs
- SqlDataReader.cs
- ResolveCriteria.cs
- MailMessageEventArgs.cs
- SchemaMerger.cs
- PageMediaType.cs
- XmlEventCache.cs
- DataList.cs
- Panel.cs
- CodeDomSerializer.cs
- SafeNativeMethodsMilCoreApi.cs