Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / WebControls / Expressions / OrderByExpression.cs / 1305376 / OrderByExpression.cs
#if ORYX_VNEXT namespace Microsoft.Web.Data.UI.WebControls.Expressions { using System.Web; using System.Web.UI.WebControls; #else namespace System.Web.UI.WebControls.Expressions { #endif using System; using System.Collections.ObjectModel; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Web.Resources; using System.Web.UI; [ PersistChildren(false), ParseChildren(true, "ThenByExpressions") ] public class OrderByExpression : DataSourceExpression { private const string OrderByMethod = "OrderBy"; private const string ThenByMethod = "ThenBy"; private const string OrderDescendingByMethod = "OrderByDescending"; private const string ThenDescendingByMethod = "ThenByDescending"; private Collection_thenByExpressions; public string DataField { get { return (string)ViewState["DataField"] ?? String.Empty; } set { ViewState["DataField"] = value; } } public SortDirection Direction { get { object o = ViewState["Direction"]; return o != null ? (SortDirection)o : SortDirection.Ascending; } set { ViewState["Direction"] = value; } } [PersistenceMode(PersistenceMode.InnerDefaultProperty)] public Collection ThenByExpressions { get { if (_thenByExpressions == null) { // _thenByExpressions = new Collection (); } return _thenByExpressions; } } public override IQueryable GetQueryable(IQueryable source) { if (source == null) { return null; } if (String.IsNullOrEmpty(DataField)) { throw new InvalidOperationException(AtlasWeb.Expressions_DataFieldRequired); } ParameterExpression pe = Expression.Parameter(source.ElementType, String.Empty); source = CreateSortQueryable(source, pe, Direction, DataField, false /* isThenBy */); foreach (ThenBy thenBy in ThenByExpressions) { source = CreateSortQueryable(source, pe, thenBy.Direction, thenBy.DataField, true /* isThenBy */); } return source; } private static IQueryable CreateSortQueryable(IQueryable source, ParameterExpression parameterExpression, SortDirection direction, string dataField, bool isThenBy) { string methodName = isThenBy ? GetThenBySortMethod(direction) : GetSortMethod(direction); Expression propertyExpression = ExpressionHelper.CreatePropertyExpression(parameterExpression, dataField); return source.Call(methodName, Expression.Lambda(propertyExpression, parameterExpression), source.ElementType, propertyExpression.Type); } private static string GetSortMethod(SortDirection direction) { switch (direction) { case SortDirection.Ascending: return OrderByMethod; case SortDirection.Descending: return OrderDescendingByMethod; default: Debug.Fail("shouldn't get here!"); return OrderByMethod; } } private static string GetThenBySortMethod(SortDirection direction) { switch (direction) { case SortDirection.Ascending: return ThenByMethod; case SortDirection.Descending: return ThenDescendingByMethod; default: Debug.Fail("shouldn't get here!"); return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. #if ORYX_VNEXT namespace Microsoft.Web.Data.UI.WebControls.Expressions { using System.Web; using System.Web.UI.WebControls; #else namespace System.Web.UI.WebControls.Expressions { #endif using System; using System.Collections.ObjectModel; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Web.Resources; using System.Web.UI; [ PersistChildren(false), ParseChildren(true, "ThenByExpressions") ] public class OrderByExpression : DataSourceExpression { private const string OrderByMethod = "OrderBy"; private const string ThenByMethod = "ThenBy"; private const string OrderDescendingByMethod = "OrderByDescending"; private const string ThenDescendingByMethod = "ThenByDescending"; private Collection _thenByExpressions; public string DataField { get { return (string)ViewState["DataField"] ?? String.Empty; } set { ViewState["DataField"] = value; } } public SortDirection Direction { get { object o = ViewState["Direction"]; return o != null ? (SortDirection)o : SortDirection.Ascending; } set { ViewState["Direction"] = value; } } [PersistenceMode(PersistenceMode.InnerDefaultProperty)] public Collection ThenByExpressions { get { if (_thenByExpressions == null) { // _thenByExpressions = new Collection (); } return _thenByExpressions; } } public override IQueryable GetQueryable(IQueryable source) { if (source == null) { return null; } if (String.IsNullOrEmpty(DataField)) { throw new InvalidOperationException(AtlasWeb.Expressions_DataFieldRequired); } ParameterExpression pe = Expression.Parameter(source.ElementType, String.Empty); source = CreateSortQueryable(source, pe, Direction, DataField, false /* isThenBy */); foreach (ThenBy thenBy in ThenByExpressions) { source = CreateSortQueryable(source, pe, thenBy.Direction, thenBy.DataField, true /* isThenBy */); } return source; } private static IQueryable CreateSortQueryable(IQueryable source, ParameterExpression parameterExpression, SortDirection direction, string dataField, bool isThenBy) { string methodName = isThenBy ? GetThenBySortMethod(direction) : GetSortMethod(direction); Expression propertyExpression = ExpressionHelper.CreatePropertyExpression(parameterExpression, dataField); return source.Call(methodName, Expression.Lambda(propertyExpression, parameterExpression), source.ElementType, propertyExpression.Type); } private static string GetSortMethod(SortDirection direction) { switch (direction) { case SortDirection.Ascending: return OrderByMethod; case SortDirection.Descending: return OrderDescendingByMethod; default: Debug.Fail("shouldn't get here!"); return OrderByMethod; } } private static string GetThenBySortMethod(SortDirection direction) { switch (direction) { case SortDirection.Ascending: return ThenByMethod; case SortDirection.Descending: return ThenDescendingByMethod; default: Debug.Fail("shouldn't get here!"); return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
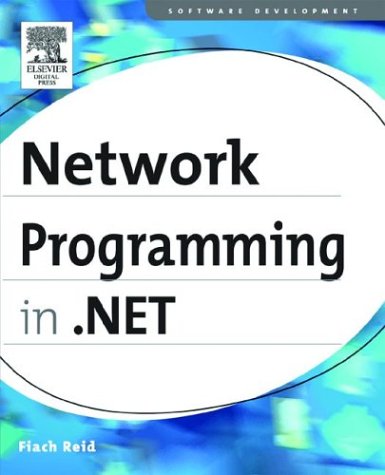
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CustomErrorsSectionWrapper.cs
- BamlRecords.cs
- WebPartCatalogCloseVerb.cs
- Expressions.cs
- XmlNode.cs
- QilPatternVisitor.cs
- BamlLocalizer.cs
- DefaultTraceListener.cs
- BaseCodePageEncoding.cs
- ConsumerConnectionPoint.cs
- HtmlShim.cs
- ScriptControlDescriptor.cs
- UncommonField.cs
- CompilationPass2TaskInternal.cs
- TextTreeInsertUndoUnit.cs
- EdmFunctionAttribute.cs
- VectorAnimation.cs
- TypeInfo.cs
- SQLChars.cs
- QilSortKey.cs
- FileChangesMonitor.cs
- OleDbException.cs
- InstanceLockLostException.cs
- SerializationSectionGroup.cs
- WebPartEditVerb.cs
- HttpAsyncResult.cs
- SymbolDocumentInfo.cs
- UIInitializationException.cs
- SoundPlayerAction.cs
- ShaderEffect.cs
- TemplatedMailWebEventProvider.cs
- cookieexception.cs
- ResourcesBuildProvider.cs
- Latin1Encoding.cs
- GetParentChain.cs
- AdobeCFFWrapper.cs
- ProfileInfo.cs
- BindingGraph.cs
- ZoneMembershipCondition.cs
- LowerCaseStringConverter.cs
- unsafeIndexingFilterStream.cs
- InstalledVoice.cs
- AccessibilityApplicationManager.cs
- PagesSection.cs
- Int32AnimationUsingKeyFrames.cs
- ImageMapEventArgs.cs
- DoWorkEventArgs.cs
- FontDifferentiator.cs
- DataBindingHandlerAttribute.cs
- MailAddressCollection.cs
- ClientBuildManagerCallback.cs
- Timer.cs
- AssemblyName.cs
- Converter.cs
- XmlText.cs
- ValuePattern.cs
- ResourceDescriptionAttribute.cs
- ServiceBehaviorElement.cs
- FontEmbeddingManager.cs
- HtmlInputCheckBox.cs
- StylusDevice.cs
- DataGridLinkButton.cs
- BatchStream.cs
- DragDropManager.cs
- UriScheme.cs
- TreeNodeStyleCollection.cs
- AsyncSerializedWorker.cs
- ResourceExpression.cs
- IgnoreDataMemberAttribute.cs
- DbConnectionHelper.cs
- ZipFileInfo.cs
- ToolStripSplitStackLayout.cs
- CompositionAdorner.cs
- PersistChildrenAttribute.cs
- ConnectionStringSettingsCollection.cs
- IdentityHolder.cs
- ContextInformation.cs
- _NestedMultipleAsyncResult.cs
- SafeFileMappingHandle.cs
- mediaeventshelper.cs
- SqlInfoMessageEvent.cs
- HandlerBase.cs
- HandleScope.cs
- StackSpiller.cs
- CompositeCollection.cs
- DataSourceControl.cs
- OperationContextScope.cs
- Accessors.cs
- SubqueryRules.cs
- ShaperBuffers.cs
- AssertSection.cs
- DeflateStream.cs
- HtmlForm.cs
- SchemaType.cs
- ClipboardProcessor.cs
- TcpClientCredentialType.cs
- ObjectDataSourceDisposingEventArgs.cs
- SqlDataRecord.cs
- MessagePartDescriptionCollection.cs
- HtmlInputButton.cs